Java Performance Issues: Uncovering the Memory Leak Mystery
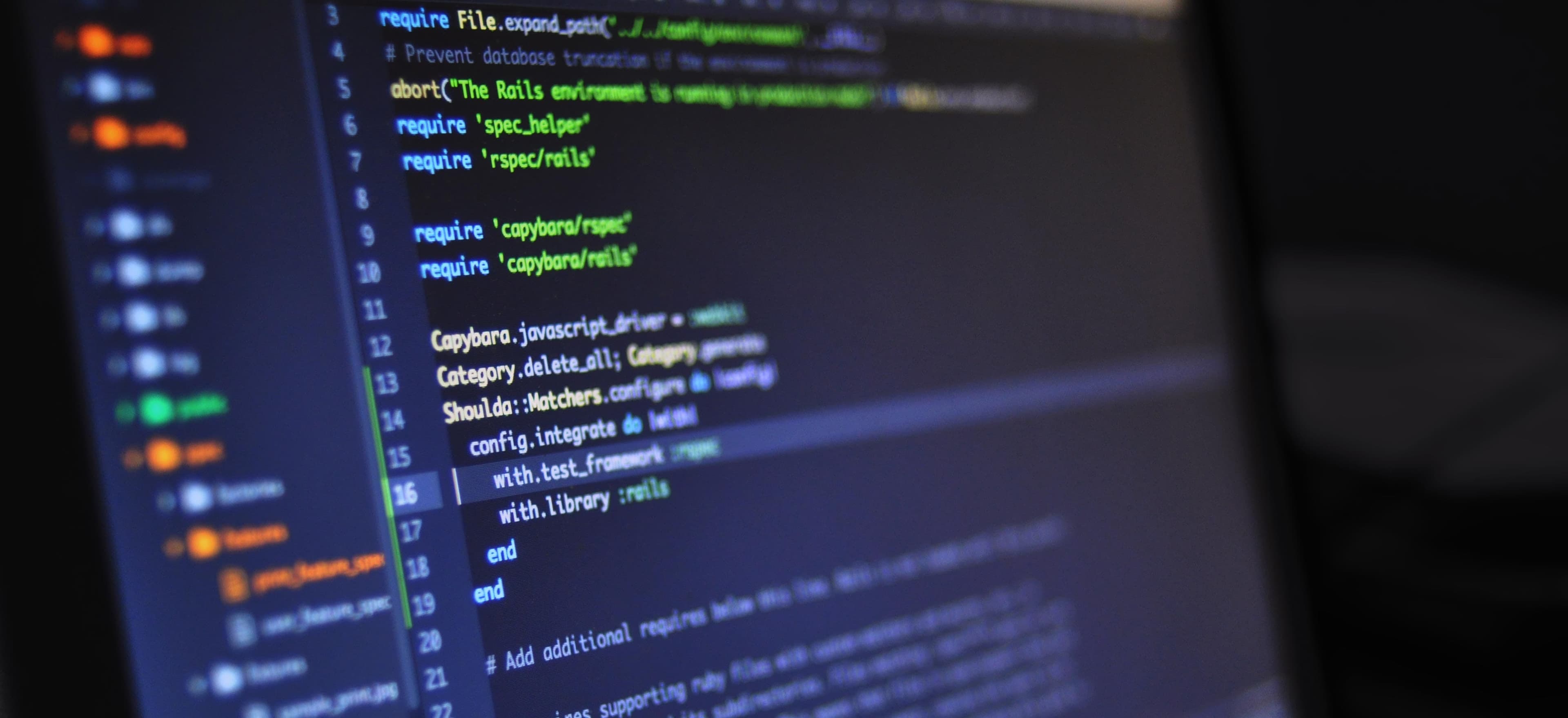
- Published on
Java Performance Issues: Uncovering the Memory Leak Mystery
Java is widely recognized for its ability to manage memory automatically through its garbage collection feature. Yet, even with this robust system, developers often find themselves facing one of the most dreaded challenges in software development: memory leaks. Understanding and resolving these leaks is crucial for maintaining the performance and reliability of Java applications. In this blog post, we'll delve into memory leaks in Java, how to identify them, and provide practical solutions.
What is a Memory Leak?
A memory leak occurs when a Java application retains references to objects that are no longer needed, preventing the garbage collector from reclaiming that memory. This can lead to increased memory consumption and, eventually, out-of-memory errors. Developers may mismanage object creation, leading to memory not being released when it's supposed to be.
Why Memory Leaks Matter
Memory leaks can result in performance degradation over time and may ultimately lead to application crashes. For high-traffic applications, such as web services and enterprise-level solutions, the consequences can be severe, impacting user experience and service reliability.
Common Causes of Memory Leaks in Java
To effectively address memory leaks, it's important to understand their common causes:
1. Unintentional Object References
When objects are unintentionally held in memory, the garbage collector cannot reclaim them. This can happen through static collections, singletons, or even inner classes holding references to their enclosing class.
public class Cache {
private static List<String> cachedData = new ArrayList<>();
public static void addData(String data) {
cachedData.add(data);
}
}
In the above example, the static list retains references indefinitely, potentially leading to a memory leak.
2. Improper Use of Listener Patterns
Listener patterns are a common design strategy, but failing to unregister listeners can lead to leaks.
public class EventSource {
private List<EventListener> listeners = new ArrayList<>();
public void register(EventListener listener) {
listeners.add(listener);
}
public void notifyListeners() {
for (EventListener listener : listeners) {
listener.onEvent();
}
}
}
In this scenario, if listeners are not unregistered during object destruction, they will remain in memory, leading to a buildup over time.
3. Long-Lived Collections
Holding references in collections without cleaning them can exacerbate memory issues.
public class UserSessionManager {
private Map<String, UserSession> sessions = new HashMap<>();
public void addSession(String id, UserSession session) {
sessions.put(id, session);
}
public void removeSession(String id) {
sessions.remove(id);
}
}
If sessions aren't properly removed, memory consumption will continue to grow as new sessions are added.
Identifying Memory Leaks
Before resolving memory leaks, it’s critical to identify them. Here are some effective methodologies:
1. Profiling Tools
Java provides several tools for monitoring and analyzing memory usage:
- VisualVM: A free tool bundled with the JDK that allows you to monitor the performance of your Java application in real-time.
- Eclipse Memory Analyzer (MAT): A powerful tool for analyzing heap dumps. It can help identify memory leaks and their causes.
2. Heap Dumps
Taking a heap dump provides a snapshot of your application's memory at a certain point, which can help you analyze object references and allocation.
// Trigger a heap dump from your Java application
jmap -dump:live,format=b,file=heapDump.hprof <process_id>
3. Garbage Collection Logs
Enabling garbage collection logging can help track memory usage over time:
java -Xlog:gc*:file=gc.log -jar myApp.jar
By analyzing this log, you can observe memory allocation patterns and identify abnormalities.
Best Practices to Avoid Memory Leaks
Prevention is always the best approach. Here are some best practices:
1. Use Weak References
In scenarios like caching where you need temporary storage, consider using WeakReference
or SoftReference
.
class Cache {
private Map<String, WeakReference<String>> cache = new HashMap<>();
public void add(String key, String value) {
cache.put(key, new WeakReference<>(value));
}
}
Weak References allow objects to be garbage collected when memory is needed, thus reducing the risk of leaks.
2. Clean Up Resources
If you're using resources like database connections, it's essential to close them explicitly.
try (Connection conn = dataSource.getConnection()) {
// Perform operations
} catch (SQLException e) {
e.printStackTrace();
}
// Automatic Resource Management (ARM) ensures closure
This ensures that resources are freed immediately after use.
3. Unregister Listeners
Remember to unregister any listeners you've registered during object destruction.
public void shutdown() {
eventSource.unregister(this);
}
Proper cleanup code greatly reduces the chances of memory leaks.
Wrapping Up
Memory leaks pose a significant challenge in Java, but understanding their causes and learning how to identify and prevent them can vastly improve the performance of your applications. By integrating best practices, using appropriate tools, and remaining vigilant about memory management, you can protect your applications from potential leaks.
For more in-depth reading on effective memory management in Java, you may want to refer to Java Performance Tuning or explore Oracle’s Garbage Collection Tuning Guide.
By implementing these strategies, you'll be well on your way to creating robust and efficient Java applications. Happy coding!
Checkout our other articles