Overcoming TDD Challenges with Transformation Priority
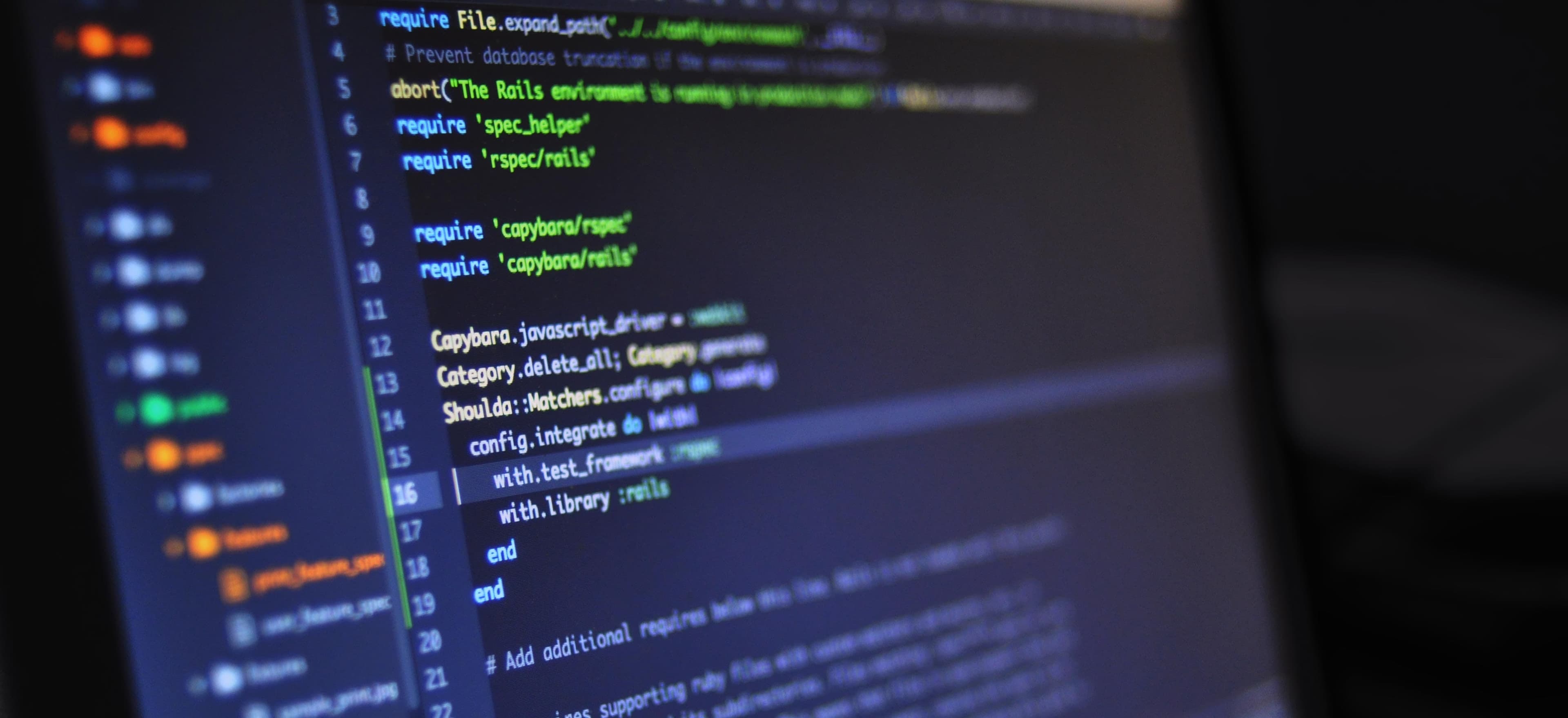
- Published on
Overcoming TDD Challenges with Transformation Priority
Test-Driven Development (TDD) is a widely-used methodology in software development that encourages writing tests before writing the actual code. This approach promotes better code quality, cleaner designs, and facilitates a more agile development process. However, adopting TDD is not without its challenges. Developers often face hurdles such as understanding requirements clearly, managing complex tests, and coping with resistance to change within teams.
In this blog post, we will explore the concept of Transformation Priority and how it can help overcome common challenges faced in TDD. We will also provide practical examples and code snippets to illustrate the discussion.
Understanding Transformation Priority
Transformation Priority is a principle that suggests prioritizing changes based on their impact on functionality. This means that whenever you consider changes in code, evaluate them based on how they might transform or enhance your software's features. By doing this, you can streamline your approach to TDD and make the development process less cumbersome.
The TDD Cycle Explained
Before diving deeper into Transformation Priority, let's briefly revisit the TDD cycle, which consists of three primary phases:
- Red: Write a test that defines a function or improvements of a feature. Initially, this test fails since the functionality hasn't been implemented yet.
- Green: Write the minimum amount of code necessary to make the test pass. This can lead to what is often referred to as "bare minimum," ensuring speed in getting results.
- Refactor: Improve the code while ensuring that all tests still pass. This helps in enhancing code quality and readability.
Common TDD Challenges
Now that we've established the foundation of TDD, let’s discuss some challenges that developers commonly face:
- Defining Requirements: Sometimes, it's difficult to express clear requirements for tests, leading to ambiguity.
- Test Complexity: As projects grow, tests can become complex, making maintenance difficult.
- Team Resistance: Teams may resist adopting TDD practices, especially if they perceive it as slowing down the development process.
How Transformation Priority Helps
Transformation Priority can help mitigate these TDD challenges in the following ways:
1. Clarifying Requirements
By focusing on transformations, developers can define more precise requirements. Instead of thinking broadly about what a feature should do, consider how the feature transforms the state of an application.
For example, consider a banking application that allows users to transfer money between accounts. Instead of overly complex requirements like "User can transfer money," the requirement can be transformed into: "If a user requests a transfer of X amount from Account A to Account B, then Account A should decrease by X, and Account B should increase by X."
Code Snippet: Defining Transfer Functionality
public class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
this.balance = initialBalance;
}
public void transfer(BankAccount toAccount, double amount) {
if (amount > 0 && this.balance >= amount) {
this.balance -= amount; // Decrease from sender's account
toAccount.balance += amount; // Increase in recipient's account
} else {
throw new IllegalArgumentException("Invalid transfer amount.");
}
}
}
In this snippet, the transfer
method checks for valid conditions before performing the operation. This simplicity in requirement, driven by Transformation Priority, leads to clearer tests.
2. Managing Test Complexity
When we look at how a certain piece of functionality transforms the state, it becomes easier to focus our tests on specific outcomes rather than on the entire method or class.
For instance, instead of testing the entire transfer
function for various conditions in one blow, we can separate our tests using the transformation principle.
Code Snippet: Unit Tests for Transfer Functionality
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class BankAccountTest {
@Test
public void testSuccessfulTransfer() {
BankAccount sender = new BankAccount(1000);
BankAccount recipient = new BankAccount(500);
sender.transfer(recipient, 200);
assertEquals(800, sender.getBalance());
assertEquals(700, recipient.getBalance());
}
@Test
public void testTransferInvalidAmount() {
BankAccount sender = new BankAccount(1000);
BankAccount recipient = new BankAccount(500);
assertThrows(IllegalArgumentException.class, () -> {
sender.transfer(recipient, -100);
});
}
}
In these unit tests, we clearly define what transformation we expect from performing the transfer. This straightforward approach reduces complexity and enhances maintainability, as changes to the class or the tests will be more contained and manageable.
3. Reducing Team Resistance
Transformation Priority not only simplifies the coding process but can also facilitate discussions within teams. When developers understand the transformations, it becomes easier to reach consensus on feature implementations or requirements. Engaging the team in discussions about transformations fosters collaboration and can reduce resistance to TDD.
Best Practices to Implement Transformation Priority
To effectively incorporate Transformation Priority into your TDD workflow, consider these best practices:
- Define Clear Transformations: Encourage team members to articulate functionality through transformations clearly.
- Modularize Tests: Write tests in a modular fashion, focusing on single transformations at a time.
- Collaborative Efforts: Foster open communication and collaborative discussions within the team regarding transformations.
The Closing Argument
In conclusion, the principles of Transformation Priority act as a guiding light in overcoming the common challenges associated with Test-Driven Development. By focusing on how functions transform the system rather than just relying on vague requirements, developers can create clearer codes, simpler tests, and an overall smoother development process.
Implementing TDD effectively requires consistent practice and adaptation. So, keep refining your approach, revisit the transformation mindset often, and lead your development team towards greater success.
For additional reading on Test-Driven Development and best practices, check out resources from Martin Fowler's website and The Clean Code Blog.
Embrace the transformation, and happy coding!
Checkout our other articles