Overcoming Common Development Roadblocks for Success
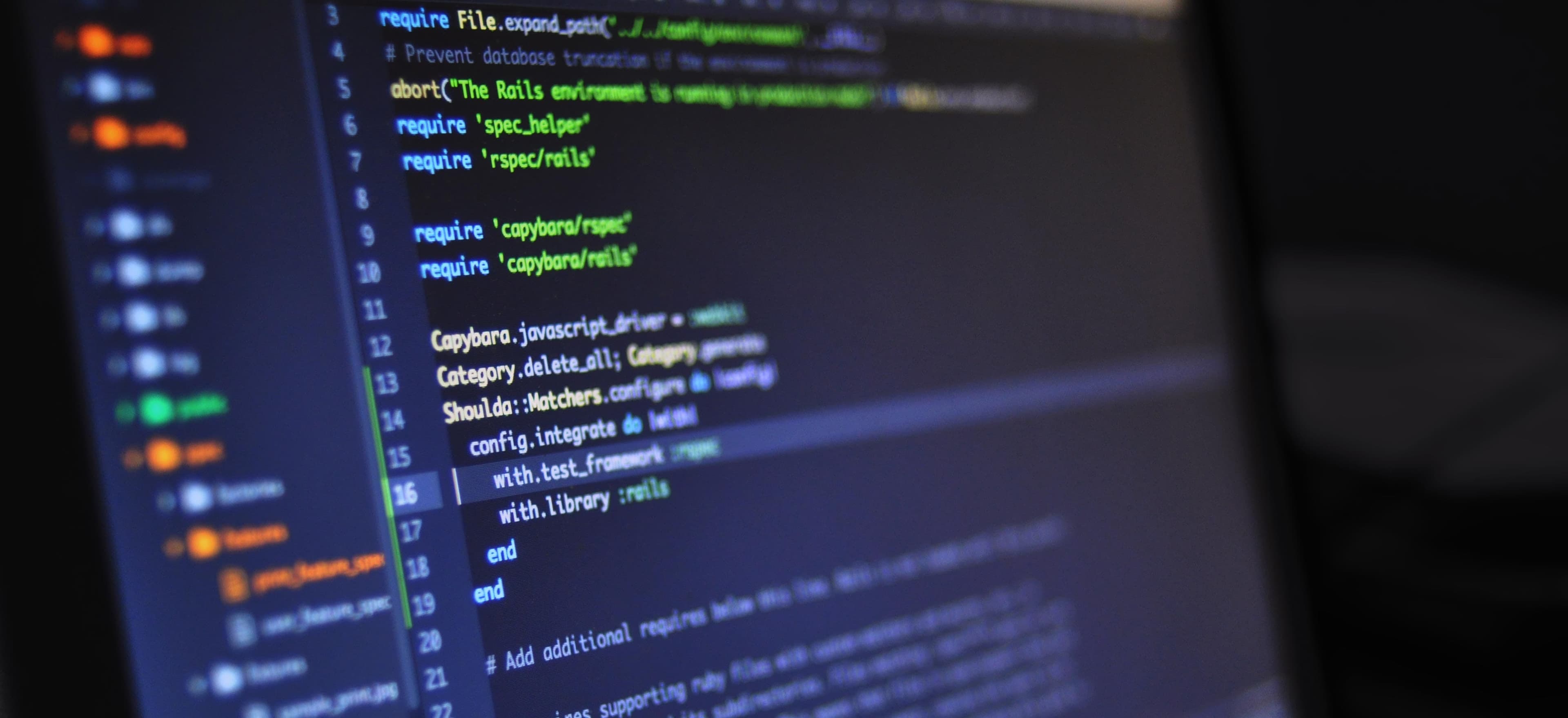
- Published on
Overcoming Common Development Roadblocks for Success in Java
In the ever-evolving field of software development, navigating obstacles is a common theme. Java, being one of the most widely used programming languages, presents developers with its own unique set of challenges. This blog post aims to illuminate common roadblocks encountered in Java development, providing practical solutions to overcome these hurdles and achieve greater success in your programming endeavors.
Understanding the Common Roadblocks
Before we delve into solutions, let’s identify some common development roadblocks you may encounter while working with Java.
1. Compiling Errors
Compiling errors can be a sore point for new and seasoned developers alike. These errors often occur due to missing semicolons, mismatched brackets, or incorrect syntax. Compiler errors can derail your workflow, leading to frustration.
2. Dependency Management
In large Java projects, managing dependencies can be complex. Conflicting libraries or incorrect versioning can lead to a compromised application. The beauty of Java’s ecosystem lies in its libraries, but that very abundance can foster inconsistencies.
3. Performance Issues
Performance is a critical aspect of any application. Java, although renowned for its speed and efficiency, is not immune to performance pitfalls. Inefficient algorithms or misuse of memory can lead to lagging applications.
4. Debugging Challenges
Debugging is an integral part of the development process. However, without a systematic approach, tracing the source of a bug can turn into a daunting task.
5. Lack of Documentation
Good documentation is essential for successful software development. Lack of comments in the code or insufficient API documentation can make it challenging for developers to understand existing codebases.
Now that we’ve identified these roadblocks, let's explore the strategies to overcome them.
Strategies for Overcoming Development Roadblocks
1. Streamline Your Compiling Process
To mitigate compiling errors, a strong understanding of Java syntax is fundamental. Additionally, utilizing Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse can greatly reduce compiling errors.
Example: Using an IDE
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
IntelliJ IDEA will highlight syntax errors in real-time, allowing you to correct them before attempting to compile.
Why This Matters
A robust IDE not only improves your coding accuracy but also provides tools for code completion, reducing the likelihood of syntax errors.
2. Effective Dependency Management with Maven
Java projects often involve multiple third-party libraries. Utilizing a build automation tool like Maven can simplify dependency management.
To set up Maven, create a pom.xml
file:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>Demo</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.9</version>
</dependency>
</dependencies>
</project>
Why This Matters
Maven handles version conflicts and can download dependencies automatically, saving you from manually managing libraries across various projects. For more information about Maven, check this resource.
3. Improving Performance
To tackle performance issues, consider the following best practices:
- Choose the right data structures: Some data structures are more efficient than others based on the specific use case.
- Minimize object creation: Frequent object creation can lead to memory issues.
- Profile your application using tools like VisualVM or YourKit.
For instance:
List<String> names = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
names.add("Name " + i);
}
In this case, using ArrayList is efficient for sequential access, but if frequent insertions are needed, consider using LinkedList.
Why This Matters
Understanding the complexity of data structures and algorithms leads to more optimized applications, enhancing user satisfaction.
4. Adopting Structured Debugging Techniques
When debugging, structure your approach by:
- Replicating the issue.
- Isolating variables.
- Using conditional breakpoints.
Example: Using Breakpoints
public class DebugExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
// Set a breakpoint here to inspect 'numbers[i]'
}
}
}
Setting a breakpoint allows you to inspect variable states at runtime, leading to quicker resolution of issues.
Why This Matters
Having a structured debugging process helps maintain focus and reduces the anxiety often associated with troubleshooting.
5. Emphasizing Proper Documentation
Finally, proper documentation is indispensable. Adopting a standard comment style and maintaining up-to-date documentation within your code and on external platforms can ensure clarity.
For example:
/**
* Calculates the square of a number.
*
* @param number The number to be squared.
* @return The square of the input number.
*/
public int square(int number) {
return number * number;
}
Why This Matters
Good documentation facilitates maintenance and enhances collaboration between team members. A well-documented codebase reduces onboarding time for new developers.
Closing Remarks
Overcoming roadblocks in Java development requires a thoughtful approach and a willingness to adapt. By implementing strategies like error management through IDEs, utilizing Maven for dependency management, optimizing application performance, structured debugging techniques, and prioritizing documentation, you pave the way toward successful and efficient Java programming.
As you continue your journey in software development, the challenges you face will only serve as stepping stones to your growth. Embrace them, learn from them, and you will find success in the dynamic landscape of Java development.
Feel free to share your experiences and strategies in the comments below! For further reading on Java development best practices, explore Java Tutorials.
Happy coding!