Avoiding Overcomplication: Mastering the Decorator Pattern
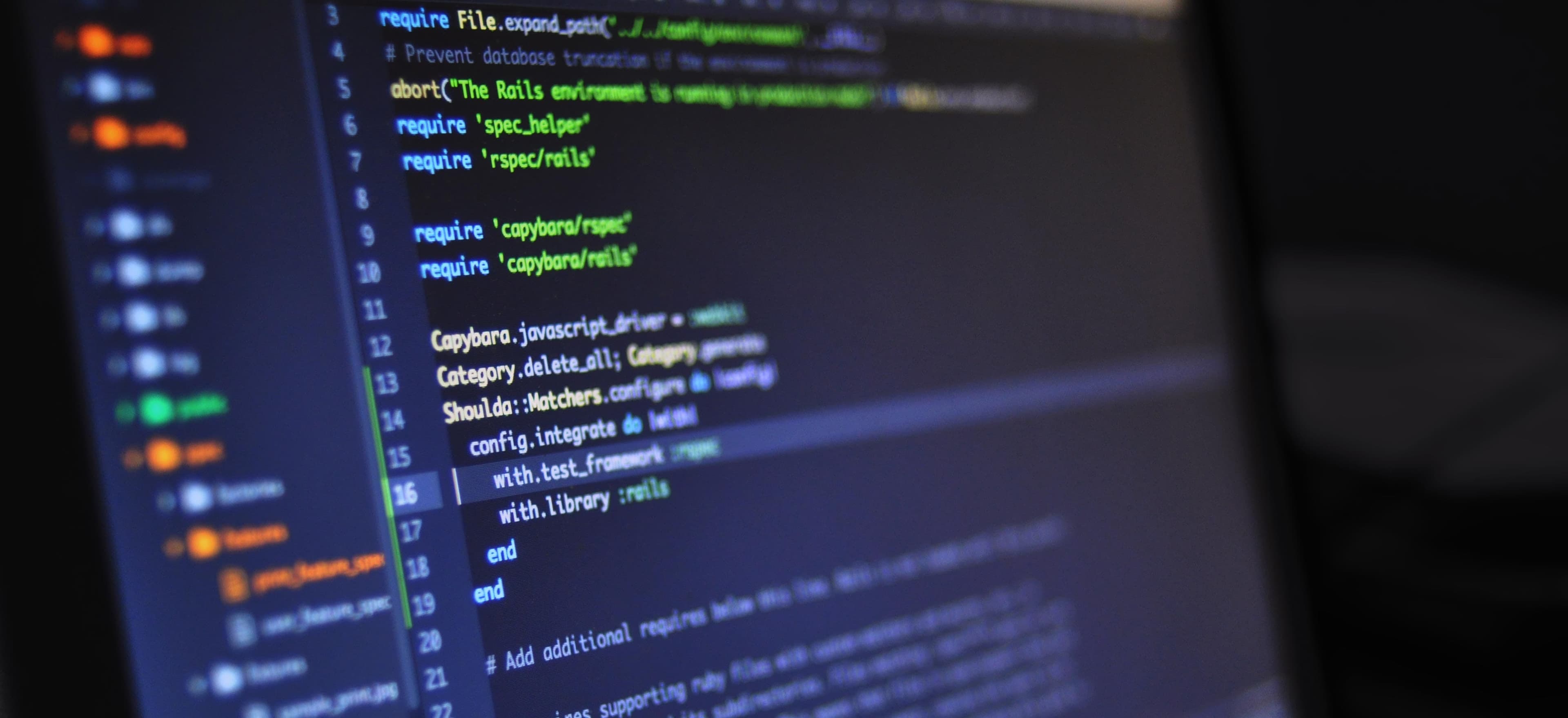
- Published on
Avoiding Overcomplication: Mastering the Decorator Pattern in Java
Java is a powerful object-oriented programming language that provides numerous design patterns to address various programming challenges. Among these, the Decorator Pattern is particularly useful when you want to add new responsibilities to objects dynamically. This pattern helps you avoid the pitfalls of subclassing, promoting better software design, and enhanced flexibility.
In this blog post, we'll delve deep into the Decorator Pattern, explain its purpose, structure, and provide clear examples to illustrate its effectiveness.
What is the Decorator Pattern?
The Decorator Pattern falls under the category of structural design patterns. It allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class. It provides a flexible alternative to subclassing for extending functionality.
Key Benefits:
- Single Responsibility Principle: By using decorators, you can keep your classes focused on a single concern.
- Open/Closed Principle: The pattern allows classes to be open for extension but closed for modification.
- Flexibility: With decorators, you can stack multiple functionalities on an object seamlessly.
Structural Overview
The Decorator Pattern consists of four main components:
- Component: This is the interface or abstract class for objects that can have responsibilities added to them.
- Concrete Component: This is a class that implements the Component interface. It defines the core behavior.
- Decorator: This class implements the Component interface and has a reference to a Component object. It delegates the operations to the wrapped component and adds some additional behavior.
- Concrete Decorators: These implement the Decorator class and add responsibilities.
UML Representation
Here is a basic representation of the Decorator Pattern:
+------------------+
| Component |
+------------------+
|
+------------------+
| ConcreteComponent |
+------------------+
|
+---------------+---------------+
| |
+------------------+ +------------------+
| Decorator | | ConcreteDecoratorA|
+------------------+ +------------------+
| +Component | | +Operation() |
+------------------+ +------------------+
| |
+------------------+ +------------------+
| ConcreteDecoratorB| | ConcreteDecoratorC|
+------------------+ +------------------+
| +Operation() | | +Operation() |
+------------------+ +------------------+
Implementation in Java
Let's take a look at a more tangible example to clarify how the Decorator Pattern operates in Java.
Step 1: Create the Component Interface
The first step is to define the Component
interface. This includes a core method that will be implemented by concrete components.
public interface Coffee {
String getDescription();
double cost();
}
Step 2: Implement a Concrete Component
Next, we’ll have an implementation of our Coffee
interface. This will act as the base component.
public class SimpleCoffee implements Coffee {
@Override
public String getDescription() {
return "Simple Coffee";
}
@Override
public double cost() {
return 2.00; // Base price of simple coffee
}
}
Step 3: Create the Decorator Base Class
We will now create a CoffeeDecorator
that implements the Coffee
interface and holds a reference to a Coffee
object. This allows us to expand its functionality.
public abstract class CoffeeDecorator implements Coffee {
protected Coffee decoratedCoffee;
public CoffeeDecorator(Coffee coffee) {
this.decoratedCoffee = coffee;
}
@Override
public String getDescription() {
return decoratedCoffee.getDescription();
}
@Override
public double cost() {
return decoratedCoffee.cost();
}
}
Step 4: Implement Concrete Decorators
Now we'll implement some concrete decorators—like milk and sugar—to modify the base functionality.
Milk Decorator
public class MilkDecorator extends CoffeeDecorator {
public MilkDecorator(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return decoratedCoffee.getDescription() + ", Milk";
}
@Override
public double cost() {
return decoratedCoffee.cost() + 0.50; // Add cost for milk
}
}
Sugar Decorator
public class SugarDecorator extends CoffeeDecorator {
public SugarDecorator(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return decoratedCoffee.getDescription() + ", Sugar";
}
@Override
public double cost() {
return decoratedCoffee.cost() + 0.20; // Add cost for sugar
}
}
Step 5: Testing the Decorators
Let's create a main class to put our decorators to test.
public class CoffeeShop {
public static void main(String[] args) {
Coffee coffee = new SimpleCoffee();
System.out.println(coffee.getDescription() + " $" + coffee.cost());
coffee = new MilkDecorator(coffee);
System.out.println(coffee.getDescription() + " $" + coffee.cost());
coffee = new SugarDecorator(coffee);
System.out.println(coffee.getDescription() + " $" + coffee.cost());
}
}
Output
When you run the above code, here's what you would expect:
Simple Coffee $2.0
Simple Coffee, Milk $2.5
Simple Coffee, Milk, Sugar $2.7
As we can see, the Decorator Pattern helps us build complex functionalities from simpler components just by stacking decorators, which is cleaner than creating multiple subclasses for different coffee types.
The Bottom Line
The Decorator Pattern is a fundamental tool in any Java developer's toolkit. It allows for more manageable, flexible, and maintainable code. By promoting the separation of concerns, you can avoid overcomplication and create a system that's easy to extend.
For further reading on Java design patterns, check out Refactoring Guru's Design Patterns. You can also look into the Java Design Patterns official documentation.
Implement these patterns wisely, and they will immensely enhance your programming skills and software design. Happy coding!
Checkout our other articles