Common Pitfalls of Method Overloading in Java
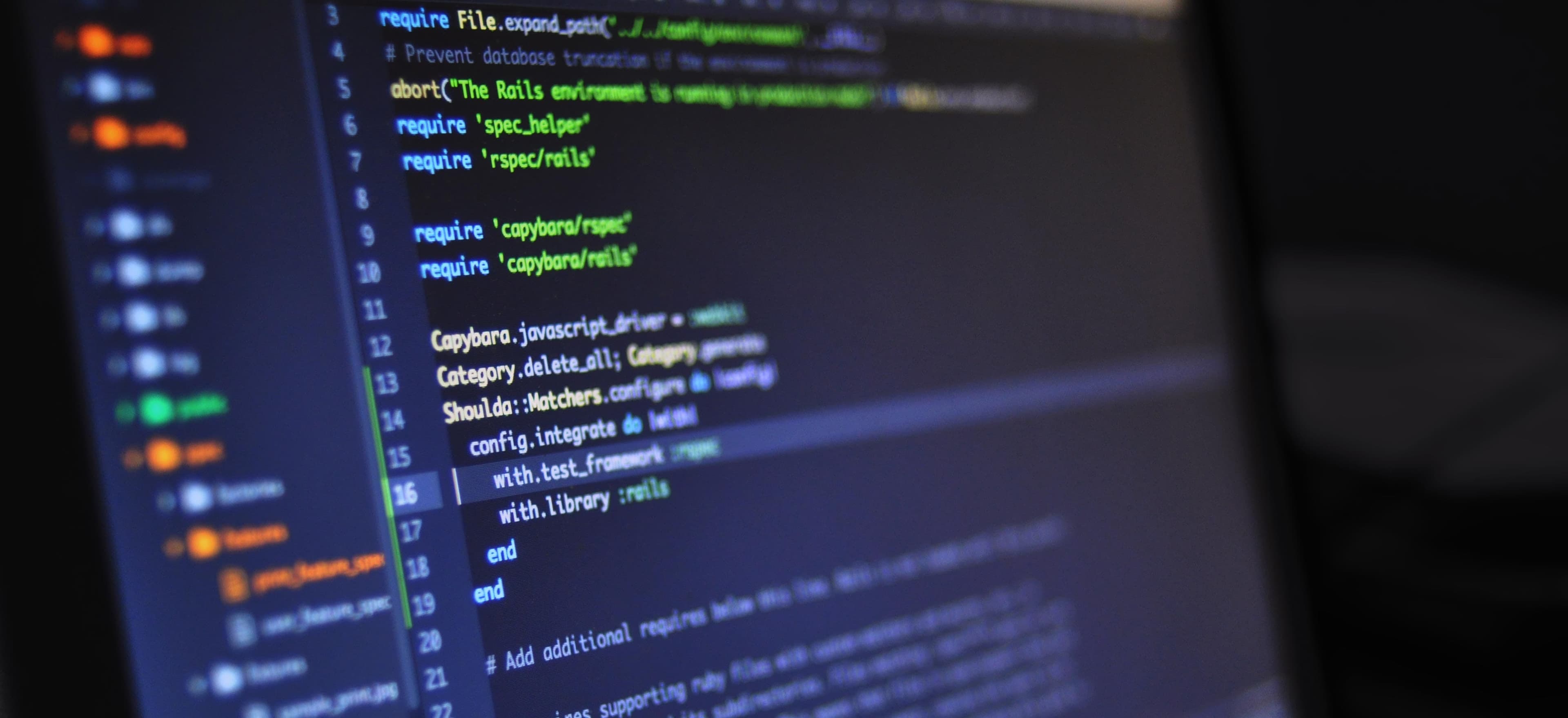
- Published on
Common Pitfalls of Method Overloading in Java
Java is a versatile and powerful programming language that allows developers to create robust applications. One feature that Java supports is method overloading, where multiple methods can have the same name but different parameters. While this can make code cleaner and more intuitive, it also introduces several pitfalls. In this blog post, we will explore these common pitfalls of method overloading in Java and how to avoid them.
What is Method Overloading?
Before diving into the pitfalls, let's clarify what method overloading is. Method overloading occurs when two or more methods in the same class have the same name but different parameter lists. The compiler differentiates these methods based on the number, type, and order of parameters.
Example of Method Overloading
Here’s a simple example to illustrate the concept of method overloading:
public class MathOperations {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
public int add(int a, int b, int c) {
return a + b + c;
}
}
In this example, we have three add
methods that differ in the types and number of parameters. This makes it easy to call the appropriate add
method based on the input parameters.
Pitfall 1: Ambiguity in Method Calls
One of the most common pitfalls in method overloading is ambiguity. This happens when the compiler cannot resolve which method to invoke because multiple methods match the call.
Example: Ambiguous Method Call
public class Test {
public void display(int a, float b) {
System.out.println("Integer and Float");
}
public void display(float a, int b) {
System.out.println("Float and Integer");
}
public static void main(String[] args) {
Test obj = new Test();
obj.display(10, 20); // Ambiguous call
}
}
In the above code, the call to obj.display(10, 20)
is ambiguous. The compiler cannot determine whether to call the display(int, float)
or display(float, int)
method. To avoid this issue, ensure that there are enough differences in method parameter types to uniquely identify each method.
How to Avoid:
- Consider changing the method signatures to reduce ambiguity.
- Use distinct parameter types to guide the compiler.
Pitfall 2: Confusion with Automatic Type Promotion
Java has an automatic type promotion feature, but it can lead to unexpected behavior in overloaded methods. This occurs when primitives are promoted during method calls.
Example: Type Promotion
public class OverloadExample {
public void print(int a) {
System.out.println("Integer: " + a);
}
public void print(double a) {
System.out.println("Double: " + a);
}
public static void main(String[] args) {
OverloadExample obj = new OverloadExample();
obj.print(9); // Calls print(int)
obj.print(9.5); // Calls print(double)
obj.print(9.0f); // Calls print(double), not print(int)
}
}
In this example, passing a float (9.0f) causes the method to invoke print(double)
, not the intended print(int)
. This can create confusion if not handled properly.
How to Avoid:
- Ensure explicit casts are used in cases where type promotion can affect method resolution.
- Clearly document the expected data types for method parameters.
Pitfall 3: Varied Parameter Successions
Another notable pitfall is related to the order of parameters when overloading methods. If the same types of parameters are used but in different sequences, it can lead to complications during method calls.
Example: Order of Parameters
public class ParameterOrder {
public void process(float f, int i) {
System.out.println("Float & Integer");
}
public void process(int i, float f) {
System.out.println("Integer & Float");
}
public static void main(String[] args) {
ParameterOrder obj = new ParameterOrder();
obj.process(5.0f, 10); // Calls process(float, int)
obj.process(10, 5.0f); // Calls process(int, float)
}
}
In this case, the order significantly changes the invoked method, leading to potential confusion.
How to Avoid:
- Be cautious when using similar types in different orders.
- Consider providing clear method names that describe the expected parameter order.
Pitfall 4: Overloading with Varargs
Using varargs (variable-length arguments) can be a source of confusion in overloaded methods. This is because a method accepting varargs is a "catch-all," which can easily overshadow other overloaded methods.
Example: Varargs Overloading
public class VarargsExample {
public void show(int... numbers) {
System.out.println("Varargs Method");
}
public void show(int number) {
System.out.println("Single Integer Method");
}
public static void main(String[] args) {
VarargsExample obj = new VarargsExample();
obj.show(5); // Calls single integer method
obj.show(5, 10); // Calls varargs method
}
}
In this example, if you pass a single integer, the show(int)
method is called instead of show(int...)
, which can be misleading.
How to Avoid:
- Avoid relying heavily on varargs in overloaded scenarios.
- Use clear names for methods or remove ambiguity to ensure clarity.
Pitfall 5: Readability and Maintainability
Overloading can make the code less readable, especially when multiple methods share the same name but have unclear differences in parameters.
Example: Readability
public class User {
public void updateUser(String name) { /* ... */ }
public void updateUser(int id) { /* ... */ }
public void updateUser(String name, int id) { /* ... */ }
}
While overloading here works, it can be hard to decipher which updateUser
method to call based on the parameters supplied—especially as the project grows.
How to Avoid:
- Use method names that succinctly signify the action being taken.
- When possible, favor different method names over overloading, especially in larger classes.
Summary of Avoiding Overloading Pitfalls
- Avoid ambiguity. Ensure method signatures can be clearly distinguished.
- Be cautious with type promotions. Always check the implications of data types.
- Consider parameter order. Be aware of how swapping parameters affects method resolution.
- Use varargs judiciously. Clarify when and how to use variable-length arguments.
- Prioritize readability. Clear method names can often replace the need for overloading.
Overloading is a powerful feature in Java that, when used correctly, can significantly enhance code clarity and usability. However, being aware of these common pitfalls will make your code less prone to confusion and more maintainable in the long run.
For a deeper dive into Java methods and best practices, check out Java Method Overloading and explore other resources available for mastering Java.
By staying conscious of these pitfalls, you will create more robust, error-free, and easier-to-understand code—a good practice for any Java developer.