Why UTF-8 to ISO-8859-1 Conversion Can Break Your Data
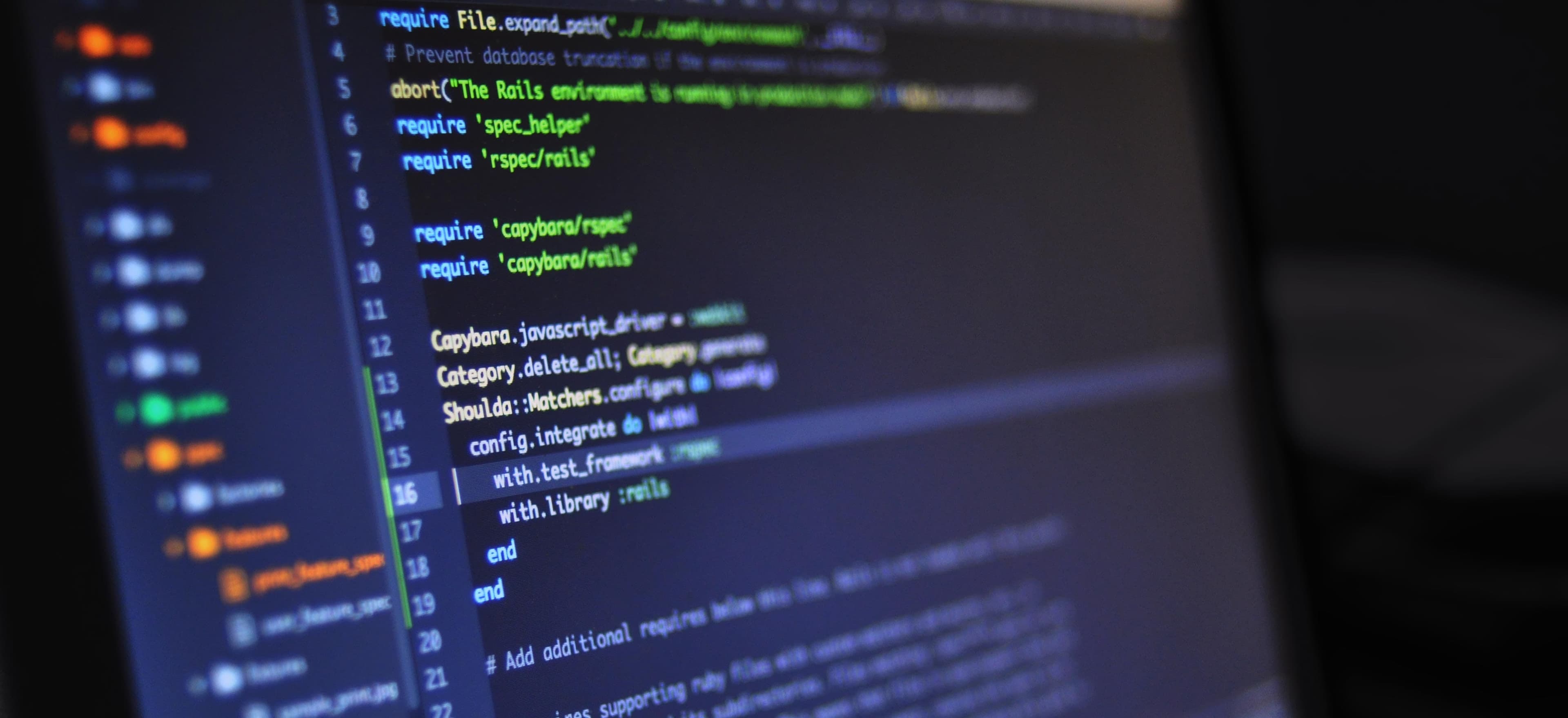
- Published on
Why UTF-8 to ISO-8859-1 Conversion Can Break Your Data
Data encoding is a critical aspect of modern computing. With numerous encoding standards available, developers often face the challenge of ensuring that text is correctly encoded and decoded across different systems. One common scenario is the conversion of UTF-8 to ISO-8859-1. While it may seem like a simple task, this conversion can lead to significant data loss or corruption if not executed carefully. In this blog post, we will explore why this happens, provide code snippets to illustrate the key points, and discuss best practices for handling character encoding.
Understanding Character Encoding
At its core, character encoding is a system that pairs each character in a given character set with a specific code point. There are various encoding schemes, but the two we will focus on are UTF-8 and ISO-8859-1.
UTF-8
UTF-8 (8-bit Unicode Transformation Format) is a variable-width character encoding capable of encoding all possible characters in Unicode. It uses one to four bytes for each character, making it extremely versatile. This flexibility allows UTF-8 to handle languages far and wide, including symbols and emoji.
ISO-8859-1
ISO-8859-1, also known as Latin-1, is a single-byte character encoding scheme that can represent the first 256 Unicode characters. It covers most Western European languages but lacks support for many characters found in other languages, especially those with accent marks or characters from non-Latin scripts.
The Problem with Conversion
When converting from UTF-8 to ISO-8859-1, problems arise primarily due to the way characters are represented. Here is a clear breakdown of potential pitfalls:
-
Character Loss: UTF-8 can encode diverse characters using multiple bytes. When these characters are forced into the single-byte ISO-8859-1 standard, there is a risk of losing important characters entirely.
-
Data Corruption: If a byte sequence from UTF-8 cannot be directly mapped to ISO-8859-1, the resulting data may either be misinterpreted or produce unexpected symbols.
Example
Let's consider a simple Java code snippet that demonstrates this issue:
import java.nio.charset.StandardCharsets;
public class CharsetConversion {
public static void main(String[] args) {
// Original string with special character
String original = "Café"; // 'é' is represented in UTF-8
// Convert to UTF-8 bytes
byte[] utf8Bytes = original.getBytes(StandardCharsets.UTF_8);
// Attempt to convert to ISO-8859-1
// This could lead to a loss of character information
String iso8859String = new String(utf8Bytes, StandardCharsets.ISO_8859_1);
// Note the potential issue here
System.out.println("UTF-8 String: " + original);
System.out.println("Converted to ISO-8859-1: " + iso8859String);
}
}
Explanation of the Code
- Original String: The variable
original
holds a UTF-8 string that includes a non-ISO-8859-1 character, 'é'. - UTF-8 Encoding: The string is converted to a byte array in UTF-8 format using
getBytes
. - ISO-8859-1 Conversion: The byte array is then converted back to a string using ISO-8859-1 encoding.
- Output: The printed output demonstrates how specific characters may corrupt or become unrecognizable.
When executing this code, you will notice that the output could be Caf?
, indicating a data loss where the 'é' character cannot be represented in ISO-8859-1.
Avoiding Data Loss
Here are some best practices to avoid data loss while converting between encoding formats:
-
Check Compatibility: Always check if the characters in your UTF-8 strings can be represented in ISO-8859-1. If you're dealing with characters outside the ISO-8859-1 range, consider maintaining them in UTF-8.
-
Use Fallback Mechanisms: If a character cannot be converted, consider using a fallback mechanism that flags or replaces these characters, like
?
or another placeholder. -
Library Support: Utilize libraries that handle character encoding more gracefully. Libraries like Apache Commons Codec offer a more robust solution for encoding issues.
-
Data Validation: Implement strict validation rules to ensure the integrity of your data post-conversion.
Improved Code Example
Here's an enhanced code snippet that checks for valid character conversion:
import java.nio.charset.StandardCharsets;
public class SafeCharsetConversion {
public static void main(String[] args) {
String original = "Café";
byte[] utf8Bytes = original.getBytes(StandardCharsets.UTF_8);
// Check if conversion is valid
String iso8859String = new String(utf8Bytes, StandardCharsets.ISO_8859_1);
// Validate if the original and converted strings match
if (!original.equals(iso8859String)) {
System.out.println("Warning: Data loss occurred during conversion!");
iso8859String = iso8859String.replace('?', '�'); // Optional: indicate an unrecognized character
}
System.out.println("UTF-8 String: " + original);
System.out.println("Converted to ISO-8859-1: " + iso8859String);
}
}
Explanation of the Improvements
- Validation Check: We check if the original string matches the converted string. If not, a warning is displayed.
- Optional Replacement: If data loss occurs, an indication character ('�') could be used to show where the problem happened, helping developers understand the issue.
Final Thoughts
Understanding and managing character encoding can significantly enhance the robustness of your applications. The conversion from UTF-8 to ISO-8859-1, while simple in theory, can introduce challenges if not handled correctly. By utilizing the best practices highlighted in this post, developers can avoid common pitfalls and ensure that their data remains intact and reliable.
For further reading on character encodings, check out Wikipedia’s page on Character Encodings and the Oracle Java Documentation.
By keeping these principles in mind, you will be better equipped to navigate the complexities of character encoding, thereby preserving data integrity in your applications.