Mastering Pivot Tables with Java Streams: Common Pitfalls
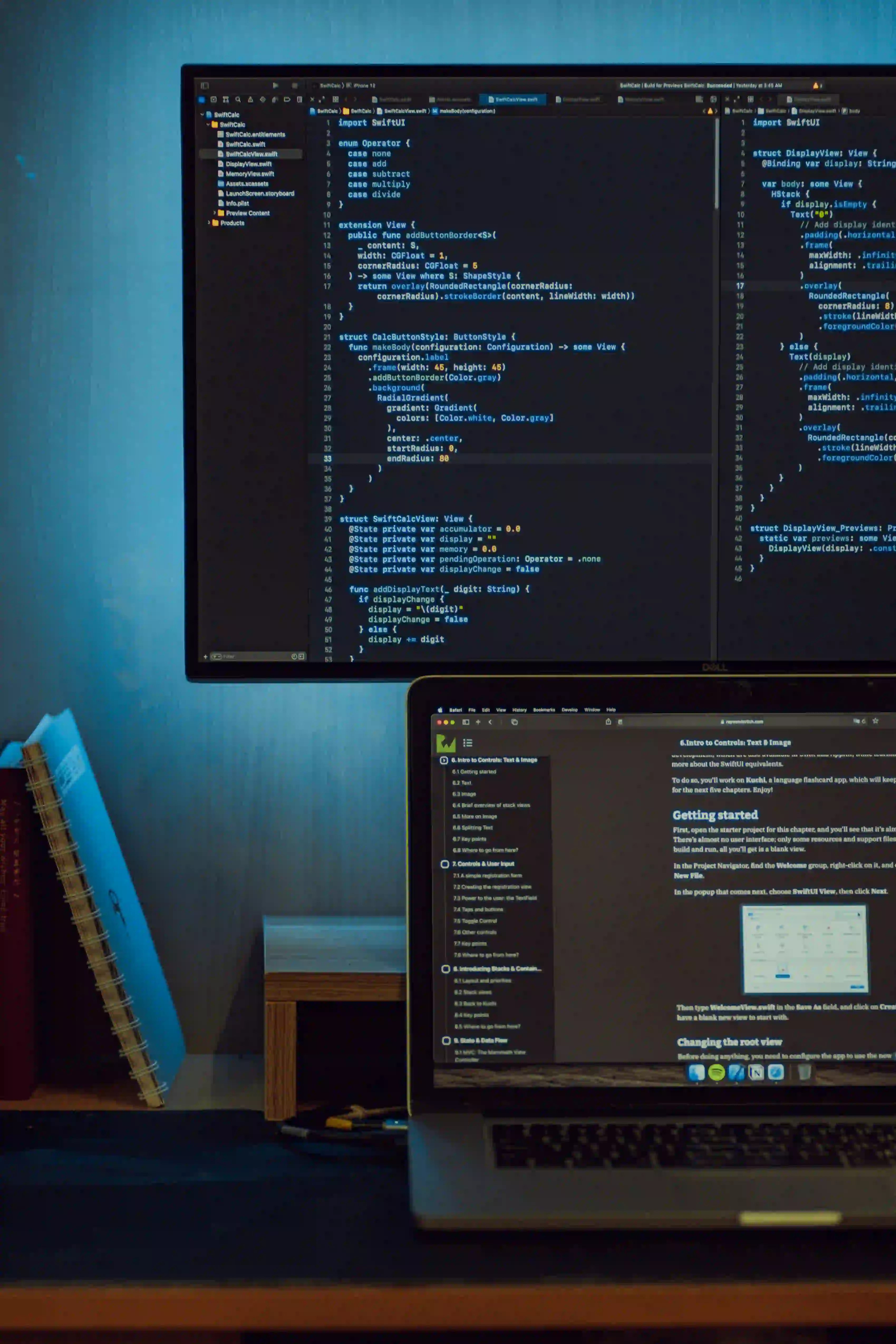
Mastering Pivot Tables with Java Streams: Common Pitfalls
Pivot tables are a powerful tool for data manipulation and analysis, commonly used in data analysis software like Excel. In the realm of Java programming, we can utilize Java Streams to emulate this functionality effectively. However, several common pitfalls may undermine our attempts to create efficient and readable code. In this blog post, we will explore the art of mastering pivot tables using Java Streams, while also addressing mistakes to avoid, and providing exemplary code snippets with explanations.
What is a Pivot Table?
A pivot table allows the user to summarize and analyze data from a large dataset. It typically organizes information in a way that reveals patterns and trends, making reporting easier. The end result often includes aggregated values like counts, sums, or averages.
To illustrate our approach, let’s consider an example dataset of sales transactions:
| Product | Category | Amount | |----------|----------|--------| | Widget A | Gizmos | 10 | | Widget B | Gizmos | 20 | | Widget A | Gadgets | 15 | | Widget C | Gizmos | 30 | | Widget B | Gadgets | 25 |
Our goal is to pivot this data to find the total sales amount per category. A pivot table in this context would have Category
as rows and the total Amount
as values.
Setting the Stage: Java Streams Overview
Java Streams, introduced in Java 8, provide a functional approach to processing sequences of elements, making data manipulation concise and expressive. They are ideal for working with collections and allow for operations like filtering, mapping, and reducing.
Basic Code Structure
Here is a sample code snippet that demonstrates how to achieve pivoting with Java Streams:
import java.util.*;
import java.util.stream.*;
public class SalesDataPivot {
public static void main(String[] args) {
// Sample dataset
List<Sale> sales = Arrays.asList(
new Sale("Widget A", "Gizmos", 10),
new Sale("Widget B", "Gizmos", 20),
new Sale("Widget A", "Gadgets", 15),
new Sale("Widget C", "Gizmos", 30),
new Sale("Widget B", "Gadgets", 25)
);
// Pivoting the data
Map<String, Integer> pivotTable = sales.stream()
.collect(Collectors.groupingBy(
Sale::getCategory,
Collectors.summingInt(Sale::getAmount)
));
System.out.println("Pivot Table:");
pivotTable.forEach((category, total) ->
System.out.printf("Category: %s, Total: %d%n", category, total));
}
}
class Sale {
private String product;
private String category;
private int amount;
public Sale(String product, String category, int amount) {
this.product = product;
this.category = category;
this.amount = amount;
}
public String getProduct() {
return product;
}
public String getCategory() {
return category;
}
public int getAmount() {
return amount;
}
}
Code Explanation
-
Data Definition:
☕snippet.javaList<Sale> sales = Arrays.asList(/* ... */);
We create a list of
Sale
objects to store our dataset. -
Stream Processing:
☕snippet.javaMap<String, Integer> pivotTable = sales.stream()
We convert the list into a stream to enable various operations.
-
Grouping and Aggregating:
☕snippet.javaCollectors.groupingBy(Sale::getCategory, Collectors.summingInt(Sale::getAmount))
We group the sales by
Category
and sum the correspondingAmount
. -
Output: We print the pivot table using
forEach
.
Common Pitfalls
Despite the elegance of Java Streams, there are pitfalls. Let's examine some that can lead to performance issues or inaccuracies.
1. Incorrect Grouping Logic
Mistake: Failing to properly define the grouping criteria can yield an inaccurate pivot table. For instance:
Map<String, Integer> wrongPivotTable = sales.stream()
.collect(Collectors.groupingBy(
Sale::getProduct, // Incorrect grouping by Product instead of Category
Collectors.summingInt(Sale::getAmount)
));
Solution: Always reassess your grouping criteria. Ensure it aligns with your analysis objectives—group by Category
in our use case.
2. Handling Null Values
Mistake: If your dataset contains null values, they can throw exceptions or skew results.
Solution: Utilize optional methods or filters to handle nulls gracefully. Example:
Map<String, Integer> safePivotTable = sales.stream()
.filter(sale -> sale.getCategory() != null) // Filter out null categories
.collect(Collectors.groupingBy(
Sale::getCategory,
Collectors.summingInt(Sale::getAmount)
));
3. Forgetting to Handle Large Datasets
Mistake: Attempting to process very large datasets without considerations for performance might cause memory issues.
Solution: Take advantage of parallel streams for larger datasets:
Map<String, Integer> parallelPivotTable = sales.parallelStream()
.collect(Collectors.groupingBy(
Sale::getCategory,
Collectors.summingInt(Sale::getAmount)
));
Additional Functionalities
Java Streams provide additional functionalities that can enhance your pivoting process further. Here are some to consider:
-
Custom Aggregation: Instead of just summing values, consider calculating averages or counting unique items for a more comprehensive analysis.
-
Multi-Level Pivoting: You can perform multi-level pivots by nesting collectors, allowing for deeper data analysis.
-
Sorting Results: After obtaining the pivot table, you can sort the results for better readability:
☕snippet.javaMap<String, Integer> sortedPivotTable = pivotTable.entrySet() .stream() .sorted(Map.Entry.comparingByValue()) .collect(Collectors.toMap( Map.Entry::getKey, Map.Entry::getValue, (e1, e2) -> e1, LinkedHashMap::new ));
My Closing Thoughts on the Matter
Mastering pivot tables with Java Streams opens endless possibilities for data manipulation and analysis. However, be wary of common pitfalls that can arise during this process, such as incorrect grouping, handling of nulls, and performance issues with large datasets. Through the application of proper techniques and best practices, you can harness the full power of Java Streams for your pivoting tasks.
We hope this guide enhances your understanding of pivot tables and equips you with the knowledge needed to utilize Java Streams effectively. For deeper dives into Java Streams and advanced data processing techniques, check out Oracle's Official Java Documentation.
Happy coding!