Mastering Java Process Size Monitoring on IBM AIX
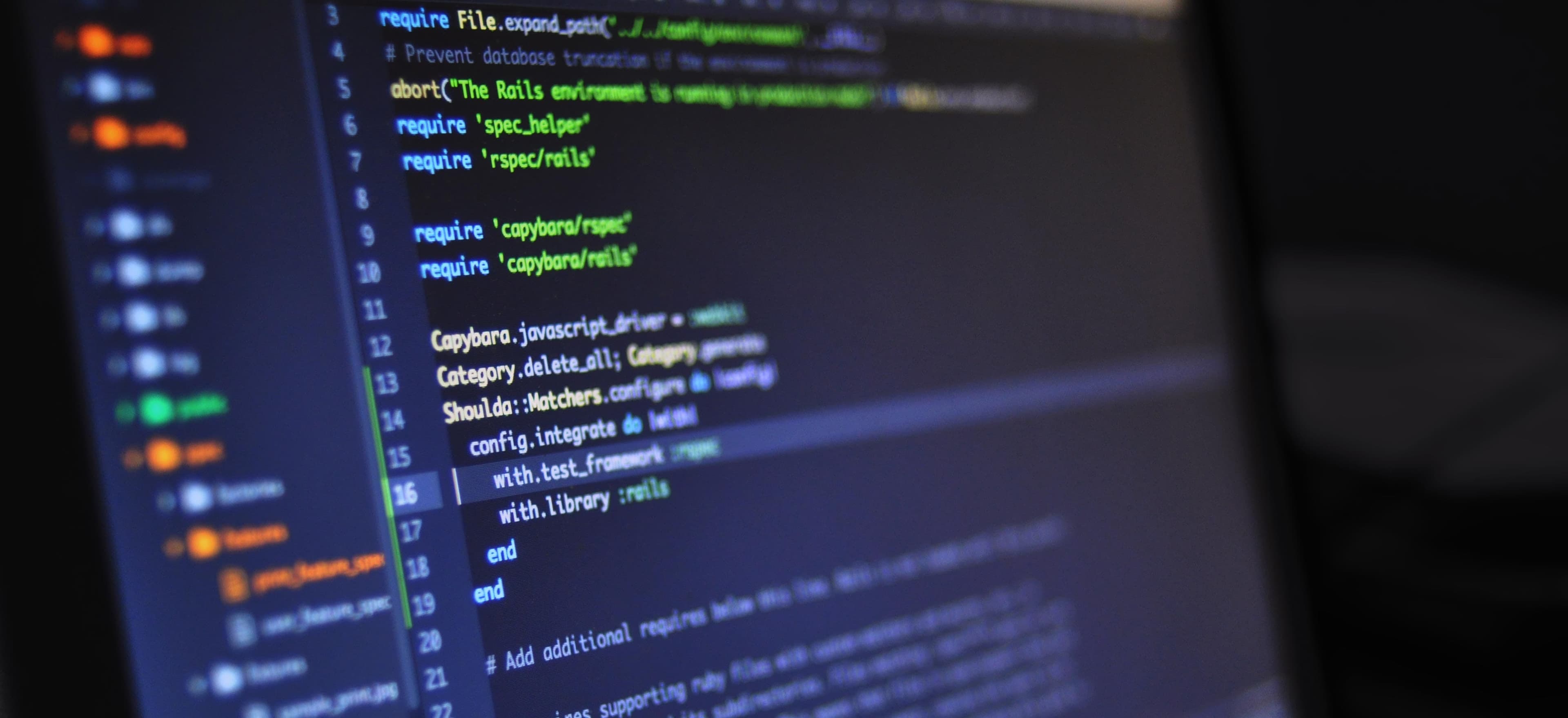
- Published on
Mastering Java Process Size Monitoring on IBM AIX
As organizations increasingly rely on Java applications to handle complex tasks, efficient process management has become paramount, especially in enterprise environments like IBM AIX. Monitoring Java process sizes not only helps in optimizing memory usage but also ensures that applications run smoothly without excessive resource consumption.
In this blog post, we will cover:
- Fundamental concepts in Java memory management
- Techniques for monitoring Java process sizes on IBM AIX
- Code snippets with commentary to illustrate practical implementation
- Tools and resources for effective monitoring
Understanding Java Memory Management
Java memory management is an essential concept that developers need to grasp. The Java Virtual Machine (JVM) allocates memory for various components of a Java application, primarily as follows:
- Heap Memory: This is where Java objects are stored. The size of the heap is crucial for performance.
- Stack Memory: This memory is used for the execution of threads. Each thread has its own stack.
- Method Area: This stores class structures like metadata, constants, and static variables.
Understanding how the JVM utilizes memory will allow you to better monitor and control resource usage.
Monitoring Java Process Sizes on IBM AIX
Using the ps
Command
One of the simplest ways to view the process size on AIX is by using the ps
command. The following command gives you details about Java processes running on the system:
ps -ef | grep java
This command displays all processes with 'java' in their names. The output includes PID, CPU usage, and memory consumption.
Leveraging jstat
Java ships with a utility called jstat
, which provides information about the performance of the JVM.
Example Usage:
jstat -gcutil <pid> <interval> <count>
This observes garbage collection statistics. Here’s the breakdown:
<pid>
: The process ID of the Java application.<interval>
: How often to output the data (in milliseconds).<count>
: The number of times to repeat the output.
Explanation of Output:
This command gives you information on different memory spaces, including young and old generations. Monitoring generations' usage assists in understanding how effective garbage collection is.
Utilizing VisualVM
For a graphical interface, VisualVM is an excellent tool. It extracts detailed insights about heap memory, thread states, and CPU usage directly from the JVM.
- Installation: Follow instructions provided on the VisualVM website.
- Connecting to AIX: Ensure that both VisualVM and your AIX server are reachable over the network.
Code Snippet: Implementing a Memory Monitor
To actively monitor memory usage during runtime, consider integrating a custom memory monitor within your Java application. Below is an example of a simple Java class that logs memory usage:
import java.lang.management.ManagementFactory;
import java.lang.management.MemoryMXBean;
import java.lang.management.MemoryUsage;
public class MemoryMonitor {
private final MemoryMXBean memoryMXBean;
public MemoryMonitor() {
memoryMXBean = ManagementFactory.getMemoryMXBean();
}
public void logMemoryUsage() {
MemoryUsage heapMemoryUsage = memoryMXBean.getHeapMemoryUsage();
MemoryUsage nonHeapMemoryUsage = memoryMXBean.getNonHeapMemoryUsage();
System.out.println("Heap Memory Usage: " + heapMemoryUsage.getUsed() / (1024 * 1024) + " MB");
System.out.println("Non-Heap Memory Usage: " + nonHeapMemoryUsage.getUsed() / (1024 * 1024) + " MB");
}
public static void main(String[] args) {
MemoryMonitor monitor = new MemoryMonitor();
// Log memory usage every 5 seconds
while (true) {
monitor.logMemoryUsage();
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
Why This Code Matters
This code continuously monitors and logs heap and non-heap memory usage every 5 seconds. The insights gained from this can help developers optimize memory handling and detect potential memory leaks. Key benefits include:
- Real-Time Monitoring: Helps in taking immediate actions if memory consumption spikes unexpectedly.
- Custom Notifications: You can easily enhance the logging mechanism to integrate with alert systems, like sending notifications when memory utilization crosses a threshold.
Best Practices for Java Monitoring on AIX
-
Regular Monitoring: Consistently track your application's memory usage. Stagnation in heap memory can signal potential memory leaks.
-
Testing Under Load: Always test memory performance under simulated load conditions. This helps in identifying bottlenecks that can cause performance degradation.
-
Tune Garbage Collection: Sometimes, adjusting JVM options for garbage collection can prevent memory issues. Use parameters like
-XX:+UseG1GC
or-Xms
and-Xmx
to define initial and maximum heap sizes.
Final Thoughts
Monitoring Java process sizes on IBM AIX is a vital part of maintaining robust applications in today's technological landscape. Whether you prefer command-line tools like ps
, jstat
, or graphical interfaces like VisualVM, understanding how to extract and analyze this information will greatly enhance your application management skills.
For deeper insights into JVM tuning, refer to the Oracle documentation on JVM options.
With the techniques and tools discussed, you are well-equipped to master Java process size monitoring on IBM AIX. Continue exploring your application performance, and you will maintain a competitive edge in developing scalable and efficient Java applications.