Troubleshooting Custom Boolean Types in Hibernate JPA
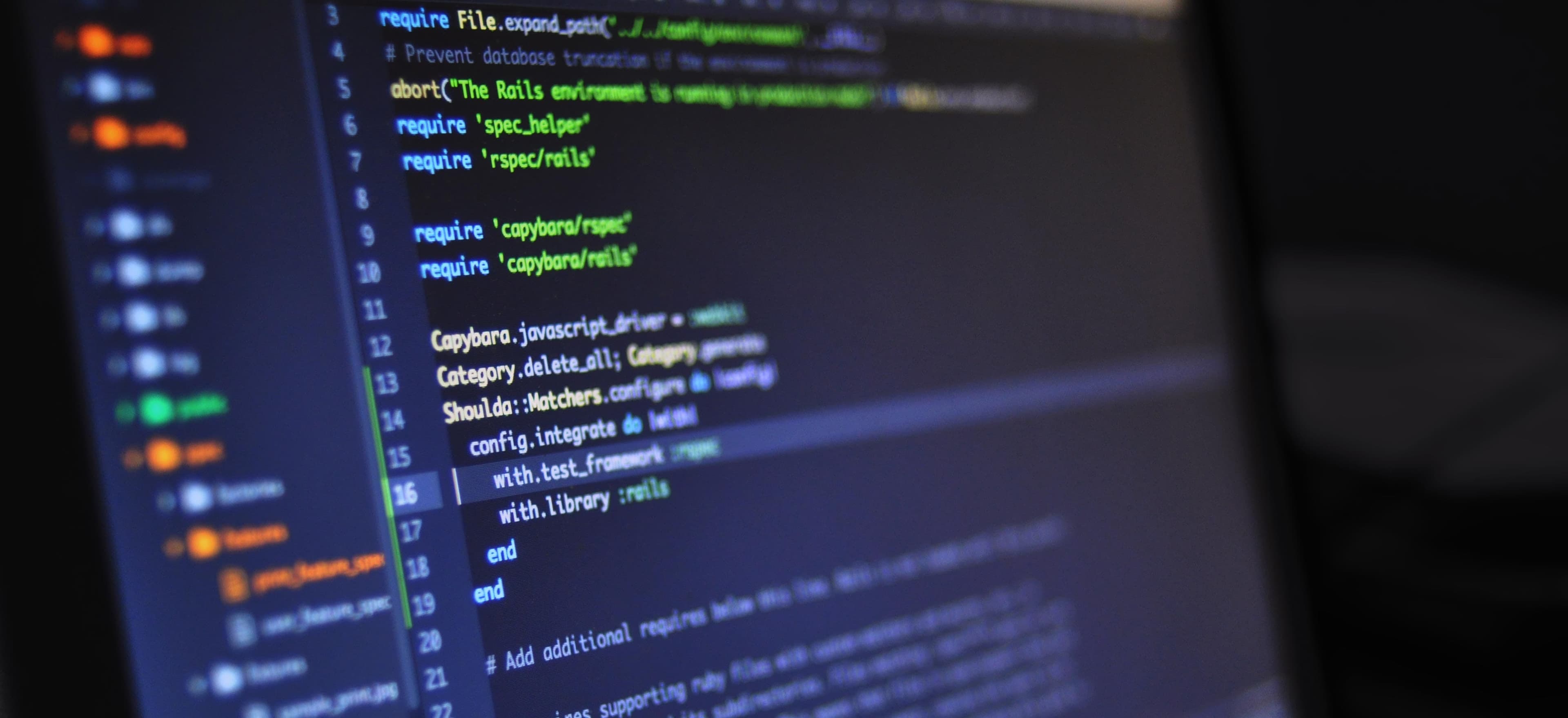
- Published on
Troubleshooting Custom Boolean Types in Hibernate JPA
When working with Hibernate and JPA (Java Persistence API), you may find yourself needing to use custom data types in your entity models. One common requirement is the manipulation of boolean types. While Java has a native boolean type, Hibernate offers the ability to create custom implementations which can be beneficial for various business logic and database design choices. In this post, we will explore how to troubleshoot and implement custom boolean types effectively in your Hibernate JPA applications.
Understanding Custom Boolean Types
Custom boolean types in Hibernate can be helpful when you want to handle cases that aren’t strictly true or false. Perhaps you need to represent states such as YES, NO, or UNKNOWN in your database, or you want to store the boolean value as an integer (0 for false, 1 for true) for compatibility reasons with legacy systems.
Why Custom Boolean Types?
- Database Compatibility: Some databases might not support boolean data types directly.
- Business Logic Representation: You might need additional states which a simple true/false cannot convey.
- Performance Optimization: Custom implementations may offer performance benefits based on your application’s specific requirements.
Implementing Custom Boolean Types in Hibernate
Before diving into troubleshooting, let's implement a basic example of a custom boolean type.
Step 1: Define Custom Boolean Type
First, create a BooleanType
class that implements UserType
. This will allow Hibernate to understand how to handle your custom boolean values.
import org.hibernate.engine.spi.SharedSessionContractImplementor;
import org.hibernate.usertype.UserType;
import java.io.Serializable;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Types;
public class BooleanType implements UserType {
@Override
public int[] sqlTypes() {
return new int[] { Types.INTEGER }; // Store as integer in DB
}
@Override
public Class<Boolean> returnedClass() {
return Boolean.class;
}
@Override
public boolean equals(Object x, Object y) {
return (x == y) || (x != null && x.equals(y));
}
@Override
public int hashCode(Object x) {
return x == null ? 0 : x.hashCode();
}
@Override
public Object nullSafeGet(ResultSet rs, String[] names, SharedSessionContractImplementor session, Object owner) throws SQLException {
int value = rs.getInt(names[0]);
return value == 1; // 1 represents true
}
@Override
public void nullSafeSet(PreparedStatement st, Object value, int index, SharedSessionContractImplementor session) throws SQLException {
st.setInt(index, (Boolean.TRUE.equals(value) ? 1 : 0)); // Set 1 for true and 0 for false
}
// Additional required methods can be implemented here
}
Why This Implementation?
The above implementation does the following:
-
Database Type: Uses
Types.INTEGER
to define how the custom boolean will be stored in the database. -
Value Conversion: In
nullSafeGet
, the method converts the integer from the database back into a boolean type. Similarly,nullSafeSet
converts the boolean into an integer for storage. -
Equality and Hashing: Methods like
equals
andhashCode
ensure the proper working of collections and caching.
Step 2: Annotate the Entity
With the custom type ready, apply it to a JPA entity:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import org.hibernate.annotations.Type;
@Entity
public class User {
@Id
@GeneratedValue
private Long id;
@Type(type = "com.yourpackage.BooleanType")
private Boolean active;
// Getters and Setters
}
Troubleshooting Common Issues
While working with custom boolean types, you might encounter various challenges. Below are some common issues and solutions.
Issue 1: Incorrect Data Being Retrieved
If you're getting unexpected boolean values (such as getting null
for active users), check how data is stored in the database. Make sure that:
- The column in the database is limited to acceptable values (0, 1).
- The
nullSafeGet
method accurately handles unexpected database values.
Solution
You can implement logging in nullSafeGet
for debugging.
@Override
public Object nullSafeGet(ResultSet rs, String[] names, SharedSessionContractImplementor session, Object owner) throws SQLException {
int value = rs.getInt(names[0]);
if (rs.wasNull()) {
return null; // Handle null from db
}
return value == 1; // Return proper boolean
}
This will help trace what values are actually retrieved from the database.
Issue 2: Hibernate Mapping Exceptions
If your application throws mapping exceptions, ensure that your custom type is correctly referenced. In the entity, you should:
- Use the full qualified class name for your type.
- Ensure that the custom type library is accessible on the classpath.
Solution
Instead of @Type(type = "com.yourpackage.BooleanType")
, consider using @org.hibernate.annotations.Type(type = "com.yourpackage.BooleanType")
, which explicitly tells Hibernate to use your custom mapping.
Issue 3: Mismatched Database Schema
If the database schema does not match the expected model, you may encounter various errors. Check the following:
- The database column type must match the expected definition in the
sqlTypes
method of yourUserType
implementation. - Ensure migrations are up to date.
Solution
Run a database migration check. Ensure that the column types align properly:
ALTER TABLE user MODIFY active INT; -- or your desired type
Final Considerations
Working with custom boolean types in Hibernate JPA can enhance your application's functionality and efficiency when properly implemented. However, you may face certain challenges. By understanding the critical aspects of UserType
implementations, database compatibility, and making sure your data is consistent, you can successfully troubleshoot and optimize your application.
For more references on Hibernate custom types, check out the Hibernate User Types Documentation.
We encourage continual learning and experimentation in Java development. Troubleshoot and refine your skills as you work with these powerful tools. Happy coding!
Checkout our other articles