Troubleshooting Metro Table Issues in JavaFX Applications
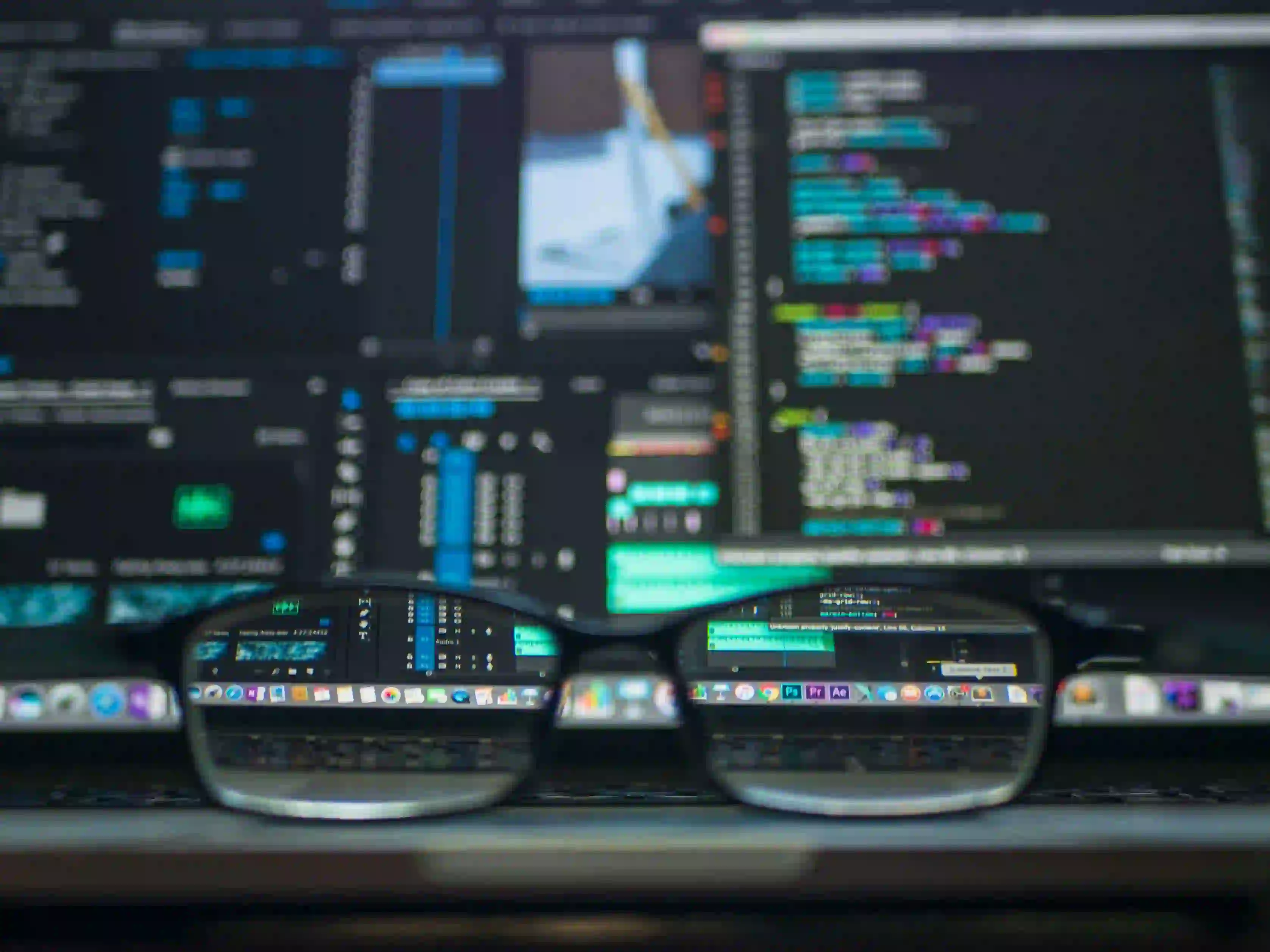
Troubleshooting Metro Table Issues in JavaFX Applications
JavaFX is a powerful library to create rich client applications in Java. However, with its flexibility come challenges, particularly when working with complex UI components like Metro Tables. These tables, which offer a modern aesthetic, can exhibit issues that might confuse developers, from rendering problems to data binding glitches. In this blog post, we will explore common issues with Metro Tables in JavaFX applications and provide effective troubleshooting techniques.
What is a Metro Table?
The Metro Table in JavaFX is a stylized version of a TableView. It presents data in a clear and visually appealing manner, often used in applications that prioritize user experience. The Metro design themes emphasize:
- Clean lines: Offering a modern, flat interface.
- Color contrast: Improving readability and focus.
- Responsive design: Adjusting to various screen sizes.
Why Choose Metro Tables?
The Metro design can enhance the usability of your application by making it easier for users to find and interact with information. Its appealing look can significantly improve user engagement, especially in data-heavy applications.
Common Issues with Metro Tables
Despite the great advantages of using Metro Tables, developers may encounter various challenges. Let's delve into the most common issues and their potential solutions.
1. Data Not Rendering Properly
One of the more frustrating problems is when the table does not render data properly. The symptoms could range from no data appearing in the table to partial rendering of rows.
Troubleshooting Steps:
- Check Data Binding: The primary reason for this issue is typically faulty data binding. Ensure that your items are correctly bound to the TableView.
ObservableList<Person> data = FXCollections.observableArrayList();
data.add(new Person("John", "Doe"));
data.add(new Person("Jane", "Smith"));
tableView.setItems(data);
This code ensures that the data you want to display is linked properly to the TableView
. Always confirm that the data type in your observable list matches the data type expected by the TableColumn
.
- Validate the Cell Factories: If you're using custom cell factories, ensure that they’re implemented correctly. A common mistake is returning null or not rendering the data as expected.
TableColumn<Person, String> firstNameColumn = new TableColumn<>("First Name");
firstNameColumn.setCellValueFactory(new PropertyValueFactory<>("firstName"));
Here, the PropertyValueFactory ties the column to the correct property in the Person class. Make sure that the property names match.
2. Performance Issues with Large Data Sets
When dealing with large data sets, performance can degrade, leading to sluggish table interactions.
Troubleshooting Steps:
- Lazy Loading: Implement lazy loading of data. Instead of loading all the data at once, consider using pagination to load smaller chunks.
// Load data for the current page
List<Person> currentPageData = personService.getPeopleForPage(currentPage, pageSize);
tableView.setItems(FXCollections.observableArrayList(currentPageData));
By limiting the visible data, you decrease memory usage and improve responsiveness.
- Optimize Data Structures: Utilize more efficient data structures in your application. For instance, if you are frequently sorting or filtering data, consider using a
List
instead of a set or array.
3. User Interaction Issues
Metro Tables may not respond properly to user input, such as sorting or filtering. These issues can stem from a number of factors.
Troubleshooting Steps:
- Event Handlers: Ensure that event handlers are correctly attached to the Metro Table. Use the appropriate listeners that react to user interactions.
firstNameColumn.setOnEditCommit(event -> {
Person person = event.getRowValue();
person.setFirstName(event.getNewValue());
});
This snippet ensures that when a user edits a cell, the data model is also updated, maintaining data integrity.
- Table Filter: If you use a filtering feature, confirm it functions as expected. Here’s a simple filtering mechanism:
FilteredList<Person> filteredData = new FilteredList<>(data, b -> true);
searchField.textProperty().addListener((observable, oldValue, newValue) -> {
filteredData.setPredicate(person -> {
if (newValue == null || newValue.isEmpty()) {
return true; // No filter applied
}
String lowerCaseFilter = newValue.toLowerCase();
return person.getFirstName().toLowerCase().contains(lowerCaseFilter);
});
});
tableView.setItems(filteredData);
In this code, we establish a binding on the search field input to filter the displayed data. Proper predicates can enhance user experience significantly.
4. Styling and Appearance Issues
Styling your Metro Table might not always produce the desired outcomes, resulting in visual issues.
Troubleshooting Steps:
- CSS Styling: If the table styles do not apply as expected, ensure your CSS files are correctly linked, and the styles are specified accurately.
.table-view {
-fx-background-color: #f0f0f0;
-fx-border-color: #d0d0d0;
}
Link your CSS file in the JavaFX application using:
scene.getStylesheets().add(getClass().getResource("style.css").toExternalForm());
- Node Hierarchy: Pay attention to the node hierarchy of your Metro Table. Sometimes, issues arise from incorrectly nesting elements or the order of style application.
Useful Resources
For developers seeking to deepen their understanding of JavaFX and Metro Tables, the following resources provide excellent guidance:
- JavaFX Documentation: The official documentation is an invaluable resource for comprehensive information about the JavaFX library.
- CSS Styling in JavaFX: Learn how to effectively use CSS with JavaFX to customize your controls.
To Wrap Things Up
Working with Metro Tables in JavaFX can elevate your application's user experience. However, lagging performance, rendering issues, and styling complications can create obstacles during development. By following the troubleshooting steps outlined in this blog post and using the provided code snippets, you can effectively navigate and resolve these challenges.
JavaFX continues to be an excellent choice for building modern applications, and by mastering Metro Tables, you’ll be well-equipped to deliver beautiful, functional UIs. Don't hesitate to explore more. Happy coding!
Feel free to leave your thoughts or questions in the comments below!