How to Safeguard Against Dynamic Java Code Injection
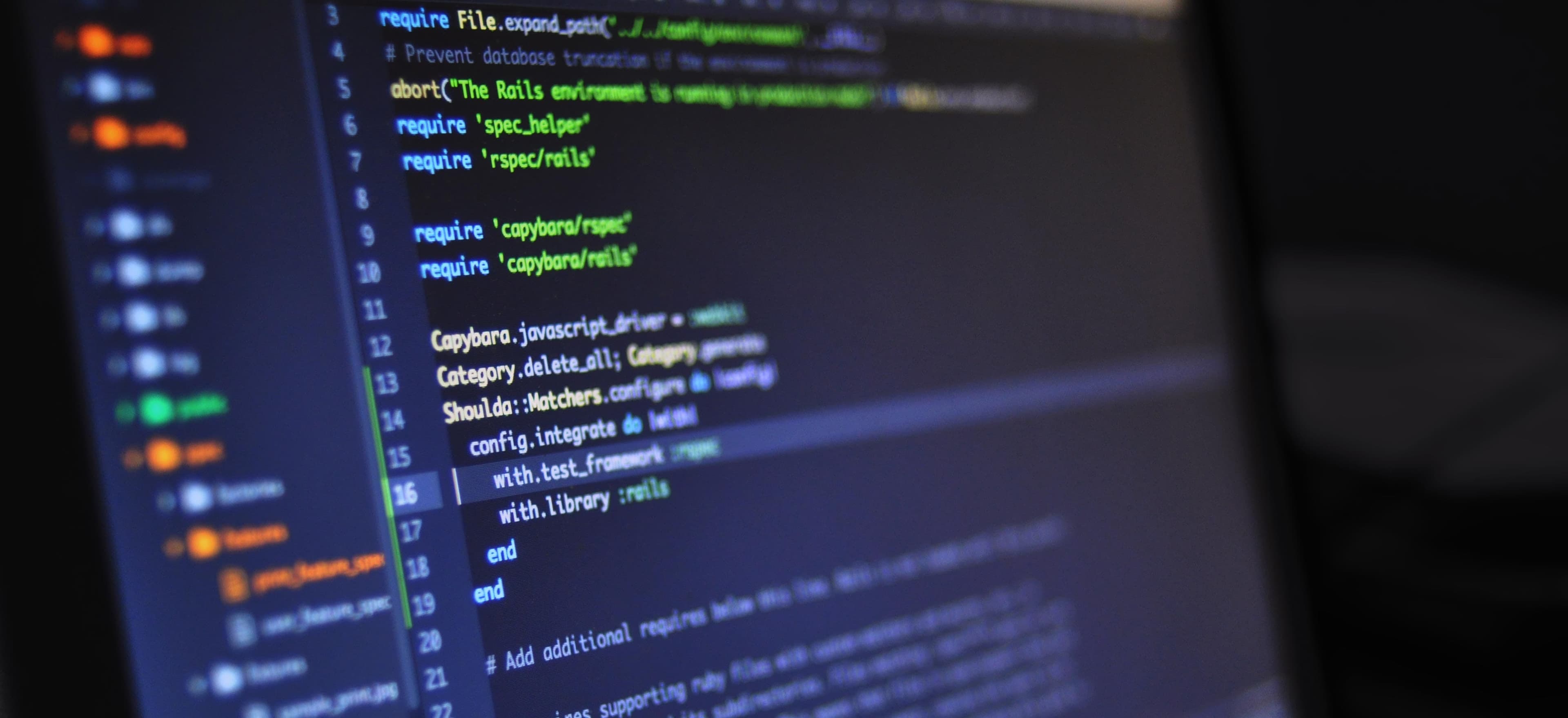
- Published on
How to Safeguard Against Dynamic Java Code Injection
Dynamic code injection is a method attackers use to exploit vulnerabilities in applications, particularly in languages like Java, where dynamic execution of code can lead to significant security breaches. As developers, it is crucial to understand how to protect your applications from such vulnerabilities. This blog post will provide a thorough examination of dynamic Java code injection and offer robust strategies to safeguard against it.
Understanding Dynamic Code Injection
Dynamic code injection occurs when an attacker is able to inject code into a program at runtime that the program interprets as valid code. In Java, this can happen through various means, such as evaluating expressions or using reflection. One common example is the use of JavaScript
or Groovy
evaluation inside Java applications.
While dynamic features can enhance application flexibility, they also introduce vulnerabilities if not handled correctly. Consider the following simplistic scenario:
public class CodeExecutor {
public void execute(String dynamicCode) {
// Dangerous if dynamicCode is user-controlled
ScriptEngine engine = new ScriptEngineManager().getEngineByName("JavaScript");
try {
engine.eval(dynamicCode);
} catch (ScriptException e) {
e.printStackTrace();
}
}
}
In the above snippet, if the dynamicCode
variable contains user-controlled input, an attacker could provide code that could compromise system integrity. For example, they might execute malicious commands like file deletion or unauthorized data access.
Common Vulnerabilities Leading to Dynamic Code Injection
-
User Input Unsanitized: If user input is processed without proper validation or sanitization, it opens pathways for injection attacks.
-
Reflection API Misuse: Using Java’s reflection capabilities without sufficient checks can allow invocation of unintended methods or access to unauthorized fields.
-
Insecure Scripting: Using an insecure scripting engine can allow attackers to inject scripts that the application will execute with the same privileges as the application itself.
Best Practices to Safeguard Against Dynamic Code Injection
1. Avoid Dynamic Code Execution
The best way to prevent dynamic code injection is to refrain from executing dynamic code in the first place. If your application can be designed without evaluating external code, you should pursue that design.
If you must use dynamic execution, try to minimize its scope and use cases. For example, replacing dynamic evaluation with a hard-coded function that processes allowed commands can reduce risks.
2. Input Validation and Sanitization
Always validate and sanitize user inputs before using them in your application. When expecting numeric or predefined string values, ensure they conform to strict formats and types.
import java.util.regex.Pattern;
public class InputValidator {
private static final Pattern NUMBER_PATTERN = Pattern.compile("^[0-9]+$");
public static boolean isValidNumber(String input) {
return NUMBER_PATTERN.matcher(input).matches();
}
}
Use the above input validation approach when dealing with user input to ensure it meets your expectations before processing. This can drastically reduce the risk of injection via invalid inputs.
3. Use Security Libraries
Security libraries can help manage dynamic behaviors while providing a sandboxed environment. Libraries like the SecurityManager help define policies that can restrict what code can be executed.
System.setSecurityManager(new SecurityManager());
By defining policies such as banning certain classes or accessing files, you can add an additional layer of protection against dynamic code injection.
4. Code Reviews and Static Analysis
Regular code reviews are vital to find areas where dynamic code execution may be used. Automated tools can also help.
Tools like FindBugs or SonarQube can flag risky code patterns and suggest safer practices.
5. Implement Role-Based Access Control
Control the permissions associated with dynamically executed code by implementing role-based access controls. By assigning specific permissions to user roles, you can restrict what functionality can be abused.
if (userRole.equals("ADMIN")) {
// Allow execution of sensitive operations
} else {
throw new SecurityException("Insufficient permissions");
}
6. Exception Handling and Logging
Finally, ensure you handle exceptions properly and log incidents of unusual behavior. If a user input is detected as potentially malicious, log it for further investigation.
try {
engine.eval(dynamicCode);
} catch (ScriptException e) {
System.err.println("Execution failed: " + e.getMessage());
logSecurityIncident(e);
}
Implementing careful logging practices provides insight into potential attack vectors and aids in rectifying vulnerabilities before they can be exploited.
A Final Look
Dynamic code injection is a critical threat to Java applications, but with conscious coding practices, robust input validation, and implementation of security measures, developers can effectively safeguard against it. Avoid executing untrusted code whenever possible. Validate and sanitize all user input, leverage security tools, and maintain strict access control measures.
Security is a continuous learning process. Stay informed, conduct regular audits, and adapt to new threats as they arise. Appropriate security strategies will greatly enhance the resilience of your Java applications against dynamic code injection attacks.
By understanding the mechanisms and applying these practices, you can fortify your Java applications against potential vulnerabilities.
For deeper insights into secure coding practices, consider reading OWASP's Secure Coding Practices resource, which encapsulates various strategies to develop secure software.
Implement these practices today to ensure a safer coding environment.
By following the presented strategies and principles, you can significantly reduce the likelihood of dynamic Java code injection affecting your applications. Stay safe, and happy coding!