Ensuring JavaFX UI Responsiveness: Common Pitfalls
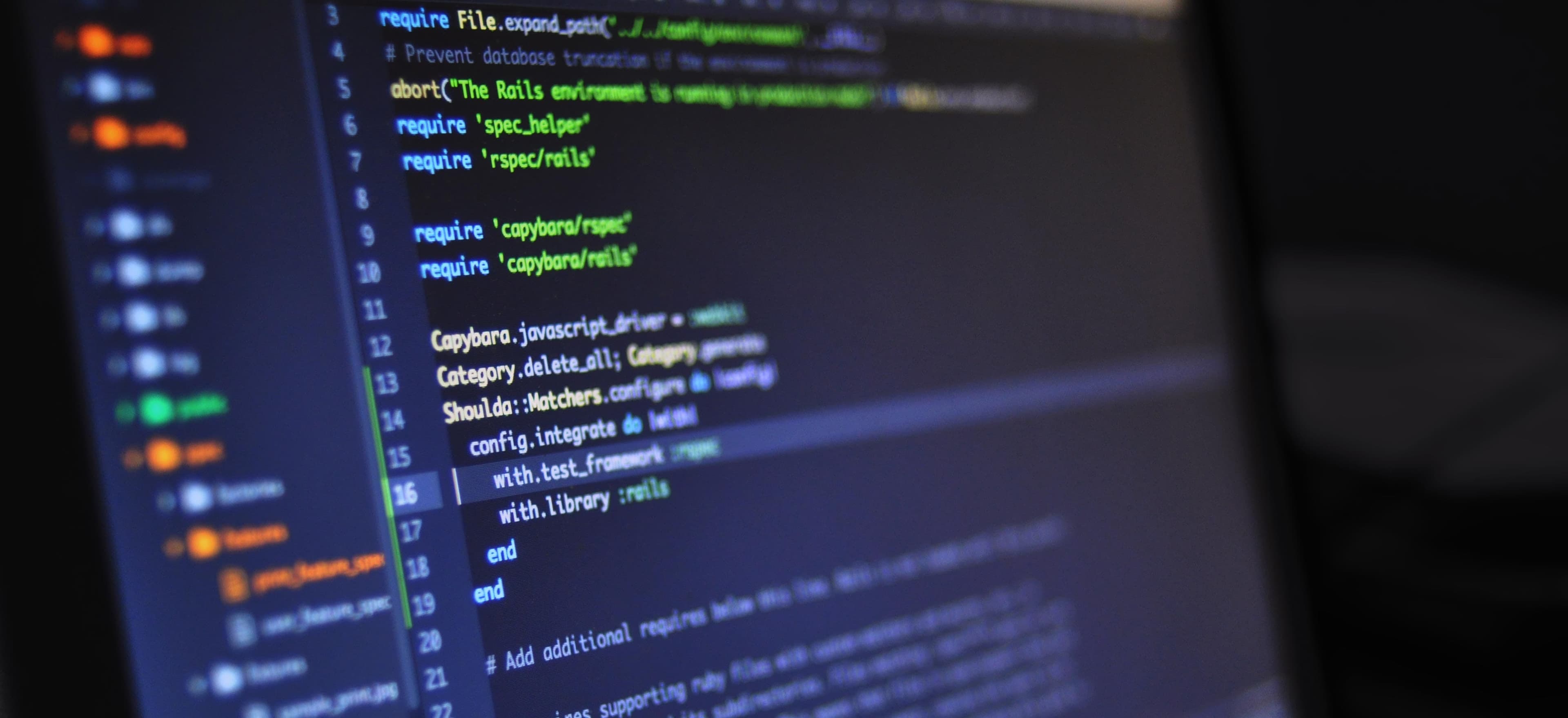
- Published on
Ensuring JavaFX UI Responsiveness: Common Pitfalls
JavaFX offers powerful features for creating rich user interfaces in Java applications. However, the challenges of maintaining a responsive UI often lead to pitfalls that developers must navigate. In this article, we will explore common mistakes that can hinder your JavaFX application's responsiveness and provide practical solutions.
Why UI Responsiveness Matters
A responsive UI is crucial for user experience. Users expect smooth interactions, quick feedback, and an interface that remains functional even during heavy processing. When an application becomes unresponsive, it can frustrate users and lead to decreased satisfaction and engagement.
Understanding the JavaFX Thread Model
Before diving into specific pitfalls, it is essential to understand how JavaFX operates. JavaFX applications run on a single thread known as the JavaFX Application Thread. This thread is responsible for handling user interface events and rendering the UI.
If you perform long-running tasks on the JavaFX Application Thread, your application will freeze. To tackle this, heavy tasks should be delegated to a background thread, allowing the JavaFX Application Thread to remain responsive.
Common Pitfalls and Solutions
Pitfall 1: Long-Running Tasks on the UI Thread
One of the most frequent mistakes is performing time-consuming operations on the UI thread. This includes tasks such as network requests, file I/O, or intensive calculations.
Example Code Snippet
import javafx.application.Application;
import javafx.concurrent.Task;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ResponsiveUIExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Start Task");
button.setOnAction(e -> {
button.setDisable(true); // Disable the button to prevent multiple clicks
startLongRunningTask(button);
});
StackPane root = new StackPane(button);
primaryStage.setScene(new Scene(root, 300, 250));
primaryStage.setTitle("Responsive UI Example");
primaryStage.show();
}
private void startLongRunningTask(Button button) {
Task<Void> task = new Task<Void>() {
@Override
protected Void call() throws Exception {
// Simulate a long-running task
Thread.sleep(5000);
return null;
}
@Override
protected void succeeded() {
button.setDisable(false); // Re-enable button upon success
}
};
new Thread(task).start(); // Start the task in a new thread
}
public static void main(String[] args) {
launch(args);
}
}
In this example, when a button is clicked, a time-consuming task is started in a new thread. It keeps the UI responsive and disables the button until the task completes.
Pitfall 2: Excessive Use of Binding
JavaFX’s property binding allows dynamic updates to the UI. However, improper use can lead to performance issues. For example, if too many bindings are created or complex calculations are done during binding updates, the application can lag.
Solution
Use bindings judiciously, and avoid complex operations within bindings. Instead, calculate values in separate methods or properties and bind simpler expressions.
Pitfall 3: Ignoring Platform.runLater
When you need to update UI components from a background thread, it's critical to do so on the JavaFX Application Thread. This is where Platform.runLater
proves invaluable.
Example Code Snippet
import javafx.application.Application;
import javafx.application.Platform;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class UpdateUIExample extends Application {
private Label label;
@Override
public void start(Stage primaryStage) {
label = new Label("Waiting for updates...");
StackPane root = new StackPane(label);
primaryStage.setScene(new Scene(root, 300, 250));
primaryStage.setTitle("Update UI Example");
primaryStage.show();
new Thread(() -> {
try {
Thread.sleep(3000); // Simulate background work
Platform.runLater(() -> label.setText("Updated from background thread!")); // Update UI on JavaFX thread
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
}
public static void main(String[] args) {
launch(args);
}
}
In this example, the label is updated from a background thread safely using Platform.runLater
, ensuring the UI remains responsive.
Pitfall 4: Unoptimized Resource Loading
Loading images, stylesheets, or other resources can also block the UI thread if done synchronously. This can cause noticeable delays in the UI.
Solution
Load resources in the background, or use lazy loading strategies. For instance, load images only when they become visible.
Pitfall 5: Inefficient UI Updates and Redrawing
Frequent updates to UI elements can lead to performance degradation. For instance, updating a scene graph multiple times in quick succession can cause flickering and a sluggish response.
Solution
Batch UI updates whenever possible. For example, update multiple properties at once rather than one at a time.
In Conclusion, Here is What Matters
Maintaining a responsive JavaFX UI is critical for user satisfaction. By avoiding common pitfalls such as executing long-running tasks on the UI thread, misusing bindings, neglecting thread safety when updating UI components, ignoring resource loading optimizations, and minimizing excessive updates, developers will create smoother, more enjoyable user experiences.
For more advanced JavaFX techniques, consider Referencing the Official JavaFX Documentation, which provides comprehensive information and examples.
By adhering to the principles discussed in this article, developers will significantly enhance the responsiveness of their JavaFX applications, leading to improved user experience and overall satisfaction. Happy coding!