Automate AndroidManifest Versioning for Smooth Releases
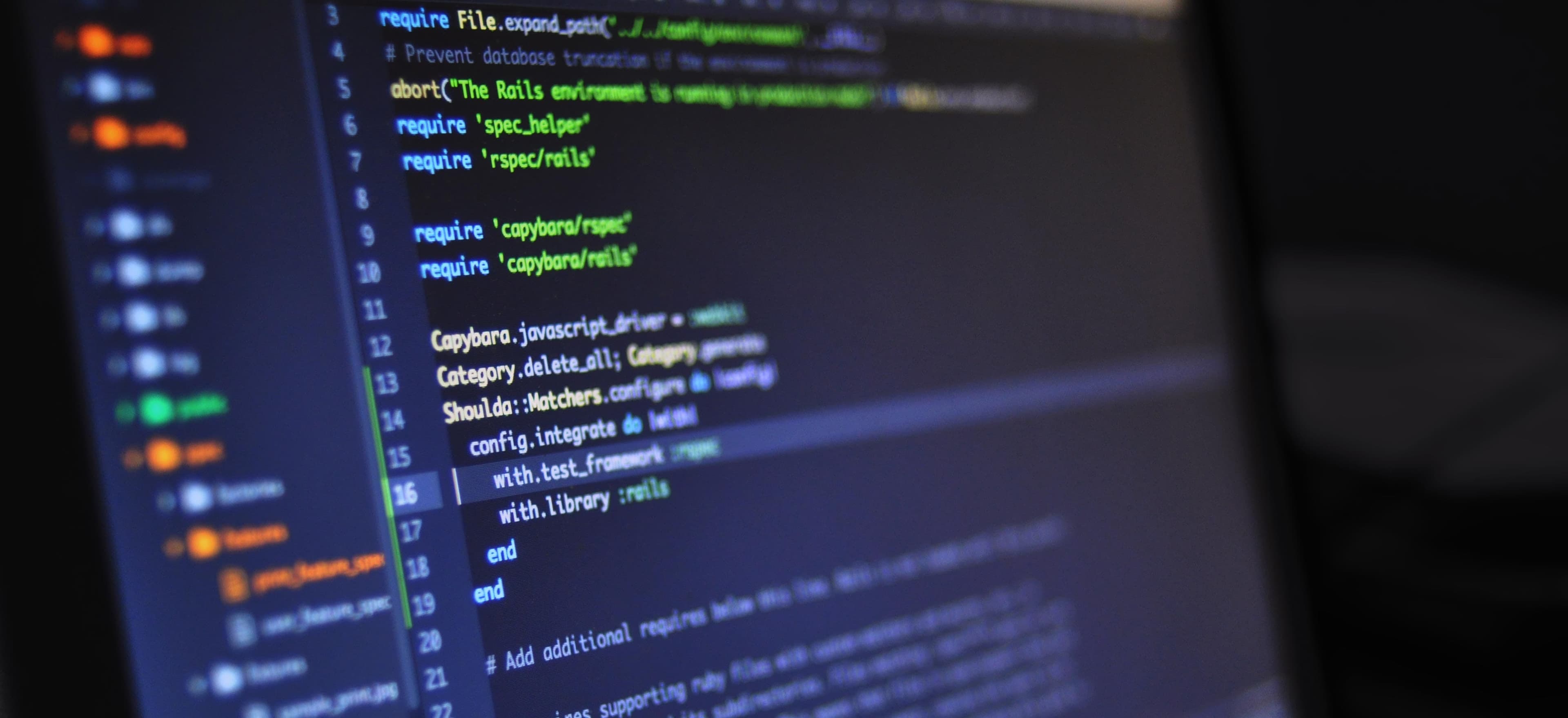
- Published on
Automate AndroidManifest Versioning for Smooth Releases
In the ever-evolving world of mobile app development, managing versioning in your AndroidManifest.xml
can become a cumbersome task, particularly when you're striving for fast and efficient releases. Every change to your app can impact its versioning, and ensuring consistency can be tricky. This blog post dives deep into automating the versioning of your AndroidManifest.xml
for Android applications, making your release process smoother.
Why Automation Matters
Before we dive into the technical aspects, let's discuss why automation is indispensable for versioning:
-
Consistency: Manual versioning can lead to human error. Automation ensures that your version numbers are consistent throughout your project.
-
Efficiency: By automating the versioning process, you save countless hours that would otherwise be spent on repetitive tasks.
-
Collaboration: In teams where multiple developers are contributing, having automated versioning avoids conflicts and miscommunications regarding the version number.
To illustrate this point, consider the scenario where a developer forgets to update the version when pushing minor changes. This can lead to confusion, bugs in production, and a poor user experience.
Setting Up Automated Versioning
Prerequisites
Ensure you have the following tools installed:
- Git: for version control.
- Gradle: to build Android applications.
- Java Development Kit (JDK): to run various Java-based tools.
Step 1: Define Your Versioning Strategy
Before automating, you need a clear versioning strategy. A common approach is Semantic Versioning (SemVer), which uses three segments:
- Major Version: Increments when you introduce incompatible API changes.
- Minor Version: Increments when you add functionality in a backwards-compatible manner.
- Patch Version: Increments when you make backwards-compatible bug fixes.
Step 2: Create a version.properties
File
Create a version.properties
file in your project root. This file will store your current version numbers. Here’s how it looks:
# version.properties
versionName=1.0.0
versionCode=1
Step 3: Update Your build.gradle
Next, you'll need to modify your app/build.gradle
file to read in the versioning information from the version.properties
file.
// app/build.gradle
def versionPropsFile = file("version.properties")
def Properties versionProperties = new Properties()
versionPropsFile.withInputStream { stream ->
versionProperties.load(stream)
}
android {
...
defaultConfig {
versionCode versionProperties['versionCode'].toInteger()
versionName versionProperties['versionName']
}
}
Commentary on the Code
In this code snippet, we:
- Load the version properties from
version.properties
. - Assign the
versionCode
andversionName
defined in the properties file to the app's default configuration.
This simple reader not only helps in keeping things organized but also ensures easy updates to the versioning system.
Step 4: Automate the Incrementing Process
To automate the process of incrementing the version code and name, let's create a custom Gradle task. This task will read the current version, increment it as required, and write the new version back to the version.properties
file.
task bumpVersion {
doLast {
def versionPropsFile = file('version.properties')
def Properties versionProperties = new Properties()
versionPropsFile.withInputStream { stream ->
versionProperties.load(stream)
}
// Incrementing logic
def currentVersion = versionProperties['versionName'].tokenize('.')
def newPatchVersion = currentVersion[2].toInteger() + 1
def newVersionName = "${currentVersion[0]}.${currentVersion[1]}.$newPatchVersion"
int currentVersionCode = versionProperties['versionCode'].toInteger()
versionProperties['versionCode'] = (currentVersionCode + 1).toString()
versionProperties['versionName'] = newVersionName
// Write changes back to version.properties
versionPropsFile.withWriter { writer ->
versionProperties.store(writer, null)
}
println "Updated to versionName: $newVersionName with versionCode: ${versionProperties['versionCode']}"
}
}
Commentary on the Incrementing Logic
- Reading Version: We read the current version from the
version.properties
file. - Incrementing: The patch version is incremented by one. You may also easily modify this logic to handle minor and major version increments based on your release type.
- Writing Back: After incrementing the values, we write them back into
version.properties
.
Step 5: Integrate Git Hooks (Optional)
To ensure that your version bump occurs automatically upon certain triggers (like before a commit), you can integrate this task into Git hooks.
Create a file named pre-commit
:
#!/bin/sh
./gradlew bumpVersion
Make this file executable:
chmod +x .git/hooks/pre-commit
With this setup, every time you make a commit, your version will increment automatically. This guarantees that you will always have a new version for each commit, thus streamlining your release process further.
Testing Your Automation
After implementing these changes, run the Gradle task to bump the version:
./gradlew bumpVersion
Check your version.properties
file to see if the version has been updated correctly. You can also build your project to see it reflected in the APK.
A Final Look
Automating your AndroidManifest.xml
versioning can dramatically streamline your development workflow, reduce human error, and keep your releases perfectly aligned with your versioning strategy. By creating a simple automation process using Gradle and Git, you empower your team to focus on building amazing features rather than tedious version management.
For further reading, check out the official Android documentation on Managing App Versions.
Start implementing this automation today, and enjoy smoother releases on your Android projects!