Effortless Queue to List Conversion in Java: A Step-by-Step Guide
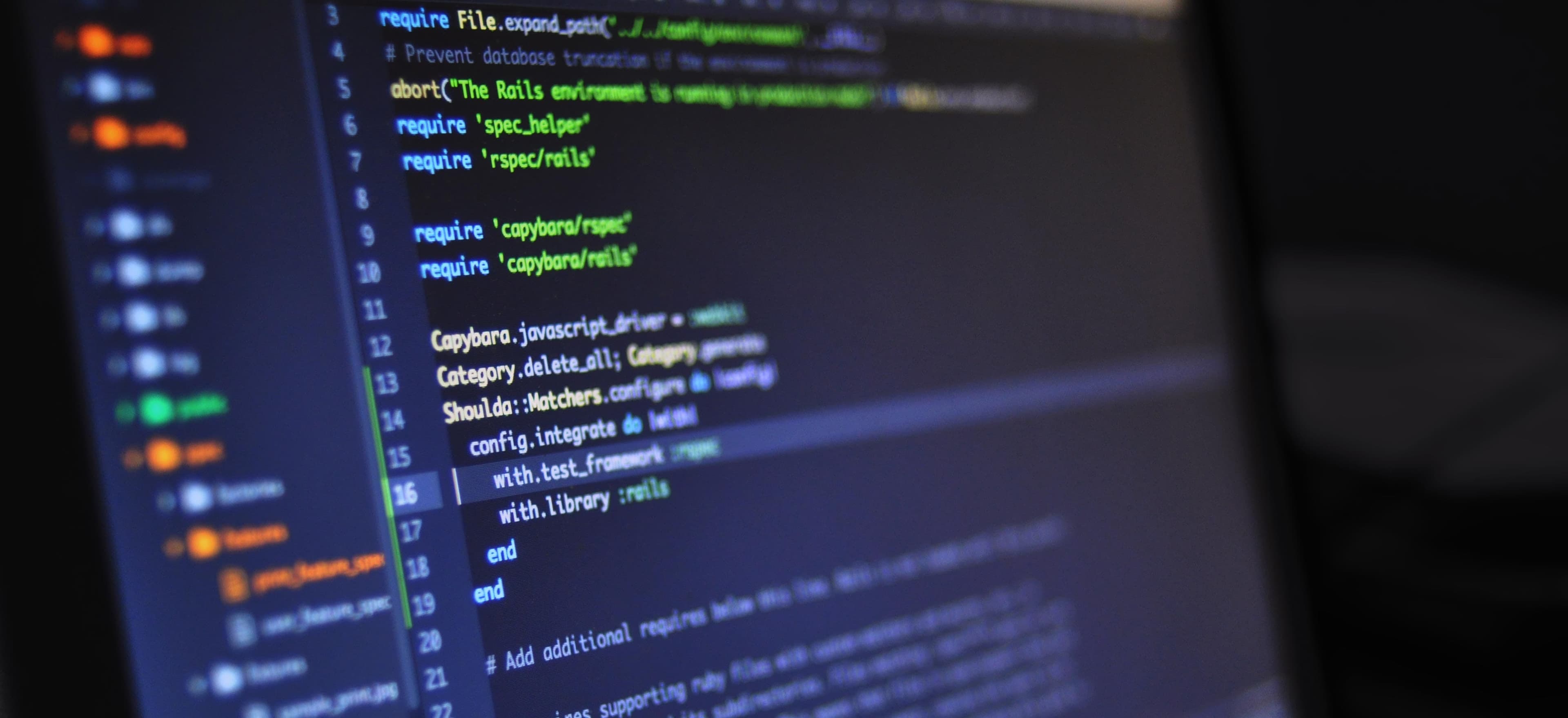
- Published on
Effortless Queue to List Conversion in Java: A Step-by-Step Guide
In Java, data structures like queues and lists are essential for organizing and managing collections of data. Often, you might find yourself needing to convert a queue to a list for easier data manipulation. In this blog post, we'll explore how to perform this conversion effortlessly, providing examples and best practices along the way.
Understanding Data Structures: Queue vs. List
Before diving into the conversion process, let’s briefly recap what a queue and a list are in the context of Java's Collections Framework.
- Queue: A queue is a linear collection that follows the First-In-First-Out (FIFO) principle. It is typically used for tasks such as scheduling and managing threading.
- List: A list is an ordered collection that allows for duplicate elements. Lists provide versatile data operations, including random access and variable size.
Java provides several implementations for both interfaces. The LinkedList
and ArrayDeque
classes are common choices for queues, while ArrayList
and LinkedList
are popular list implementations.
The Need for Conversion
There are several reasons you might want to convert a queue to a list:
- Data Processing: You may want to manipulate the data in a more flexible manner.
- Random Access: Lists offer better performance for accessing elements by index.
- Library Compatibility: Some libraries or APIs only accept lists as parameters.
Step-by-Step Conversion from Queue to List
Step 1: Import Necessary Packages
First, you need to import the required classes. Ensure you include the java.util
package, which contains the necessary classes for both queues and lists.
import java.util.Queue;
import java.util.LinkedList;
import java.util.List;
import java.util.ArrayList;
Step 2: Create a Queue and Populate It
Next, let’s create a queue and add some elements. We'll use the LinkedList
class to implement our queue.
Queue<String> queue = new LinkedList<>();
queue.add("Apple");
queue.add("Banana");
queue.add("Cherry");
Why LinkedList?
Using LinkedList
is advantageous here because it efficiently supports insertion and deletion operations, which are paramount for queues.
Step 3: Convert the Queue to a List
Now that we have populated our queue, we can easily convert it into a list. The simplest way to achieve this is by using the ArrayList
constructor which can accept a collection as a parameter.
List<String> list = new ArrayList<>(queue);
Full Example: Queue to List Conversion
Putting it all together, here’s a complete example demonstrating the conversion process.
import java.util.Queue;
import java.util.LinkedList;
import java.util.List;
import java.util.ArrayList;
public class QueueToListExample {
public static void main(String[] args) {
// Step 1: Create and populate the queue
Queue<String> queue = new LinkedList<>();
queue.add("Apple");
queue.add("Banana");
queue.add("Cherry");
// Step 2: Convert the queue to a list
List<String> list = new ArrayList<>(queue);
// Step 3: Display the list
System.out.println("Converted List: " + list);
}
}
Output
When running the above code, you should see the following output:
Converted List: [Apple, Banana, Cherry]
This output confirms that our queue is successfully converted into a list while maintaining the order of elements.
Alternative Methods for Conversion
While the method shown is the most straightforward, there are other ways to convert a queue to a list. Here are two additional methods:
Method 1: Using a Loop
You can also create a list manually by iterating through the queue and adding each element.
List<String> listManual = new ArrayList<>();
while (!queue.isEmpty()) {
listManual.add(queue.poll());
}
Why Use a Loop?
This method allows you to process each element before adding it to the list, useful for manipulations or transformations during the transition.
Method 2: Using Java Streams
For those using Java 8 or higher, you can take advantage of the Stream API for a more elegant solution.
List<String> listStream = queue.stream().toList();
Why Streams?
Streams provide a more functional and fluent approach to handling data, making your code cleaner and often more readable.
Performance Considerations
While converting a queue to a list is generally efficient, it is essential to be aware of potential performance implications when dealing with large datasets.
- Memory Usage: Converting a queue to a list duplicates the data in memory. Ensure you have sufficient resources, especially with large collections.
- Time Complexity: The conversion typically operates in O(n) time complexity, where n is the number of elements in the queue.
Closing the Chapter
Converting a queue to a list in Java is a straightforward task that can enhance your data manipulation capabilities. Whether you choose the simple constructor approach, manual iteration, or Java Streams, each method has its unique advantages.
By understanding your data structure needs and employing the right conversion techniques, you can make your Java applications more robust and flexible.
For further reading on Java Collections and data structure manipulation, explore the official Java Documentation and consider diving into more advanced topics like parallel processing with streams and concurrency with queues.
Feel free to share your conversion experiences or implementations in the comments below! Happy coding!
Checkout our other articles