Transforming Flat Scrollbars: A Guide to SWT Customization
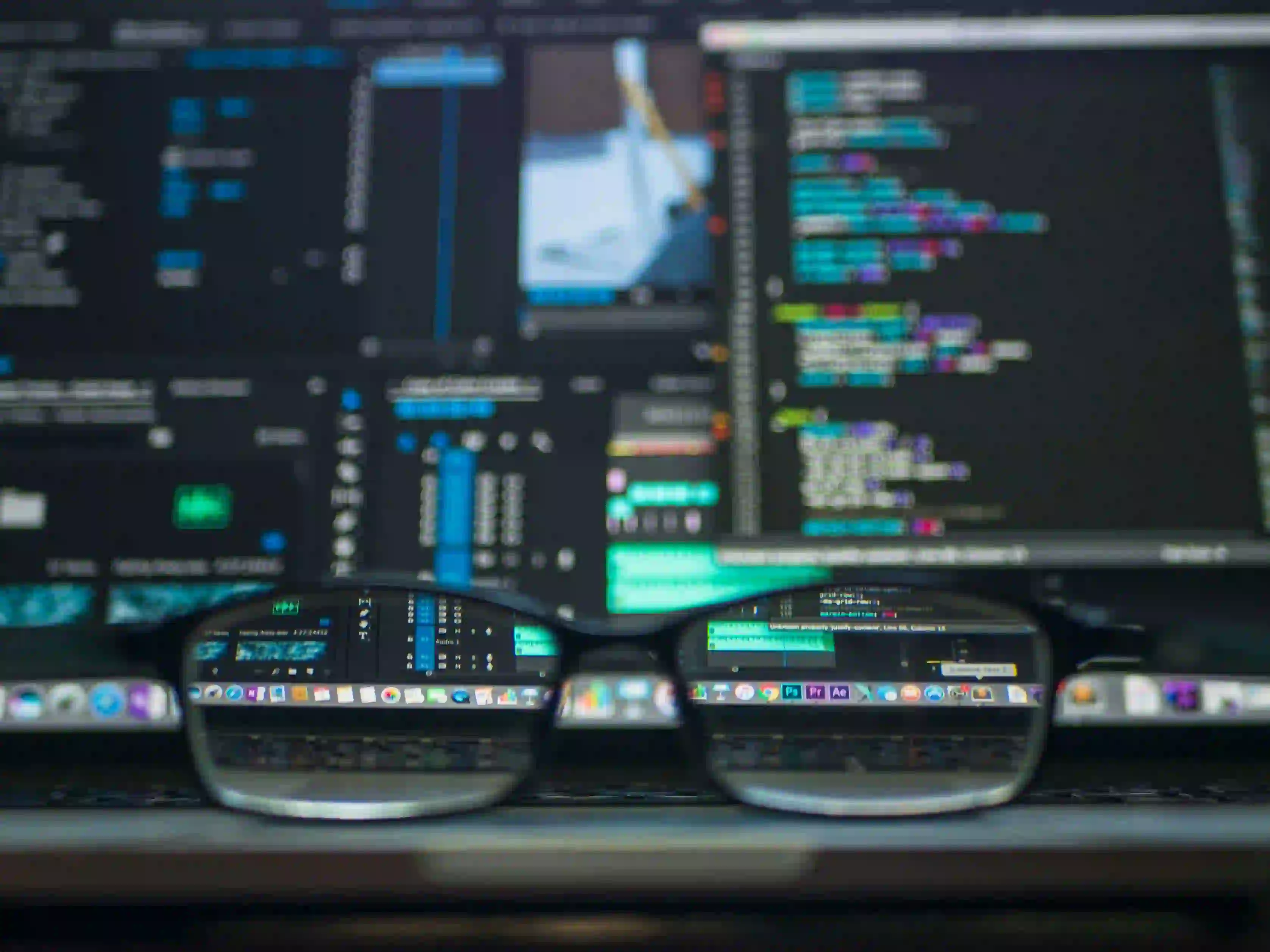
Transforming Flat Scrollbars: A Guide to SWT Customization
The Approach
The Standard Widget Toolkit (SWT) provides a rich set of GUI components for Java developers, particularly those focused on building cross-platform applications. Among these components, scrollbars are pivotal for user interaction, especially in applications dealing with large datasets or extensive content.
This blog post will delve into transforming standard flat scrollbars in SWT, enhancing their aesthetic appeal and providing a better user experience. We will discuss step-by-step customization, demonstrate essential code snippets, and explain the rationale behind each approach.
Why Customize Scrollbars?
By default, scrollbars in SWT applications are functional but often lack visual distinction. The transformation of these scrollbars aims to:
- Enhance visual aesthetics: A customized scrollbar can align with the overall application design.
- Improve usability: Custom scrollbars can provide users with visual feedback.
- Brand identity: Unique components can reinforce the identity of an application.
With that said, let's dive into how you can customize scrollbars in SWT effectively.
Setting Up Your Environment
Before we start customizing, ensure you have the following setup:
- Java Development Kit (JDK): Version 8 or higher.
- Eclipse IDE: The most common IDE for SWT development. Download it from Eclipse Official Site.
- SWT Library: If you haven't already included SWT in your project, you can download the latest version from SWT Releases Page.
Here’s how you can include the SWT library in your Eclipse Project:
- Right-click on your project in the Project Explorer.
- Select "Build Path" > "Add External Archives."
- Navigate to the downloaded SWT jar file and add it.
Basic Scrollbar Implementation
First, let’s implement a simple SWT application with a scrollbar. This will serve as our starting point.
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
import org.eclipse.swt.widgets.ScrollBar;
public class BasicScrollbarExample {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("Basic Scrollbar Example");
shell.setSize(300, 200);
Text textArea = new Text(shell, SWT.MULTI | SWT.BORDER | SWT.V_SCROLL | SWT.H_SCROLL);
textArea.setBounds(10, 10, 260, 150);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
Explanation
- Text Widget: A multi-line text area is created with vertical and horizontal scrollbars.
- The application initializes a shell and displays the text area where text will overflow, prompting the need for scrollbars.
Customizing Scrollbars
Now, let's customize the scrollbars. SWT allows us to achieve this by drawing custom components.
Step 1: Create a Canvas for Custom Scrollbars
We need a canvas for drawing the custom scrollbars.
import org.eclipse.swt.graphics.Color;
import org.eclipse.swt.graphics.GC;
import org.eclipse.swt.widgets.Canvas;
public class CustomScrollbarCanvas extends Canvas {
private int scrollPosition = 0;
private Color scrollbarColor;
public CustomScrollbarCanvas(Shell parent) {
super(parent, SWT.BORDER);
scrollbarColor = new Color(getDisplay(), 100, 100, 255); // Light blue
this.addPaintListener(event -> {
GC gc = event.gc;
gc.setBackground(scrollbarColor);
// Draw the scrollbar
gc.fillRectangle(10, scrollPosition, 20, 100); // Example dimensions
});
this.setBounds(250, 10, 30, 150);
}
public void setScrollPosition(int position) {
this.scrollPosition = position;
this.redraw();
}
}
Explanation
- GC (Graphics Context): The
GC
class is utilized to handle custom drawing. - Color: A custom light blue color for the scrollbar.
- Redraw Method: When the scroll position changes, a redraw occurs to reflect this change.
Step 2: Integrating the Custom Scrollbar
Now, integrate the CustomScrollbarCanvas
into the existing BasicScrollbarExample
.
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class CustomScrollbarExample {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("Custom Scrollbar Example");
shell.setSize(300, 200);
Text textArea = new Text(shell, SWT.MULTI | SWT.BORDER | SWT.V_SCROLL | SWT.H_SCROLL);
textArea.setBounds(10, 10, 220, 150);
CustomScrollbarCanvas customScrollbar = new CustomScrollbarCanvas(shell);
// Handle scroll events
textArea.addListener(SWT.MouseWheel, event -> {
int newPosition = customScrollbar.getScrollPosition() - event.count;
customScrollbar.setScrollPosition(Math.max(0, Math.min(newPosition, 150))); // Limit scroll position
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
Explanation
- Mouse Scroll Listener: This captures mouse wheel events to update the scrollbar's position dynamically.
- Scroll Bounds: We limit the scrollbar's position to avoid out-of-bounds scrolling.
Enhancing User Interaction with Hover Effects
Adding hover effects can significantly improve the user experience.
Step 1: Update the Custom Scrollbar Canvas for Hover Effects
Modify the CustomScrollbarCanvas
to handle mouse hover events.
public class CustomScrollbarCanvas extends Canvas {
private Color hoverColor;
private boolean isHovered;
public CustomScrollbarCanvas(Shell parent) {
super(parent, SWT.BORDER);
scrollbarColor = new Color(getDisplay(), 100, 100, 255);
hoverColor = new Color(getDisplay(), 150, 150, 255); // Darker blue
this.addMouseMoveListener(event -> {
if (event.x > 10 && event.x < 30 && event.y > scrollPosition && event.y < scrollPosition + 100) {
isHovered = true;
redraw();
} else {
isHovered = false;
redraw();
}
});
// Rest of your paint logic...
}
@Override
public void paintControl(PaintEvent e) {
GC gc = e.gc;
gc.setBackground(isHovered ? hoverColor : scrollbarColor);
gc.fillRectangle(10, scrollPosition, 20, 100);
}
}
Explanation
- Mouse Move Listener: This listens for mouse movements to determine if the mouse hovers over the scrollbar.
- Dynamic Background Color: The scrollbar’s color changes depending on the hover state for better visibility.
A Final Look
Customizing scrollbars in SWT can significantly elevate your application's design and usability. By leveraging SWT's capabilities in custom drawing and event handling, you can create visually appealing and user-friendly components.
This guide walks through creating a customized scrollbar step-by-step, including engaging visuals and interactive elements. Embracing such customization can lead to a refined user experience and a cohesive application interface.
For more detailed information on SWT, visit the official Eclipse SWT Documentation.
Next Steps
- Explore additional SWT features and their customization.
- Experiment with other widgets and their visual properties.
- Share your custom SWT components or discuss your experience on forums to inspire others.
Whether you're developing a new application or enhancing an existing one, remember that thoughtful customization sets an application apart. Happy coding!