Common Pitfalls in OAuth2 Bearer Token Implementation
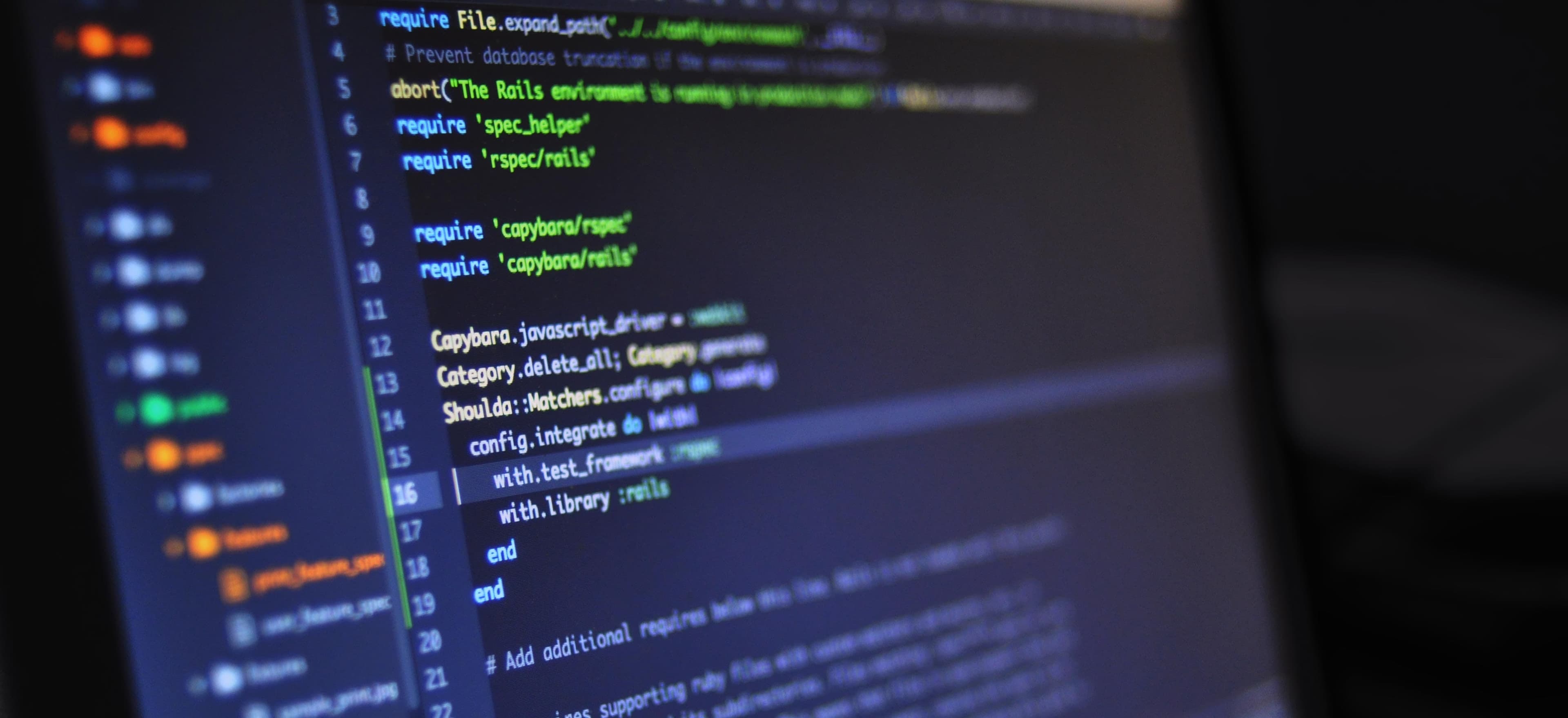
- Published on
Common Pitfalls in OAuth2 Bearer Token Implementation
OAuth2 has become the go-to framework for securing APIs. With its wide adoption, however, developers often encounter several pitfalls during implementation. Understanding and avoiding these common mistakes can save developers from significant security vulnerabilities and usability issues. In this blog post, we will explore these pitfalls, provide code snippets for better understanding, and offer practical solutions.
Table of Contents
- What is OAuth2?
- Common Pitfalls in OAuth2 Bearer Token Implementation
- Lack of Proper Token Storage
- Not Using HTTPS
- Token Scope Mismanagement
- Expired Tokens
- Unrestricted Token Revocation
- Best Practices for Secure OAuth2 Implementation
- Conclusion
What is OAuth2?
Before diving into the pitfalls, let's briefly discuss what OAuth2 is. OAuth2 is an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service. This is accomplished through a process that involves the user granting permission, which audits access via bearer tokens.
A bearer token is a type of access token—the way an application proves its identity to an API. When used correctly, OAuth2 can be both powerful and secure.
Common Pitfalls in OAuth2 Bearer Token Implementation
1. Lack of Proper Token Storage
Problem One of the most common pitfalls is improper handling of bearer tokens. Store them insecurely, and malicious parties can hijack them.
Solution Tokens should never be stored in local storage or regular cookies. Instead, consider using secure cookies.
Example
// Setting a secure cookie
Cookie cookie = new Cookie("access_token", YOUR_ACCESS_TOKEN);
cookie.setHttpOnly(true);
cookie.setSecure(true); // Only sent over HTTPS
response.addCookie(cookie);
This code snippet outlines how to create a secure cookie for storing an access token. The setHttpOnly
flag prevents JavaScript access, while the setSecure
flag ensures the cookie is only sent over secure connections.
2. Not Using HTTPS
Problem Using HTTP instead of HTTPS exposes bearer tokens during transmission. This leaves your application vulnerable to man-in-the-middle (MitM) attacks.
Solution Always enforce HTTPS to secure the entire transaction process, from user authorization to API calls.
Best Practice Most cloud providers support SSL certificates, so enabling HTTPS is usually straightforward. Use frameworks such as Spring Security to enforce HTTPS at the configuration level.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.requiresChannel()
.anyRequest()
.requiresSecure();
}
}
By implementing the above Spring Security configuration, all HTTP requests are automatically redirected to HTTPS.
3. Token Scope Mismanagement
Problem Another frequent mistake involves mismanaging token scopes. It’s tempting to provide overly broad access to resources. This can lead to unnecessary exposure of sensitive data.
Solution Define the least privilege principle in scope management. Only grant permissions that are absolutely necessary for an application to function.
Example
// Define scopes
String[] SCOPES = { "read:user", "update:profile" };
Always limit the scopes you define. In the example above, a user is only allowed to read user data or update their profile—no more, no less.
4. Expired Tokens
Problem Not properly handling token expiration can confuse users. If a token expires, the application may fail and experience significant disruptions.
Solution Implement a robust token refresh mechanism. This allows clients to obtain new tokens without requiring the user to reauthenticate.
Example
public String refreshToken(String expiredToken) {
if (isTokenExpired(expiredToken)) {
return generateNewToken();
}
return expiredToken;
}
In this snippet, the method checks if the token is expired. If it is, a new token is generated and returned. This seamless flow ensures users can continue to access resources without repeated logins.
5. Unrestricted Token Revocation
Problem Many applications fail to implement token revocation correctly, allowing tokens to linger even when access should be revoked (e.g., when a user logs out).
Solution Implement a mechanism for revoking tokens promptly and ensure that tokens are invalidated immediately after logout.
Example
public void revokeToken(String token) {
tokenStore.remove(token); // Invalidate the token
}
The code snippet above demonstrates how to revoke the token from storage, ensuring that once a user logs out, access via the invalidated token is no longer possible.
Best Practices for Secure OAuth2 Implementation
- Use Strong Cryptography: Use cryptographic best practices, such as RSA for signing tokens.
- Implement Rate Limiting: Protect your API from brute force attacks by implementing rate limiting.
- Utilize Short-Lived Tokens: Keep access tokens short-lived to minimize risk. Combine this with refresh tokens for usability.
- Conduct Regular Security Audits: Regular reviews of your OAuth2 implementation can help identify vulnerabilities.
For further reading on implementing OAuth2 correctly, visit OAuth's Official Documentation or dive into Spring Security OAuth2.
The Last Word
OAuth2 bearer tokens provide a flexible way to secure APIs, but common pitfalls can expose systems to vulnerabilities. By being aware of these pitfalls and following best practices, developers can ensure a clean, secure implementation.
To recap, be mindful of how you store tokens, enforce HTTPS, manage scopes judiciously, handle token expiration efficiently, and implement robust revocation features. With this knowledge, you're well on your way to a secure OAuth2 bearer token implementation.
Happy coding!