Mastering JSON Parsing: Avoiding Unknown Properties in Java
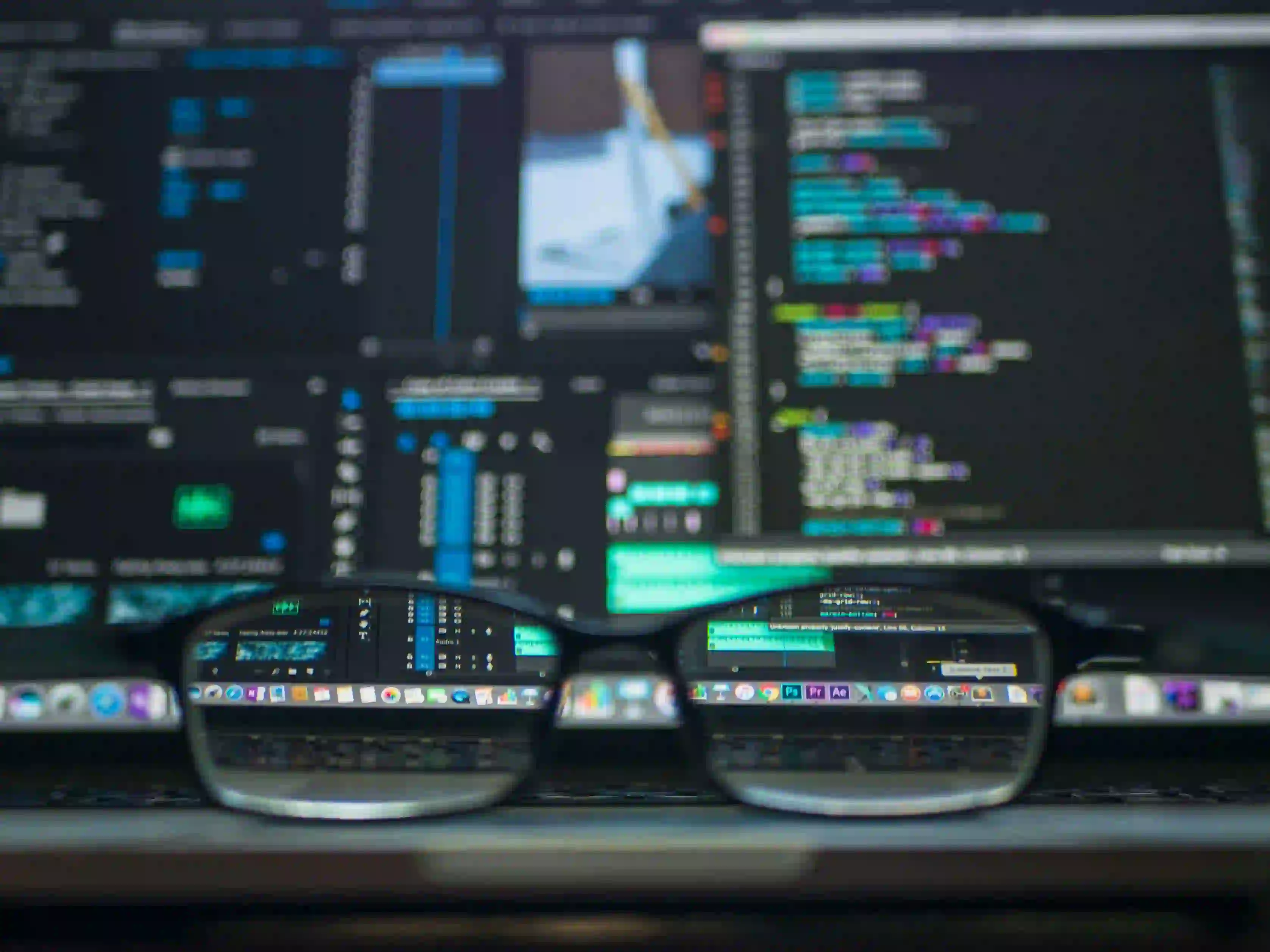
Mastering JSON Parsing: Avoiding Unknown Properties in Java
JSON (JavaScript Object Notation) is widely recognized as a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. In Java, parsing JSON is a common task, especially when building web applications or consuming APIs. However, one common issue developers face is handling unknown properties in JSON objects.
In this blog post, we will explore effective strategies to manage unknown properties when parsing JSON in Java, focusing on popular libraries like Jackson and Gson. We’ll also include practical code snippets to illustrate the solutions clearly.
Understanding JSON Structure
Before diving into parsing strategies, let's briefly discuss JSON structure. JSON is based on key-value pairs. Here's a simple example:
{
"name": "John Doe",
"age": 30,
"unknownProperty": "This can cause issues"
}
In this example, suppose you have a Java class that maps to this JSON structure:
public class Person {
private String name;
private int age;
// Getters and setters
}
If you attempt to deserialize the JSON into the Person
class using Jackson or Gson, the unknown property ("unknownProperty") will lead to parsing errors or exceptions. Let's explore ways to resolve this challenge.
Using Jackson for JSON Parsing
Jackson is a powerful library for processing JSON in Java. It provides various annotations and configuration options that can help you handle unknown properties. Here’s how to do it.
Configuring Jackson to Ignore Unknown Properties
One straightforward method to handle unknown properties is by configuring Jackson to ignore them. This ensures that any properties not defined in the Java class will be disregarded during deserialization. Here's how you can do that:
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.databind.ObjectMapper;
@JsonIgnoreProperties(ignoreUnknown = true)
public class Person {
private String name;
private int age;
// Getters and setters
}
Now, let's see how you can deserialize JSON with this setting:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) {
String jsonString = "{\"name\":\"John Doe\",\"age\":30,\"unknownProperty\":\"This can cause issues\"}";
ObjectMapper objectMapper = new ObjectMapper();
try {
Person person = objectMapper.readValue(jsonString, Person.class);
System.out.println("Name: " + person.getName());
System.out.println("Age: " + person.getAge());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
- The
@JsonIgnoreProperties(ignoreUnknown = true)
annotation tells Jackson to skip any properties that do not match the fields in thePerson
class during deserialization. - In the example above,
unknownProperty
will be ignored, and the program will successfully create aPerson
object containing only the valid fields.
Why Ignore Unknown Properties?
Ignoring unknown properties can be especially useful when dealing with dynamic JSON structures, such as responses from APIs that may change over time or include extra data. By implementing this strategy, your application becomes more resilient to unexpected changes.
Using Gson for JSON Parsing
Gson is another popular library for JSON processing in Java. Much like Jackson, Gson also provides mechanisms to deal with unknown properties.
Configuring Gson to Ignore Unknown Properties
To instruct Gson to ignore unknown fields, you can set its configuration as follows:
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class Person {
private String name;
private int age;
// Getters and setters
}
public class JsonExample {
public static void main(String[] args) {
String jsonString = "{\"name\":\"John Doe\",\"age\":30,\"unknownProperty\":\"This can cause issues\"}";
Gson gson = new GsonBuilder().setLenient().create();
Person person = gson.fromJson(jsonString, Person.class);
System.out.println("Name: " + person.getName());
System.out.println("Age: " + person.getAge());
}
}
Explanation
- The
GsonBuilder().setLenient()
method allows Gson to ignore unknown properties when parsing JSON. - Similarly, unknown properties will not affect the deserialization of the
Person
object, resulting in a clean output.
Why Choose Gson?
Gson is an excellent choice for developers looking for simplicity and ease of use. Its configuration style is straightforward, making it a quick solution for ignoring unknown properties without extensive setup.
Bringing It All Together
JSON parsing is an essential skill for any Java developer. Handling unknown properties gracefully can prevent runtime errors and enhance application robustness. Whether you prefer Jackson or Gson, both libraries offer effective ways to ignore unknown fields during parsing.
By applying the techniques discussed in this blog, you can confidently work with JSON data, ensuring that your code remains clean and maintainable.
References
Master JSON parsing in Java, avoid pitfalls, and create resilient applications. Happy coding!