Essential Java Articles: Avoiding Common Learning Pitfalls
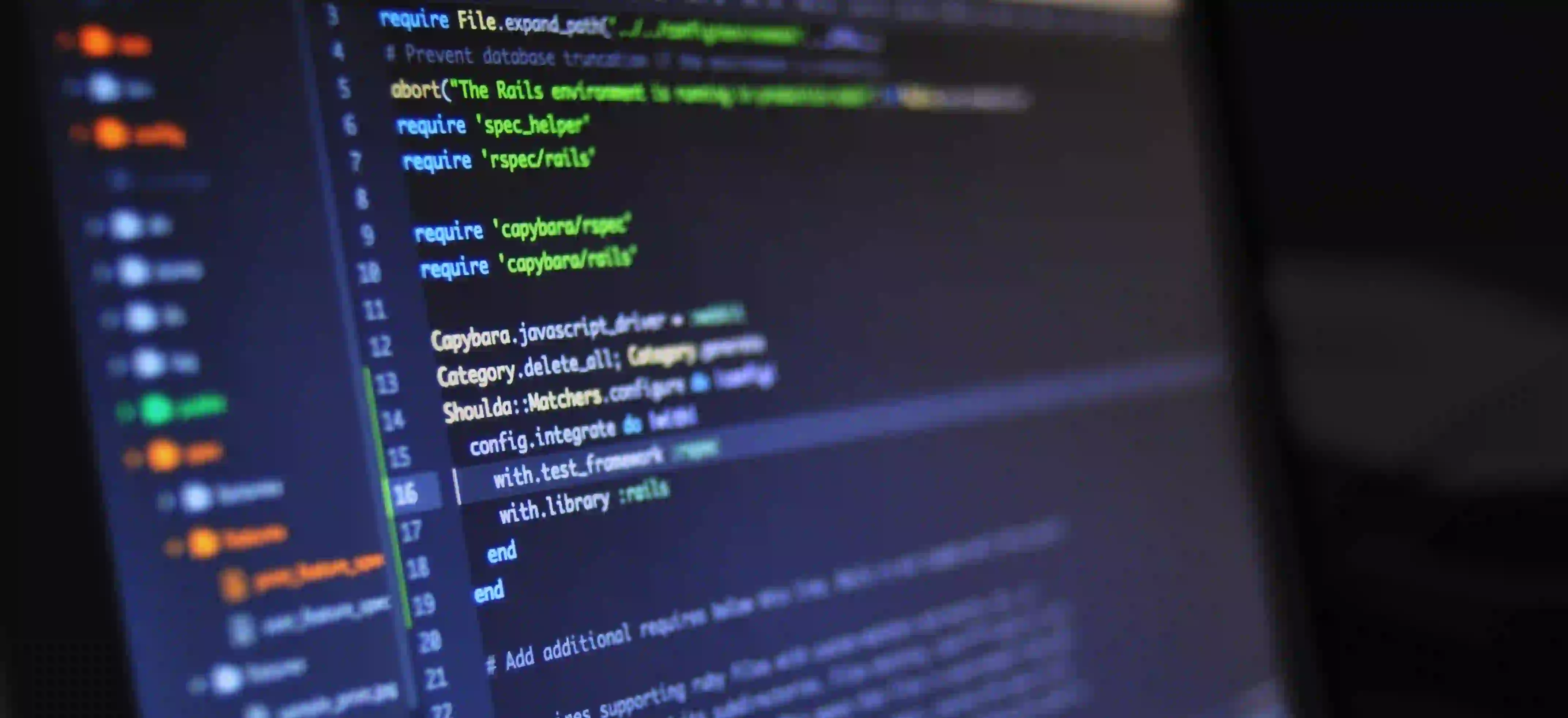
Essential Java Articles: Avoiding Common Learning Pitfalls
Java is a versatile and powerful programming language that has been the backbone of many large-scale applications. However, the journey of mastering Java can be riddled with challenges. In this blog post, we will explore some common learning pitfalls, offering guidance and resources to help you navigate the complexities of Java programming.
Understanding the Java Environment
Before diving into coding, it's essential to set up your Java environment correctly. Using the wrong version can lead to compatibility issues. Always ensure you are using the latest stable version of Java.
Example Code Snippet: Installing Java
# Update package index
sudo apt update
# Install the OpenJDK package
sudo apt install openjdk-11-jdk
# Verify installation
java -version
This straightforward installation code allows you to quickly get Java up and running on your system. The command java -version
ensures that Java is installed correctly, displaying the installed version.
Common Pitfalls in Learning Java
1. Ignoring Java Fundamentals
Newcomers often skip essential topics. Mastering Java fundamentals—like variables, data types, and control structures—is vital.
Important Concepts:
- Variables and Data Types: Understanding different data types like
int
,String
, andboolean
helps you manage data effectively. - Control Structures: Loops and conditionals are foundational. Mastering these allows you to control the flow of your programs.
Example Code Snippet: Control Structures
public class ControlStructures {
public static void main(String[] args) {
int number = 5;
// Using an if-else statement
if (number > 0) {
System.out.println("The number is positive.");
} else {
System.out.println("The number is non-positive.");
}
// Using a for loop
for (int i = 0; i < number; i++) {
System.out.println("Counting: " + i);
}
}
}
In the example above, the if-else
statement provides a clear decision-making structure, while the for
loop demonstrates repetition. These are essential tools in any programmer's kit.
2. Neglecting Object-Oriented Programming (OOP)
Java is an object-oriented language. Many learners struggle with OOP principles such as inheritance, encapsulation, and polymorphism.
Why OOP is Important
- Encapsulation helps in bundling data and methods, ensuring better data protection.
- Inheritance promotes code reuse and establishes a hierarchical relationship between classes.
- Polymorphism allows one interface to be used for a general class of actions.
Example Code Snippet: OOP Concepts
// Defining a base class
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
// Inheriting the base class
class Dog extends Animal {
@Override
void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog(); // Upcasting
myDog.sound(); // Calls the overridden method
}
}
In this code, we define an Animal
class and a Dog
subclass. The sound
method illustrates polymorphism—calling the same method name, but with different behaviors.
3. Overlooking Exception Handling
Java's robust exception handling is often dismissed. Beginners tend to ignore try
, catch
, and finally
blocks, which can lead to unhandled errors during runtime.
Why Use Exception Handling
Handling exceptions ensures your program can gracefully manage errors, maintaining a better user experience.
Example Code Snippet: Exception Handling
public class ExceptionHandling {
public static void main(String[] args) {
try {
int result = divide(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero");
} finally {
System.out.println("Execution completed.");
}
}
public static int divide(int a, int b) {
return a / b;
}
}
In the example above, the divide
method attempts to divide two integers. If the denominator is zero, the program catches the ArithmeticException
, displaying a user-friendly message.
Learning Resources
1. Official Documentation
Java's official documentation is the go-to resource for in-depth understanding. It's crucial for understanding core libraries and APIs.
2. Books
Investing in a comprehensive book can ease the learning process. Some popular choices include:
- "Effective Java" by Joshua Bloch
- "Java: The Complete Reference" by Herbert Schildt
3. Online Courses
Platforms like Coursera and edX offer structured courses that guide you through Java programming step by step.
Best Practices for Beginners
1. Write Code Daily
Practice makes perfect. Allocate time daily to write code. This persistence will reinforce concepts.
2. Collaborate and Share
Participate in coding communities like Stack Overflow or GitHub. Sharing your code and receiving feedback can immensely speed up your learning.
3. Build Projects
Start small—build applications that interest you. This tangible output will not only reinforce your learning but also enhance your portfolio.
In Conclusion, Here is What Matters
Learning Java comes with its challenges. By addressing common pitfalls, understanding critical concepts, and utilizing available resources, you can smoothly navigate your Java journey. Remember to practice, seek help when needed, and above all, remain curious.
For further reading and more tips, explore these resources:
Mastering Java is a gradual process. A steady approach will empower you to build efficient and powerful applications. Happy coding!