Why Event Sourcing Can Lead to Data Inconsistency Issues
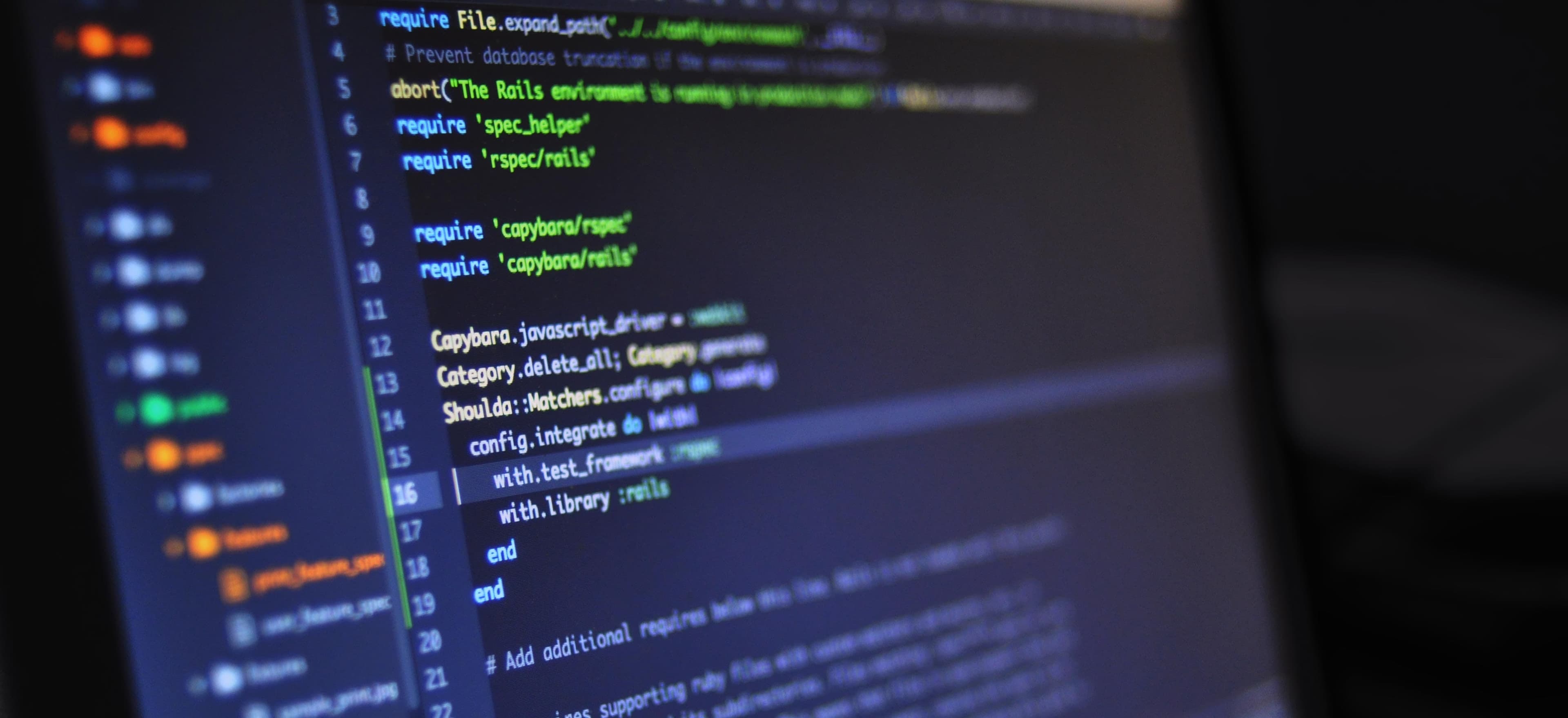
- Published on
Why Event Sourcing Can Lead to Data Inconsistency Issues
Event sourcing is a powerful architectural pattern that emphasizes storing the changes in state as a series of events rather than merely storing the current state. This approach is touted for various benefits, such as improved scalability, the ability to reconstruct past states, and enhanced traceability. However, it's not without its challenges. One significant drawback is the potential for data inconsistency. In this post, we'll explore why event sourcing can lead to data inconsistency issues, while providing practical insights and solutions.
What is Event Sourcing?
Before we delve deeper into the potential pitfalls of event sourcing, let's clarify what it is.
In a typical object-oriented application, you might represent a User object with properties like username and email. The traditional approach updates the current state of the User when necessary. By contrast, with event sourcing, every change to a User is stored as an event. For instance:
- UserCreated
- UserEmailChanged
- UserDeleted
Each of these events captures the change in a temporal sequence, allowing for a complete history of what has occurred to that User.
Key Benefits of Event Sourcing
- Auditability: Since every change is stored as an event, you can maintain a complete audit trail.
- Temporal Queries: You can reconstruct an object’s state at any point in time.
- Decoupling: The event-driven nature allows services to be designed independently.
Despite these benefits, it’s crucial to understand the trade-offs, especially concerning data consistency.
The Challenges of Data Inconsistency in Event Sourcing
1. Event Ordering
Problem
When events are stored without guaranteeing the order in which they were generated, it can lead to inconsistent views of the data. For instance, if a User's email was changed and then immediately marked as deleted, the system must accurately reflect this sequence. If the events are processed out of order, the User may appear deleted without changes applied.
Solution
Leveraging event stores that facilitate ordered event processing is essential. Systems like Apache Kafka offer guarantees on message ordering, ensuring that events are processed in the order they are produced.
public void updateUserEmail(String userId, String newEmail) {
try {
eventStore.save(new UserEmailChanged(userId, newEmail));
// Simulate further processing
eventStore.save(new UserEmailUpdated(userId));
} catch (Exception e) {
// Log and handle exception
}
}
In this example, if the event store cannot guarantee order, the consistency is at risk.
2. Event Versioning
Problem
Over time, the schema of events may evolve, especially in large systems. When the way events are represented changes, consumers may struggle to process older events correctly, leading to inconsistencies.
Solution
Implementing versioning within your event model can help manage these changes. For instance, appending a version field to your events supports backward compatibility as the application evolves.
public class UserEmailChanged {
private final String userId;
private final String newEmail;
private final int version;
public UserEmailChanged(String userId, String newEmail, int version) {
this.userId = userId;
this.newEmail = newEmail;
this.version = version;
}
}
3. Event Duplication
Problem
When systems are distributed, network failures or retries can lead to events being published more than once. This duplication can cause unexpected state changes.
Solution
A unique identifier for each event can mitigate this problem. By keeping track of processed event IDs, you can avoid reprocessing the same event multiple times.
public void processEvent(UserEmailChanged event) {
if (!eventAlreadyProcessed(event.getId())) {
// Process the event
markEventAsProcessed(event.getId());
}
}
4. Event Deletion and Soft Deletes
Problem
The semantics of deleting an entity can become complicated in event sourcing. If you merely mark an event as deleted, it may leave behind inconsistent states, especially if there are associated events that still remain.
Solution
Instead of a soft delete, create explicit events that represent deletion. This keeps the history intact and allows for complete reconstruction.
public void deleteUser(String userId) {
eventStore.save(new UserDeleted(userId));
// Further actions related to deletion can be tracked
}
5. Consumer State Management
Problem
Different consumers of the event stream may interpret events differently or may not consume events in a timely manner. This can lead to inconsistencies between various systems that rely on the same data.
Solution
Using a standardized protocol for event consumption can improve consistency. Ensure that all consumers handle events similarly and implement retry mechanisms when there are consumption failures.
public void consumerHandler(Event event) {
try {
userService.handleEvent(event);
} catch (Exception e) {
// Retry logic can be implemented
}
}
Closing the Chapter
Event sourcing is indeed a powerful pattern that can facilitate complex systems architecture. However, navigating the waters of data consistency can prove challenging. To address these issues, it’s essential to:
- Ensure event ordering is maintained throughout your systems.
- Implement event versioning to accommodate schema changes.
- Guard against event duplication by tracking event identifiers.
- Use explicit deletion events instead of soft deletes to maintain data integrity.
- Standardize consumer behavior and handling to create a uniform state across systems.
By being cognizant of these challenges and embracing best practices, teams can leverage event sourcing effectively while minimizing the risks of data inconsistency.
If you want to learn more about event sourcing and its nuances, consider diving into resources like Martin Fowler's Event Sourcing and Understanding Event-Driven Architecture.
Embracing event sourcing can transform your system's architecture, provided you take the necessary steps to ensure data consistency amidst the myriad events that shape your application's state.
Checkout our other articles