Unlocking Java 8: Choosing the Best Garbage Collector for Speed
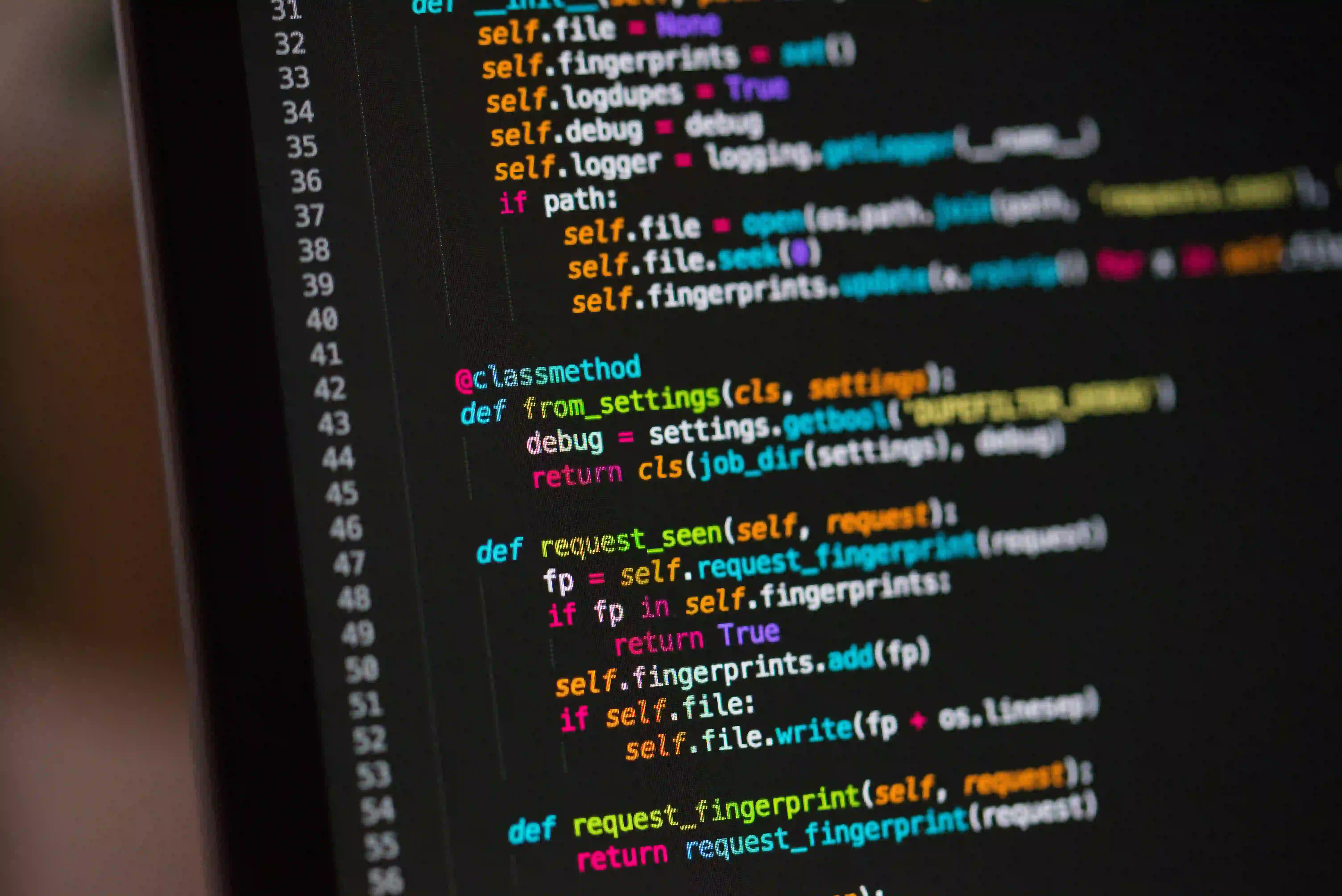
Unlocking Java 8: Choosing the Best Garbage Collector for Speed
In the ever-evolving world of Java, one critical aspect that developers need to understand is the Garbage Collector (GC). With the release of Java 8, there were significant updates and improvements in garbage collection mechanisms. The choice of the right garbage collector can markedly affect the performance of your applications, particularly in terms of speed. This blog post aims to explore the various garbage collectors available in Java 8, their features, and how to choose the one that best fits your needs.
Understanding Garbage Collection in Java
Garbage Collection is an automatic process of reclaiming memory by deleting objects that are no longer in use. The primary goal is to free up resources and prevent memory leaks. Java uses several garbage collection algorithms, each with unique performance characteristics and tuning parameters.
Why is Garbage Collection Important?
- Automatic Memory Management: Developers can focus on writing code without worrying about manual memory allocation and deallocation.
- Performance Optimization: A well-tuned Garbage Collector can considerably enhance application performance by ensuring efficient memory usage.
- Scalability: The right GC can enable applications to scale seamlessly, handling varying loads and request patterns efficiently.
Types of Garbage Collectors in Java 8
Java 8 introduced a variety of garbage collectors. Understanding each is essential for making an informed decision. Here's a brief rundown of the primary options:
1. Serial Garbage Collector
The Serial GC is designed for single-threaded applications. It's simple, using a single thread for all garbage collection tasks. This can make it advantageous for applications with small datasets or environments with limited resources.
Pros:
- Low overhead
- Simple implementation
Cons:
- Not suitable for multi-threaded applications
- Can lead to long pause times
Usage Example:
java -XX:+UseSerialGC -jar YourApp.jar
2. Parallel Garbage Collector (Throughput Collector)
The Parallel GC aims to maximize throughput by utilizing multiple threads for both minor and major collections. It is particularly effective for batch processing applications where throughput is critical.
Pros:
- Efficient for multi-core architectures
- Reduces the time spent in garbage collection
Cons:
- May lead to long pauses during full garbage collection
- Tuning can be complex
Usage Example:
java -XX:+UseParallelGC -jar YourApp.jar
3. Concurrent Mark-Sweep (CMS) Collector
The CMS collector is designed for applications that require low pause times. It uses multiple threads for garbage collection while the application continues running, minimizing latency.
Pros:
- Fast collection phases result in lower application pauses
- Good for interactive applications
Cons:
- Can lead to fragmentation
- More CPU-intensive
Usage Example:
java -XX:+UseConcMarkSweepGC -jar YourApp.jar
4. G1 (Garbage First) Collector
The G1 GC is designed for applications with large heaps and aims to provide predictable pauses. It divides the heap into regions and prioritizes collection based on the most garbage collected first.
Pros:
- Predictable pause times
- Handles large heaps efficiently
Cons:
- Complexity in tuning
- May not perform as well as others in specific scenarios
Usage Example:
java -XX:+UseG1GC -jar YourApp.jar
How to Choose the Best Garbage Collector
Selecting the best garbage collector for your application depends on various factors. Here are some considerations to guide your decision:
Application Type
- Single-threaded Applications: Opt for the Serial GC.
- Large Analytics or Batch Jobs: Choose the Parallel GC for higher throughput.
- Low Latency Requirements: CMS or G1 would be more appropriate.
Memory Footprint
If your application has a large memory footprint, the G1 collector might be the best option due to its efficient memory management and regional garbage collection strategy.
Performance Requirements
Consider not just the speed of garbage collections but the application's overall performance. Monitor and conduct tests to determine the best trade-offs between CPU usage and pause times.
Tuning Capabilities
Java offers various flags that allow you to tune garbage collectors to fit your needs. Here are a couple of useful flags for tuning:
-Xmx
: Sets the maximum heap size.-XX:MaxGCPauseMillis
: Targets the maximum time your application spends in garbage collection.
A Practical Example
Here's how to select the G1 collector for an application that requires low pause time, while also making use of tuning flags for optimal performance.
java -XX:+UseG1GC -Xmx2g -Xms1g -XX:MaxGCPauseMillis=200 -jar YourApp.jar
In this command:
- The G1 collector is employed,
- The maximum heap size is set to 2GB,
- The minimum heap size is 1GB,
- The maximum pause time is limited to 200 milliseconds.
This configuration can help ensure that your application performs smoothly under varying loads.
Monitoring Garbage Collection
Once you have selected your garbage collector and adjusted your parameters, it's crucial to monitor its performance. Tools like Java VisualVM and Garbage Collection Logs can provide insights into how well your GC is managing memory. Enable GC logging by adding the following flags:
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -Xloggc:gc.log
This will generate logs that can help diagnose performance issues related to garbage collection.
To Wrap Things Up
Choosing the right Garbage Collector in Java 8 can be a game-changing decision for your application's performance. By understanding the strengths and weaknesses of each GC option, and tuning them appropriately, you can optimize your Java applications for speed and efficiency.
For further reading on Java's memory management and the nuances of garbage collection, keep an eye on the following resources:
- Java Documentation on Garbage Collection
- Performance Tuning with Java Garbage Collection
With careful selection and tuning, you can unlock the full potential of your Java applications by effectively managing memory through intelligent garbage collection strategies. Happy coding!