Struggling with MVP? Common Pitfalls and Solutions Explained
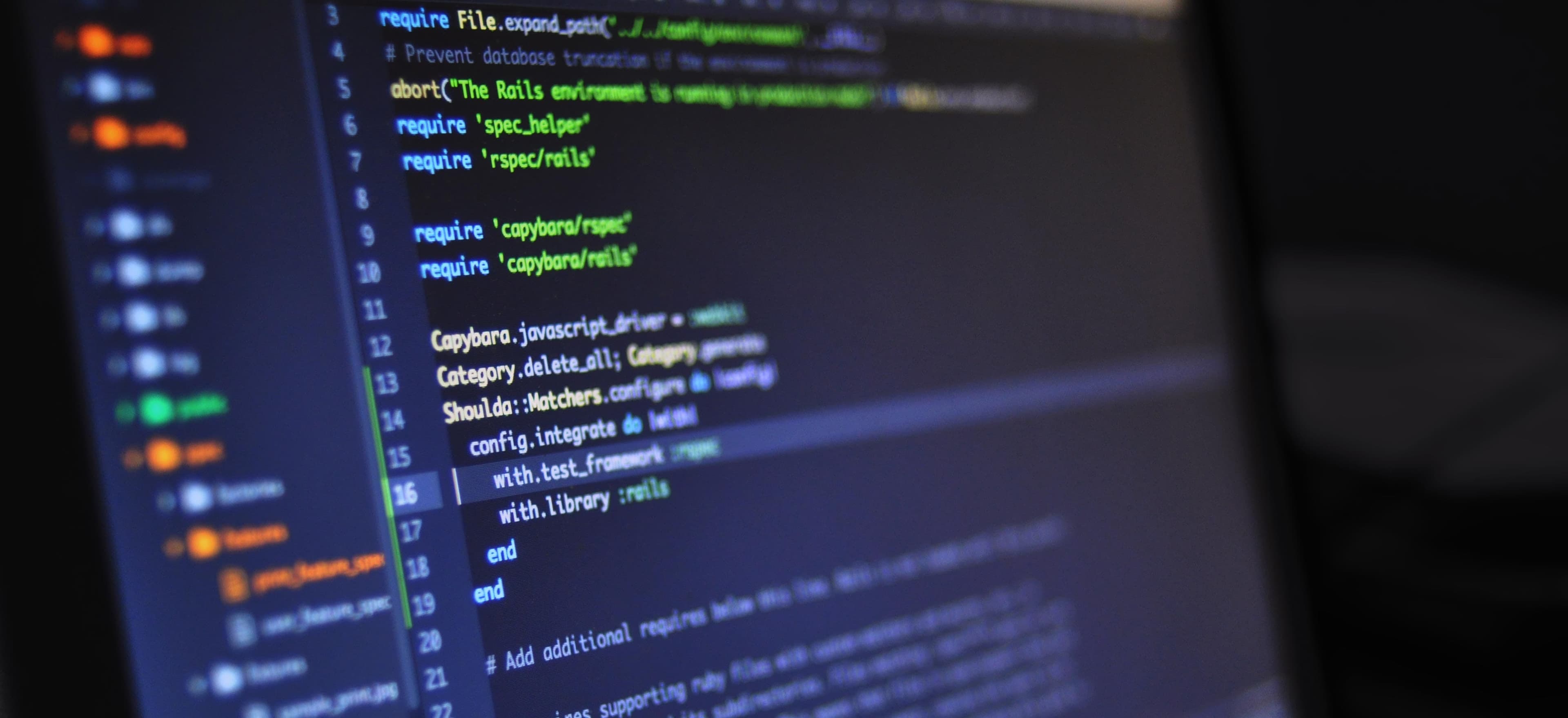
- Published on
Struggling with MVP? Common Pitfalls and Solutions Explained
Creating a Minimum Viable Product (MVP) is a pivotal step in the entrepreneurial journey. An MVP allows you to validate your business idea with minimal resources and gather user feedback to fine-tune your product. However, this process isn't without its challenges. Let's explore some common pitfalls you might encounter while developing your MVP and provide actionable solutions to help you navigate these hurdles.
What Is an MVP?
Before diving into the problems, it's essential to clarify what an MVP is. An MVP is a product with just enough features to satisfy early adopters and provide feedback for future development. The goal is to quickly get your concept into the hands of users to learn what works and what doesn't.
Common Pitfalls in MVP Development
1. Lack of Clear Goals
Pitfall: Many startups begin developing their MVP without clearly established goals. This lack of direction can lead to wasted effort and resources.
Solution: Define clear, measurable goals before you start. Consider questions like:
- What problem am I trying to solve?
- Who are my target users?
- What core features must my MVP include to provide value?
Setting specific goals helps you keep your focus and determine the right features to include in your MVP.
2. Overcomplicating the Product
Pitfall: It's easy to get carried away and add too many features to your MVP. This can dilute the core idea, making your product more complex than it needs to be.
Solution: Prioritize features based on user needs. Start with the absolute essentials that solve the primary problem you identified. Use techniques such as the MoSCoW method (Must have, Should have, Could have, Won't have) to prioritize effectively.
Code Snippet for Feature Prioritization (Java)
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
public class FeaturePrioritizer {
public static void main(String[] args) {
HashMap<String, String> features = new HashMap<>();
features.put("Feature A", "Must have");
features.put("Feature B", "Should have");
features.put("Feature C", "Could have");
List<String> priorityList = prioritizeFeatures(features);
System.out.println("Prioritized Features: " + priorityList);
}
public static List<String> prioritizeFeatures(HashMap<String, String> features) {
List<String> orderedFeatures = new ArrayList<>();
// Iterate through the map to order features based on priority.
features.entrySet().stream()
.filter(entry -> entry.getValue().equals("Must have"))
.forEach(entry -> orderedFeatures.add(entry.getKey()));
features.entrySet().stream()
.filter(entry -> entry.getValue().equals("Should have"))
.forEach(entry -> orderedFeatures.add(entry.getKey()));
features.entrySet().stream()
.filter(entry -> entry.getValue().equals("Could have"))
.forEach(entry -> orderedFeatures.add(entry.getKey()));
return orderedFeatures;
}
}
In this code snippet, we define a method to prioritize features based on their necessity. This approach helps teams focus on what truly matters.
3. Neglecting User Feedback
Pitfall: Some entrepreneurs ignore user feedback after the launch, believing they know what users want. This can lead to a product that misses the mark.
Solution: Actively solicit and analyze user feedback. Use surveys, interviews, and analytics to gather insights about user behavior and preferences.
4. Ignoring Market Research
Pitfall: Jumping into development without understanding the market landscape can lead to an MVP that’s out of sync with user needs or competitive offerings.
Solution: Conduct thorough market research. Identify who your competitors are and what they offer. Analyze their weaknesses and strengths. Use resources like Statista and Crunchbase for insightful data.
5. Underestimating Development Time and Costs
Pitfall: Entrepreneurs often underestimate the time and financial resources needed to develop their MVP. This can lead to rushed projects and subpar products.
Solution: Create a detailed project timeline and budget. Break the development process into phases and set realistic deadlines.
Code Snippet for Time Estimation (Java)
import java.util.HashMap;
public class DevelopmentTimeline {
public static void main(String[] args) {
HashMap<String, Integer> tasks = new HashMap<>();
tasks.put("Design UI", 10); // Estimated days
tasks.put("Develop Backend", 15);
tasks.put("Testing", 5);
int totalEstimatedTime = estimateTotalTime(tasks);
System.out.println("Total Estimated Development Time: " + totalEstimatedTime + " days");
}
public static int estimateTotalTime(HashMap<String, Integer> tasks) {
return tasks.values().stream().mapToInt(Integer::intValue).sum();
}
}
Here, we created a simple method to estimate the total development time based on individual tasks. This helps keep the project on track.
6. Failing to Measure Success
Pitfall: Many MVPs are released with little thought to how success will be measured. Without metrics, it’s challenging to know if you achieved your goals.
Solution: Define success metrics before your MVP launch. Key Performance Indicators (KPIs) might include user engagement rates, conversion rates, or customer satisfaction levels.
7. Not Iterating Based on Data
Pitfall: After launch, some companies don’t iterate on their MVP based on real user data, which can stymie growth.
Solution: Establish an iterative development process. Use Agile methodologies to adapt and evolve your product based on ongoing user feedback and performance data. Tools like Trello or Jira can help manage these changes.
8. Neglecting Marketing and User Acquisition
Pitfall: Even the best MVP will fail if no one knows about it. Startups often overlook marketing, thinking that a great product will sell itself.
Solution: Develop a robust marketing strategy that includes social media promotion, email marketing, and possibly paid advertising. Consider platforms like Buffer or Hootsuite for effective social media management.
In Conclusion, Here is What Matters
Creating a Minimum Viable Product is a significant milestone for any startup, but it comes with its own set of challenges. By acknowledging and addressing these common pitfalls from the start, you stand a better chance of building a successful MVP that meets user needs and sets the tone for future growth.
Remember, the primary goal of an MVP is to validate your concept quickly while maintaining focus on the essential features. Emphasize user feedback and iterative improvement, and you'll be well on your way to creating a product that resonates with your target audience.
Navigating the world of MVP can be challenging, but with dedication and the right strategies, you can avoid pitfalls and set up a solid foundation for your startup's success. Always keep learning, adapting, and innovating.
By strategizing effectively, maintaining a clear focus, and staying committed to your users, you can transform your MVP into a fully-fledged product that meets market demands.
Checkout our other articles