Overcoming Common Pitfalls in Strategy Design Patterns
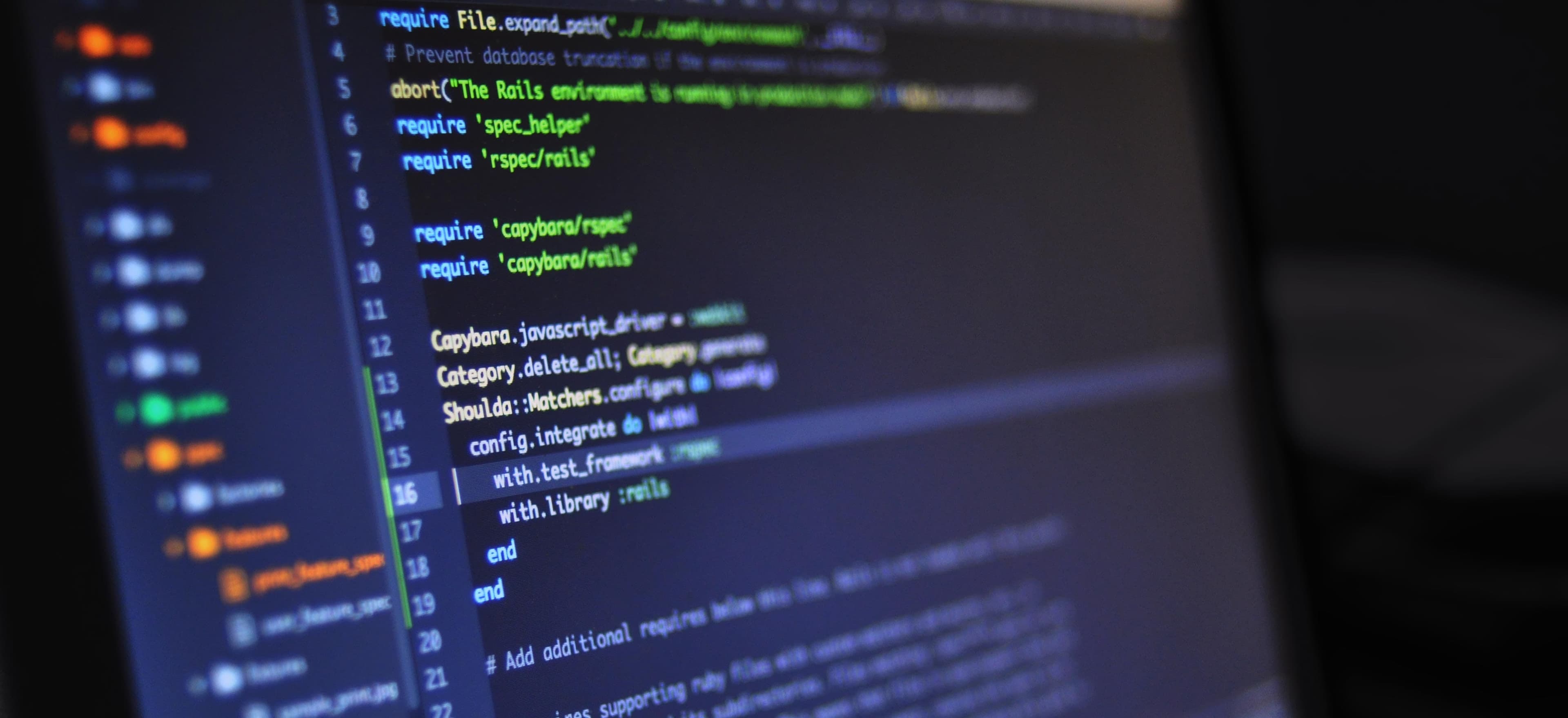
- Published on
Overcoming Common Pitfalls in Strategy Design Patterns
In the ever-evolving world of software design, the Strategy Design Pattern stands as an invaluable tool. It allows developers to define a family of algorithms, encapsulate each one, and make them interchangeable. The Strategy Pattern is particularly useful for applications that require dynamic behavior based on different conditions or user inputs. However, despite its benefits, developers often stumble over common pitfalls when implementing this pattern.
In this article, we will explore these pitfalls, provide solutions, and illustrate our points with Java code snippets. This way, you can not only understand the concept but also effectively apply the Strategy Pattern in your projects.
Understanding the Strategy Design Pattern
Before diving into the pitfalls, let’s ground ourselves in the fundamentals of the Strategy Pattern. It consists of three primary components:
- Context: Maintains a reference to a strategy object.
- Strategy Interface: Declares a common interface for all supported algorithms.
- Concrete Strategies: Implements the strategy interface.
Code Overview
Here’s a simplified example to illustrate:
// Strategy Interface
public interface PaymentStrategy {
void pay(int amount);
}
// Concrete Strategy A
public class CreditCardPayment implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using Credit Card.");
}
}
// Concrete Strategy B
public class PayPalPayment implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using PayPal.");
}
}
// Context
public class ShoppingCart {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void checkout(int amount) {
if (paymentStrategy == null) {
throw new IllegalStateException("Payment strategy not set.");
}
paymentStrategy.pay(amount);
}
}
In the above code, users can easily switch between different payment options without altering the ShoppingCart
class. However, let's explore common pitfalls that could undermine this flexible structure.
Common Pitfalls in Strategy Design Patterns
1. Overcomplicating Strategy Implementations
While it might seem tempting to create multiple strategies for every possible use case, this can lead to unnecessary complexity. For instance, if your application only requires two methods of payment, it’s overkill to create separate strategy classes for every combination of those payments.
Solution
Limit the use of the Strategy Pattern to scenarios where behavior genuinely varies. Use inheritance and polymorphism judiciously.
2. Poor Naming Conventions
When it comes to strategy classes, descriptive naming is crucial. Without clear names, it can become challenging to understand what each class does.
Solution
Adopt meaningful names that clearly articulate their purpose. For example, instead of using generic names like PaymentMethodA
, opt for something specific, such as CreditCardPayment
.
3. Rigidity in Changing Strategies
Another common issue arises when the context tightly couples to specific strategy implementations. This weakens the design and makes changing or extending strategies cumbersome.
Solution
Leverage Dependency Injection to inject the appropriate strategy into the context. This approach enables easy swapping of strategies without altering the context.
Code Example for Dependency Injection
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class PaymentService {
private PaymentStrategy paymentStrategy;
// Constructor with dependency injection
@Autowired
public PaymentService(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void processPayment(int amount) {
paymentStrategy.pay(amount);
}
}
In this example, the PaymentService
can receive any implementation of PaymentStrategy
, making it more flexible and testable.
4. Not Taking Advantage of Composition
Often, developers implement multiple strategies with inheritance when composition would suffice. This can lead to an unnecessary hierarchy of classes.
Solution
Consider using composition over inheritance whenever possible. For example, if a new payment method shares functionality with existing methods, encapsulate that functionality into a common class and let the strategies delegate to it.
Code Example for Composition
public abstract class PaymentMethod {
protected String transactionId;
public void generateTransactionId() {
this.transactionId = "txn_" + System.currentTimeMillis();
}
}
public class CreditCardPayment extends PaymentMethod implements PaymentStrategy {
@Override
public void pay(int amount) {
generateTransactionId();
System.out.println("Paid " + amount + " using Credit Card. Transaction ID: " + transactionId);
}
}
public class PayPalPayment extends PaymentMethod implements PaymentStrategy {
@Override
public void pay(int amount) {
generateTransactionId();
System.out.println("Paid " + amount + " using PayPal. Transaction ID: " + transactionId);
}
}
By leveraging composition, we maintain a clear design while promoting code reuse.
5. Neglecting Strategy Selection Logic
Sometimes, the logic for selecting which strategy to use can become tangled and complex, dramatically reducing code maintainability.
Solution
Centralize the strategy selection logic either in a separate class or method. This ensures clarity and reduces clutter in the context or application logic.
Code Example for Strategy Selection
public class PaymentProcessor {
public PaymentStrategy selectPaymentStrategy(String method) {
switch (method.toLowerCase()) {
case "creditcard":
return new CreditCardPayment();
case "paypal":
return new PayPalPayment();
default:
throw new IllegalArgumentException("No such payment method.");
}
}
}
This segregation ensures that the application can easily extend support for new payment methods without altering existing code.
Wrapping Up
The Strategy Design Pattern can significantly enhance code flexibility and maintainability. However, it is imperative to avoid common pitfalls that may lead to unwieldy implementations. Remember to keep your strategies simple, meaningful, and loosely coupled.
By adhering to best practices, such as proper naming conventions and utilizing composition over inheritance, we can unlock the full potential of the Strategy Pattern in our Java applications.
For further reading on design patterns, consider visiting Refactoring Guru and Baeldung. These resources provide deeper insights and example-driven explanations that will strengthen your understanding of this crucial design approach.
By effectively leveraging the Strategy Design Pattern, you not only improve your code but also make it more adaptable to future changes. Happy coding!
Checkout our other articles