Struggling with Load Balancing in Microservices? Here's How!
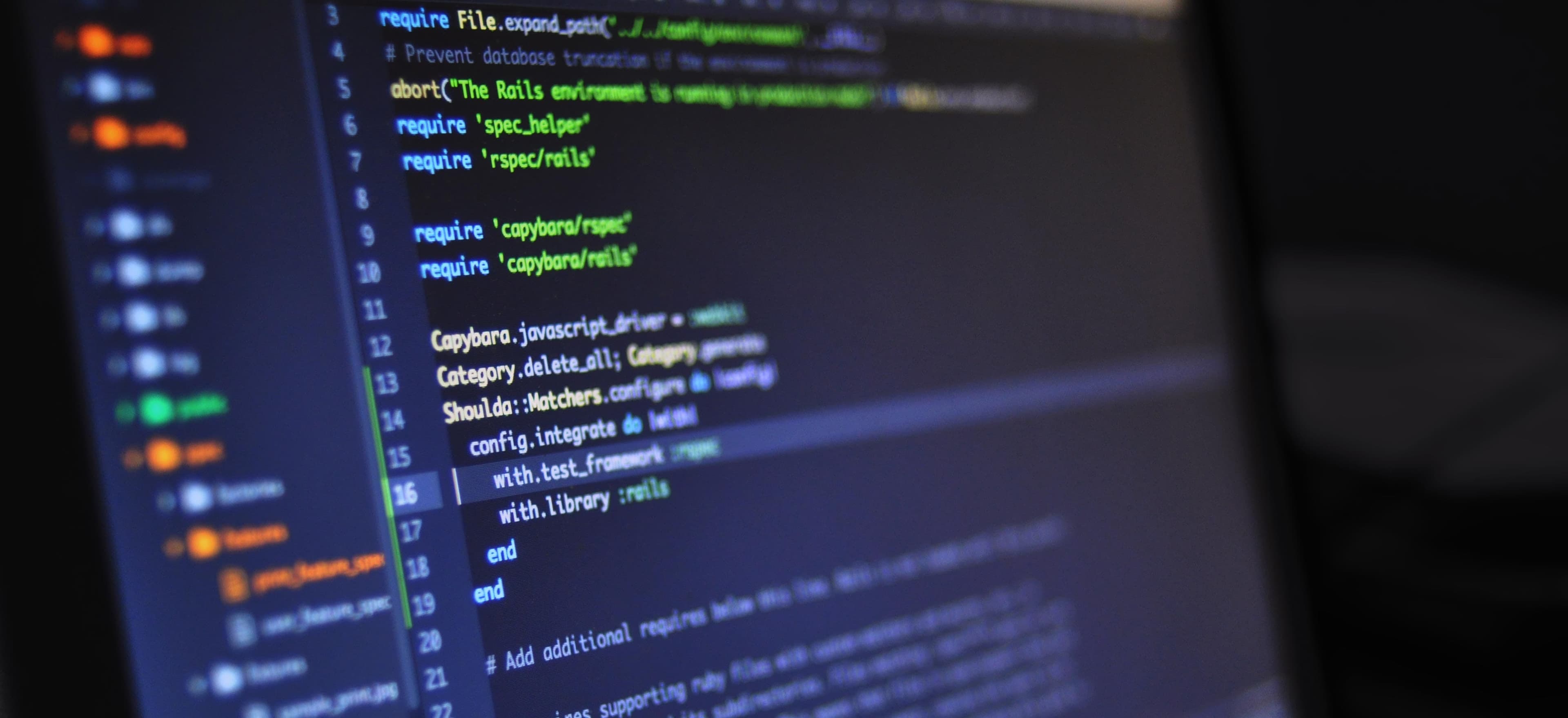
- Published on
Struggling with Load Balancing in Microservices? Here's How!
In today's cloud-native world, microservices have gained immense popularity due to their scalability, flexibility, and resilience. However, with great power comes great responsibility, particularly when it comes to ensuring efficient load balancing across these microservices. In this blog post, we will delve into the significance of load balancing in microservices, explore different load balancing strategies, and provide practical tips to implement effective load balancing in your systems.
What is Load Balancing?
Load balancing is the process of distributing incoming network traffic across multiple servers. This ensures that no single server becomes overwhelmed, allowing applications to maintain high availability and reliability. In a microservices architecture, effective load balancing plays a critical role in optimizing resource utilization and improving performance.
Why Load Balancing is Crucial for Microservices
-
Improved Performance: Load balancers prevent bottlenecks by distributing requests evenly across multiple instances of a service, leading to faster response times.
-
Scalability: As demand increases, you can easily add more service instances without significant reconfiguration. Load balancing allows your infrastructure to grow naturally in response to user demand.
-
High Availability: A load balancer can detect unhealthy service instances and redirect traffic to healthy ones, ensuring your application remains operational even in the face of server failures.
-
Traffic Management: Different traffic types (such as API calls, web requests, etc.) can be routed to different service instances based on rules defined in the load balancer.
Load Balancing Strategies
Understanding the different load balancing strategies is invaluable for selecting the right approach for your microservices architecture. Let's explore the most common ones:
1. Round Robin
Round Robin is one of the simplest load balancing algorithms. In this method, incoming requests are distributed to each service instance sequentially.
Example Scenario: If you have three service instances A, B, and C, the requests will be served in the following order: A → B → C → A → B → C...
This method works well when all instances have similar capacities. However, it may not be the best choice for services with varying loads.
2. Least Connections
In this strategy, a load balancer forwards the incoming request to the instance with the fewest active connections. This way, the system maximizes capacity and minimizes response time.
Example Code Snippet: Below is a theoretical implementation of a Least Connections load balancer.
import java.util.HashMap;
import java.util.Map;
public class LeastConnectionsLoadBalancer {
private Map<String, Integer> connectionCounts = new HashMap<>();
public void registerInstance(String instance) {
connectionCounts.put(instance, 0);
}
public String selectInstance() {
return connectionCounts.entrySet().stream()
.min(Map.Entry.comparingByValue())
.map(Map.Entry::getKey)
.orElseThrow(() -> new RuntimeException("No instances available"));
}
public void reportConnection(String instance) {
connectionCounts.put(instance, connectionCounts.get(instance) + 1);
}
}
In this example, we maintain a map that tracks the number of connections to each instance and select the one with the least connections.
3. IP Hash
IP Hash is a strategy that directs a request to a specific instance based on the client’s IP address. This ensures that users consistently receive responses from the same instance, which can improve cache performance.
Why use IP Hash?
- Users experience faster retrieval of data.
- Avoids session issues as the same client is directed to the same server.
4. Random
A simple approach where requests are directed to a service instance at random. The randomness can help distribute load but may cause uneven resource utilization—especially when instances have varying capacities.
Implementing Load Balancing in Microservices
Implementing effective load balancing in a microservices architecture can be achieved through various tools and frameworks designed for this purpose. Here, we will analyze some popular options:
1. Nginx
Nginx is a widely used web server that can act as a load balancer. By its configuration, you can define how requests should be distributed across your microservices.
http {
upstream backend {
server backend1.example.com;
server backend2.example.com;
server backend3.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
}
In this example, Nginx routes incoming requests to the backend servers defined in the upstream
block.
2. Kubernetes
Kubernetes provides built-in load balancing. When you create a Service in Kubernetes, it automatically load balances traffic across all pods that match the selector.
apiVersion: v1
kind: Service
metadata:
name: my-service
spec:
selector:
app: my-app
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: LoadBalancer
In the above configuration, Kubernetes will distribute traffic coming to my-service
to all pods labeled with app: my-app
.
3. AWS Elastic Load Balancing
If you're using AWS, the Elastic Load Balancing (ELB) service can help manage load balancing without much effort. With Auto Scaling and ELB, you can ensure high availability and distribute loads just by setting rules in the AWS management console.
In Conclusion, Here is What Matters: Load Balancing Best Practices
While load balancing can significantly enhance your microservices architecture, it is equally essential to follow best practices:
-
Monitor Performance: Regularly monitor the performance of each service instance and identify anomalies.
-
Implement Health Checks: Use health checks to ensure traffic is only routed to healthy, functional service instances.
-
Select the Appropriate Strategy: Choose a load balancing strategy based on your application's needs, user behavior, and resource capacities.
-
Utilize Redundancy: Having multiple load balancers can provide extra reliability and fault tolerance.
-
Test Under Load: Simulate high load conditions regularly to evaluate your load balancing strategy.
By understanding and implementing effective load balancing in your microservices architecture, you will not only enhance user experience but also ensure scalable and maintainable applications.
For more in-depth insights into microservices and load balancing, check out these helpful resources:
Whether you are new to microservices or an experienced user, this guide serves as a foundation for navigating through the complexities of load balancing and improving performance by optimizing traffic distribution.
Checkout our other articles