Overcoming Docker Logging Challenges in Jenkins CI/CD
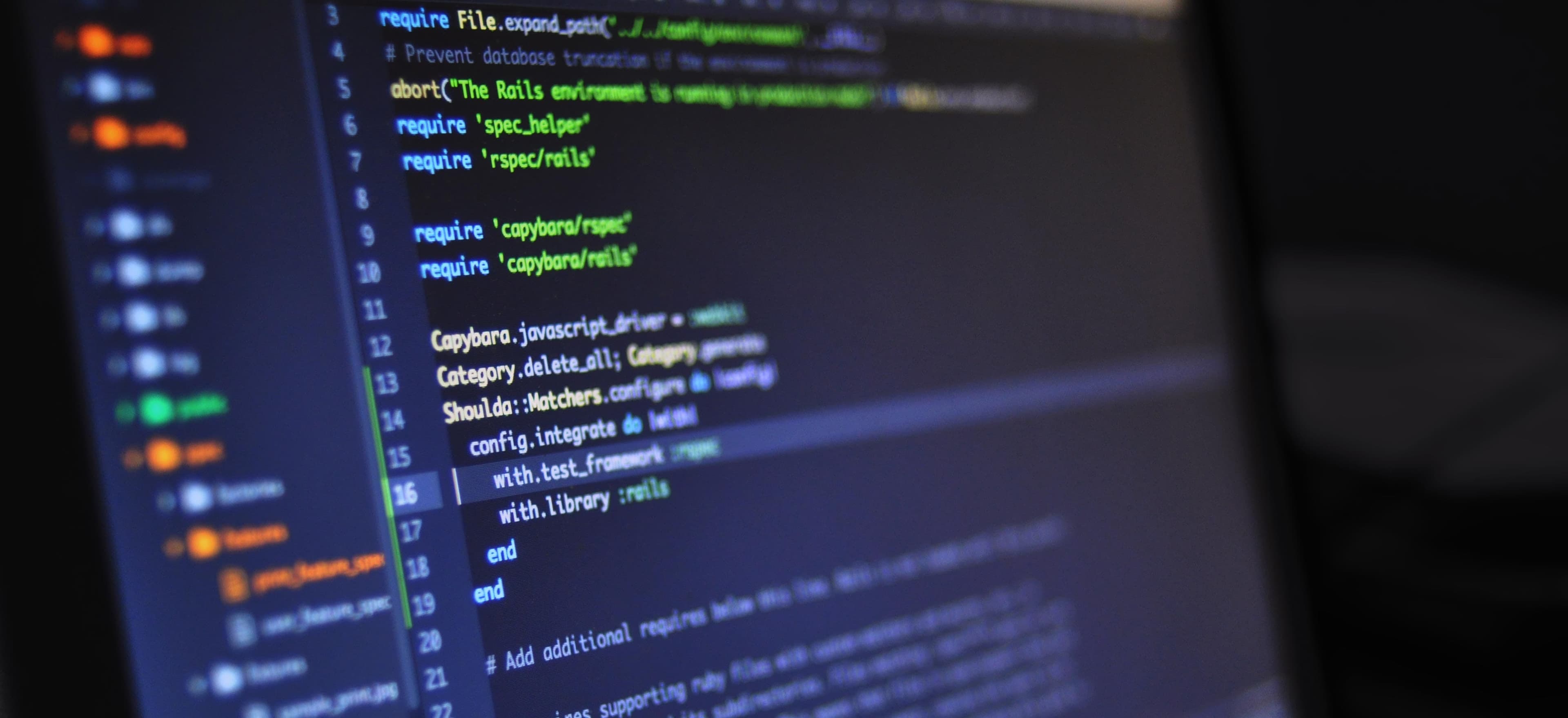
- Published on
Overcoming Docker Logging Challenges in Jenkins CI/CD
In the world of DevOps, the need for efficient logging has become increasingly critical. Logging helps teams monitor application health, troubleshoot issues, and gain insights into usage patterns. For teams utilizing Jenkins as their CI/CD tool in Docker containers, there are unique logging challenges that can arise. In this blog post, we’ll explore how to overcome these challenges effectively, ensuring that your CI/CD pipelines remain robust and maintainable.
Understanding Docker and Jenkins Logging
Before diving into solutions, let's clarify what logging in the context of Docker and Jenkins entails. Docker containers are ephemeral, meaning they can start and stop frequently. This poses challenges for logging because logs can easily be lost when containers are removed or restarted.
Jenkins traditionally runs as a standalone application, but when containerized, log management becomes complex without a properly defined strategy. The good news is that with the right approaches, you can overcome these challenges and streamline your logging processes.
Logging Modalities in Docker
Docker provides various logging drivers that allow you to manage how logs are handled. These drivers can be configured in your Docker daemon or within individual containers. Here are some common logging drivers:
- json-file: This is the default logging driver, which logs container output to JSON files on the host filesystem.
- syslog: Sends log entries to a syslog server for centralized logging.
- journald: Integrates with the systemd journal, allowing logs to be managed through systemd.
- fluentd: For situations requiring sophisticated logging setups with forwarding possibilities.
- gelf: Sends logs to a Graylog Extended Format (GELF) endpoint, useful for cloud logging strategies.
While the default json-file
driver may suffice for simple setups, more advanced systems require tailored approaches for logging.
Challenges Faced in Docker Logging for Jenkins
1. Log Rotation
Log rotation is crucial to prevent logs from consuming excessive disk space. Without rotation, Jenkins builds might fail due to disk space issues.
2. Aggregation Needs
In large infrastructures where multiple Docker containers run Jenkins agents, aggregating these logs into a central location can be complex.
3. Loss of Logs
With ephemeral containers, logs that are not forwarded or persisted can be lost. Developers need immediate access to logs during failure troubleshooting.
4. Format Consistency
Ensuring log format consistency across multiple microservices can be challenging, leading to difficulties in parsing and analyzing logs.
Solutions to Overcome Logging Challenges
Here are effective strategies to address logging challenges in your Jenkins CI/CD with Docker.
1. Implement Log Rotation
To enable log rotation, Docker’s json-file
can be configured. Here's how you can set this in your docker-compose.yml
:
version: '3'
services:
jenkins:
image: jenkins/jenkins:lts
logging:
driver: "json-file"
options:
max-size: "10m"
max-file: "3"
Why Log Rotation?
Setting max-size
limits how large a log file can get before it’s rotated and keeping a specific number of rotated files (max-file
) ensures that you don’t run out of disk space.
2. Use a Centralized Logging Solution
Consider using ELK Stack (Elasticsearch, Logstash, and Kibana) for centralized logging. This allows seamless aggregation, storage, and analysis of logs generated by your Jenkins CI/CD pipelines.
- Use Logstash to ingest logs from various sources.
- Store logs in Elasticsearch.
- Visualize and analyze logs using Kibana.
Here's an example snippet to configure Logstash to read Jenkins container logs:
input {
file {
path => "/var/lib/docker/containers/*/*.log"
type => "docker"
start_position => "beginning"
}
}
output {
elasticsearch {
hosts => ["http://localhost:9200"]
index => "jenkins-%{+YYYY.MM.dd}"
}
}
Why Centralized Logging? Centralized logging provides a single pane of glass for all Jenkins activities, allowing faster diagnosis of issues by correlating logs from different containers.
3. Use Logging Drivers Wisely
Depending on your architecture, consider logging drivers that fit your requirement. For instance, if you're in a microservices architecture, the gelf
logging driver can send logs to logging systems like Graylog.
Example configuration:
logging:
driver: "gelf"
options:
gelf-address: "udp://localhost:12201"
Why Choose the Right Driver? Using the appropriate logging driver ensures that you’re aligning your log management with your application's requirements, thus preventing future pitfalls.
4. Standardize Log Formats
When developing pipelines, ensure that all logs follow a standard format. Using structured logging can wrap your messages in a consistent JSON object.
Example Java code for structured logging:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class JenkinsPipeline {
private static final Logger logger = LoggerFactory.getLogger(JenkinsPipeline.class);
public void executeBuild(String jobName) {
logger.info("{\"event\": \"build_start\", \"job\": \"" + jobName + "\"}");
// Pipeline logic
logger.info("{\"event\": \"build_end\", \"job\": \"" + jobName + "\"}");
}
}
Why Standardize? Standardized log formats allow easier parsing and querying, which is beneficial when integrating with log aggregation systems.
5. Monitor Log Health
Set up alerts based on log patterns or errors to address potential issues proactively. Using tools like Prometheus and Grafana can offer insight into log metrics over time.
In Conclusion, Here is What Matters
Docker logging within Jenkins CI/CD pipelines presents distinct challenges, but with intentional strategy and effective practices, teams can overcome these hurdles. Implement log rotation, utilize centralized logging solutions like the ELK Stack, standardize log formats, and monitor log health to ensure your logging practices are robust.
For more insights on best practices for logging in Docker and Jenkins, consider checking out The Twelve-Factor App to better understand the role of logging in modern applications.
Investing time in configuring and enhancing your logging strategy now will pay dividends later, as your infrastructure scales. Happy logging!