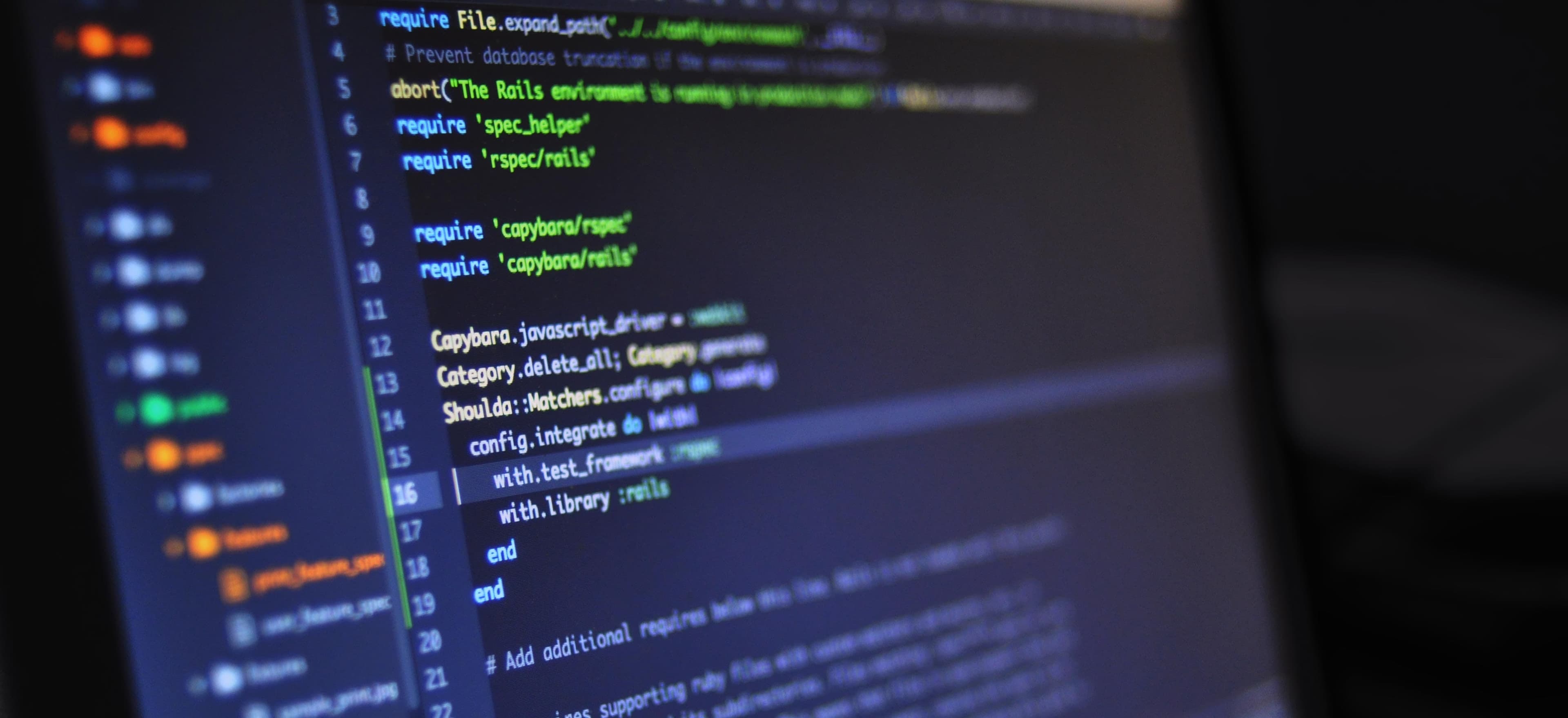
- Published on
Securing Remember Me Functionality in Apache Wicket
When building web applications, user experience is of utmost importance. One common feature that enhances user convenience is the "Remember Me" functionality. This feature allows users to remain logged into the application even after closing the browser. In Apache Wicket, implementing and securing this functionality can be challenging, but it is essential to protect your users' data.
In this blog post, we will explore how to implement and secure the "Remember Me" functionality in an Apache Wicket application. We will discuss the importance of security considerations, provide code snippets with detailed explanations, and showcase best practices.
Understanding the "Remember Me" Functionality
Before diving into the implementation, let's clarify what "Remember Me" actually does. When a user opts for this functionality, you usually store a token—often on the user's device via cookies—that allows them to bypass the login process on subsequent visits. This is convenient, but if not secured properly, it can pose security risks, such as session hijacking or unauthorized access.
Security Risks
- Cookies Vulnerability: If an attacker can access a user's cookie, they can impersonate that user.
- Token Expiry: If tokens do not expire after a defined period, they can be exploited indefinitely.
- Insufficient Encryption: Weakly encrypted tokens can be reversed or tampered with.
Therefore, implementing a secure "Remember Me" feature is crucial not only for functionality but for preserving user trust.
Steps to Implement "Remember Me" in Apache Wicket
Step 1: Add Dependencies
Make sure you have Apache Wicket dependencies in your pom.xml
file if you are using Maven:
<dependency>
<groupId>org.apache.wicket</groupId>
<artifactId>wicket-spring</artifactId>
<version>9.9.0</version> <!-- Use the latest version -->
</dependency>
Step 2: Create a Login Form
Create a login form that includes a checkbox for "Remember Me." Here's an example:
public class LoginPage extends WebPage {
public LoginPage() {
Form<Void> form = new Form<Void>("loginForm") {
@Override
protected void onSubmit() {
// Handle login logic
handleLogin(get("username").getDefaultModelObjectAsString(),
get("password").getDefaultModelObjectAsString(),
get("rememberMe").getDefaultModelObjectAsBoolean());
}
};
TextField<String> username = new TextField<>("username", Model.of(""));
TextField<String> password = new PasswordTextField("password", Model.of(""));
CheckBox rememberMe = new CheckBox("rememberMe", Model.of(false));
form.add(username);
form.add(password);
form.add(rememberMe);
add(form);
}
}
In this code snippet, we create a form with username, password fields, and a "Remember Me" checkbox.
Step 3: Handling the Login Logic
Next, implement the handleLogin
method. This method will authenticate the user and set up the "Remember Me" functionality:
private void handleLogin(String username, String password, boolean rememberMe) {
// Authenticate user with your authentication service
if (authenticate(username, password)) {
// Create a session token
String token = generateRememberMeToken(username);
if (rememberMe) {
rememberUser(token);
}
// Redirect to the main page or wherever
setResponsePage(MainPage.class);
} else {
error("Invalid username or password");
}
}
Step 4: Secure the Token
To securely generate a token, use a strong random generator and ensure that it is securely stored:
private String generateRememberMeToken(String username) {
// Generate a secure random token
SecureRandom random = new SecureRandom();
byte[] bytes = new byte[24];
random.nextBytes(bytes);
// Convert bytes to Base64 string or Hex format
String token = Base64.getUrlEncoder().withoutPadding().encodeToString(bytes);
// Store the token in database or server-side
storeTokenInDatabase(username, token);
return token;
}
Step 5: Implementing Cookie Management
You must set the cookie configuration appropriately to enhance security:
private void rememberUser(String token) {
Cookie cookie = new Cookie("rememberMe", token);
cookie.setHttpOnly(true); // Prevents JavaScript access
cookie.setMaxAge(30 * 24 * 60 * 60); // Expire in 30 days
getRequestCycle().getResponse().addCookie(cookie);
}
Step 6: Automatic Login
When a user returns to the application, check for the existence of the token and log them in automatically:
public class MyApplication extends WebApplication {
@Override
public void init() {
super.init();
// Check cookies
String rememberMeToken = getCookieValue("rememberMe");
if (rememberMeToken != null) {
String username = authenticateWithToken(rememberMeToken);
if (username != null) {
// Log the user in
signInUser(username);
}
}
}
private String authenticateWithToken(String token) {
// Look up username based on the token in the database
return findUsernameByToken(token);
}
private void signInUser(String username) {
// Logic to sign in the user
}
}
The Bottom Line and Best Practices
In this blog post, we've discussed how to implement and secure the "Remember Me" functionality within an Apache Wicket application. Remember that security isn’t a one-time task; it requires regular audits and updates.
Here are some best practices:
- Always use Secure Cookies: Use the
HttpOnly
andSecure
flags. - Expire Tokens: Implement a mechanism to regularly expires tokens and rotate them.
- Monitor Login Attempts: Implement rate limiting to prevent brute force attacks.
- Educate Users: Provide tips on creating strong passwords and recognizing phishing attempts.
For more information on securing your web applications, consider checking the Apache Wicket documentation here. Also, reviewing general web security practices can provide valuable insights into reinforcing your application's defenses. You can read more on web application security here.
By incorporating these measures, you can provide a seamless user experience while ensuring that your application remains secure. Happy coding!