Is TDD Really Cost-Effective for Your Team?
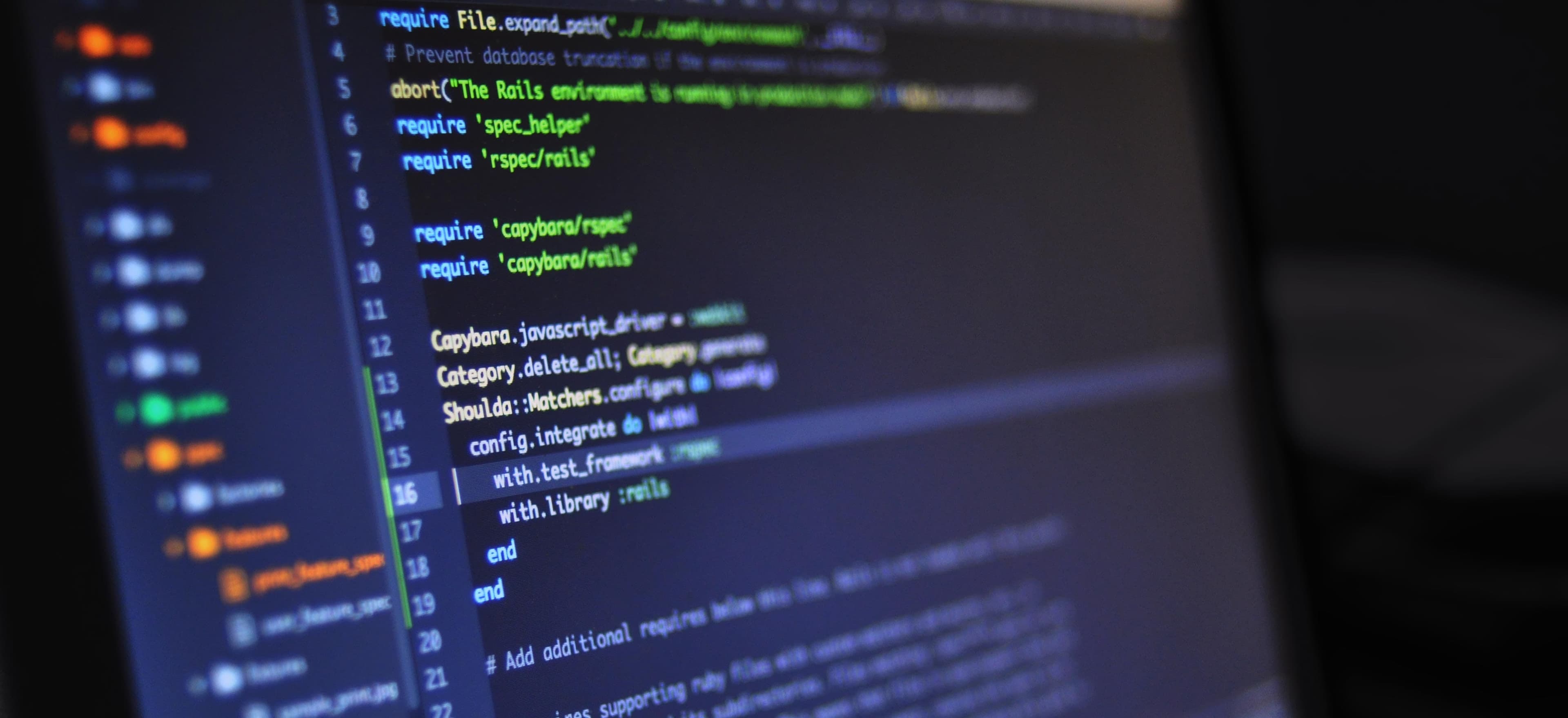
- Published on
Is TDD Really Cost-Effective for Your Team?
Test-Driven Development (TDD) is a software development methodology that emphasizes writing tests before writing the corresponding code. It’s a concept that has stirred debates about its cost-effectiveness over time. This blog post will discuss both the pros and cons of TDD, delve into its implications for team dynamics and productivity, and provide examples to illustrate how it can be implemented using Java.
What is TDD?
To understand whether TDD is cost-effective for your team, it's crucial to grasp its core principles. TDD follows a simple cycle known as Red-Green-Refactor:
- Red: Write a failing test for a new feature.
- Green: Write the minimum code needed to pass the test.
- Refactor: Optimize and clean up the code while keeping tests green.
This cycle allows developers to maintain a safety net of tests that can help ensure that code changes do not introduce new bugs.
The Benefits of TDD
-
Improved Code Quality: TDD encourages writing code that is modular and easily testable. By designing code with tests in mind, developers are often led to create better-organized and cleaner code.
-
Fewer Bugs: Since tests are written before the code, TDD can help catch bugs early in the development process. Errors found during the development of a feature tend to be easier to fix than those found later.
-
Clear Requirements: TDD forces developers to think critically about the requirements from the beginning. When writing tests, a developer is essentially defining what success means for a specific functionality, creating a clear understanding of what is to be built.
-
Documentation: The test cases themselves serve as documentation. They illustrate how the code should behave, making it easier for new team members to understand the codebase.
The Downsides of TDD
-
Initial Time Investment: Writing tests before writing code can slow down the initial phases of development. The time spent on tests can feel excessive compared to the direct coding efforts.
-
Learning Curve: If your team is not familiar with TDD, there will be a learning curve involved. Teams new to this methodology may struggle to adapt their existing workflows, potentially leading to project delays.
-
Test Maintenance: As with any codebase, test code can become difficult to maintain. Over time, the costs associated with keeping tests updated can offset some of the benefits.
Is TDD Cost-Effective?
To answer this question, we need to consider the context of your specific team and project.
-
Team Experience and Expertise: If your team has experience with TDD, the short-term costs of writing tests can be mitigated by the long-term benefits of fewer bugs, better code quality, and clearer requirements.
-
Nature of the Project: TDD shines in projects with changing requirements or frequent updates. For stable projects, the initial overhead might not justify the long-term benefits.
-
Time to Market: For projects where speed is crucial, TDD may initially seem costly. However, many teams find that the bug-catching capabilities of TDD actually lead to faster delivery of final products.
Implementing TDD in Your Java Projects
Example of TDD in Java
Let’s see how TDD can be applied in a Java context through a simple example of a service that calculates the sum of two numbers.
Step 1: Write the Test (Red)
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "Sum should be 5");
}
}
Commentary: Initially, this test for the add
method fails because the Calculator
class has not been implemented yet. However, it clearly defines the expected behavior, which is to produce a sum of 5 when adding 2 and 3.
Step 2: Write the minimal code (Green)
public class Calculator {
public int add(int a, int b) {
return a + b; // the simplest implementation achieves what's needed for the test to pass
}
}
Commentary: The implementation of the add
method is straightforward. It does what the test expects, and at this point, all tests should pass.
Step 3: Refactor
public class Calculator {
public int add(int a, int b) {
return Integer.sum(a, b); // using built-in method for clarity
}
}
Commentary: During the refactoring phase, we opted to use Integer.sum()
. This makes the intention clearer and the code cleaner. The test remains intact, ensuring that we haven't broken any existing functionality.
In Conclusion, Here is What Matters: TDD’s Cost-effectiveness
In conclusion, the cost-effectiveness of Test-Driven Development largely depends on your team’s familiarity with the methodology, the nature of your projects, and the long-term goals of your software development lifecycle.
While TDD can potentially slow down the initial development phases, its long-term benefits in terms of code quality, reduced bugs, and maintainability often outweigh those early costs. TDD is a valuable practice for creating robust, high-quality Java applications if implemented correctly.
If you are still questioning if TDD is right for your team, start small. Implement it on a new feature or a small project and measure the results. The insights gathered will guide your larger architecture decisions and possibly validate the cost-effectiveness of TDD for your team.
Feel free to explore more about TDD on resources like the Martin Fowler's blog or JUnit Documentation for more in-depth knowledge.
Happy coding!