Understanding Spark's Memory Management Issues and Solutions
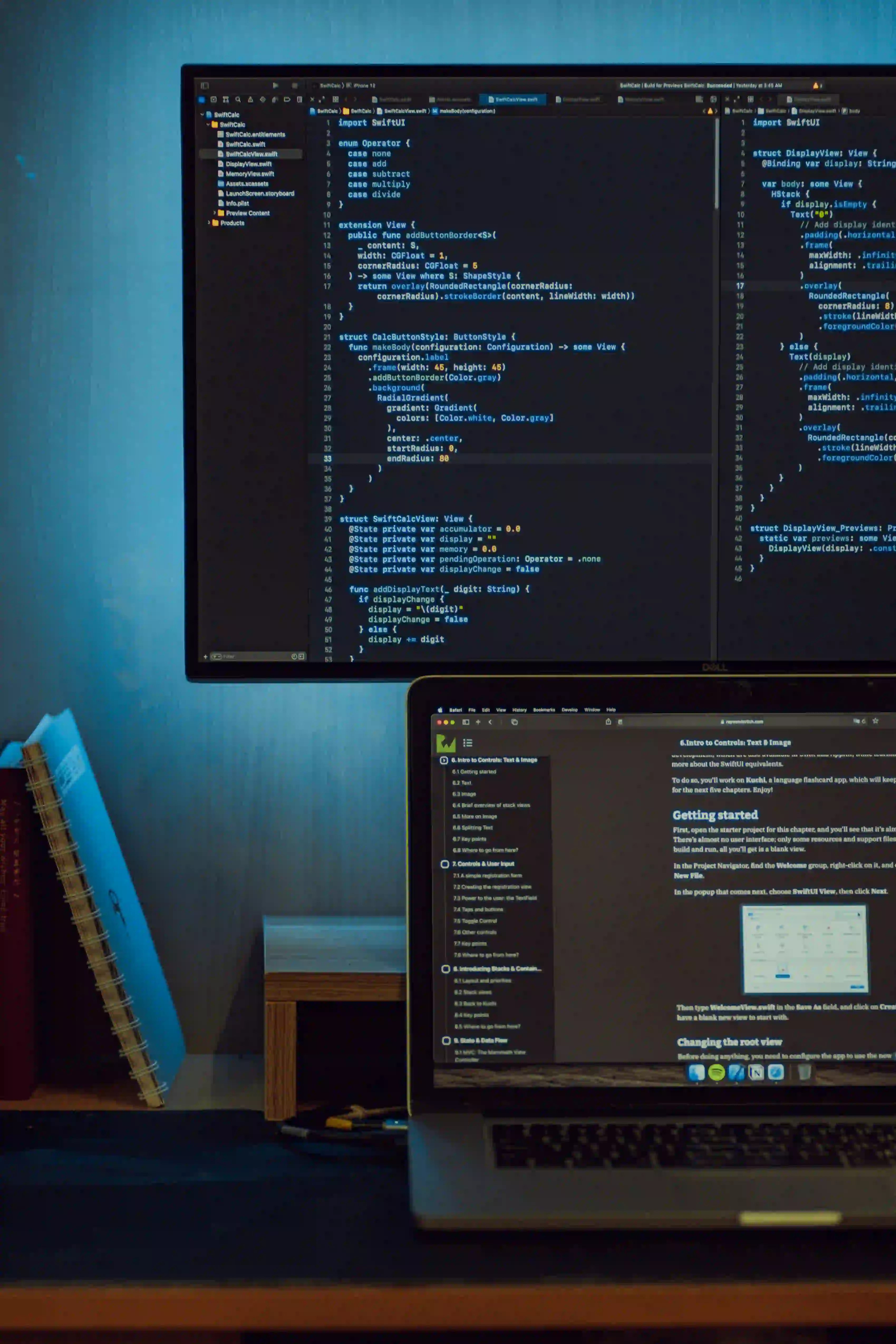
Understanding Spark's Memory Management Issues and Solutions
Apache Spark has become one of the most popular distributed computing frameworks in recent years, especially for big data processing tasks. One of the key components of Spark’s architecture is its memory management, which significantly affects performance. In this guide, we will delve into Spark’s memory management issues and solutions, ensuring you understand how to optimize memory usage for optimal performance.
Why Memory Management Matters in Spark
Memory management is crucial because it directly impacts the performance of your Spark applications. Spark uses memory for caching data, performing computations, and managing intermediate results. If memory isn’t effectively managed, it can lead to slow performance, frequent garbage collection events, and application failure.
Memory Management Overview
Spark's memory is split into two main regions:
- Execution Memory: Used for computation and processing tasks, such as shuffling, aggregation, and caching.
- Storage Memory: Used for storing data cached in memory to speed up access times in future operations.
Properly tuning these regions can lead to significant performance improvements. Understanding how to allocate and manage resources will help you build more efficient Spark applications.
Common Memory Management Issues
1. Out of Memory Errors
One of the most common issues encountered is the Out of Memory (OOM) error. This occurs when Spark tries to allocate more memory than is available in the JVM heap. OOM errors can severely disrupt your application and lead to costly downtime.
Example Scenario
Suppose your Spark job reads a large dataset and performs operations that require large intermediate results. If the resultant data exceeds the available memory, Spark will throw an OOM error.
2. Excessive Garbage Collection
Garbage collection (GC) is the process of automatically reclaiming memory occupied by objects that are no longer in use. While necessary, excessive GC activity can lead to performance degradation as threads are paused frequently to clear memory.
How to Identify GC Issues
You can monitor GC activity using logs and tools like VisualVM or the GCEasy tool. In your Spark job, look for the frequency of GC pauses and the time taken for each GC cycle.
3. Data Skew
Data skew occurs when data partitions are unevenly distributed across the cluster nodes, resulting in some tasks taking significantly longer to complete than others. This can lead to resource contention and inefficient memory usage.
Example Scenario
If a dataset has some partitions with very high cardinality and others with very low, the tasks on the high-cardinality partitions will require more memory and time, causing delays.
Solutions to Memory Management Issues
1. Tuning Memory Settings
Configuring the right memory settings is crucial for handling memory effectively. Here are some key configurations:
- spark.executor.memory: Defines the amount of memory allocated to each executor.
- spark.driver.memory: Defines the memory allocated to the driver program.
- spark.memory.fraction: This setting controls the fraction of heap space used for execution and storage memory.
Example Configuration
spark-submit --executor-memory 4G --driver-memory 2G ...
This command allocates 4 GB of memory to executors and 2 GB to the driver, which might suit a mid-sized application well.
2. Using Broadcast Variables
When dealing with large datasets, consider using broadcast variables to efficiently send large datasets to all nodes instead of shipping copies of the data with every task.
Example Code Snippet
import org.apache.spark.SparkContext;
SparkContext sc = new SparkContext("local", "Broadcast Variable Example");
int[] dataArray = {1, 2, 3, 4, 5};
final Broadcast<int[]> broadcastData = sc.broadcast(dataArray);
sc.parallelize(Arrays.asList(1, 2, 3, 4))
.map(x -> broadcastData.value()[x - 1])
.collect();
Why Use Broadcast Variables?
This code snippet demonstrates broadcasting an array so that each executor can access it without transferring the full dataset repeatedly. This reduces both memory usage and network traffic.
3. Partitioning Data Effectively
Ensure that your data is properly partitioned to prevent data skew. You can repartition your data using the repartition
or coalesce
methods.
Example Code Snippet
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.SparkSession;
SparkSession spark = SparkSession.builder()
.appName("Repartition Example")
.getOrCreate();
Dataset<Row> df = spark.read().json("path/to/data.json");
Dataset<Row> repartitionedDf = df.repartition(10); // Repartitioning into 10 partitions
Why Repartition Data?
The repartition
method allows you to redistribute data evenly across partitions. This can help avoid scenarios where certain partitions are much larger than others, leading to better memory usage.
4. Monitoring and Tuning
Using Spark’s built-in tools like the Spark UI can help you monitor memory usage, execution plans, and overall performance. Always examine the stages of your job for any bottlenecks or excessive memory usage.
Additional Resources
For further reading, the following align well with our discussion:
- Spark’s Official Memory Management Documentation
- An In-depth Look at Spark Performance Tuning
The Closing Argument
Memory management in Spark is a complex but critical aspect that determines the performance of your applications. By understanding issues like OOM errors, excessive garbage collection, and data skew, and knowing how to mitigate them through effective tuning, the use of broadcast variables, and proper data partitioning, you can significantly improve the efficiency and performance of your Spark applications.
As you continue developing with Spark, keep these strategies in mind. Regular monitoring and tuning will help ensure that your applications run smoothly and efficiently, making the most of your resources.
Call to Action
Are you ready to dive deeper into optimizing your Spark applications? Start implementing these strategies in your next project and see how they can transform your data processing tasks!
Happy coding!