Troubleshooting Async Issues in Java 8 CompletableFuture & RxJava
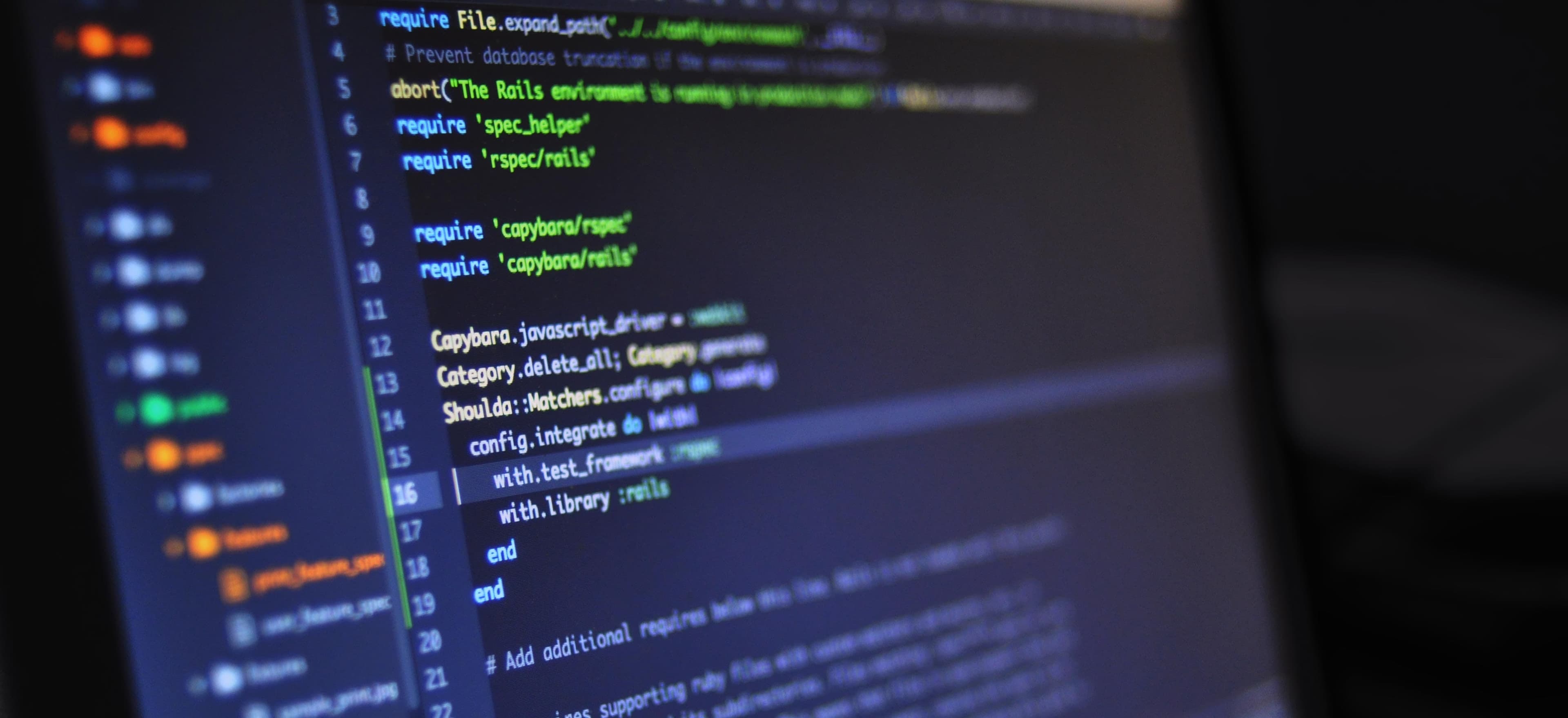
- Published on
Troubleshooting Async Issues in Java 8 CompletableFuture & RxJava
Java introduced the CompletableFuture
class in Java 8, opening the door for developers to embrace asynchronous programming with ease. On the other hand, RxJava, a library for composing asynchronous and event-based programs using observable sequences, has become a standard in reactive programming. However, as with any powerful tool, challenges can arise. In this blog post, we will explore common async issues with CompletableFuture
and RxJava, along with effective troubleshooting techniques.
Table of Contents
- Understanding
CompletableFuture
- Common Issues with
CompletableFuture
- Issue 1: Blocking Calls
- Issue 2: Exception Handling
- Understanding RxJava
- Common Issues with RxJava
- Issue 1: Backpressure Management
- Issue 2: Threading
- Conclusion
- Further Reading
1. Understanding CompletableFuture
CompletableFuture
provides a powerful way to handle asynchronous computations. By allowing methods to execute in the background, it helps avoid blocking the main thread, which is crucial for responsive applications.
Example: Basic CompletableFuture Usage
Here’s a simple example to illustrate the usage:
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture.supplyAsync(() -> {
// Simulating a long-running task
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
return "Result of the asynchronous computation";
}).thenAccept(result -> {
System.out.println("Received: " + result);
});
// Prevent main thread from terminating before async task completes
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Commentary
In this code, supplyAsync
runs a computation asynchronously, and thenAccept
processes the result once it's complete. Without the sleep at the end, the main thread could terminate before our async computation finishes.
2. Common Issues with CompletableFuture
Issue 1: Blocking Calls
One common pitfall in asynchronous programming is inadvertently blocking calls. Using blocking I/O operations within the CompletableFuture
can lead to thread starvation and defeat the purpose of asynchronous execution.
How to Troubleshoot
- Avoid Blocking I/O: Place any blocking calls outside the asynchronous method.
- Use Non-blocking APIs: For data access, prefer non-blocking APIs that don’t block the calling thread.
Example of a Blocking Call
CompletableFuture.supplyAsync(() -> {
// This will block the async computation
return readFromDatabase(); // Simulated blocking call
});
Solution
Instead of blocking, consider using asynchronous database drivers that allow for non-blocking calls.
Issue 2: Exception Handling
Exceptions are another frequent issue when using CompletableFuture
. Unhandled exceptions can propagate and terminate your thread unexpectedly.
How to Troubleshoot
- Use
exceptionally
Method: To handle exceptions gracefully, attach an exception handler. - Chain with
handle
Method: Thehandle
method can manage both success and failure cases.
Example of Exception Handling
CompletableFuture.supplyAsync(() -> {
throw new RuntimeException("Oops, something went wrong!");
}).exceptionally(ex -> {
System.out.println("Caught exception: " + ex.getMessage());
return "Fallback result";
}).thenAccept(result -> {
System.out.println("Result: " + result);
});
Commentary
Here, throwing an exception in the asynchronous computation is handled gracefully with exceptionally
, ensuring that your app remains robust.
3. Understanding RxJava
RxJava is a popular framework inspired by Observer design principles that enable asynchronous programming using observable sequences. It allows composing complex asynchronous chains in a more intuitive and readable manner.
Example: Basic RxJava Usage
import io.reactivex.Observable;
public class RxJavaExample {
public static void main(String[] args) {
Observable.just("Hello", "Reactive", "World")
.subscribe(System.out::println);
}
}
Commentary
In this simple example, Observable.just
emits items, and subscribe
handles the emitted items in a streamlined manner.
4. Common Issues with RxJava
Issue 1: Backpressure Management
Handling backpressure is essential in RxJava, especially when consumers cannot keep up with producers. Failure to manage backpressure can lead to memory issues and application crashes.
How to Troubleshoot
- Use Buffering or Windowing: Employ backpressure strategies like buffering or windowing.
- Adjust the Scheduler: If a chain is facing backpressure, consider adjusting the threading model using different schedulers.
Example of Buffering
Observable.range(1, 10000)
.onBackpressureBuffer() // Buffering elements
.observeOn(Schedulers.io())
.subscribe(System.out::println);
Issue 2: Threading
Optimally selecting threads for executing your observable chains can improve performance significantly. Improper thread management often leads to unnecessary context switching and performance degradation.
How to Troubleshoot
- Use Appropriate Schedulers: Employ the right Schedulers (e.g.,
Schedulers.io()
for IO-bound work,Schedulers.computation()
for CPU-bound work). - Avoid Blocking Calls: Just like with
CompletableFuture
, blocking calls should be avoided within RxJava streams.
Example of Switching Threads
Observable.just("Data")
.subscribeOn(Schedulers.io()) // Subscribe in a background thread
.observeOn(Schedulers.computation()) // Observe computational results
.subscribe(result -> System.out.println("Processed " + result));
Commentary
By judiciously using different schedulers, you ensure that your application remains responsive while harnessing the full power of asynchronous processing.
5. Conclusion
Engaging with asynchronous programming using CompletableFuture
and RxJava can greatly enhance your Java applications. However, it’s critical to be mindful of common issues like blocking calls, exception handling, backpressure, and threading.
When working with either technology, familiarize yourself with best practices and troubleshooting techniques to mitigate potential problems. Using examples and commentary, we’ve illustrated how to address these challenges effectively.
6. Further Reading
For more information on CompletableFuture
and RxJava, explore the following resources:
With knowledge of these concepts and their practical applications, you can build responsive, robust, and efficient Java applications that thrive on asynchronous programming.
Checkout our other articles