Overcoming Common Issues with Arquillian and Open Liberty
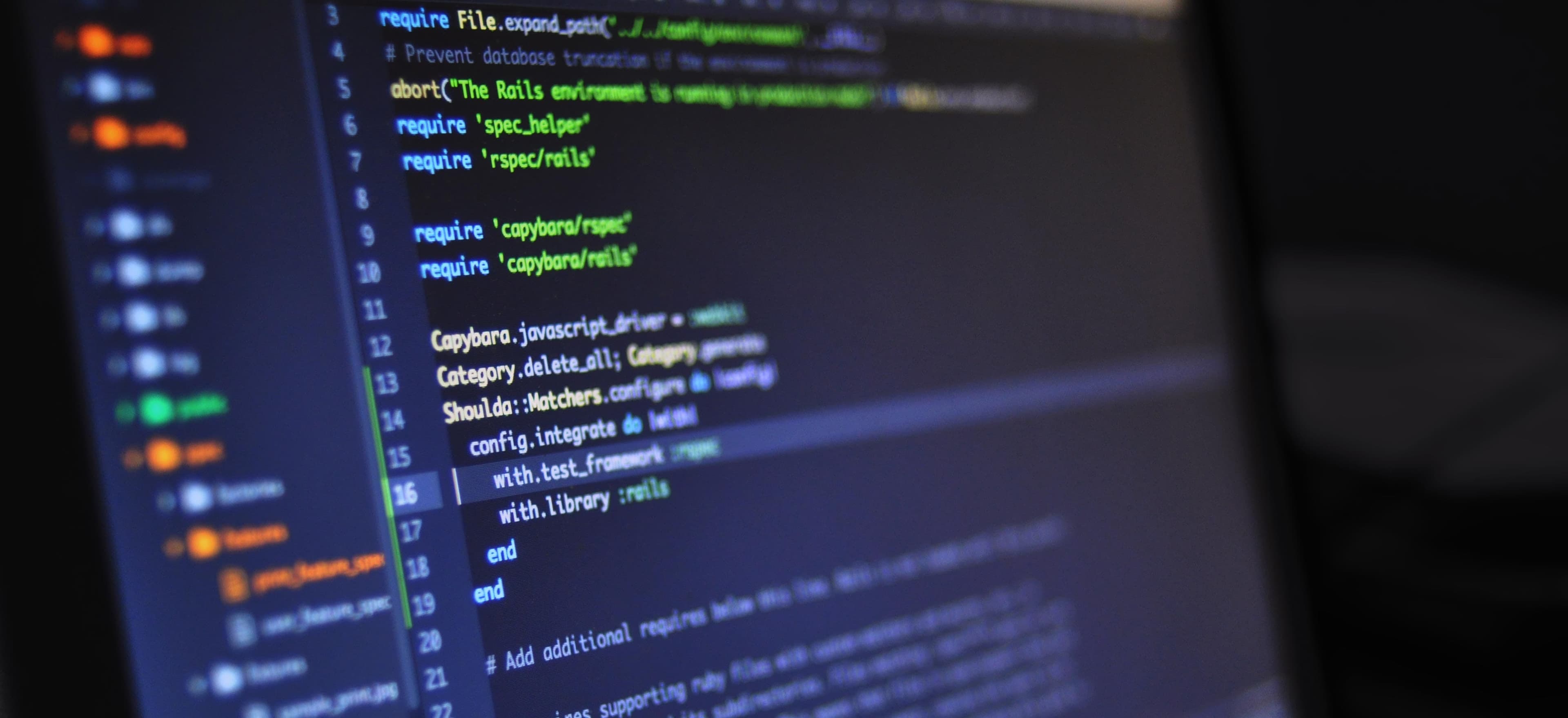
- Published on
Overcoming Common Issues with Arquillian and Open Liberty
Introduction
As Java developers working with modern microservices architectures, integrating testing frameworks efficiently is essential for delivering high-quality applications. Arquillian, a testing platform for Java, enables developers to perform integration testing easily in a real container environment. Pairing it with Open Liberty—a lightweight, cloud-native Java EE application server—further enhances the capabilities for testing enterprise applications. However, users often face common issues while getting Arquillian to work seamlessly with Open Liberty. In this post, we'll explore these challenges and uncover effective solutions.
Understanding Arquillian and Open Liberty
Before we dive into troubleshooting, it's vital to understand what Arquillian and Open Liberty bring to the table:
-
Arquillian: Arquillian simplifies the process of integration testing by managing the lifecycle of the container, allowing tests to be written as if they were unit tests, but running inside or against a real container.
-
Open Liberty: Open Liberty is a flexible application server characterized by lightweight deployments, fast startup times, and built-in support for a range of Java EE and MicroProfile technologies.
For more on Arquillian, you can check out the official documentation here. To understand Open Liberty in greater depths, explore their official site here.
Common Issues with Arquillian and Open Liberty
Let's identify some common pitfalls developers might encounter when integrating Arquillian with Open Liberty, as well as practical solutions to resolve these issues.
1. Configuration Issues
Symptoms
Initial attempts to run Arquillian tests often lead to configuration errors that halt execution. Typical messages might indicate that the necessary resources are not available.
Solution
Ensure that your arquillian.xml
file is correctly configured for your Open Liberty runtime. Here is an example configuration:
<arquillian xmlns="http://jboss.org/schema/arquillian_1_0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://jboss.org/schema/arquillian_1_0
http://jboss.org/schema/arquillian_1_0.xsd">
<test>
<container>liberty</container>
</test>
</arquillian>
Why This Matters: Specifying the right container as liberty
tells Arquillian to use Open Liberty for running the tests. A more detailed configuration can specify other properties, such as context root or additional runtime properties.
2. Dependency Resolution
Symptoms
You might experience issues with unresolved dependencies, leading to ClassNotFoundException
or NoClassDefFoundError
.
Solution
Make sure to include all necessary dependencies in your pom.xml
. Here’s a quick checklist of common dependencies required for Arquillian with Open Liberty:
<dependency>
<groupId>org.jboss.arquillian.junit</groupId>
<artifactId>arquillian-junit-container</artifactId>
<version>${arquillian.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.jboss.arquillian.container</groupId>
<artifactId>arquillian-wildfly-embedded-api</artifactId>
<version>${arquillian.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>io.openliberty</groupId>
<artifactId>wlp-arquillian-core</artifactId>
<version>${openliberty.version}</version>
<scope>test</scope>
</dependency>
Why This Matters: Each component serves a specific purpose, ensuring that Arquillian can manage the lifecycle properly and access the necessary classes at runtime.
3. Port Configuration
Symptoms
Sometimes, you may find that your tests are not connecting to the Open Liberty instance because the expected ports are not available or already in use.
Solution
Check the server.xml
configuration file for your Open Liberty server. Ensure that the HTTP and HTTPS ports are set correctly and are not conflicting with other services on your machine.
<httpEndpoint id="defaultHttpEndpoint" host="*" port="9080" />
<httpsEndpoint id="defaultHttpsEndpoint" host="*" port="9443" />
You may also want to configure the Arquillian testing environment to specify the expected ports in your test class:
@Deployment
public static Archive<?> createTestArchive() {
return ShrinkWrap.create(WebArchive.class)
.addClass(MyService.class)
// other resources
.addAsWebInfResource(EmptyAsset.INSTANCE, "beans.xml")
.addAsManifestResource(EmptyAsset.INSTANCE, "META-INF/MANIFEST.MF")
.addAsManifestResource(new StringAsset("Ports:\n9080\n9443\n"), "ports.properties");
}
Why This Matters: Customizing the ports ensures that tests can be conducted without port conflicts, allowing for smoother execution.
4. Context Initialization
Symptoms
Another prevalent issue manifests as deployment failures, where the application context fails to initialize or is unreachable during testing.
Solution
Review your @Before
methods or any application startup logic. Sometimes, dependencies may not be properly injected or managed. For example:
@PersistenceContext(unitName = "myPersistenceUnit")
private EntityManager em;
@Before
public void setUp() {
// Ensure an EntityManager is available
assertNotNull(em);
}
Why This Matters: Making sure the required contexts, such as the entity manager, are correctly initialized creates a stable environment for your tests.
5. Monitoring and Logging
Symptoms
If tests pass but the application behaves unexpectedly during integration, logging information may be sparse or unavailable.
Solution
Leverage Open Liberty's logging capabilities to gather insights into the deployed application. You can adjust the logging level in server.xml
as follows:
<loggingTrace traceSpecification="*=info:com.my.package*=debug" />
Then, check the logs during tests:
@Test
public void testMyService() {
// Log before and after invocation
Logger logger = Logger.getLogger(MyServiceTest.class.getName());
logger.info("Invoking myService");
MyService myService = new MyService();
assertEquals("Expected Result", myService.callService());
logger.info("Finished invoking myService");
}
Why This Matters: Monitoring your application through logs provides essential insights that can help troubleshoot issues as they occur.
Closing Remarks
Integrating Arquillian with Open Liberty can elevate your Java application testing to new heights. By addressing common configuration issues, dependency resolutions, port conflicts, context initializations, and logging practices, you can create a robust testing framework that ensures your application runs smoothly in production.
For a more detailed understanding and further reading, consult the official documentation for Arquillian and Open Liberty.
By leveraging these insights, you can overcome the typical challenges associated with using Arquillian and Open Liberty together, thereby optimizing your development workflow and enhancing your team's efficiency. Happy testing!
Checkout our other articles