Key Mistakes to Avoid When Implementing Enterprise Caching
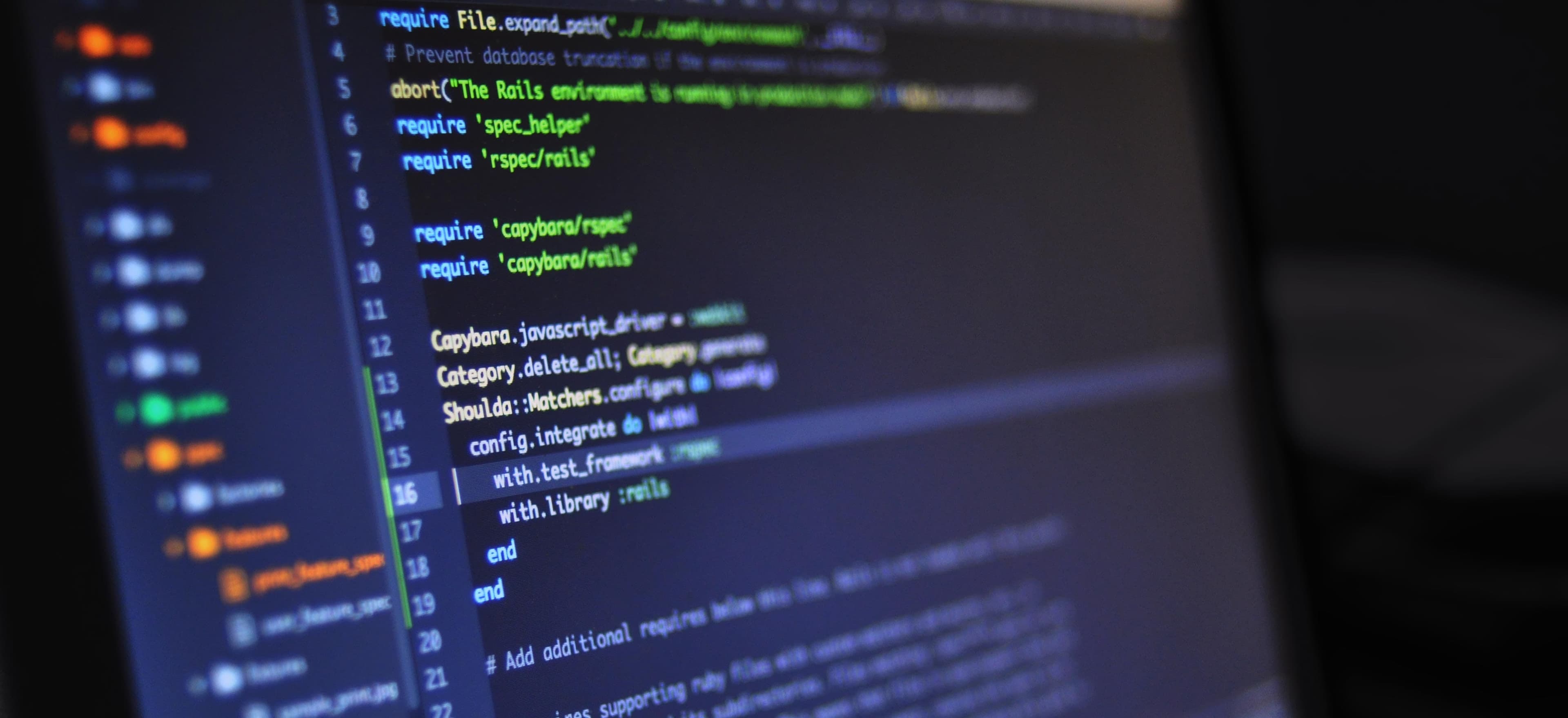
- Published on
Key Mistakes to Avoid When Implementing Enterprise Caching
In the world of enterprise applications, performance is crucial. Slow applications can lead to decreased user satisfaction and, ultimately, loss of revenue. One of the most effective strategies to enhance application performance is enterprise caching. However, the implementation of caching is not without pitfalls. In this blog post, we will explore key mistakes that organizations often make when implementing enterprise caching and how to avoid them.
Understanding the Basics of Caching
Before diving into the common mistakes, let's take a brief look at what caching is. Caching is the process of storing data in a temporary storage area (the cache) to reduce latency and improve data retrieval speeds. In enterprise applications, caching can significantly improve performance when implemented correctly.
Why Use Caching?
-
Performance Improvement: Caching dramatically reduces response times by serving data from a fast storage layer, rather than fetching it from the database for every request.
-
Scalability: Caching helps applications to handle more simultaneous users by reducing load on the back-end systems.
-
Cost-Efficiency: By decreasing the need for powerful database queries, caching can lead to lower operating costs.
Key Mistakes to Avoid
1. Not Setting Clear Caching Objectives
Mistake: Implementing caching without clear objectives can lead to misallocation of resources and confused team members.
Solution: Define the goals of your caching strategy upfront. Are you looking to improve load times, reduce database load, or enhance user experience? Setting clear objectives helps guide your approach, prioritizing caching within areas that provide the most significant return on investment.
2. Ignoring Cache Invalidation Strategies
Mistake: One of the most critical aspects of caching is invalidation. When underlying data changes, the cached data can become stale. Failing to implement an appropriate cache invalidation strategy can result in users getting outdated information.
Solution: Utilize techniques such as time-based expiration, write-through caches, or manual invalidation. Here’s a short look at how these strategies might be implemented in Java.
public class CacheManager {
private Map<String, CacheEntry> cache = new HashMap<>();
public void put(String key, Object value, long ttl) {
cache.put(key, new CacheEntry(value, System.currentTimeMillis() + ttl));
}
public Object get(String key) {
CacheEntry entry = cache.get(key);
if (entry != null) {
if (entry.isExpired()) {
cache.remove(key);
return null; // or fetch from DB
}
return entry.getValue();
}
return null; // or fetch from DB
}
private class CacheEntry {
private Object value;
private long expiryTime;
public CacheEntry(Object value, long expiryTime) {
this.value = value;
this.expiryTime = expiryTime;
}
public Object getValue() {
return value;
}
public boolean isExpired() {
return System.currentTimeMillis() > expiryTime;
}
}
}
This straightforward implementation uses a time-to-live (TTL) strategy to keep the cache fresh, ensuring that expired entries are automatically removed.
3. Overlooking the Size of the Cache
Mistake: Failing to define a maximum size for your cache can lead to significant performance issues. An unbounded cache grows indefinitely, consuming resources and potentially causing memory overflow.
Solution: Implement a cache eviction policy, such as Least Recently Used (LRU) or Least Frequently Used (LFU). An example of an LRU Cache in Java is shown below.
import java.util.LinkedHashMap;
import java.util.Map;
public class LRUCache<K, V> extends LinkedHashMap<K, V> {
private final int capacity;
public LRUCache(int capacity) {
super(capacity, 0.75f, true);
this.capacity = capacity;
}
@Override
protected boolean removeEldestEntry(Map.Entry<K, V> eldest) {
return size() > capacity;
}
}
This implementation automatically evicts the least recently used entry when the cache exceeds its specified capacity, ensuring efficient memory use.
4. Caching Too Much Data
Mistake: While it may seem advantageous to cache as much data as possible, excessive caching can lead to increased complexity and reduced performance.
Solution: Focus on caching only the most frequently accessed data. Perform an analysis of your application's data access patterns to identify which data is worth caching. For example, consider caching user sessions or configuration settings, while avoiding caching large binary files that are rarely accessed.
5. Skipping Monitoring and Analytics
Mistake: Not tracking cache performance can lead to blind spots in your application’s effectiveness. You may not realize that your caching strategy is failing until it is too late.
Solution: Implement monitoring and logging to gain insights into cache hits and misses. Tools like New Relic and Prometheus can be integrated to provide detailed analytics. Monitoring helps identify trends and optimize your caching strategy over time.
6. Failure to Test Caching
Mistake: Often, developers may overlook caching during the testing phase, assuming it will automatically work in production.
Solution: Create a robust testing strategy that includes unit tests and performance tests specifically for caching behavior. Simulate various scenarios: data retrieval, cache hit, cache eviction, and cache invalidation. Here is a simple example of a unit test for a cache.
import static org.junit.Assert.*;
import org.junit.Before;
import org.junit.Test;
public class CacheManagerTest {
private CacheManager cacheManager;
@Before
public void setUp() {
cacheManager = new CacheManager();
}
@Test
public void testCacheEviction() {
cacheManager.put("key1", "value1", 100); // TTL 100ms
try { Thread.sleep(50); } catch (InterruptedException e) {}
assertEquals("value1", cacheManager.get("key1"));
try { Thread.sleep(60); } catch (InterruptedException e) {}
assertNull(cacheManager.get("key1")); // should be expired
}
}
The above unit test verifies that data is indeed evicted from the cache after its TTL has expired.
7. Not Considering Distributed Caching
Mistake: Failing to think about distributed caching when working with a large-scale or microservices architecture can result in performance bottlenecks.
Solution: Consider using distributed caching solutions like Redis, Memcached, or Hazelcast. These technologies allow multiple application instances to tap into the same cache, facilitating efficient data retrieval across services. For example, using Redis in Java could look like this:
import redis.clients.jedis.Jedis;
public class RedisCache {
private Jedis jedis;
public RedisCache() {
this.jedis = new Jedis("localhost");
}
public void set(String key, String value) {
jedis.set(key, value);
}
public String get(String key) {
return jedis.get(key);
}
}
By integrating Redis, you can easily utilize distributed caching within your enterprise applications.
Final Thoughts
Implementing enterprise caching comes with its own set of challenges. By avoiding common mistakes such as failing to set clear objectives, neglecting cache invalidation, overlooking the cache size, caching too much data, skipping monitoring, not testing adequately, and forgetting about distributed caching, you can significantly improve the performance of your applications.
Key Takeaway: Always remember that caching is not a one-size-fits-all solution. Tailor your caching strategy to fit your specific use case, constantly refine it, and monitor it for optimal results. As you navigate the complexities of enterprise caching, these best practices will help ensure your efforts lead to successful outcomes.
For more detailed insights, consider reading resources on caching best practices.
Happy caching!
Checkout our other articles