Common Pitfalls in Aspect-Oriented Programming with Spring
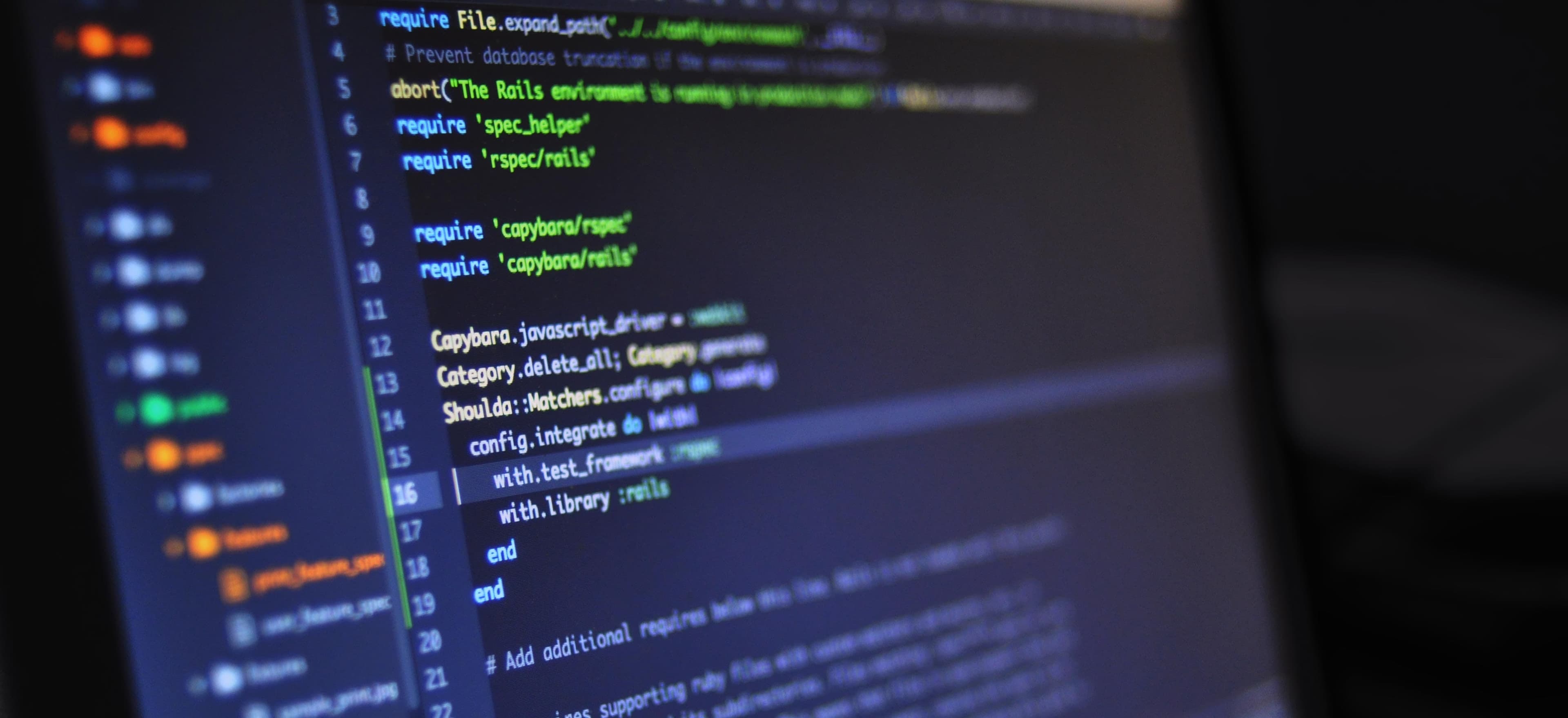
- Published on
Common Pitfalls in Aspect-Oriented Programming with Spring
Aspect-Oriented Programming (AOP) is a powerful paradigm that allows developers to separate cross-cutting concerns from primary business logic. When combined with Spring Framework, it can lead to more modular, maintainable, and cleaner code. However, if not used properly, AOP can introduce complexity and lead to unintended side effects. In this blog post, we will explore common pitfalls in Aspect-Oriented Programming specifically in the context of Spring, along with best practices to avoid them.
Understanding AOP in Spring
Before diving into the pitfalls, it's essential to grasp the core concepts of AOP in Spring. AOP allows you to define "aspects" - reusable modules that encapsulate specific functionalities like logging, security, and transaction management, among others. Spring AOP follows a proxy-based approach that applies "advice" – the additional behavior added to your code.
Key terms involved in Spring AOP include:
- Aspect: A module that cross-cuts multiple points in the program.
- Advice: Code that is executed at a certain join point (e.g., before or after method execution).
- Join Point: A specific point in the execution, like the execution of a method or a field access.
- Pointcut: An expression defining which join points an advice should be applied to.
Pitfall 1: Overusing AOP
What Happens?
One of the most common mistakes when using AOP in Spring is overusing it by applying aspects too liberally across the application. Developers may try to encapsulate every behavior as an aspect, leading to a cluttered codebase.
Why Avoid It?
When aspects are too numerous or too generic, they can reduce readability and make maintenance challenging. Debugging becomes a nightmare as it becomes hard to trace where certain behaviors are applied.
Key Takeaway
Limit the use of AOP to clear-cut cross-cutting concerns like logging, security checks, and transaction management. Each aspect should provide a significant benefit.
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logMethodAccess(JoinPoint joinPoint) {
System.out.println("Invoked: " + joinPoint.getSignature().getName());
}
}
Commentary
In the above code snippet, we define a logging aspect that logs method access within the service
package. Here, we’ve specifically targeted service methods, demonstrating a particular, well-defined use case of AOP.
Pitfall 2: Not Consideration Aspect Order
What Happens?
AOP in Spring allows multiple aspects, and if you do not carefully manage their order, you could end up with unexpected behavior. The order of execution of advice can affect application functionality especially when they are interacting.
Why Avoid It?
If two aspects are working on the same method, the order they are called could result in incorrect behaviors. For instance, logging might execute after a security check, but in some cases, you might want logging to run first.
Key Takeaway
You can control the execution order of multiple aspects by defining priorities. Use the @Order
annotation to specify the priority explicitly.
@Aspect
@Order(1)
public class SecurityAspect {
@Before("execution(* com.example.service.*.*(..))")
public void checkSecurity(JoinPoint joinPoint) {
// Security checks
System.out.println("Security check for: " + joinPoint.getSignature().getName());
}
}
Commentary
In this example, we define a security aspect and give it the highest priority, ensuring it gets executed before any other aspects, thus enforcing security checks before logs are created.
Pitfall 3: Misunderstanding Proxy-based AOP
What Happens?
Spring AOP is primarily proxy-based, which can lead to issues if you don’t understand how it works. Many developers assume that AOP applies to all objects equally, however, it only works on beans that are managed by the Spring container.
Why Avoid It?
When aspects are applied to objects not managed by Spring, they won't work as expected. If a class uses AOP and is instantiated directly without Spring, the advice won't be applied.
Key Takeaway
Only apply AOP to Spring beans. Use dependency injection to manage your objects.
@Component
public class MyService {
public void serve() {
System.out.println("Service method executed.");
}
}
//This will not have AOP applied
MyService myService = new MyService();
Commentary
To ensure AOP functions correctly, always rely on Spring-managed beans. If you need to use MyService outside of Spring’s context, consider re-evaluating your design.
Pitfall 4: Ignoring Performance Considerations
What Happens?
While AOP can help improve code management, excessive use of aspects may introduce performance overhead. This is especially true with complex pointcut expressions that need more processing during method invocation.
Why Avoid It?
Unmanaged overhead can degrade application performance, leading to slow response times and overall poor user experience.
Key Takeaway
Benchmark the application and understand the performance impact of your aspect closely. Focus on optimizing pointcut expressions.
@Aspect
@Component
public class PerformanceAspect {
@Around("execution(* com.example.service.*.*(..))")
public Object measureExecutionTime(ProceedingJoinPoint joinPoint) throws Throwable {
long startTime = System.currentTimeMillis();
Object returnValue = joinPoint.proceed(); // proceed to the target method
long endTime = System.currentTimeMillis();
System.out.println("Execution time for " + joinPoint.getSignature().getName() + ": " + (endTime - startTime) + "ms");
return returnValue;
}
}
Commentary
In the PerformanceAspect example, we use @Around
advice to measure execution time. While this does give useful metrics, evaluate its necessity depending on performance benchmarks.
Pitfall 5: Not Testing Aspects Thoroughly
What Happens?
When implementing AOP, developers often focus solely on business logic and forget to incorporate aspects into their test plans adequately. This failure can lead to undetected issues.
Why Avoid It?
Aspects can introduce modifications that affect functionality subtly. Incomplete tests may allow bugs to slip through, leading to production issues later.
Key Takeaway
Always include testing of your aspects. Unit tests should cover scenarios of aspects working as expected.
Example Unit Test
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = {AppConfig.class})
public class LoggingAspectTest {
@Autowired
private MyService myService; // Injecting Spring bean
@Test
public void testLoggingAspect() {
myService.serve(); // Should invoke the logMethodAccess method in LoggingAspect
// Verify log output through an appropriate method or framework
}
}
Commentary
In this unit test, we ensure that the log method is executed during myService.serve()
. This helps catch any bugs in logging or security aspects by validating their execution context.
The Closing Argument
Aspect-Oriented Programming can significantly enhance your Spring applications by promoting separation of concerns. However, as with any powerful tool, neglecting the common pitfalls we've discussed can lead to complications and inefficiencies.
To harness the true potential of AOP:
- Use it judiciously for clear concerns.
- Manage the execution order of aspects.
- Keep in mind the proxy nature of Spring AOP.
- Monitor performance impacts.
- Thoroughly test your aspects alongside your business logic.
By adhering to these advisable practices, you can ensure that your use of AOP remains effective and beneficial. For deep dives into AOP concepts, visit the Spring AOP Documentation for additional context. Happy coding!
This blog post should serve as both a guide and reference for developers looking to implement AOP in Spring more effectively while avoiding common pitfalls.
Checkout our other articles