Common Pitfalls in Basic Android Game Development
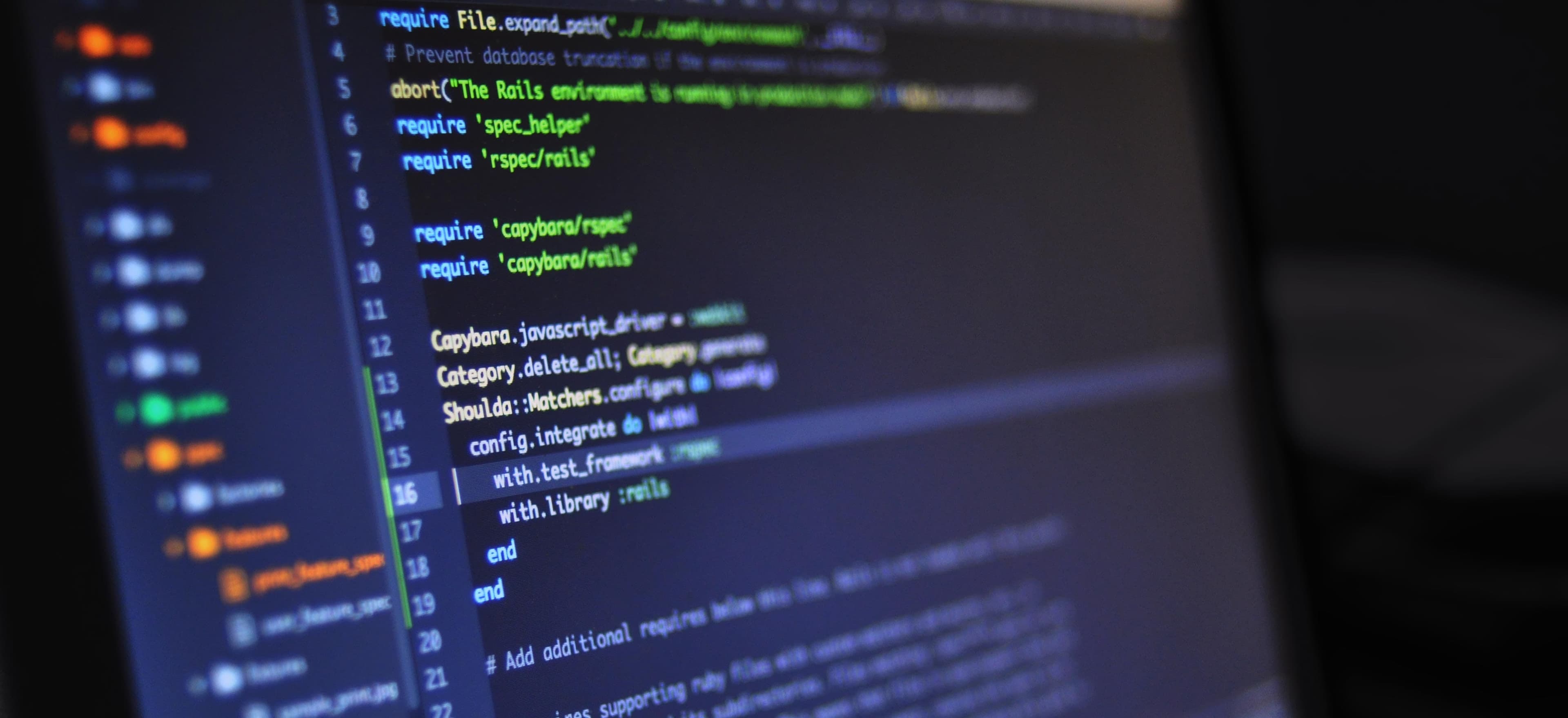
- Published on
Common Pitfalls in Basic Android Game Development
Developing games for the Android platform can be an exciting yet challenging endeavor. With the rise of mobile gaming, aspiring developers are eager to jump in, but many often trip over common pitfalls that can derail their projects. In this blog post, we will explore these pitfalls and provide practical solutions to enhance your Android game development experience.
1. Skipping the Game Design Document
What is a Game Design Document (GDD)?
A Game Design Document is essentially a blueprint for your game. It outlines the game’s mechanics, story, objectives, and resources needed.
Why You Shouldn’t Skip It
Many new developers skip this step, wishing to dive straight into coding. This might seem efficient, but without a GDD, you can lose sight of your goals. A well-structured document lays the foundation, saving you time and potential rework down the line.
# Sample Outline for a GDD
## Game Overview
- Title: Jumping Beans
- Genre: Action
- Platform: Android
## Gameplay Mechanics
- Player can jump to avoid obstacles
- Score system based on the distance traveled
Final Thoughts
Having a GDD forces you to think through your game's various aspects before you start coding, reducing confusion later in the process.
2. Focusing Too Much on Graphics
The Allure of Visuals
It can be tempting to pour all your resources into stunning graphics. While visuals are important, they should not be your primary focus.
Striking a Balance
Aim for a balance between graphics and gameplay. A visually appealing game can still flop if its core mechanics are weak. Many successful games have simple art styles but are engaging and playable.
// Setting up a simple game object with a placeholder graphic
class GameObject {
private Bitmap graphic; // Placeholder graphic
private float posX, posY;
GameObject(Bitmap graphic, float x, float y) {
this.graphic = graphic; // Use graphics later
this.posX = x;
this.posY = y;
}
void draw(Canvas canvas) {
canvas.drawBitmap(graphic, posX, posY, null);
}
}
Final Thoughts
Prioritize gameplay mechanics and user experience over flashy graphics. Your players will appreciate a game that is engaging and fun to play, regardless of its visual fidelity.
3. Underestimating Optimization
The Importance of Performance
Many developers overlook optimization in their projects. Poor performance can lead to frustrated users and uninstalls.
Basic Optimization Techniques
Utilize efficient coding practices and tools to make your game run smoothly on a range of devices. Techniques include:
- Minimizing memory usage
- Efficiently managing resources
- Directly updating graphics layers
Here’s a simple example of how you can manage resources more effectively:
// Use a singleton pattern for resource management
public class ResourceManager {
private static ResourceManager instance;
private SparseArray<Bitmap> bitmapArray;
private ResourceManager() {
bitmapArray = new SparseArray<>();
}
public static synchronized ResourceManager getInstance() {
if (instance == null) {
instance = new ResourceManager();
}
return instance;
}
public void addBitmap(int id, Bitmap bitmap) {
bitmapArray.put(id, bitmap);
}
public Bitmap getBitmap(int id) {
return bitmapArray.get(id);
}
}
Final Thoughts
Performance should be a priority in your development stages. Follow optimization principles from the outset to ensure a smoother game experience.
4. Ignoring Game Physics
Why Game Physics Matter
In many games, especially Android platformers, robust physics can enhance gameplay.
Application of Physics
Implementing basic physics such as gravity and collision detection will create a more realistic and enjoyable experience. Libraries like Box2D can help manage these aspects more effectively.
Here’s a simple illustration of integrating basic physics:
class Player {
private float velocityY = 0;
private boolean isJumping = false;
void jump() {
if (!isJumping) {
velocityY = -10; // Jump strength
isJumping = true;
}
}
void update() {
if (isJumping) {
velocityY += 0.5; // Simulate gravity
posY += velocityY; // Update position
if (posY >= groundLevel) {
posY = groundLevel; // Reset player position
isJumping = false;
}
}
}
}
Final Thoughts
Don’t neglect physics in your game. They can influence not only the player’s experience but also the overall fun and feel of the game.
5. Not Testing Thoroughly
The Testing Process
Testing is often an afterthought. Programmers might feel sure in their code, but bugs can creep in unexpectedly.
Embrace a Testing Culture
Employ unit testing, seek beta testers, and gather user feedback. Continuously iterate on your game based on this feedback. Regular testing can catch issues before they reach your players.
Example of Android Testing
You can utilize Android's built-in testing frameworks to automate the testing of your game mechanics. Here’s an example of a simple test case:
import static org.junit.Assert.*;
public class PlayerTest {
@Test
public void testJump() {
Player player = new Player();
player.jump();
assertEquals("Player should be jumping", -10, player.getVelocityY(), 0);
}
}
Final Thoughts
Effective testing should be a vital part of your development process. It can transform your game from good to great.
6. Not Utilizing the Android Development Community
The Benefits of Community Support
The Android development community is vast and invaluable. Many developers fall into the trap of tackling problems alone.
Collaborate and Share
Don’t hesitate to ask for help in forums, join groups, and participate in game jams. Resources like Android Developers and GameDev.net offer a wealth of information.
Final Thoughts
Leverage the community for solutions, tutorials, and inspiration. You’ll find that collaboration can lead to better results.
Final Thoughts
Developing an Android game is a rich, rewarding experience, but it comes with challenges. By avoiding these common pitfalls—like neglecting a solid Game Design Document, overemphasizing graphics, ignoring optimization, underestimating game physics, not engaging in thorough testing, and overlooking community resources—you can set yourself on a pathway to success.
Remember, the key to effective game development is a well-rounded approach that balances planning, execution, and continual improvement. Happy coding!
Checkout our other articles