Common Java Code Pitfalls in Agile Development
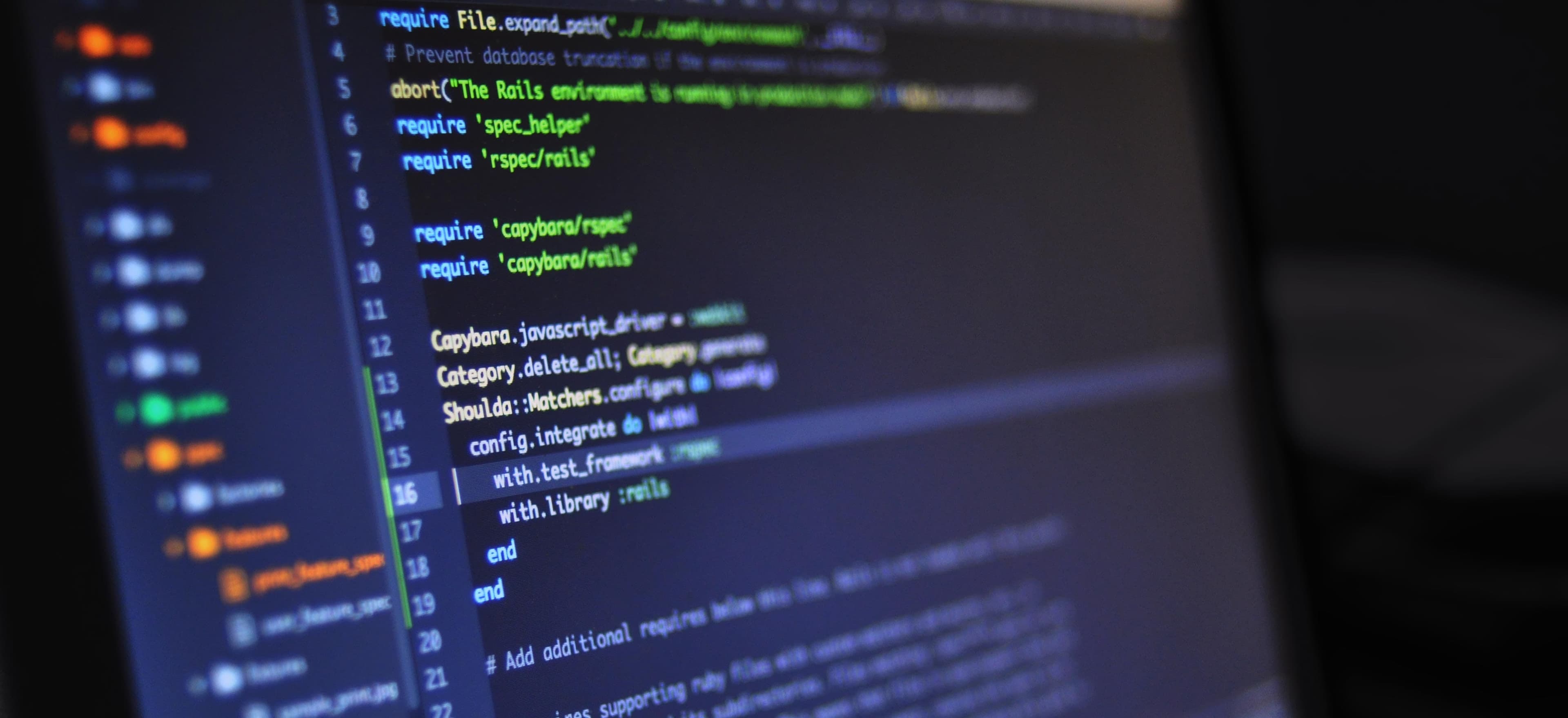
- Published on
Common Java Code Pitfalls in Agile Development
In the dynamic world of Agile development, writing clean and efficient code is imperative for maintaining pace with iterations and delivering value to stakeholders. Java, being one of the most widely used programming languages, offers rich capabilities, but it also has its share of pitfalls that can hinder productivity and software quality. In this blog post, we will explore some common Java code pitfalls in Agile development, provide insightful code examples, and discuss best practices to mitigate these issues.
1. Overusing Inheritance
The Issue
Inheritance is a powerful feature in Java, but overusing it can lead to tightly coupled code and the infamous "fragile base class problem." It's tempting to create extensive inheritance hierarchies, but this can complicate code maintenance and reduce flexibility.
Code Example
class Animal {
void makeSound() {
System.out.println("Animal sound");
}
}
class Dog extends Animal {
void makeSound() {
System.out.println("Bark");
}
}
class Poodle extends Dog {
void makeSound() {
System.out.println("Yip");
}
}
Why This Matters
Each subclass inherits the behavior of its parent class, but changes to any superclass can have unintended consequences on all derived classes. A better approach may be to favor composition over inheritance.
Recommendation
Use interfaces and composition where possible to achieve a flexible and maintainable design.
2. Ignoring NullPointerExceptions
The Issue
In Java, a NullPointerException
(NPE) is a common runtime exception indicating that the application is trying to use an object reference that has not been initialized. Ignoring possible NPE
can lead to unstable applications.
Code Example
public String getPetName(Pet pet) {
return pet.getName(); // This will throw NullPointerException if pet is null
}
Why This Matters
Receiving a NullPointerException
during runtime can disrupt the user experience and make debugging more complicated.
Recommendation
Utilize the Java Optional class to handle potential null values safely:
public String getPetName(Optional<Pet> pet) {
return pet.map(Pet::getName)
.orElse("No Pet"); // Returns "No Pet" if pet is absent
}
3. Failing to Write Unit Tests
The Issue
Unit tests are essential in Agile methodologies, enabling quick verification of code changes. Failing to implement unit tests can result in bugs re-entering the system, slowing down the development process.
Code Example
Consider a simple addition method:
public int add(int a, int b) {
return a + b; // What if we wanted to change the implementation?
}
Why This Matters
Without unit tests, altering this method or expanding its capabilities may introduce new bugs. Testing can catch these issues early.
Recommendation
Employ frameworks like JUnit to create unit tests. Here's how you could test the addition method:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class MathUtilTest {
@Test
public void testAdd() {
MathUtil mathUtil = new MathUtil();
assertEquals(5, mathUtil.add(2, 3));
}
}
4. Mismanaging Exceptions
The Issue
Java features a sophisticated exception handling mechanism; however, failing to use it properly can result in unhandled exceptions propagating throughout the codebase.
Code Example
public void readFile(String filename) {
try {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line = reader.readLine(); // May throw IOException
System.out.println(line);
} catch (IOException e) {
// Silent fail - not good
}
}
Why This Matters
Ignoring exceptions can mask underlying issues, making it hard to track down errors.
Recommendation
Always log or handle exceptions meaningfully:
public void readFile(String filename) {
try {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line = reader.readLine();
System.out.println(line);
} catch (IOException e) {
e.printStackTrace(); // Log the exception for debugging
// Optionally rethrow or handle accordingly
}
}
5. Premature Optimization
The Issue
In Agile development, the focus should often be on delivering working software quickly. Premature optimization can divert attention from implementing features that deliver business value.
Code Example
Consider optimizing a sorting algorithm before confirming that sorting is a bottleneck:
public void sortList(List<String> list) {
// Optimized code - but is it necessary right now?
Collections.sort(list, Collections.reverseOrder());
}
Why This Matters
Focusing on optimization without data can lead to wasted effort on features that may not need it.
Recommendation
Prioritize clear, functional code, defaulting to efficiency only when necessary. Use profiling tools to identify actual bottlenecks.
6. Neglecting Code Reviews
The Issue
In Agile, code reviews are crucial for fostering collaboration and sharing knowledge. Skipping reviews can lead to inconsistencies and an accumulation of technical debt.
Why This Matters
Code that isn't reviewed may hide bugs or poor design choices, leading to long-term issues.
Recommendation
Set up a robust code review process using tools like GitHub or Bitbucket. Encourage team members to provide constructive feedback. Even a simple checklist can ensure key aspects are covered.
The Bottom Line
Java provides a robust framework for building applications, but pitfalls can hamper development in an Agile environment. By combining best practices with the lessons learned discussed in this post, developers can enhance code quality, improve maintainability, and ultimately deliver better software.
In the spirit of Agile development, remember that iterative improvement applies not only to product features but also to your coding practices. With these common pitfalls identified and the best practices outlined, you can confidently navigate the complexities of Java development within agile systems.
For comprehensive Agile practices, visit Agile Alliance, and for deeper insights into writing clean Java code, check out Clean Code by Robert C. Martin.
Happy coding!
Checkout our other articles