Mastering PowerMock: Troubleshooting Static Method Mocks
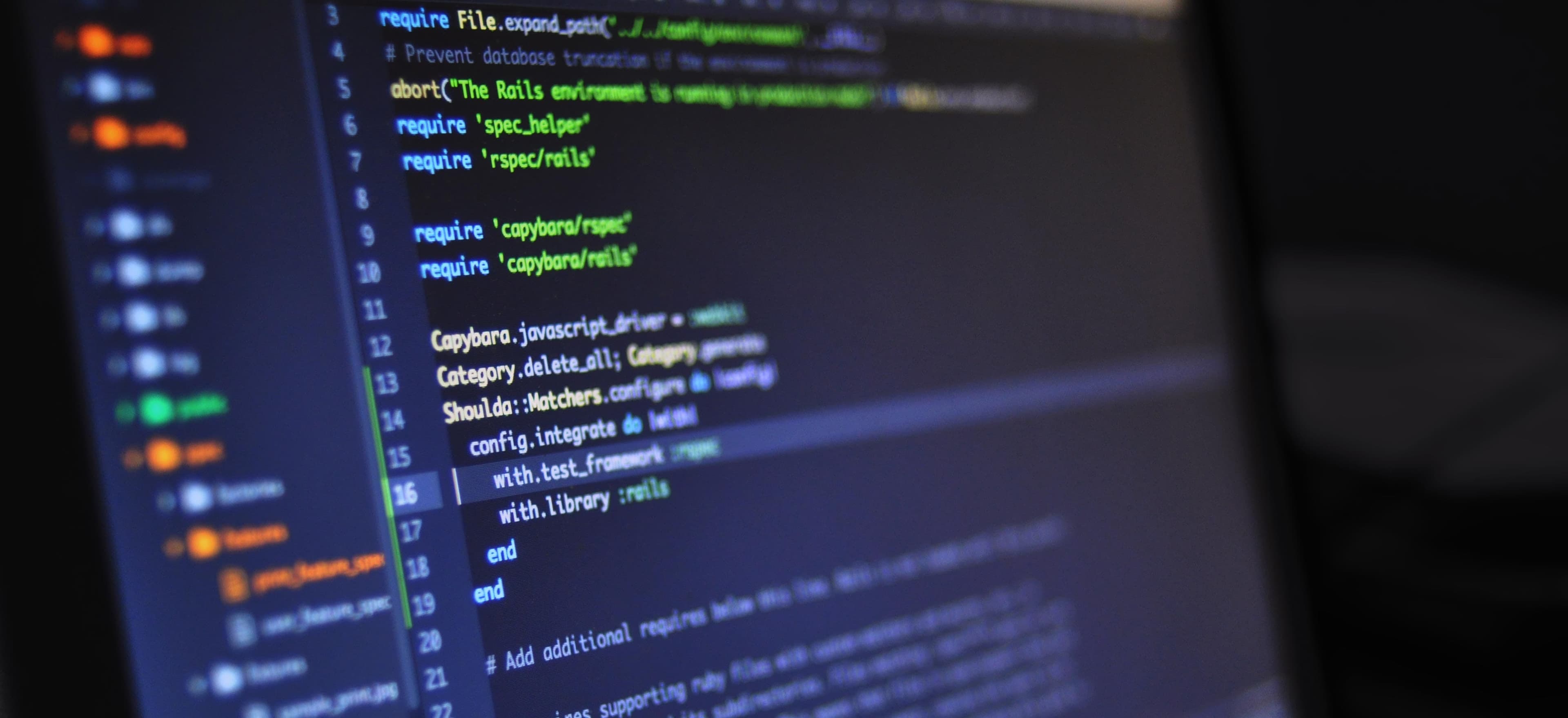
- Published on
Mastering PowerMock: Troubleshooting Static Method Mocks
Java development often involves a great deal of implementation and testing. When it comes to unit testing, mocking static methods can be particularly challenging. That's where PowerMock comes into play. In this blog post, we will delve into how to effectively use PowerMock for testing static methods, troubleshoot common issues, and improve your testing strategy.
What is PowerMock?
PowerMock is an extension of popular mocking frameworks like Mockito and EasyMock. It provides the ability to mock static methods, final classes, and constructors. While Mockito and similar frameworks excel at mocking instance methods, PowerMock can tackle the challenges presented by the above cases.
Why Mock Static Methods?
You might wonder, "Why should I mock static methods at all?" Here are a few reasons:
- Legacy Code: Often, legacy codebases use static methods extensively, making them hard to test.
- Third-Party Libraries: If your code interacts with static methods from third-party libraries, mocking is helpful for isolation during unit tests.
- Global State: Static methods can influence global state in ways that are detrimental to unit testing.
Getting Started with PowerMock
Before we dive into specific examples, we need to set up a simple Java project. PowerMock requires integration with testing frameworks such as JUnit or TestNG, along with Mockito. Here’s how to set up your Maven project.
Maven Dependency
Add the following dependencies to your pom.xml
file:
<dependencies>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>4.0.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.powermock</groupId>
<artifactId>powermock-module-junit4</artifactId>
<version>2.0.9</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.powermock</groupId>
<artifactId>powermock-api-mockito2</artifactId>
<version>2.0.9</version>
<scope>test</scope>
</dependency>
</dependencies>
Make sure to replace versions based on the latest available release. You can check the Maven Central Repository for updates.
Writing Your First Test with PowerMock
Let’s explore a simple example where we have a utility class with a static method. For illustration, consider the following code.
public class Utility {
public static String getGreeting(String name) {
return "Hello, " + name + "!";
}
}
Mocking the Static Method
To mock the getGreeting
method using PowerMock, create a test class:
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.Mockito;
import org.powermock.api.mockito.PowerMockito;
import org.powermock.core.classloader.annotations.PrepareForTest;
import org.powermock.modules.junit4.PowerMockRunner;
import static org.junit.Assert.assertEquals;
@RunWith(PowerMockRunner.class)
@PrepareForTest(Utility.class)
public class UtilityTest {
@Test
public void testGetGreeting() {
PowerMockito.mockStatic(Utility.class);
Mockito.when(Utility.getGreeting("John")).thenReturn("Mocked Hello, John!");
String result = Utility.getGreeting("John");
assertEquals("Mocked Hello, John!", result);
}
}
Explanation of the Code
-
@RunWith(PowerMockRunner.class)
: This annotation tells JUnit to use PowerMock's runner, which allows us to mock static methods. -
@PrepareForTest(Utility.class)
: This prepares theUtility
class for testing, indicating that we intend to mock its static methods. -
PowerMockito.mockStatic(Utility.class)
: This call mocks all static methods of theUtility
class. -
Mockito.when(...)
: This sets up what should happen when the static method is called with specific parameters. -
assertEquals(...)
: Finally, validate that the simulated output is as expected.
Common Issues and Troubleshooting
ClassNotFoundException
You might encounter a ClassNotFoundException
when running your PowerMock tests. This typically occurs when the class is not properly prepared for test mocking. Ensure you include @PrepareForTest
on the test class and specify the static class name.
NoClassDefFoundError
This error may arise due to incompatible library versions. Ensure that the versions of Mockito, PowerMock, and JUnit you are using are compatible. Refer to the PowerMock Compatibility Matrix for more insights.
PowerMock No Longer Replaces all Static Calls
Sometimes, it appears PowerMock is not behaving as expected. Remember that if static methods are called inside constructor initialization blocks, they may not be intercepted. Instead of calling static methods inside a constructor, consider refactoring your code to use dependency injection where possible.
Best Practices for Using PowerMock
-
Minimal Use: Only use PowerMock when absolutely necessary. PowerMock can complicate tests and may slow down your test suite.
-
Refactor to Avoid Static Methods: If possible, refactor your code to use instance methods. This makes tests easier and reduces the reliance on PowerMock.
-
Keep Tests Isolated: Mock only what's necessary to keep your tests predictable and reliable.
-
Clear Documentation: Document mocked methods and test cases to clarify your test's purpose and behavior.
-
Use Alternative Frameworks: For most modern use cases, consider frameworks focused on modern Java practices, such as JUnit 5 with Mockito or alternatives that avoid the need for PowerMock.
Wrapping Up
Testing static methods in Java using PowerMock can be straightforward when you understand the framework’s capabilities and limitations. In this blog post, we covered a practical example of mocking a static method, how to troubleshoot common issues, and best practices to follow.
By utilizing PowerMock judiciously, you can enhance the effectiveness of your unit tests and improve the quality of your codebase. For more advanced techniques and updates, stay tuned to the PowerMock official documentation.
If you have any questions or insights about using PowerMock, feel free to comment below! Happy coding!
Checkout our other articles