Mastering Multipart Requests in Android HTTP Clients
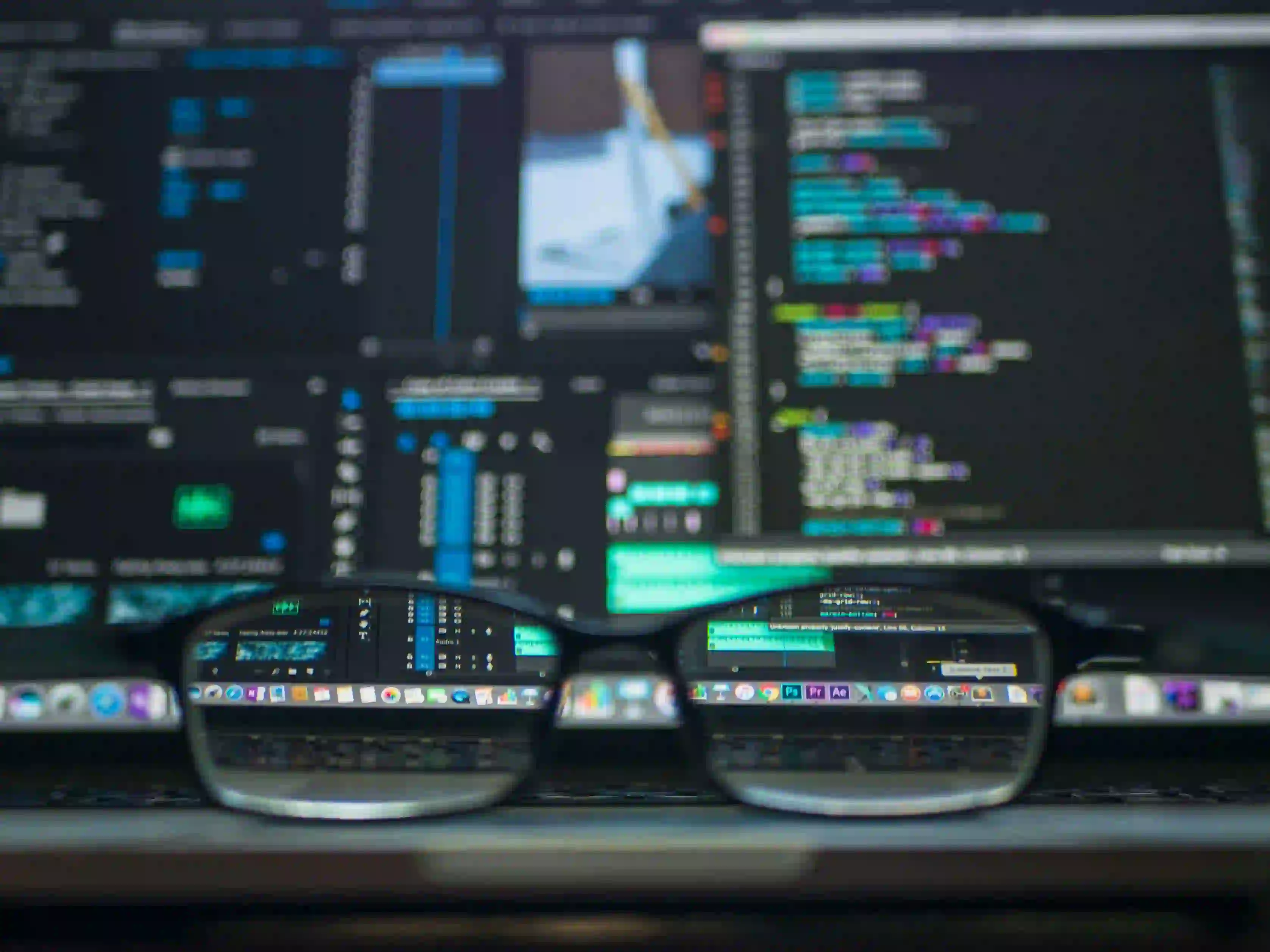
Mastering Multipart Requests in Android HTTP Clients
When developing applications for Android, handling files and images in HTTP requests is a common requirement. One of the most efficient ways to do this is through multipart requests. In this blog post, we'll delve into multipart requests, explain why they are essential, and provide examples to help you understand their practical application.
Understanding Multipart Requests
What is a Multipart Request?
A multipart request is an HTTP request that allows you to send files and data in one single request. It comprises multiple parts, each with its own content type and headers. This format is especially useful for uploading files like images, videos, and documents while simultaneously transmitting text data.
Why Use Multipart Requests?
- Efficient Uploading: Multipart requests can send multiple forms of data in a single request, reducing server load and network traffic.
- Flexibility: You can upload various files and data types in one request, making it ideal for forms that require file uploads alongside other data.
- Standard Protocol: Multipart requests adhere to the widely accepted MIME (Multipurpose Internet Mail Extensions) standards, ensuring compatibility across different platforms.
Setting Up Your Android Project
Before we dive into code examples, ensure that you have the necessary setup in your Android project.
- Add Dependencies: To manage HTTP requests easily, you can use libraries like Retrofit or OkHttp. Let's use Retrofit in this example.
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
implementation 'com.squareup.okhttp3:logging-interceptor:4.9.0'
}
Configuring Retrofit
To create a multipart request using Retrofit, first set up your Retrofit instance.
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
public class ApiClient {
private static final String BASE_URL = "https://yourapi.com/api/";
private static Retrofit retrofit = null;
public static Retrofit getClient() {
if (retrofit == null) {
retrofit = new Retrofit.Builder()
.baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.build();
}
return retrofit;
}
}
Creating the API Interface
Define the API endpoints in an interface. For a multipart upload, you'll use the @Multipart
annotation.
import okhttp3.MultipartBody;
import okhttp3.ResponseBody;
import retrofit2.Call;
import retrofit2.http.Multipart;
import retrofit2.http.POST;
import retrofit2.http.Part;
public interface ApiInterface {
@Multipart
@POST("upload")
Call<ResponseBody> uploadFile(
@Part MultipartBody.Part file,
@Part("description") RequestBody description);
}
Why Use @Multipart?
The @Multipart
annotation indicates that the request body will contain multiple parts. Each data segment must be individually annotated, allowing Retrofit to handle the data accurately.
Creating the Request Body
To upload files and data, you will also need to prepare your data using RequestBody
.
import okhttp3.MediaType;
import okhttp3.MultipartBody;
import okhttp3.RequestBody;
public void uploadFile(File file, String descriptionText) {
// Prepare the file to be uploaded
RequestBody requestFile = RequestBody.create(MediaType.parse("multipart/form-data"), file);
// Convert the file to MultipartBody.Part
MultipartBody.Part body = MultipartBody.Part.createFormData("file", file.getName(), requestFile);
// Prepare the description as RequestBody
RequestBody description = RequestBody.create(MediaType.parse("text/plain"), descriptionText);
// Call the upload service
ApiInterface apiInterface = ApiClient.getClient().create(ApiInterface.class);
Call<ResponseBody> call = apiInterface.uploadFile(body, description);
call.enqueue(new Callback<ResponseBody>() {
@Override
public void onResponse(Call<ResponseBody> call, Response<ResponseBody> response) {
if (response.isSuccessful()) {
// Request was successful
} else {
// Handle errors
}
}
@Override
public void onFailure(Call<ResponseBody> call, Throwable t) {
// Handle call failure
}
});
}
Handling Response
After executing the request, it's crucial to handle the server's response properly. This entails checking for success or failure and updating the UI accordingly.
@Override
public void onResponse(Call<ResponseBody> call, Response<ResponseBody> response) {
if (response.isSuccessful()) {
// The upload was successful
Log.d("Upload", "Success: " + response.body().string());
} else {
// The API returned an error code
Log.e("Upload", "Error: " + response.errorBody().string());
}
}
@Override
public void onFailure(Call<ResponseBody> call, Throwable t) {
// Something went wrong with the request itself
Log.e("Upload", "Failure: " + t.getMessage());
}
Important Considerations
Permissions
Make sure your application has the necessary permissions to access storage to read the files you want to upload. This requires adding storage permissions in the AndroidManifest.xml file.
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
Handling Large Files
When dealing with large files, you should consider providing user feedback, such as a progress bar. Retrofit does not natively support this for uploads but can be implemented with OkHttp.
Security
Always consider using HTTPS to secure your file uploads. Transmitting sensitive data over unsecured channels can lead to security breaches.
The Last Word
In this post, we explored multipart requests in Android and how to implement them using Retrofit. We showcased the importance of multipart requests for efficient file management and provided examples that illustrate the complete process from setup to handling responses.
For further reading, you might find the following resources helpful:
By mastering multipart requests in your Android applications, you will enhance user experience and application performance significantly. Happy coding!