Mastering CDI Named Components in Java EE 6
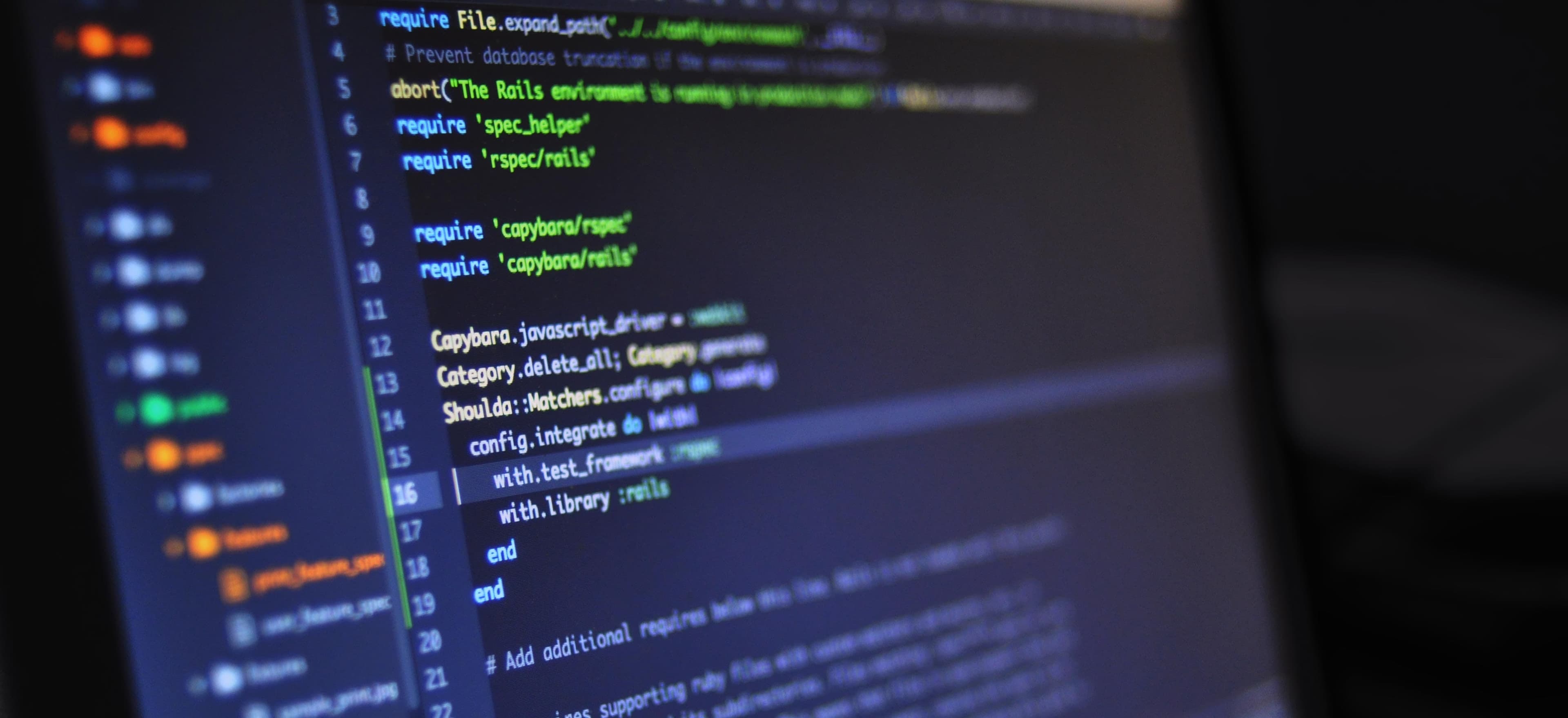
- Published on
Mastering CDI Named Components in Java EE 6
The Approach
In the realm of Java EE (Enterprise Edition), Contexts and Dependency Injection (CDI) is a powerful framework that allows developers to build lightweight, modular applications. CDI enhances the standard Java development model by providing seamless management of component lifecycles, scoping, and dependency resolution. Among its many features, the ability to name components plays a crucial role in producing maintainable and comprehensible code. This blog post will delve into the intricacies of CDI named components in Java EE 6, highlighting their significance, use cases, and providing illustrative code examples.
What are CDI Named Components?
Named components in CDI allow developers to create beans that can be looked up by a specified name within the application context. This is particularly beneficial for instances where multiple instances of the same bean class may exist, enabling developers to reference them easily using a name. The named beans also contribute to enhanced readability and maintainability and can be injected using their specified names.
Setting Up Your Environment
To get started with Java EE 6 and CDI, ensure that your development environment is properly configured. You will need:
- Java Development Kit (JDK) 6 or higher
- A compatible application server like WildFly, GlassFish, or Payara
- An Integrated Development Environment (IDE) like Eclipse, IntelliJ IDEA, or NetBeans
Maven Configuration
To facilitate project management and dependency management, we suggest setting up a Maven project. Below is an example of a pom.xml
file for a Java EE project with CDI:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>cdidemo</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<cdi.version>1.0</cdi.version>
</properties>
<dependencies>
<dependency>
<groupId>javax.enterprise</groupId>
<artifactId>cdi-api</artifactId>
<version>${cdi.version}</version>
<scope>provided</scope>
</dependency>
</dependencies>
</project>
Creating a Named Component
Let's define a simple service class that we'll name and use within our application.
Example: A Simple Greeting Service
import javax.enterprise.context.ApplicationScoped;
import javax.inject.Named;
@Named("greetingService")
@ApplicationScoped // This bean will be instantiated once and shared
public class GreetingService {
public String greet(String name) {
return "Hello, " + name + "!";
}
}
Explanation:
- @Named("greetingService"): This annotation allows the
GreetingService
bean to be referenced by the name "greetingService". - @ApplicationScoped: This scope makes the bean shared across all users and sessions, meaning only one instance exists for the entire application.
Utilizing the Named Service
Now, let’s see how we can inject this named component into another class, such as a controller. This is where CDI shines, making it simple to access your named components.
import javax.inject.Inject;
import javax.enterprise.context.RequestScoped;
import javax.inject.Named;
@Named("greetingController")
@RequestScoped // This bean lives for a single HTTP request
public class GreetingController {
@Inject
private GreetingService greetingService; // Injecting the named bean
public String getGreetingMessage(String name) {
return greetingService.greet(name); // Using the injected service
}
}
Explanation:
- @Inject: CDI uses this annotation to inject the
GreetingService
into theGreetingController
. Even though we didn’t use a qualifier, CDI resolves the named dependency on its own. - @RequestScoped: This scope means a new instance of
GreetingController
will be created for each HTTP request, fitting the needs of web applications.
Using CDI in JSF
JavaServer Faces (JSF) is a compatible technology with Java EE for building web applications. You can take advantage of the CDI named components right inside your JSF pages.
Example of a JSF page
Here’s an example of a simple JSF page that utilizes our GreetingController
.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html">
<h:head>
<title>CDI Greeting Example</title>
</h:head>
<h:body>
<h:form>
<h:inputText value="#{greetingController.name}" placeholder="Enter your name"/>
<h:commandButton value="Greet!"
action="#{greetingController.getGreetingMessage(name)}"/>
</h:form>
<h:outputText value="#{greetingController.greetingMessage}"/>
</h:body>
</html>
Key Points:
- The
greetingController
is bound to the input text for the user's name and is used to display the greeting message. - The command button invokes the method within the
GreetingController
.
Advantages of CDI Named Components
- Modularity: Using named components facilitates modularity within applications as it allows for clear separation and naming of components.
- Maintainability: Named beans enhance readability, making it easier for developers to manage dependencies across large projects.
- Dependency Injection: Simplified management of component dependencies, allowing for cleaner and less error-prone code.
Closing the Chapter
Mastering CDI in Java EE 6 is essential for developers hoping to create well-structured and maintainable applications. Named components provide an added layer of simplicity, allowing for clear, concise, and readable code. As you've seen, utilizing CDI named components can streamline your Java EE development process significantly.
For more detailed information on CDI, check the Java EE Documentation, and for best practices, consider diving into books or courses focused on CDI.
Happy coding!
Feel free to reach out if you have any questions or need further clarifications on CDI in Java EE!
Checkout our other articles