Common Pitfalls in Batch Processing and How to Avoid Them
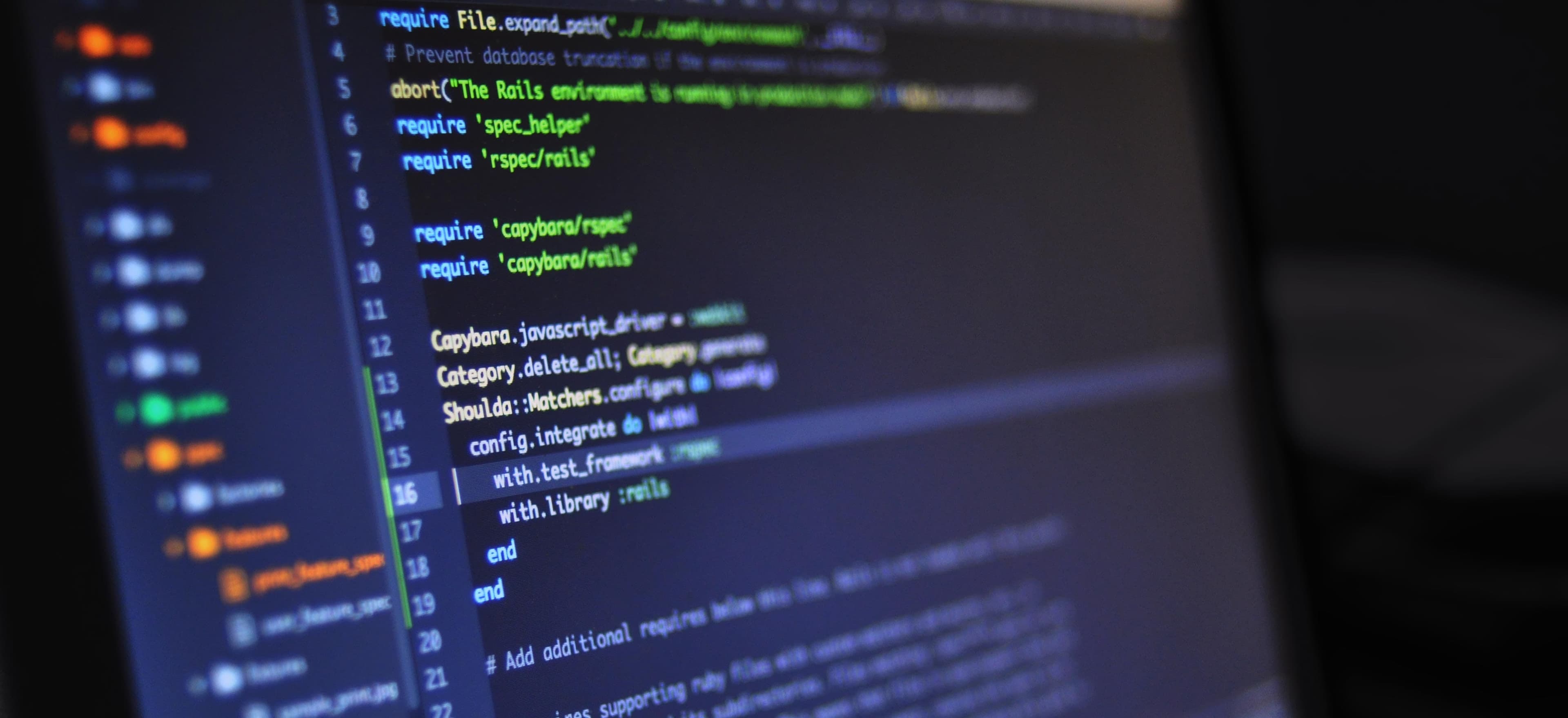
- Published on
Common Pitfalls in Batch Processing and How to Avoid Them
Batch processing is a cornerstone of modern software systems, especially in fields like data engineering, finance, and web development. The concept revolves around processing data in bulk, as opposed to real-time processing. While it can improve efficiency and reduce resource consumption, several pitfalls can arise if not handled properly. This blog post explores these common pitfalls and provides actionable strategies to avoid them.
Understanding Batch Processing
Before diving into the problems, let's clarify batch processing. It refers to the execution of a series of jobs or tasks collected and processed together at once. This paradigm is often used for:
- Data imports: Loading large datasets into databases.
- Reporting: Generating reports at regular intervals.
- Machine learning: Training models with large datasets.
While batch processing has clear advantages, developers must navigate some common pitfalls to harness its full potential.
Common Pitfalls in Batch Processing
1. Lack of Error Handling
One of the most detrimental errors in batch processing is failing to include adequate error handling. When processing numerous records, it is inevitable that some might fail—be it due to data corruption, network issues, or incorrect formatting.
Solutions:
- Implement robust error logging: Ensure your batch processing scripts log detailed error messages. This is critical for troubleshooting.
- Use transactions: If a batch process fails, you want the ability to roll back to a consistent state rather than leaving partial data processed.
Connection conn = null;
try {
conn = DriverManager.getConnection(DB_URL, USER, PASS);
conn.setAutoCommit(false); // Set auto-commit to false
// Your batch processing logic
conn.commit(); // Commit all changes if successful
} catch (SQLException e) {
if (conn != null) {
try {
conn.rollback(); // Roll back if there's an error
} catch (SQLException ex) {
ex.printStackTrace();
}
}
e.printStackTrace();
} finally {
if (conn != null) {
try {
conn.close(); // Always close connection
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Why? This code demonstrates how to manage transactions effectively. By wrapping operations in a transaction, you ensure that your system maintains a stable state, even when issues arise.
2. Ignoring Performance Metrics
Performance tuning is often an overlooked aspect of batch processing. If you ignore the metrics, your batch jobs can degrade over time, leading to slow processing and increased costs.
Solutions:
- Monitor execution time: Keep track of how long each batch job takes and look for trends.
- Profile resource usage: Measure CPU, memory, and I/O during execution to identify bottlenecks.
long startTime = System.currentTimeMillis();
// Your batch processing logic
long endTime = System.currentTimeMillis();
System.out.println("Batch processing took: " + (endTime - startTime) + " milliseconds");
Why? By logging execution times, you gain insights into performance and identify areas to optimize, such as parallel processing or query tuning.
3. Poorly Defined Job Dependencies
In scenarios where multiple batch jobs depend on each other, unclear dependency management can lead to jobs failing or executing prematurely. This confusion might result in inconsistent datasets or even data corruption.
Solutions:
- Use orchestration tools: Tools like Apache Airflow or Spring Batch allow you to define and manage job dependencies clearly.
- Document jobs thoroughly: Maintain clear documentation regarding dependencies to facilitate troubleshooting.
4. Not Providing Enough Resources
Consider this: your batch job is ready to process a large volume of data, but it remains painfully slow. Often, under-allocating resources is the cause.
Solutions:
- Scale your infrastructure: Use cloud resources that can adjust dynamically based on the workload.
- Run jobs during off-peak hours: Schedule jobs when system resources are more readily available to optimize performance.
5. Inefficient Data Loading
In many cases, the data loading strategy is inefficient. For instance, if you're loading data one record at a time instead of in bulk, you may face severe performance penalties.
Solutions:
- Use bulk inserts: Leverage database-specific features for bulk data loading. Most databases provide mechanisms to efficiently load large datasets.
PreparedStatement pstmt = conn.prepareStatement("INSERT INTO MyTable (column1, column2) VALUES (?, ?)");
for (Data record : records) {
pstmt.setString(1, record.getField1());
pstmt.setString(2, record.getField2());
pstmt.addBatch(); // Add to batch
}
pstmt.executeBatch(); // Execute all at once
Why? This bulk insert not only reduces the number of database trips but also capitalizes on the database's efficiency to handle large operations.
6. Not Designing for Scalability
Designing your batch jobs for scalability is essential. As your datasets grow, a design that works well initially might buckle under the load later.
Solutions:
- Partition your data: Break down large datasets into manageable chunks. This allows you to process multiple segments concurrently.
- Use asynchronous processing: Consider an event-driven architecture that can scale horizontally based on demand.
7. Failing to Test Rigorously
Many batch jobs are pushed to production without adequate testing. This oversight can lead to severe failures resulting in lost data or corrupted outputs.
Solutions:
- Implement unit tests: Create unit tests for your batch jobs to validate functionality.
- Conduct load testing: Simulate high loads to ensure the batch job performs well under stress.
@Test
public void testBatchProcessing() {
MyBatchProcessor processor = new MyBatchProcessor();
// Mock inputs and expected outputs
List<Data> inputData = generateTestData();
processor.runBatch(inputData);
assertEquals(expectedOutput, processor.getProcessedData());
}
Why? Unit tests ensure that your batch processing logic works as intended and helps identify issues before they reach production.
Closing the Chapter
Batch processing holds incredible potential for efficiency and resource management; however, understanding and navigating its pitfalls is crucial. By focusing on robust error handling, performance metrics monitoring, resource allocation, and thorough testing, developers can unlock the true value of batch processing.
If you want to dive deeper into advanced batch processing techniques, check out Apache Batch and learn about its capabilities. For more information on job orchestration, consider exploring Apache Airflow, which allows managing complex workflows easily.
Taking the time to address these common pitfalls will enrich your development experience and lead to more resilient, scalable applications. Happy coding!