Avoiding Pitfalls in Continuous Delivery with GitHub Commits
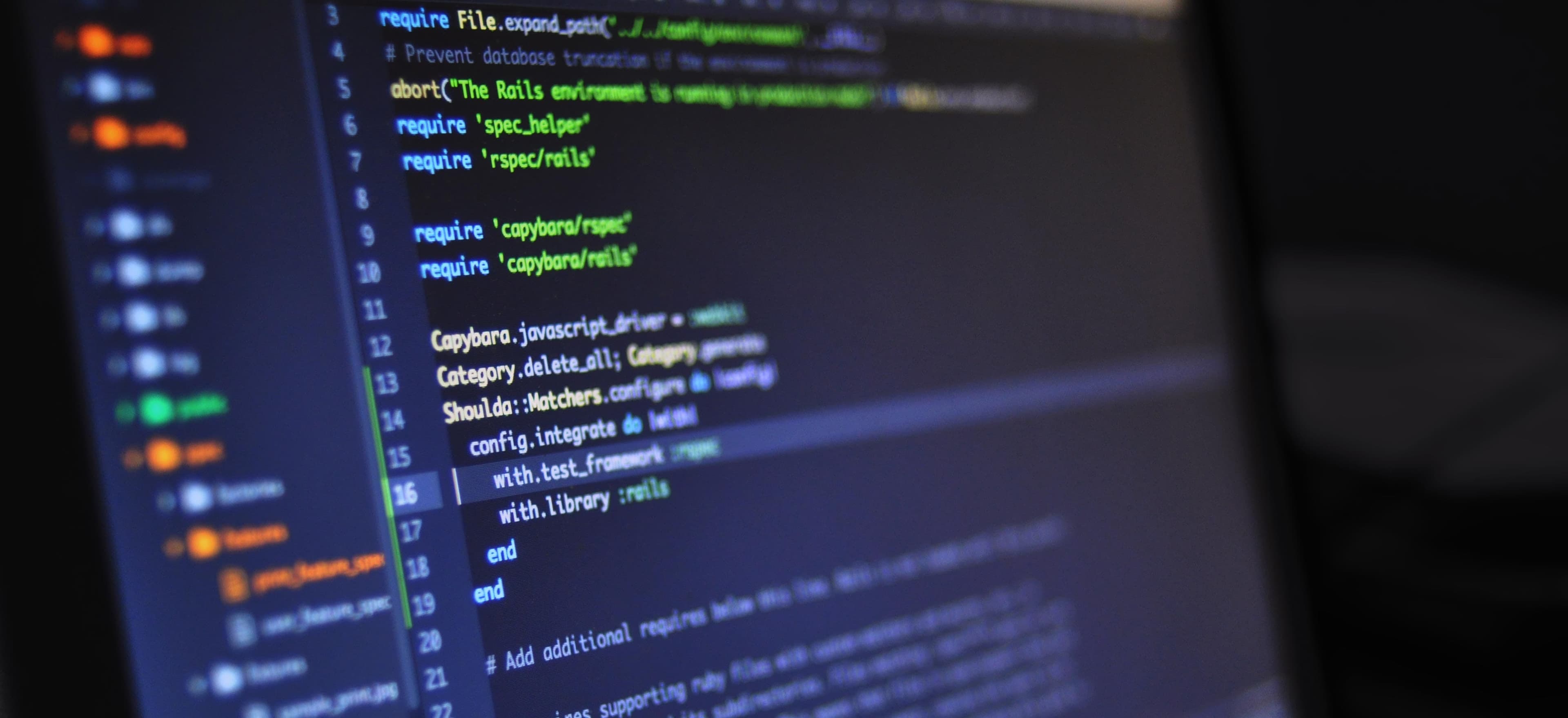
- Published on
Avoiding Pitfalls in Continuous Delivery with GitHub Commits
Continuous Delivery (CD) is a key practice in modern software development, enabling teams to deploy updates swiftly and effectively. However, as the frequency of deployments increases, so do the risks. Utilizing GitHub for version control is a common approach, and understanding how to effectively manage your commits is essential to avoiding pitfalls in this process.
In this blog post, we will explore best practices for managing commits in Continuous Delivery, highlight common pitfalls, and provide essential code snippets and commentary to help streamline your development workflow.
What is Continuous Delivery?
Continuous Delivery involves the practice of automatically preparing code changes for release to production. The goal is to ensure that software can be released reliably at any time, with minimal lead time and reduced risk. By consistently following rigorous automated testing and integration processes, CD transforms the deployment pipeline into a nearly frictionless experience.
Why Use GitHub for Continuous Delivery?
GitHub is more than a version control system; it offers a rich ecosystem for collaboration, CI/CD tools, and community-driven enhancements. Its features, including branches, pull requests, and integrations with continuous integration tools such as GitHub Actions, allow developers to maintain code quality while also enhancing team productivity.
Common Pitfalls in Continuous Delivery
When implementing Continuous Delivery with GitHub, several pitfalls can affect the workflow and integrity of the code. Here are some of the most common ones:
- Large, Multi-Purpose Commits: Committing vast changes can complicate debugging and understanding code history.
- Inconsistent Commit Messages: Commit messages serve not only as documentation but also as change logs. Inconsistencies can hinder collaboration.
- Ignoring Code Reviews: Skipping or rushing over code review processes can lead to undetected bugs and reduce overall code quality.
- Not Leveraging Branches: Not using feature branches correctly can congest the master branch and lead to conflicts.
- Neglecting Automated Tests: Bypassing tests can result in unintentional bugs being deployed to production.
Best Practices for Managing GitHub Commits
To circumvent these pitfalls, adhere to the following best practices:
1. Commit Early and Often
Frequent commits allow you to break down changes into manageable pieces, making it easier to identify when a bug was introduced. Instead of a massive commit at the end of a feature development, aim for smaller, logical commits.
git add file1.js
git commit -m "Add user authentication logic"
Here, the -m
flag allows you to write a concise and clear message. Each commit should represent a logical change in the codebase. This practice streamlines collaboration and makes tracking change history intuitive.
2. Write Meaningful Commit Messages
Commit messages should follow a standard format. A well-structured message can save time during debugging and code review.
<type>(<scope>): <subject>
<body>
- Type: e.g., feat, fix, docs, style, refactor, test, chore
- Scope: A noun describing a section of the codebase (optional)
- Subject: A brief description of the changes
Example:
feat(auth): Implement JWT authentication flow
Added JSON Web Token authentication to improve user access security. Updated login and registration endpoints.
3. Use Branches Strategically
Branching allows you to work on features independently without affecting the master branch. Use descriptive names for branches that reflect their purpose.
git checkout -b feature/user-profile
Avoid long-lived branches, as they can drift further from the base branch, leading to complicated merges later on. Aim to complete feature branches quickly and merge back into the main branch once the work is complete and tested.
4. Prioritize Code Reviews
Never underestimate the value of a second pair of eyes. Establish a process where all commits related to a feature require code review before merging. If available, tools like GitHub Pull Requests can facilitate this process.
- Review Guidelines: Ensure the review process includes checking for code style, testing coverage, and functionality.
5. Automate Testing
Automated testing is crucial for maintaining product quality. Utilize tools such as GitHub Actions to run tests automatically on push and pull request events.
Here’s a simple workflow configuration using GitHub Actions:
name: CI
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK
uses: actions/setup-java@v2
with:
java-version: '11'
- name: Run Gradle Build
run: ./gradlew build
This configuration runs tests on every push and pull request, ensuring that new changes don't break existing functionality.
The Last Word
Avoiding pitfalls in Continuous Delivery requires discipline and strategic practices in managing GitHub commits. By committing early, writing meaningful commit messages, utilizing branches effectively, prioritizing code reviews, and automating tests, teams can significantly enhance their development workflow.
Follow these guidelines as a starting point, and continue to refine your processes as your team grows. The aim of Continuous Delivery is not just rapid deployment but maintainable, high-quality code as well.
For further reading, consider looking into the following:
- Continuous Integration and Continuous Deployment
- Effective Git Commit Messages
- Introduction to GitHub Actions
Those utilizing GitHub in their Continuous Delivery workflows not only improve their deployment frequency but also enhance overall code quality and team collaboration. Happy coding!
By focusing on best practices, every developer can contribute to a successful Continuous Delivery pipeline, minimizing risk while maximizing efficiency.
Checkout our other articles