Optimizing Performance: Async Executor in Activiti Systems
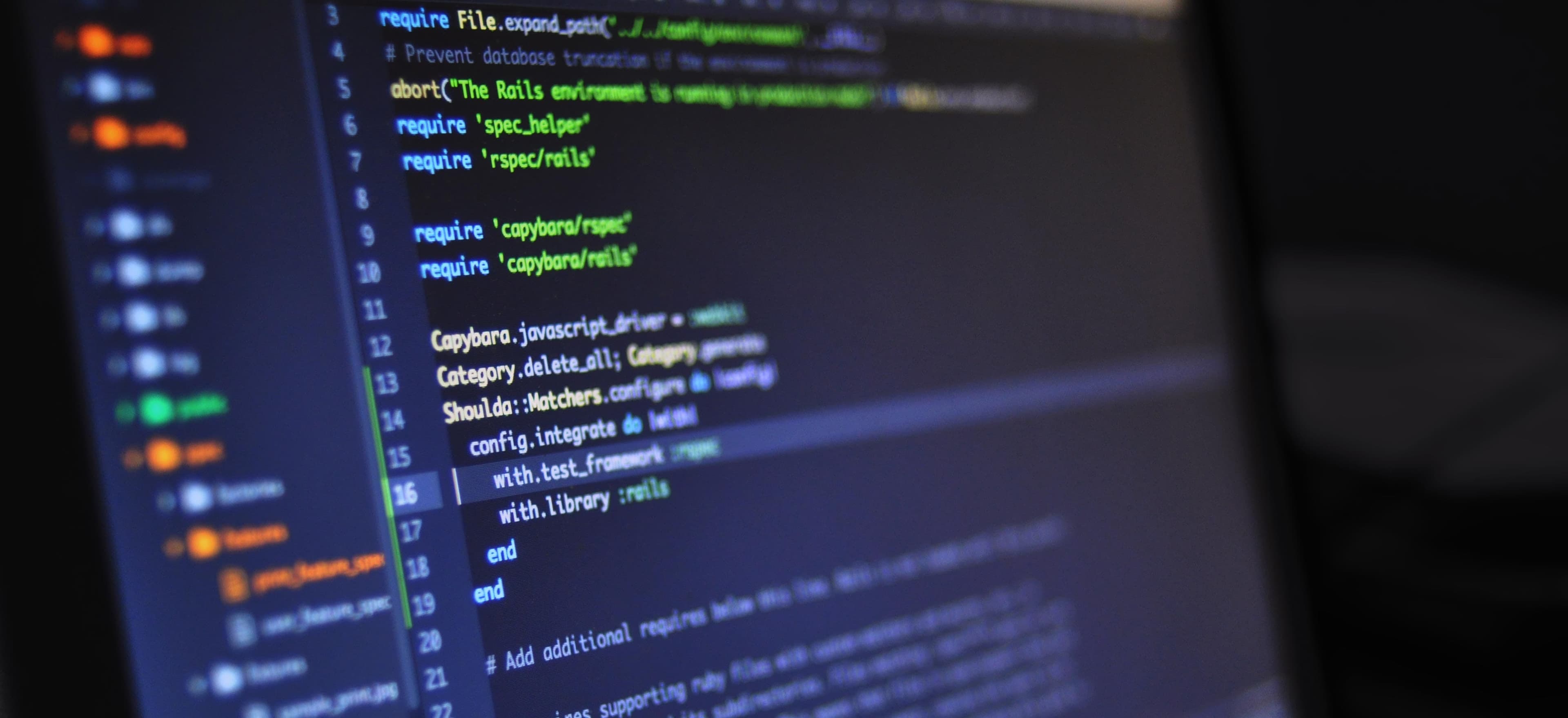
- Published on
Optimizing Performance: Async Executor in Activiti Systems
In the realm of workflow and process management, Activiti stands out as a powerful BPM (Business Process Management) engine. A critical component of managing process executions efficiently is the concept of an Async Executor. This blog post aims to explore how Async Executor can enhance performance in Activiti systems while providing practical insights and code snippets.
Understanding Activiti and Async Executor
Activiti is a lightweight workflow and business process management (BPM) platform that is used by many organizations to automate and improve business processes. With its rich features and flexibility, one of the core functionalities that it provides is the ability to execute tasks asynchronously.
The Async Executor in Activiti handles command execution in the background. By offloading heavy tasks from the main thread, it enhances the responsiveness and scalability of applications. Let's dive into its mechanisms, benefits, and implementation strategies.
Why Use Async Executor?
Before we move into the specifics of implementation, let’s discuss the notable benefits of using Async Executor:
-
Improved Responsiveness: By executing commands in the background, the main application thread remains available to handle incoming requests, significantly improving user experience.
-
Scalability: Asynchronous processing allows for better scalability, especially under load. Multiple instances of a task can be processed without blocking the main application flow.
-
Error Handling: Async commands can have retry mechanisms, allowing you to manage failures more gracefully compared to synchronous operations.
-
Resource Management: It enables better resource allocation by processing tasks when resources are available.
Configuring the Async Executor
To get started with Async Executor in your Activiti setup, you first need to ensure that it is enabled in your configuration file. Below is an example of how to configure it:
<bean id="processEngineConfiguration" class="org.activiti.spring.SpringProcessEngineConfiguration">
<!-- Other configurations -->
<property name="asyncExecutorEnabled" value="true" />
<property name="asyncExecutorActivate" value="true" />
</bean>
Explanation:
- asyncExecutorEnabled: Activating the Async Executor.
- asyncExecutorActivate: This property sets the executor to start executing tasks based on the business logic defined in your process.
Implementing Async Executor
To use the Async Executor effectively, there are several components involved, including an AsyncExecutor
bean and a configuration that defines certain parameters.
Here is a sample implementation of the Async Executor:
import org.activiti.engine.impl.cfg.AsyncExecutor;
import org.activiti.engine.impl.cfg.ProcessEngineConfigurationImpl;
public class CustomAsyncExecutorConfiguration {
public void configureAsyncExecutor(ProcessEngineConfigurationImpl processEngineConfiguration) {
AsyncExecutor asyncExecutor = new AsyncExecutor();
asyncExecutor.setWaitTimeInMillis(5000);
asyncExecutor.setMaxAsyncJobsDuePerAcquisition(3);
asyncExecutor.setActive(true);
processEngineConfiguration.setAsyncExecutor(asyncExecutor);
}
}
Why this code is important:
- Wait Time: Specifies the time to wait before checking for new jobs. This prevents overwhelming the job queue.
- Max Jobs: Defines how many jobs can be processed at the same time, balancing load and ensuring responsiveness.
- Active: Ensures the executor starts processing.
Further Configuration:
You may also define additional settings for the Async Executor:
asyncExecutor.setLockTimeInMillis(30000); // Time to hold the lock to prevent simultaneous execution
asyncExecutor.setThreadPoolSize(5); // Number of threads that can work concurrently
These configurations can be adjusted based on your system's resource capacity and your workload requirements.
Submitting Tasks Asynchronously
The next step involves submitting tasks for async execution. You can utilize the RuntimeService
for this purpose. Here is an example:
import org.activiti.engine.RuntimeService;
import org.activiti.engine.runtime.ProcessInstance;
public class ProcessStarter {
private final RuntimeService runtimeService;
public ProcessStarter(RuntimeService runtimeService) {
this.runtimeService = runtimeService;
}
public void startProcessAsync(String processDefinitionId) {
ProcessInstance processInstance = runtimeService.startProcessInstanceByKey(processDefinitionId);
System.out.println("Started process instance: " + processInstance.getId());
}
}
Why start processes asynchronously:
- It leverages the async executor capabilities, ensuring efficient resource management.
- You can handle high-volume requests without blocking, improving overall system throughput.
Handling Job Failures
Even with asynchronous processing, issues may arise. Activiti provides a mechanism for handling job failures, which is crucial for maintaining system stability. Make sure to implement a Job Handler that can be notified in case of any failures.
Here’s a simplistic approach to job retries:
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
try {
// Your custom logic here
} catch (Exception ex) {
throw new JobExecutionException(ex);
}
}
Job Execution Logic:
In this implementation, if an exception occurs, the job can be retried based on your defined strategy (like exponential backoff) which enhances the resilience of your system.
Monitoring and Metrics
Aside from error handling, it is crucial to monitor the performance of your Async Executor. Activiti provides a way to track job losses and failures, allowing you to analyze throughput and processing times.
Leverage tools like Prometheus and Grafana for performance metrics visualization. You could expose the metrics using a simple HTTP endpoint and track job metrics like:
- Total jobs processed
- Failed jobs
- Average execution time
Bringing It All Together
Integrating the Async Executor into your Activiti applications can vastly enhance performance, improve response times, and better manage resources. Proper configuration and implementation are key steps to unlocking the full potential of your workflow engine.
For deeper insights, visit the official Activiti documentation for more advanced features and optimizations.
With modern applications needing to efficiently handle concurrent tasks, adopting an Async Executor becomes more of a necessity than an option. Ensure that your workflows are optimized for performance, and remember to keep monitoring for continuous improvement.
Happy coding!
Checkout our other articles