Streamlining Recursive File System Traversal for Efficiency
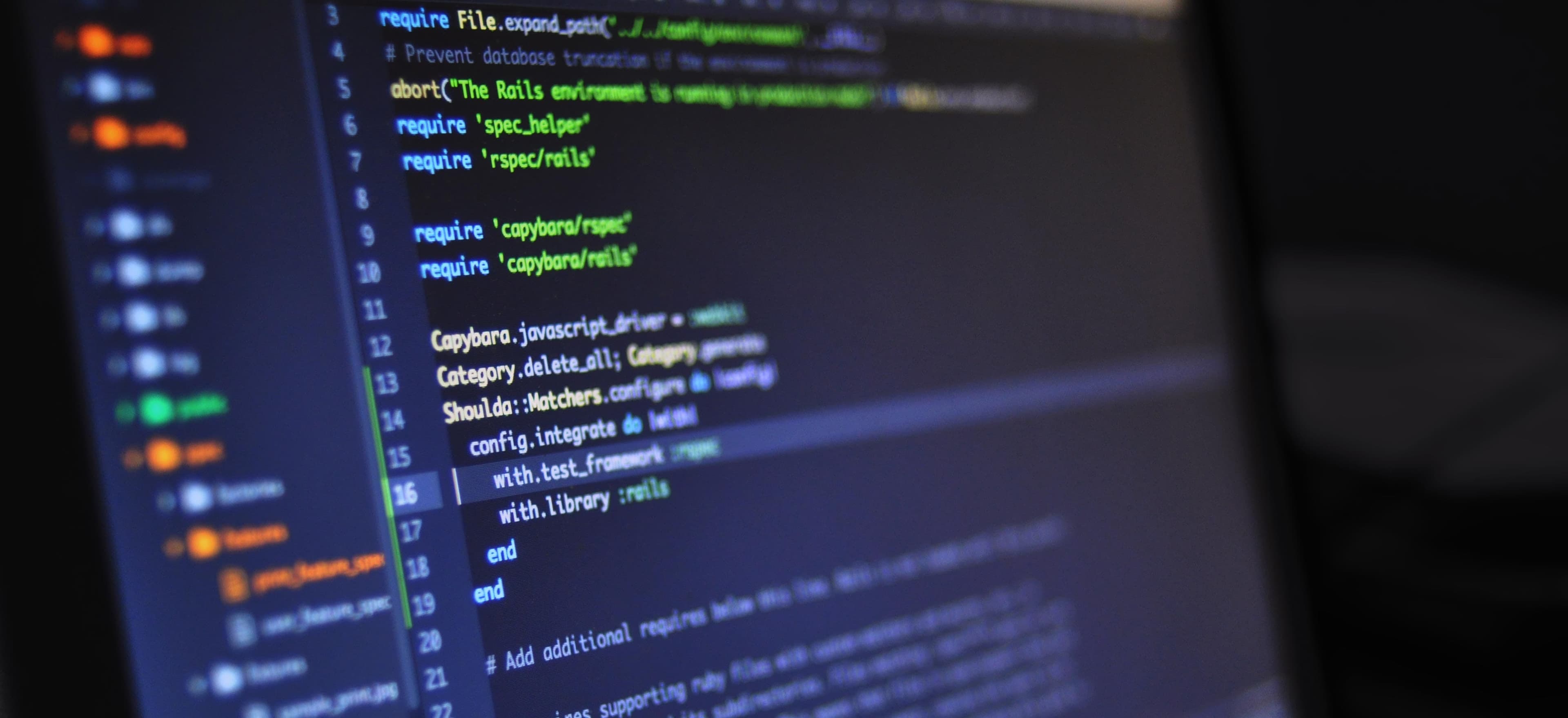
- Published on
Streamlining Recursive File System Traversal for Efficiency in Java
File system traversal is an essential operation in many applications, ranging from backup utilities to search engines. However, traversing a file system recursively poses challenges, especially in terms of performance and memory consumption. In this blog post, we will explore how to streamline recursive file system traversal in Java with a focus on efficiency.
Understanding Recursive Traversal
Recursive traversal involves visiting each file and directory within a file system hierarchy. In its simplest form, a recursive function calls itself with a new argument until it reaches a base case. While recursion provides a clean and elegant solution to navigate file systems, it can lead to significant overhead due to function call stacks and memory usage, particularly with deeply nested directories.
Why Choose Java for File Traversal?
Java is a powerful language that excels in cross-platform applications. Its rich set of libraries allows for easy manipulation of file systems. The Java NIO package, introduced in Java 7, offers a more modern, efficient way of handling file I/O operations compared to the traditional Java I/O package.
Exploring File Traversal Techniques
1. Traditional Recursive Approach
Let’s start with a basic recursive method to traverse a directory structure:
import java.io.File;
public class RecursiveTraversal {
public static void main(String[] args) {
File rootDirectory = new File("path/to/directory");
traverseDirectory(rootDirectory);
}
public static void traverseDirectory(File directory) {
if (directory.isDirectory()) {
File[] files = directory.listFiles();
for (File file : files) {
System.out.println(file.getAbsolutePath());
if (file.isDirectory()) {
traverseDirectory(file); // Recursive call
}
}
}
}
}
Commentary on the Code
- Base Case: The method checks if the current file is a directory. If not, it simply prints the file path.
- Recursive Call: If it finds a directory, it recursively calls itself to explore its contents.
While this method is straightforward, it suffers from downsides:
- Stack Overflow: For deeply nested directories, this approach can lead to a
StackOverflowError
due to too many recursive calls. - Performance: Each recursive call adds overhead to the call stack and may lead to slower performance.
2. Iterative Approach Using Stack
To avoid the pitfalls of recursion, we can use an iterative method with a stack. This approach manually manages our traversal, improving memory efficiency.
import java.io.File;
import java.util.Stack;
public class IterativeTraversal {
public static void main(String[] args) {
File rootDirectory = new File("path/to/directory");
traverseDirectory(rootDirectory);
}
public static void traverseDirectory(File root) {
Stack<File> stack = new Stack<>();
stack.push(root);
while (!stack.isEmpty()) {
File current = stack.pop();
System.out.println(current.getAbsolutePath());
if (current.isDirectory()) {
File[] files = current.listFiles();
if (files != null) {
for (File file : files) {
stack.push(file); // Push all files to the stack
}
}
}
}
}
}
Advantages of the Iterative Approach
- No Stack Overflow: With a stack data structure, we prevent the risk of stack overflow that can occur with deep recursion.
- Performance: The iterative approach often yields better performance in practice, especially with large directories.
3. Java NIO for Enhanced File Traversal
The Java NIO (New I/O) provides an even more efficient way to traverse file systems using the Files
and Path
classes.
import java.io.IOException;
import java.nio.file.DirectoryStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class NIOTraversal {
public static void main(String[] args) {
Path rootDirectory = Paths.get("path/to/directory");
try {
traverseDirectory(rootDirectory);
} catch (IOException e) {
e.printStackTrace();
}
}
public static void traverseDirectory(Path dir) throws IOException {
try (DirectoryStream<Path> stream = Files.newDirectoryStream(dir)) {
for (Path entry : stream) {
System.out.println(entry.toAbsolutePath());
if (Files.isDirectory(entry)) {
traverseDirectory(entry); // Recursive call for directories
}
}
}
}
}
Benefits of Using Java NIO
- Resource Management: The
DirectoryStream
handles resources efficiently, automatically closing streams to prevent memory leaks. - Improved Performance: NIO is optimized for high-performing I/O operations, making it suitable for dealing with large directory structures.
Best Practices for File System Traversal
1. Use NIO When Possible
Whenever possible, leverage the Java NIO package for file operations. It is designed for high performance and tuned for modern hardware.
2. Limit Depth or Use Filters
To prevent excessive memory usage, consider limiting the depth of traversal or using filters to skip unnecessary files (e.g., hidden files).
public static void traverseDirectory(Path dir, int maxDepth) throws IOException {
if (maxDepth < 0) return; // Base case for limiting depth
try (DirectoryStream<Path> stream = Files.newDirectoryStream(dir)) {
for (Path entry : stream) {
System.out.println(entry.toAbsolutePath());
if (Files.isDirectory(entry)) {
traverseDirectory(entry, maxDepth - 1); // Pass reduced depth
}
}
}
}
3. Use Modern Java Features
Utilize Java 8 streams and lambda expressions for cleaner, more readable code:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.stream.Stream;
public class StreamTraversal {
public static void main(String[] args) {
Path rootDirectory = Paths.get("path/to/directory");
try (Stream<Path> paths = Files.walk(rootDirectory)) {
paths.forEach(System.out::println); // Print all file paths
} catch (IOException e) {
e.printStackTrace();
}
}
}
Closing the Chapter
In this blog post, we have explored several approaches to recursive file system traversal in Java, focusing on efficiency and performance. From traditional recursion to iterative approaches and modern NIO capabilities, there are many ways to achieve effective file traversal. Leveraging these methods can lead to smoother applications and improved user experiences when dealing with file systems.
By adopting the best practices outlined, you can optimize your file traversal strategies, making your Java applications more robust and efficient. Choose the method that best fits your needs and remember to continuously analyze and improve your file system operations as new updates and techniques become available.
For further reading on Java NIO and file handling in Java, check out the official Oracle documentation:
Happy coding!
Checkout our other articles