Is Fairness in ReentrantLock Really Worth It?
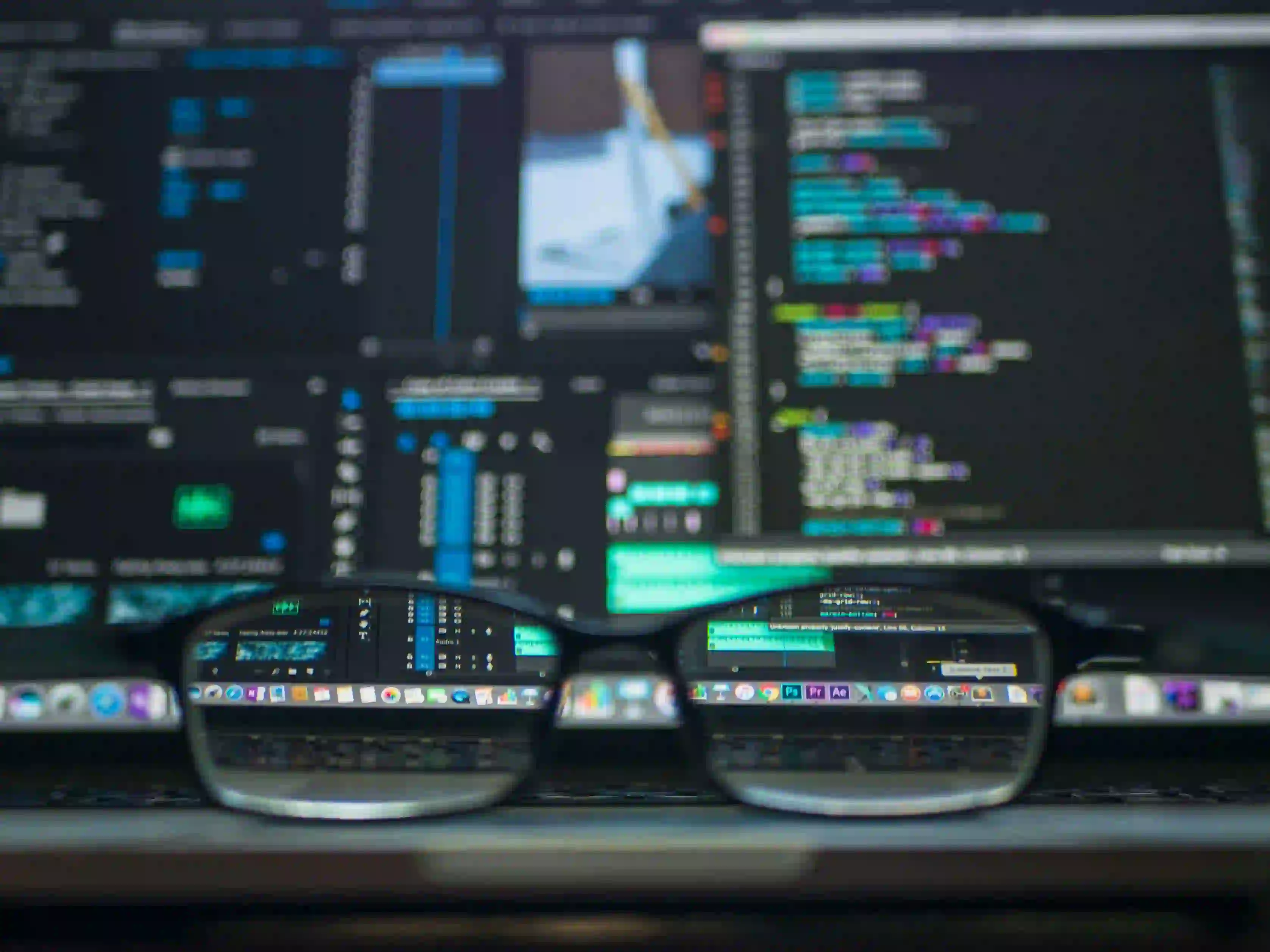
Is Fairness in ReentrantLock Really Worth It?
In the realm of Java concurrency, understanding synchronization mechanisms is crucial to building reliable and efficient applications. Among these mechanisms, ReentrantLock
is a powerful tool that provides more sophisticated capabilities than the traditional synchronized
keyword. One of the defining features of ReentrantLock
is the concept of fairness. But is implementing fairness really worth it? Let's explore this in-depth.
What is ReentrantLock
?
ReentrantLock
is a part of the java.util.concurrent.locks
package, which was introduced in Java 5. Unlike synchronized blocks, ReentrantLock
can be configured to be fair or unfair. The default implementation of ReentrantLock
is unfair, meaning that it does not guarantee the order of thread execution. The alternative is a fair lock, which provides FIFO (First In, First Out) ordering of thread access.
Code Example: Basic Usage of ReentrantLock
import java.util.concurrent.locks.ReentrantLock;
public class SimpleReentrantLock {
private final ReentrantLock lock = new ReentrantLock();
public void criticalSection() {
lock.lock();
try {
// Access shared resources
System.out.println(Thread.currentThread().getName() + " is in the critical section");
} finally {
lock.unlock();
}
}
}
In this example, the lock()
method is called to acquire the lock, and unlock()
is called to release it. The try-finally
block ensures that the lock is released, preventing deadlocks.
Fairness: What Does It Mean?
Fair Locking
In a fair ReentrantLock
, threads acquire the lock in the order they requested it. This can alleviate issues like thread starvation, where longer waiting threads may never get a chance to proceed. The following code snippet illustrates how to create a fair ReentrantLock
.
ReentrantLock fairLock = new ReentrantLock(true);
By passing true
to the constructor, we ensure that this lock will respect the order of thread requests.
Unfair Locking
On the other hand, an unfair ReentrantLock
allows threads to acquire the lock in a more flexible manner. A thread that has been waiting might lose out to a newly arrived thread. This randomness can lead to better performance in situations with high contention.
Considerations in Choosing Fairness
The choice of using a fair or unfair lock can affect the performance and responsiveness of your application. Let's discuss the scenarios where each might be beneficial.
When to Use Fair Locks
- Predictable Order: In applications where the order of execution matters, a fair lock can prevent newer threads from jumping the queue.
- Avoiding Starvation: If certain threads may be starved due to constant contention from others, a fair lock may help ensure they make progress.
When to Use Unfair Locks
- Performance: Unfair locks can provide better throughput, especially in environments with high contention, as they can reduce the overhead of managing queues.
- Low Contention: If thread contention is low, using an unfair lock may not negatively affect the system, thereby increasing performance.
Research has shown that performance can degrade significantly with fairness enabled in scenarios of high contention. It's always a sound strategy to profile your application before making decisions about locking mechanisms.
Benchmarking Performance: Fair vs. Unfair
To understand the implications of fairness, let’s look at a simple benchmark example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.locks.ReentrantLock;
public class FairnessPerformanceTest {
private final ReentrantLock fairLock = new ReentrantLock(true);
private final ReentrantLock unfairLock = new ReentrantLock(false);
private int count = 0;
public void incrementUsingFairLock() {
fairLock.lock();
try {
count++;
} finally {
fairLock.unlock();
}
}
public void incrementUsingUnfairLock() {
unfairLock.lock();
try {
count++;
} finally {
unfairLock.unlock();
}
}
public static void main(String[] args) {
FairnessPerformanceTest test = new FairnessPerformanceTest();
ExecutorService executor = Executors.newFixedThreadPool(10);
// Test fair lock
for (int i = 0; i < 1000; i++) {
executor.execute(test::incrementUsingFairLock);
}
executor.shutdown();
while (!executor.isTerminated()) { }
System.out.println("Final count using fair lock: " + test.count);
}
}
In this example, we create tasks for both fair and unfair locks, which increment a shared counter. By swapping between fair and unfair locking mechanisms, you can measure performance differences in real-world scenarios.
Potential Drawbacks of Fairness
- Overhead: The guarantees that come with fair locks introduce extra overhead that can lead to performance degradation, particularly under high contention.
- Responsiveness Issues: Although fairness may help avoid starvation, it could delay priority tasks, making the application less responsive.
Closing the Chapter: Is Fairness Worth It?
Deciding whether to use a fair ReentrantLock
hinges on your application requirements. If maintaining a FIFO order is critical and starvation of threads is a notable concern, a fair lock is the way to go. However, if you're looking for maximum performance and can tolerate the possibility of starvation, an unfair lock could be your best bet.
Ultimately, profiling and testing under realistic load conditions is the best approach. Tools like Java's JMH (Java Microbenchmark Harness) can help you get accurate performance metrics to support your decision.
For further reading, consider visiting:
As you continue navigating the complexities of Java concurrency, remember: the right synchronization strategy can make or break your application's performance and reliability. Happy coding!