Mastering Spring Profiles: Common Pitfalls to Avoid
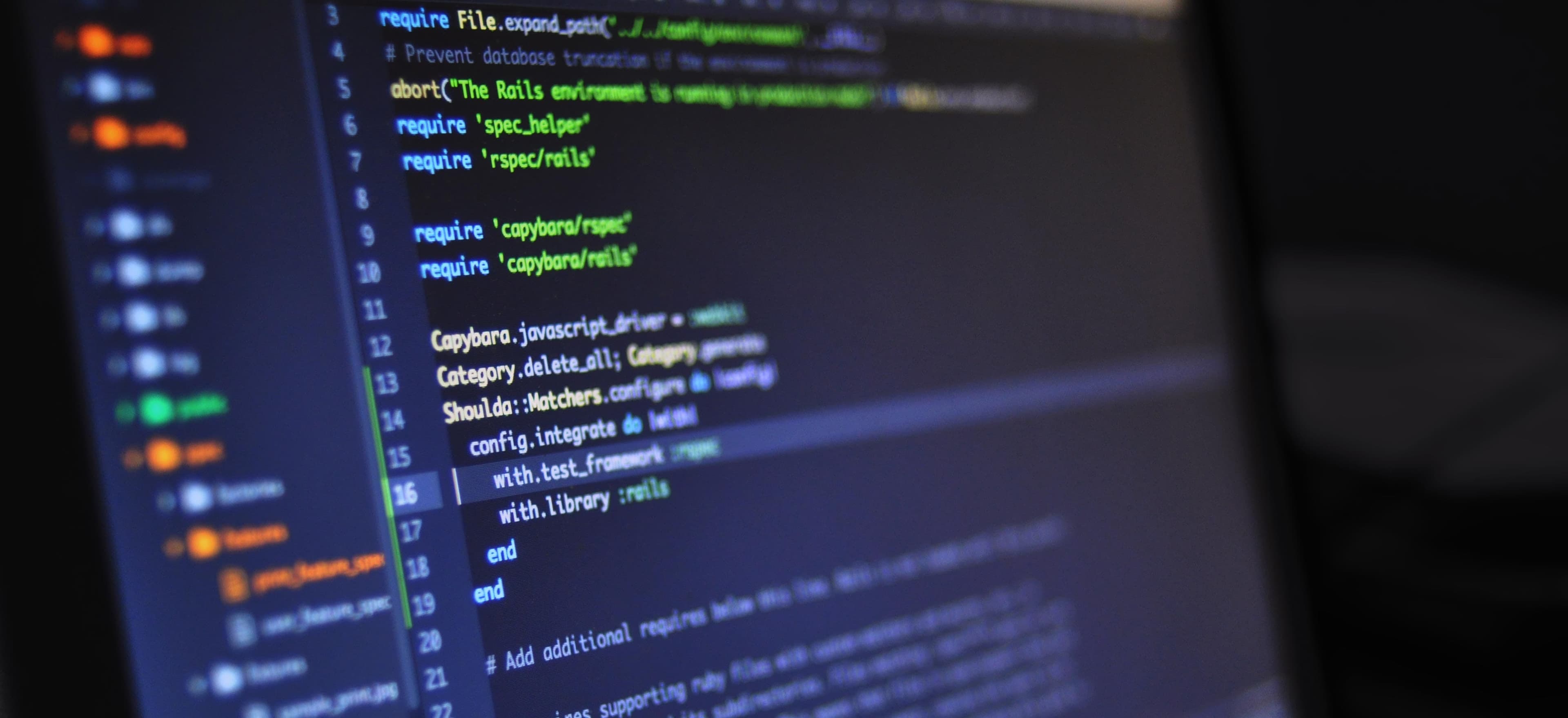
- Published on
Mastering Spring Profiles: Common Pitfalls to Avoid
Spring Profiles provide a powerful way to segregate parts of your application configuration and make it only available in certain environments. However, while they are incredibly useful, developers often run into common pitfalls that can lead to confusion and errors. In this post, we will explore these pitfalls, understand why they occur, and learn how to avoid them.
Understanding Spring Profiles
Before delving into the common pitfalls, let’s quickly outline what Spring Profiles are. Spring allows you to define different configuration settings for different environments (development, testing, production, etc.) using profiles. You can isolate configurations so that your application behaves differently based on the environment it is running in.
Basic Setup of Spring Profiles
To define a profile in Spring Boot, you typically use the application-{profile}.properties
or application-{profile}.yml
files. For example, let’s create a development
profile by defining application-development.properties
as shown below:
# application-development.properties
server.port=8080
spring.datasource.url=jdbc:mysql://localhost:3306/dev_db
spring.datasource.username=root
spring.datasource.password=password
Activating a Profile
Profiles can be activated in various ways; through the command line, IDE, or in your application code. Here is how you can activate a profile from the command line:
java -jar myapp.jar --spring.profiles.active=development
Common Pitfalls to Avoid
Let’s discuss the common pitfalls related to Spring Profiles and how to avoid them.
1. Not Defining Default Profile
One common oversight is failing to define a default profile. If no profiles are specified and you have not set a default, Spring will not know which configurations to load.
Solution:
You can define a default profile in application.properties
with:
spring.profiles.default=default
This ensures that when no profile is set, your application still runs with the default configurations.
2. Profile-Specific Beans
It’s easy to get carried away and define beans specific to a profile without realizing it might conflict with other profiles.
Example:
@Configuration
@Profile("development")
public class DevDatabaseConfig {
@Bean
public DataSource dataSource() {
// Development data source configuration
return new HikariDataSource();
}
}
@Configuration
@Profile("production")
public class ProdDatabaseConfig {
@Bean
public DataSource dataSource() {
// Production data source configuration
return new HikariDataSource();
}
}
Solution:
Always ensure that bean definitions do not overlap unless intended. Use careful naming conventions to avoid confusion.
3. Overlapping Configurations
Having overlapping properties in your profile-specific configuration files can cause unexpected behavior.
Example:
# application-default.properties
spring.datasource.url=jdbc:mysql://localhost:3306/default_db
# application-development.properties
spring.datasource.url=jdbc:mysql://localhost:3306/dev_db
Potential Issue:
If you reference both properties in your code, the application can use either of them, depending on how they are resolved.
Solution:
Use @ConditionalOnProperty
annotations to ensure that certain beans only load when specific properties exist.
4. Not Testing Profiles Thoroughly
Often, developers assume that if the application works in one profile, it will work in all others. Failing to test different profiles exhaustedly can cause hard-to-diagnose issues in production.
Solution:
Implement integration tests specific to each profile to verify the configurations and beans behave as expected.
@RunWith(SpringRunner.class)
@ActiveProfiles("development")
@SpringBootTest
public class DevEnvironmentTests {
@Autowired
private DataSource dataSource;
@Test
public void testDatabaseConnection() {
assertNotNull(dataSource);
}
}
Testing ensures that your configurations behaves as expected, ultimately reducing runtime errors.
5. Missing Bean Inheritance
When defining beans in parent context, they may not be available in child contexts due to incorrect profile configurations.
Solution:
Ensure that beans are defined at the appropriate level based on the necessity and inheritance chain.
Here is an example of a parent class with profile-specific child classes:
@Configuration
public abstract class BaseConfig {
@Bean
public abstract SomeService someService();
}
@Configuration
@Profile("development")
public class DevConfig extends BaseConfig {
@Override
public SomeService someService() {
return new DevSomeService();
}
}
@Configuration
@Profile("production")
public class ProdConfig extends BaseConfig {
@Override
public SomeService someService() {
return new ProdSomeService();
}
}
This structure ensures that relevant services are provided based on activated profiles.
6. Configuration Files Location
Placing profile-specific configuration files in the wrong location can lead to them not being recognized by Spring.
Solution:
Make sure your profile files are located in src/main/resources
.
7. Misuse of Spring Profiles in CI/CD
Using different configurations without careful management in CI/CD pipelines can lead to deploying the wrong environment configuration.
Solution:
Always confirm the active profile in your CI/CD configuration files and include validation steps to ensure the correct profile is set during builds.
8. Ignoring Security Configurations
Neglecting to adequately secure the application.properties
files can expose sensitive information like database passwords.
Solution:
Consider using a cloud-based configuration server or environment variables to manage sensitive configurations.
spring.datasource.password=${DB_PASSWORD}
In Conclusion, Here is What Matters
Spring Profiles are a powerful feature that, when used correctly, can help you organize your application better. However, pitfalls are lurking at every corner. Understanding these pitfalls and implementing the solutions discussed in this post can make your journey with Spring Profiles much smoother.
By adopting best practices like thorough testing of profiles, managing overlapping configurations, and ensuring security in your properties files, you can master the use of Spring Profiles and enhance the reliability of your applications.
For more information on handling Spring Profiles, consider checking the official Spring Documentation.
Happy coding!
Checkout our other articles