Mastering Dynamodb Mapper: Common Mapping Pitfalls
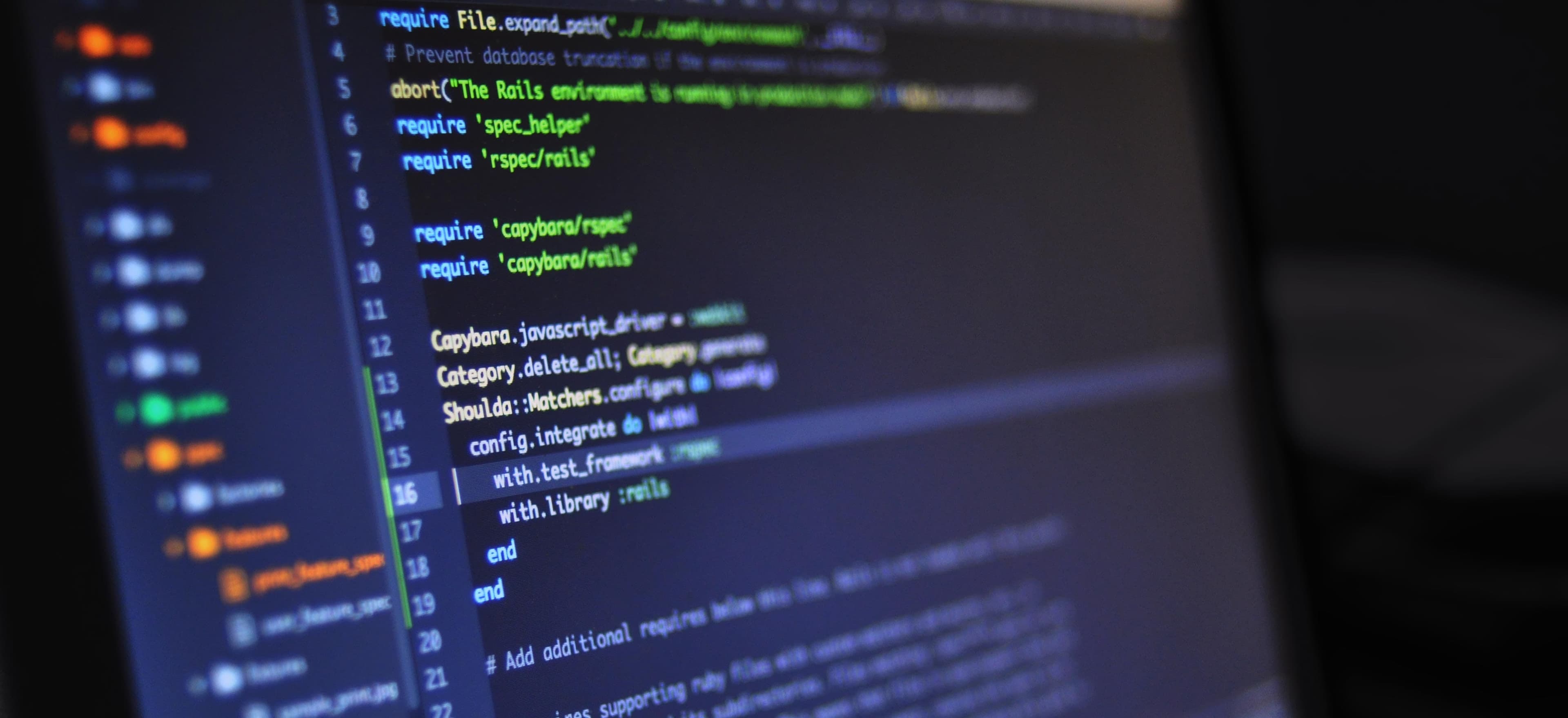
- Published on
Mastering DynamoDB Mapper: Common Mapping Pitfalls
Amazon DynamoDB is a fully managed NoSQL database service that provides fast and predictable performance with seamless scalability. With its ability to handle large amounts of data and high-traffic applications, it has become a preferred choice for developers. However, as with any technology, working with DynamoDB can bring certain challenges. One such challenge lies in the mapping of objects to DynamoDB items using the DynamoDB Mapper. In this blog post, we will explore common mapping pitfalls faced by developers and how to master them.
What is DynamoDB Mapper?
The DynamoDB Mapper is a high-level Java API that simplifies the interaction with DynamoDB. This object persistence framework allows developers to map their Java objects to DynamoDB items effortlessly. It abstracts the complexities of data modeling and the underlying query syntax while providing annotations to define specific behaviors.
Setting Up DynamoDB Mapper
To use DynamoDB Mapper in your Java applications, you will need to include the AWS SDK. Ensure you have the SDK in your project dependencies. If you're using Maven, include the following in your pom.xml
:
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk-dynamodb</artifactId>
<version>1.12.300</version>
</dependency>
After adding the dependency, you can initialize the DynamoDB client like this:
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB;
import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder;
import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBMapper;
public class DynamoDBConfig {
private final AmazonDynamoDB amazonDynamoDB;
private final DynamoDBMapper dynamoDBMapper;
public DynamoDBConfig() {
this.amazonDynamoDB = AmazonDynamoDBClientBuilder.standard().build();
this.dynamoDBMapper = new DynamoDBMapper(amazonDynamoDB);
}
public DynamoDBMapper getDynamoDBMapper() {
return dynamoDBMapper;
}
}
This sets the stage for using DynamoDB Mapper. Now, let's dive into some common mapping pitfalls.
Common Mapping Pitfalls
1. Incorrect Annotations
The first and most critical pitfall occurs when developers misuse annotations. Sometimes, using inappropriate or missing annotations can lead to unexpected behavior. Key annotations include @DynamoDBTable
, @DynamoDBHashKey
, and @DynamoDBRangeKey
.
Example
import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBHashKey;
import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBTable;
@DynamoDBTable(tableName = "User")
public class User {
private String userId;
private String name;
@DynamoDBHashKey
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Why It's Important: Properly annotating key attributes ensures DynamoDB Mapper understands how to uniquely identify each item. Without these annotations, your items might not persist or behave as expected.
2. Ignoring Data Types
DynamoDB is schema-less, which means you can use various data types within your attributes. However, if you fail to use the correct Java types, it could result in data being saved incorrectly.
Example
public class User {
private String userId;
private Integer age; // Integer expected here
@DynamoDBHashKey
public String getUserId() {
return userId;
}
@DynamoDBAttribute
public Integer getAge() {
return age;
}
}
Why It's Important: DynamoDB stores data based on types. If you attempt to store a String when an Integer is expected, it results in runtime exceptions or loss of data integrity. Always double-check the data types between Java and DynamoDB.
3. Incomplete Getters and Setters
For the Mapper to work effectively, all properties that need to be persisted in the database must have their corresponding getters and setters. Missing these can lead to incomplete data being saved.
Example
import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBAttribute;
public class User {
private String userId;
private String email; // Missing getter and setter
@DynamoDBHashKey
public String getUserId() {
return userId;
}
}
Why It's Important: Failing to have a getter/setter pair means that the data cannot be serialized/deserialized properly, leading to lost attributes. Complete your classes to cover all fields.
4. Overlooking Consistency
DynamoDB offers two consistency models: eventual and strong consistency. It's essential to understand which model to use when reading data.
Example
User user = dynamoDBMapper.load(User.class, userId);
The above line loads the user item using the eventual consistency model by default.
Why It's Important: If your application relies on reading the most up-to-date information, you should use strong consistency. Here’s how:
DynamoDBMapperConfig config = DynamoDBMapperConfig.builder()
.withConsistentReads(DynamoDBMapperConfig.CONSISTENT_READS)
.build();
User user = dynamoDBMapper.load(User.class, userId, config);
Understanding and correctly applying the consistency models is crucial for the integrity of your application’s data reads.
5. Not Managing Versioning
When dealing with highly dynamic data, managing versions of your items becomes essential. DynamoDB offers a feature called optimistic locking.
Example
import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBVersionAttribute;
public class User {
private String userId;
private Long version; // Version attribute for optimistic locking
@DynamoDBHashKey
public String getUserId() {
return userId;
}
@DynamoDBVersionAttribute
public Long getVersion() {
return version;
}
}
Why It's Important: Implementing versioning allows you to prevent lost updates in concurrent environments. If two processes attempt to update the same item simultaneously, the one with the older version will fail, maintaining data integrity.
6. Failing to Handle Null Values
DynamoDB doesn’t store attributes that are null. If you fail to handle null values properly, you might end up with incomplete data.
Example
public class User {
private String userId;
private String address; // Can be null
@DynamoDBHashKey
public String getUserId() {
return userId;
}
@DynamoDBAttribute
public String getAddress() {
return address;
}
}
Why It's Important: If address
is not set, it won't be stored in DynamoDB. Be proactive in managing optional fields to maintain data integrity and consistency.
Final Considerations
Mastering the DynamoDB Mapper is crucial for developers looking to leverage the power of DynamoDB in their applications. By avoiding common pitfalls—such as improper annotations, ignoring data types, incomplete getter/setter methods, inconsistency, lack of version control, and the handling of null values—you can ensure the smooth operation of your data layer.
For additional reading on DynamoDB best practices, check AWS’s official documentation or this comprehensive guide on object mapping.
By applying these principles, you will not only enhance your understanding of the DynamoDB Mapper but also improve the overall resilience and reliability of your applications. Happy coding!