The Hidden Challenges of Garbage Collection Analysis
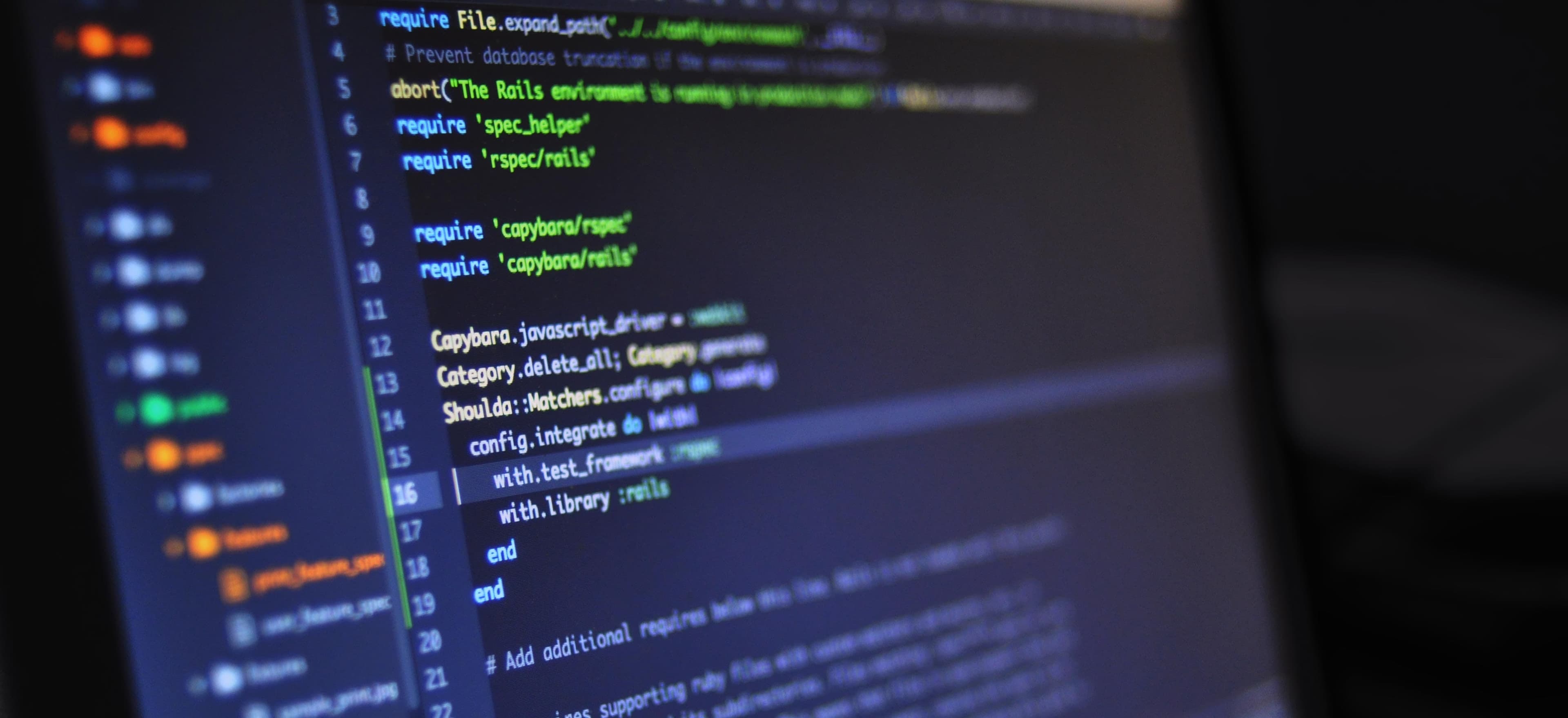
- Published on
The Hidden Challenges of Garbage Collection Analysis in Java
Garbage Collection (GC) in Java is both a powerful feature and a hidden complexity that can lead to performance bottlenecks and unexpected behaviors. While many developers utilize garbage collection without a deep understanding of its intricacies, the impact of GC on application performance cannot be understated. In this blog post, we will delve into the challenges associated with analyzing garbage collection, its implications for Java applications, and strategies to tackle these challenges effectively.
Understanding Garbage Collection
Garbage Collection is an automatic memory management feature in Java that helps reclaim memory occupied by objects that are no longer in use. The JVM (Java Virtual Machine) periodically checks for unreachable objects and frees up memory, thus preventing memory leaks and runtime crashes.
Why Garbage Collection Matters
The way in which garbage collection is handled can significantly affect the performance of Java applications. Unoptimized GC processes can lead to:
- Increased Latency: Unexpected pauses in application performance.
- Higher CPU Utilization: Excessive processing time spent on collecting garbage.
- Memory Leaks: Unreleased memory leading over time to out-of-memory errors.
Common JVM Garbage Collectors
Java offers several garbage collection algorithms, each with its specific use cases:
- Serial Garbage Collector: A single-threaded collector that is good for small applications and environments with limited resources.
- Parallel Garbage Collector: Uses multiple threads for minor garbage collection, suitable for applications that require high throughput.
- CMS (Concurrent Mark-Sweep) Collector: Designed for low-latency applications by performing much of the garbage collection concurrently.
- G1 (Garbage First) Collector: Aimed at large applications by partitioning the heap into regions and collecting incrementally.
For further reading about the different garbage collectors, explore the Oracle documentation.
The Hidden Challenges of Garbage Collection Analysis
While garbage collection plays a crucial role in memory management, analyzing its behavior presents multiple challenges:
1. Complex Metrics
Garbage collectors produce various metrics, including pause times, heap size, and allocation rates. Changing any one of these can have cascading effects on performance, making it difficult to correlate specific actions with performance outcomes.
System.gc(); // Request garbage collection, but it is not guaranteed to run immediately.
Why: The System.gc() call suggests that the JVM performs garbage collection, yet it does not guarantee execution. Over-reliance on this method can lead to unpredictable performance.
2. Non-Deterministic Behaviors
Garbage collection runs are often non-deterministic. The JVM decides when to run the garbage collector based on its own heuristics, which may not align with application needs.
- Unpredicted pauses can happen during high load.
- Applications may require real-time performance guarantees.
3. Multi-threading Issues
In a multi-threaded application, accessing shared variables during garbage collection can lead to inconsistencies if not handled correctly. This requires careful synchronization, which can introduce its overhead, complicating the scenario further.
class MyConcurrentClass {
private volatile int sharedResource;
public void increment() {
sharedResource++; // Potentially problematic during GC.
}
}
Why: The volatile keyword ensures visibility across threads, but during garbage collections, if threads are paused, updates may not be flushed as expected.
4. Memory Footprints
Memory footprint analysis regulates how much Java heap memory your application uses. While it is important to monitor usage, misinterpretation can lead to over-provisioning memory, causing wasted resources or even impacting performance.
5. Tool Limitations
There are several tools available for monitoring and analyzing garbage collection, including:
- JVisualVM
- JConsole
- Garbage Collection Logs (GC logs)
However, understanding the output from these tools can be challenging. Not all data may be relevant, and interpreting it requires familiarity with JVM internals.
Strategies for Effective Garbage Collection Analysis
1. Enable Detailed GC Logging
Modern JVMs allow for detailed GC logs to be generated, providing insights into garbage collection events. You can enable logging via JVM parameters:
java -XX:+PrintGCDetails -XX:+PrintGCTimeStamps -Xloggc:mygc.log -jar MyApp.jar
Why: By logging GC details and timestamps, it becomes easier to analyze the frequency and duration of garbage collection over time.
2. Analyze Metrics with Tools
Utilizing tools like Elastic APM can help aggregate logs and visualize metrics efficiently. They can create dashboards that simplify tracking impacts over time.
3. Optimize Object Creation
Minimizing the creation of temporary objects can significantly reduce GC pressure on the heap. Utilizing object pools or caching frequently used objects can mitigate this.
class ObjectPool {
private List<MyObject> availableObjects = new ArrayList<>();
public MyObject acquire() {
// Reuse an object instead of creating a new one
return availableObjects.isEmpty() ? new MyObject() : availableObjects.remove(0);
}
public void release(MyObject obj) {
availableObjects.add(obj); // Add back to the pool
}
}
Why: This strategy reduces the frequency of allocations and subsequently minimizes garbage collection cycles.
4. Tuning JVM Parameters
Adjusting JVM parameters can also lead to better performance. Parameters like the heap size can be tweaked based on application load. For example:
java -Xms512m -Xmx2048m -jar MyApp.jar
Why: Setting the initial and maximum heap size can lead to more stable garbage collection cycles.
5. Regular Performance Testing
Conducting regular performance tests under varying loads can help reveal how your application behaves with respect to garbage collection. Tools like JMeter can be used for stress-testing and identifying potential bottlenecks.
Final Considerations
The complexity of garbage collection in Java poses challenges that require careful analysis and strategic handling. Understanding the intricacies of metric interpretation, behavioral predictability, and multi-threading effects is crucial for optimizing performance. By employing effective strategies, developers can navigate these hidden challenges successfully and enhance their application's performance.
For continuous learning, consider diving deeper into JVM internals and GC algorithms. Resources like the Java Performance Tuning Book can provide further insight into this critical aspect of Java programming.
By conducting thorough garbage collection analysis, developers can become better stewards of application performance, ensuring robust, efficient, and high-quality Java applications.
Checkout our other articles