Mastering Execution Time Measurement in Java Spring
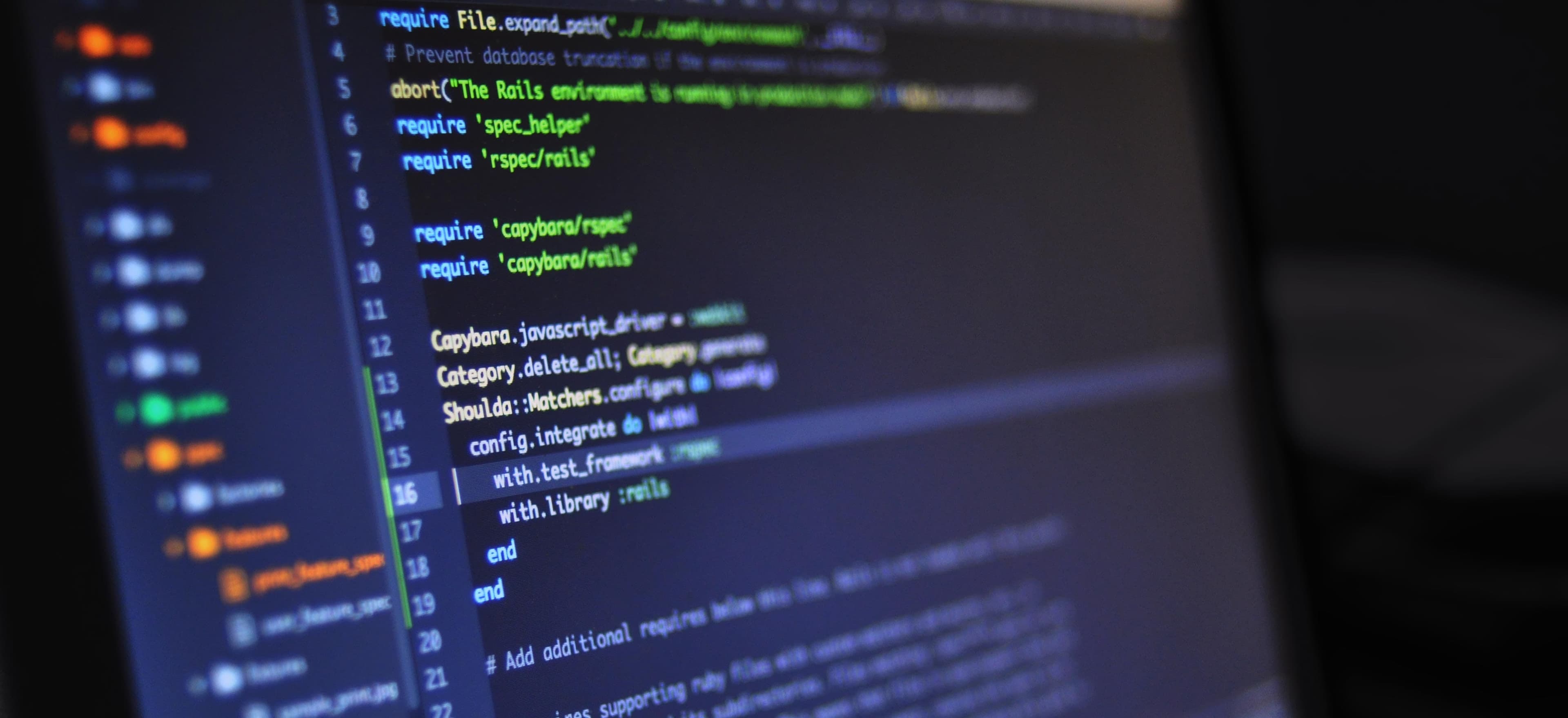
- Published on
Mastering Execution Time Measurement in Java Spring
In the world of software development, performance is king. Users expect quick responses and smooth interactions from applications. For Java developers, especially those leveraging the Spring framework, understanding how to measure and optimize execution time is crucial. This blog post dives deep into efficient techniques for measuring execution time in Java Spring, along with best practices and code snippets to illustrate concepts.
Why Measure Execution Time?
Before we get into the nitty-gritty of code, let’s discuss why measuring execution time is vital. Understanding how long your methods take to execute can help:
- Identify Bottlenecks: Locate slow methods that may be affecting overall performance.
- Optimize Processes: Make informed decisions about where to focus your optimization efforts.
- Enhance User Experience: By minimizing execution time, you can deliver a seamless user experience, leading to higher satisfaction and retention.
Tools and Techniques in Java Spring
1. Built-in Metrics with Spring Boot
Spring Boot comes equipped with built-in metrics enabled by the Spring Actuator module. It provides detailed insights into the performance and health of your application.
Adding Spring Actuator
To utilize Spring Actuator, add the dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Next, you can expose different metrics by configuring the application.properties
:
management.endpoints.web.exposure.include=health,metrics
Accessing Metrics
Once configured, you can access various metrics, including execution time, by hitting the /actuator/metrics
endpoint. This endpoint gives you insights into methods and their execution durations.
2. Using AOP (Aspect-Oriented Programming)
A more sophisticated way to measure execution time in specific methods is to use Aspect-Oriented Programming (AOP). AOP allows you to separate cross-cutting concerns, like logging and performance measurement, from your business logic.
Implementing AOP for Execution Time Measurement
First, ensure you have the AOP starter in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
Next, create an aspect class to handle the execution time measurement:
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class ExecutionTimeAspect {
private static final Logger logger = LoggerFactory.getLogger(ExecutionTimeAspect.class);
@Around("execution(* com.example.demo.service..*(..))")
public Object measureExecutionTime(ProceedingJoinPoint joinPoint) throws Throwable {
long start = System.currentTimeMillis();
Object proceed = joinPoint.proceed();
long executionTime = System.currentTimeMillis() - start;
logger.info("Method {} executed in {} ms", joinPoint.getSignature(), executionTime);
return proceed;
}
}
Explanation of the Code
@Aspect
: Indicates that this class is an aspect.@Around
: Specifies that our advice applies around method executions matching the given pointcut.ProceedingJoinPoint
: Allows you to proceed with the next advice/method execution.- The execution time is calculated as the difference between the current time before and after executing the method.
3. JMH (Java Microbenchmark Harness)
For more granular and sophisticated performance measurements, consider using JMH. JMH is best suited for benchmarking the performance of micro-operations in Java.
A Simple Benchmark Example
Add JMH to your Maven dependencies:
<dependency>
<groupId>org.openjdk.jmh</groupId>
<artifactId>jmh-core</artifactId>
<version>1.35</version>
</dependency>
<dependency>
<groupId>org.openjdk.jmh</groupId>
<artifactId>jmh-generator-annprocess</artifactId>
<version>1.35</version>
</dependency>
Create a benchmark class:
import org.openjdk.jmh.annotations.*;
import java.util.concurrent.TimeUnit;
@BenchmarkMode(Mode.AverageTime)
@OutputTimeUnit(TimeUnit.MILLISECONDS)
public class MyBenchmark {
@Benchmark
public void testMethod() {
// Simulated work
for (int i = 0; i < 1000; i++) {
System.out.println("Processing item " + i);
}
}
}
Running the Benchmark
You can run the benchmark from the command line once your project is built, or integrate it into your IDE:
java -jar my-benchmark.jar
Final Thoughts
Measuring execution time in Java Spring applications is essential for identifying issues that could hinder performance. Whether you choose to use Spring Actuator for built-in metrics, implement AOP for tailored measurements, or leverage JMH for advanced benchmarking, there are multiple approaches to help you gain insights into your application's performance.
Final Thoughts
Performance optimization is a continuous cycle. Implementing execution time measurement provides actionable insights that can significantly improve your application.
Implement the techniques discussed in this post, and you will be well on your way to mastering execution time measurement in Java Spring applications!
Resources
Feel free to dive deeper into each topic, and empower your Java applications with finely-tuned performance measurements!
Checkout our other articles