Understanding Transaction Isolation: Spring Visibility Issues
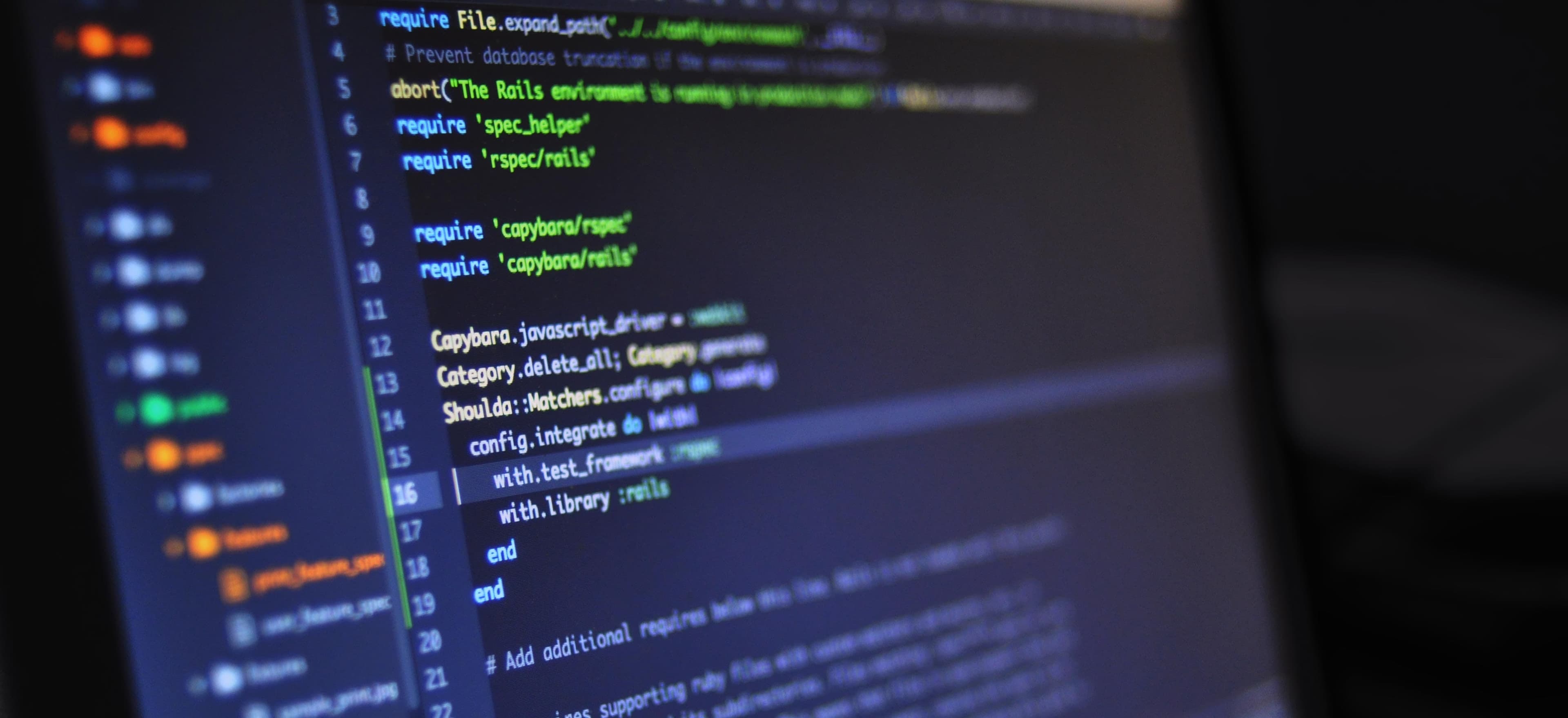
- Published on
Understanding Transaction Isolation: Spring Visibility Issues
In modern application development, one of the most critical aspects of working with databases involves managing how transactions interact with each other. This interaction can significantly affect application performance and data consistency. In this blog post, we will delve deep into transaction isolation levels in Spring, discuss visibility issues that can arise, and highlight how to effectively manage these challenges.
What is Transaction Isolation?
Transaction isolation is a property that defines how and when changes made by one transaction become visible to other transactions. It is a crucial concept in database management systems (DBMS), ensuring data integrity and consistency.
The ACID Properties
ACID stands for Atomicity, Consistency, Isolation, and Durability. These properties are critical for achieving reliable transactions.
- Atomicity: Ensures that all operations within a transaction are completed successfully or none at all.
- Consistency: Guarantees that a transaction brings the database from one valid state to another.
- Isolation: Determines how transaction integrity is visible to other transactions.
- Durability: Ensures that the results of a successful transaction are permanent, even in the case of a system failure.
Transaction Isolation Levels
The SQL standard defines four transaction isolation levels, each balancing between performance and consistency:
- Read Uncommitted: Allows dirty reads. This means a transaction can read changes made by others that have not yet been committed.
- Read Committed: Prevents dirty reads. Transactions can't see uncommitted changes made by other transactions.
- Repeatable Read: Ensures that if a transaction reads data multiple times, it will see the same values every time. It prevents non-repeatable reads.
- Serializable: The highest level of isolation, which prevents all concurrency issues, but it is also the most restrictive and impacts performance.
Spring’s Approach to Handling Isolation Levels
In Spring, you can set transaction isolation levels using the @Transactional
annotation. Here’s how you do it.
import org.springframework.transaction.annotation.Transactional;
public class UserService {
@Transactional(isolation = Isolation.READ_COMMITTED)
public void updateUserName(Long userId, String newName) {
// Code to update user name in the database
}
}
In this code snippet, we are specifying that the updateUserName
method should use the READ COMMITTED isolation level. This means that it will not be able to see uncommitted changes from other transactions, ensuring that the name update is clean and consistent after it's executed.
Why Isolation Matters
Let’s explore some practical scenarios to understand why transaction isolation levels are vital.
-
Concurrent Transactions: In high-traffic applications, many transactions are happening simultaneously. Without proper isolation levels, you may end up with a situation where a transaction reads data that is in the process of being changed by another transaction, leading to inconsistent results.
-
Data Integrity: Consider a banking application that needs to ensure that no two transactions can deduct funds from the same account simultaneously, resulting in overdrafts. Using a serialization level prevents this unwanted behavior.
Visibility Issues in Spring
Visibility issues typically arise when transactions run concurrently, and their isolation levels are not set correctly. Here, we’ll discuss common visibility issues developers face:
Dirty Reads
A dirty read occurs when a transaction reads data that has not been committed by another transaction. This can lead to inconsistencies.
Example of Dirty Read Scenario:
@Transactional(isolation = Isolation.READ_UNCOMMITTED)
public void readUncommittedData() {
// This might read data that is about to be committed by another transaction
}
In this scenario, if you read data from a transaction that is later rolled back, your application has made decisions based on invalid data.
Non-Repeatable Reads
This occurs when a transaction reads the same record twice and gets different values.
Example of Non-Repeatable Read:
@Transactional(isolation = Isolation.REPEATABLE_READ)
public void readMultipleTimes() {
User user = userRepository.findById(userId);
// ... perform some logic
User userAgain = userRepository.findById(userId); // This could be different!
}
By using the REPEATABLE READ isolation level, you ensure the data remains consistent throughout the transaction scope.
Phantom Reads
Phantoms occur when a transaction reads a set of rows that match a certain condition, and a subsequent read within the same transaction finds rows that match the condition but were added by another transaction.
Example of Phantom Read:
@Transactional(isolation = Isolation.SERIALIZABLE)
public void checkEmployeeCount() {
List<Employee> employees = employeeRepository.findAllActive();
// ... perform updates and checks
}
Using SERIALIZABLE ensures that no new rows can be added to the employees
table while you're processing your transaction.
Mitigating Visibility Issues
1. Choose the Right Isolation Level
Understand the needs of your application. High isolation levels improve data integrity at the cost of performance. Lower levels provide better performance but risk inconsistency.
2. Partitioning and Sharding
For high-traffic applications, consider partitioning or sharding your database. This approach distributes transactions across multiple database instances, reducing contention and improving performance while ensuring isolation.
3. Optimistic Locking
Implement optimistic locking to handle concurrent updates safely. By using a versioning strategy (a timestamp or version number), your application can avoid overwriting uncommitted changes from other transactions.
4. Spring Locking Mechanisms
Spring provides various mechanisms to support locking. Using features such as @Version
for optimistic locking can automatically manage versioning. For example:
@Entity
public class User {
@Id
private Long id;
@Version
private Long version;
private String name;
// getters and setters
}
This will prevent inconsistent updates if two transactions attempt to update the same record.
A Final Look
Managing transaction isolation and visibility issues in Spring is critically important for ensuring data integrity and consistency in your applications. By understanding the different isolation levels and their implications, you can make better decisions on how to balance performance and integrity.
For more comprehensive guidance on transaction management in Spring, check the Spring Transaction Documentation and the Transactional Management Guide.
In the world of concurrent applications, awareness and consideration of transaction isolation principles are the keys to building robust systems. Implement these best practices, and your applications will thrive under the pressure of competing demands.
Checkout our other articles