Debugging Java's Chain of Responsibility: Common Pitfalls
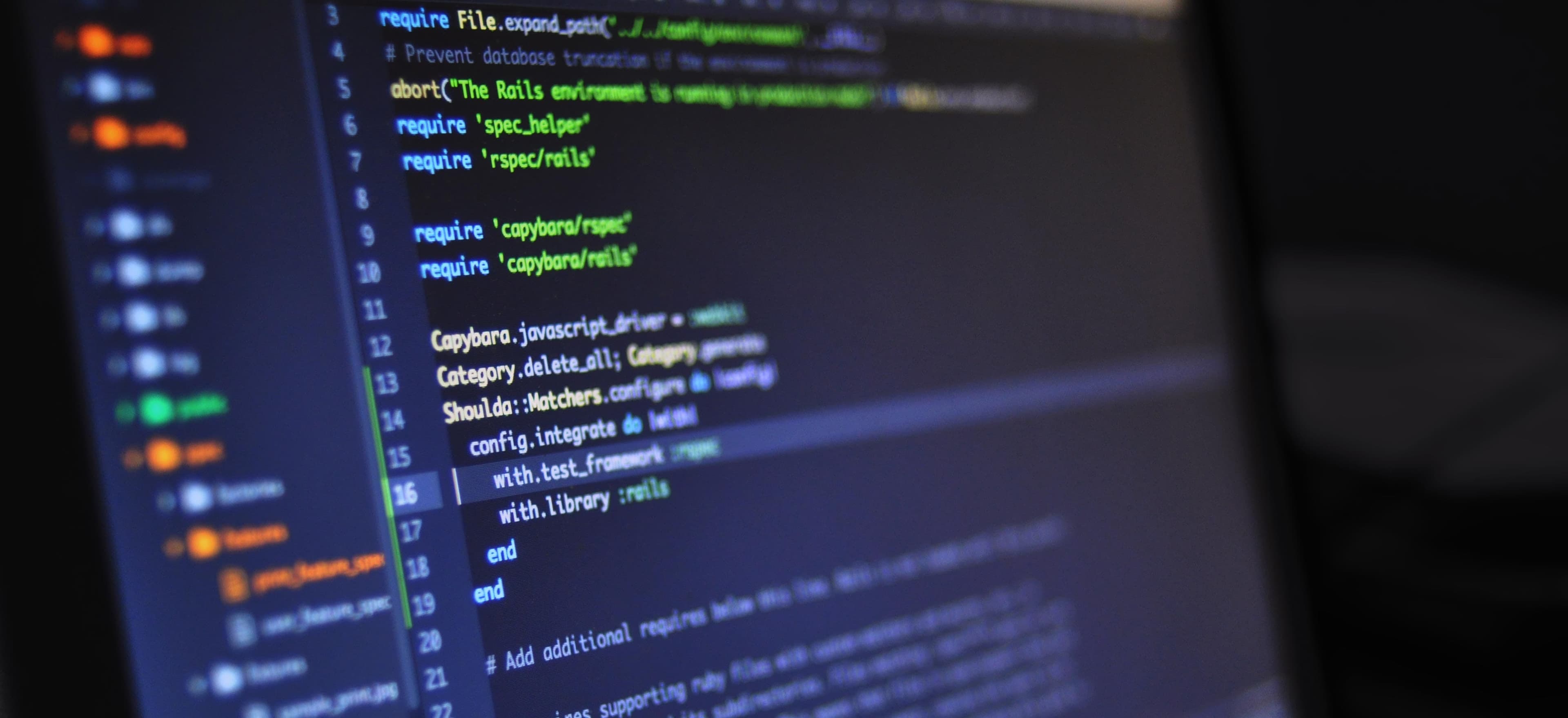
- Published on
Debugging Java's Chain of Responsibility: Common Pitfalls
The Chain of Responsibility (CoR) is a behavioral design pattern that allows an object to pass a request along a chain of potential handlers until one of them either handles the request or the end of the chain is reached. This pattern is incredibly useful in scenarios like GUI event handling, middleware in servers, or processing multiple filters in frameworks.
However, like any programming paradigm, using CoR can lead to some common pitfalls, especially during debugging. This blog post will walk you through these pitfalls, provide practical Java examples, and share strategies to overcome them.
Understanding the Chain of Responsibility Pattern
Before diving into the debugging challenges, let’s clarify how the CoR pattern operates.
Basic Structure
At its core, the chain of responsibility consists of:
- Handler Interface: Defines the method to handle requests.
- Concrete Handlers: Implements the handling logic and forwards the request along the chain if not processed.
- Client: Initiates requests and holds the first handler in the chain.
Here's a sample implementation:
interface Handler {
void setNext(Handler handler);
void handleRequest(String request);
}
abstract class AbstractHandler implements Handler {
private Handler nextHandler;
@Override
public void setNext(Handler handler) {
this.nextHandler = handler;
}
protected void passToNext(String request) {
if (nextHandler != null) {
nextHandler.handleRequest(request);
}
}
}
In this snippet, the Handler
interface defines the contract for all handlers, and the AbstractHandler
provides the functionality to set the next handler in the chain and pass requests along it.
Concrete Handlers
Now, let’s create a couple of concrete handlers:
class ConcreteHandlerA extends AbstractHandler {
@Override
public void handleRequest(String request) {
if (request.equals("A")) {
System.out.println("Handler A processed request: " + request);
} else {
passToNext(request);
}
}
}
class ConcreteHandlerB extends AbstractHandler {
@Override
public void handleRequest(String request) {
if (request.equals("B")) {
System.out.println("Handler B processed request: " + request);
} else {
passToNext(request);
}
}
}
Here, ConcreteHandlerA
and ConcreteHandlerB
handle requests based on their specific logic. If a request does not match, the handlers pass the request to the next handler in the chain.
Client Code
The client code sets up the chain and initiates the request:
public class Client {
private Handler chain;
public Client() {
// Setting up the chain: A -> B
chain = new ConcreteHandlerA();
Handler b = new ConcreteHandlerB();
chain.setNext(b);
}
public void processRequest(String request) {
chain.handleRequest(request);
}
}
Common Pitfalls in Debugging
While the Chain of Responsibility pattern can simplify request handling, it can also introduce complexity that challenges debugging. Here are some common pitfalls to watch out for.
1. Silent Failures
One of the most significant issues when implementing the CoR pattern is silent failures. If a request is not handled, the last handler in the chain just passes it along without providing feedback.
Solution: Implement logging or exceptions.
protected void passToNext(String request) {
if (nextHandler != null) {
nextHandler.handleRequest(request);
} else {
System.err.println("No handler found for request: " + request);
}
}
2. Circular Chains
Developers sometimes mistakenly create circular chains, where a handler references itself either directly or indirectly, leading to infinite loops.
Solution: Enforce a maximum depth or monitor the chain with a counter.
3. Misconfigured Chains
If the chain is misconfigured, some requests may bypass handlers incorrectly. This leads to unexpected behavior, especially when handlers have overlapping responsibilities.
Solution: Validate the chain upon initialization.
public void validateChain() {
Set<Handler> seen = new HashSet<>();
Handler current = this;
while (current != null) {
if (seen.contains(current)) {
throw new IllegalStateException("Circular reference detected!");
}
seen.add(current);
current = current.next(); // Get the next handler
}
}
4. Unexpected Request Types
Handlers often have explicit conditions under which they accept requests. If the request type changes (for example, from String to a custom Request class), a handler might fail to handle it, causing confusion.
Solution: Use polymorphism to handle different request types.
5. Lack of Unit Tests
Without proper validation through unit tests, pitfalls may go unnoticed until runtime.
Solution: Write extensive unit tests for each handler to ensure that every possible request is handled correctly.
import static org.junit.jupiter.api.Assertions.*;
class ChainOfResponsibilityTest {
@Test
void testHandlerAProcessesRequestA() {
Client client = new Client();
client.processRequest("A");
// Assertions to validate that the request is handled by A
}
@Test
void testHandlerBProcessesRequestB() {
Client client = new Client();
client.processRequest("B");
// Assertions to validate that the request is handled by B
}
@Test
void testUnprocessedRequest() {
Client client = new Client();
client.processRequest("C");
// Expected outcome of unprocessed request, maybe logged or an exception
}
}
Final Considerations
The Chain of Responsibility pattern can greatly enhance the flexibility of your Java applications. However, it also poses unique challenges. By being aware of the common pitfalls and implementing the provided solutions, you can effectively debug your CoR implementations.
For more design patterns in Java, consider exploring the Gang of Four Design Patterns or the Java Design Patterns Cheat Sheet.
Remember, while design patterns serve as valuable guidelines, the key to effective development is combining these patterns with sound coding practices and thorough testing. Happy coding!