Why Java 6, 8, and 9 Users Need a Unified API Solution
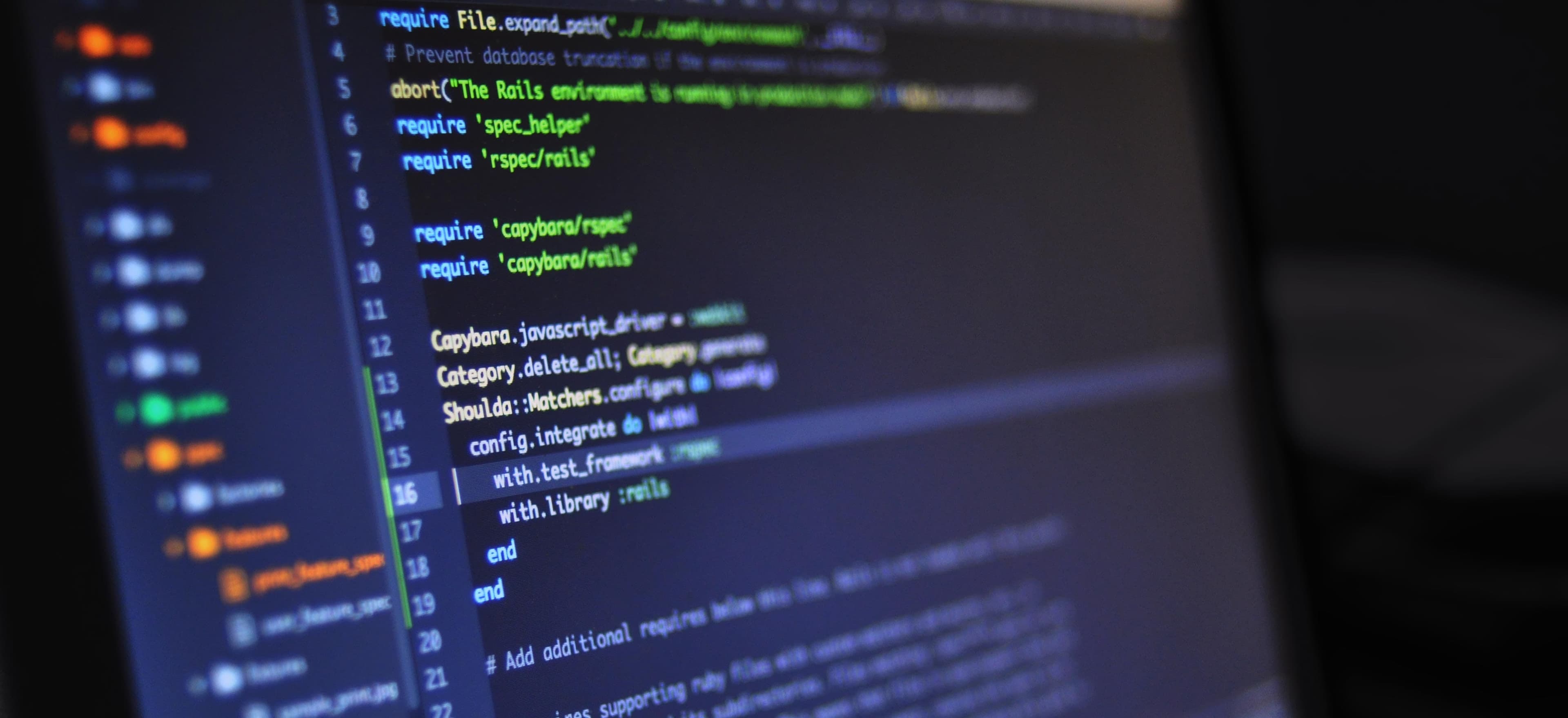
- Published on
Why Java 6, 8, and 9 Users Need a Unified API Solution
Java continues to evolve rapidly, and with these evolutions come significant changes to its APIs. As users navigate different versions—Java 6, 8, and 9—they may find themselves juggling multiple APIs that don't always align. This disparity can lead to inefficiencies, increased development time, and a steeper learning curve for new programmers. In this article, we will delve into the reasons why Java 6, 8, and 9 users need a unified API solution, exploring the complexities involved and the advantages such an approach would bring.
Understanding the Version Differences
Evolution of Java APIs
The journey from Java 6 to Java 9 introduced considerable upgrades and reworked APIs. Java 6 focused on performance enhancements and introduced important well-known APIs like the Java Compiler API and Scripting API. Conversely, Java 8 was a revolutionary release with the introduction of Lambda Expressions, Streams, and Optional—features that significantly changed how developers approach data manipulation.
Java 9, however, introduced the Java Platform Module System (JPMS) and a host of other refinements enhancing modular programming, making it easier to manage large codebases.
Each successive version brought forward beneficial features, but companies, teams, and individual developers often find themselves maintaining legacy code in older versions like Java 6, while trying to leverage new functionalities from Java 8 or the modular capabilities of Java 9.
Fragmented Ecosystem Challenges
This fragmented ecosystem creates multiple challenges:
-
Compatibility Issues: Older code may not work seamlessly with new features. For instance, developers wanting to use streams from Java 8 in a Java 6 project might hit compatibility roadblocks.
-
Learning Curve: New team members often must spend time learning various APIs for different versions, which can hinder productivity.
-
Code Maintenance: Maintaining multiple versions of a codebase increases the complexity of updates and enhancements.
What is a Unified API Solution?
Definition and Importance
A unified API solution seeks to provide a cohesive interface that spans multiple Java versions. It allows developers to abstract the differences, enabling them to leverage the full potential of Java without having to wrestle with compatibility issues. Essentially, it enables the seamless integration of diverse features in a way that feels holistic and unified.
Here are some key benefits of implementing a unified API solution:
- Simplified Development: Developers can focus on building features without needing to worry about which version of the API to use.
- Consistent Design Patterns: A unified approach promotes the adoption of design patterns that enhance readability and maintainability.
- Enhanced Collaboration: Team members can work on the same codebase without diving into version-specific quirks.
Key Advantages of a Unified API
1. Streamlined Codebase Management
With a unified API, developers are less likely to encounter fragmented code as they work on different modules of a project. For instance, instead of having isolated libraries for Java 6 and 8, a unified API allows access to unified libraries that streamline implementation.
Here's a simple illustration:
public class UnifiedAPIExample {
private List<String> items;
public UnifiedAPIExample() {
// Using Java 8 Stream API for consistency
this.items = new ArrayList<>(Arrays.asList("Item1", "Item2", "Item3"));
}
public List<String> getUpperCaseItems() {
return items.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
}
}
Commentary:
This example utilizes Java 8's Stream API to process a list, allowing developers to adopt modern practices even while maintaining legacy systems. The clean, functional style promotes better readability, ensuring that the code remains understandable regardless of team members' experiences with differing Java versions.
2. Cross-Version Compatibility
Unified APIs enable smoother transitions between Java versions. For developers still reliant on Java 6, the new libraries can provide minimal interfaces and allow for legacy support. By maintaining backward compatibility, it offers a bridge to the latest features without necessitating immediate system-wide updates.
3. Community Support and Documentation
A unified API often leads to a more engaged developer community. When everyone depends on the same set of libraries and tools, documentation grows richer and community support becomes more robust.
Consider checking out resources like Baeldung for Java 8 introductions or Javadoc for the official Java API documentation which exemplifies the differences in various Java versions.
Implementing a Unified API Solution
Strategy for Implementation
To implement a unified API solution, consider the following strategies:
-
Adopt Modern Libraries: Embrace libraries that prioritize backward compatibility while implementing new functionalities. Libraries like Apache Commons provide extensive tools that work smoothly across various Java versions.
-
Encapsulate Legacy Code: Encapsulate older APIs within a new unified interface. This promotes separation of concerns and allows you to migrate legacy code incrementally.
-
Version Control and Testing: Maintain version controls and ensure comprehensive automated testing across different Java versions to catch any discrepancies early.
@Test
public void testGetUpperCaseItems() {
UnifiedAPIExample api = new UnifiedAPIExample();
List<String> result = api.getUpperCaseItems();
assertEquals(Arrays.asList("ITEM1", "ITEM2", "ITEM3"), result);
}
Commentary:
This test checks the functionality of getUpperCaseItems()
. The unified method abstracts all the version-specific differences, allowing developers to focus on validating behavior rather than contending with version variances.
The Closing Argument
The landscape of Java version compatibility entails numerous challenges, especially for those using Java 6, 8, or 9. A unified API solution presents valuable opportunities to streamline development, enhance productivity, and facilitate cohesive collaboration among teams. By embracing a unified approach, developers can work more efficiently, ensuring they leverage the strengths of each version while maintaining compatibility with their legacy systems.
Unified APIs are not just about avoiding challenges; they are about enabling innovation. Each new Java version comes with powerful features designed to improve performance, scalability, and developer productivity. As we carry forward the evolution of Java, prioritizing a unified API solution is not merely beneficial; it's essential for modern software development.
For further reading on Java API updates and understanding what's new, consider visiting Oracle's official Java documentation, which provides comprehensive insights into the changes and how you can leverage them for your projects.
Checkout our other articles