Common Pitfalls in Spring Integration Message Processing
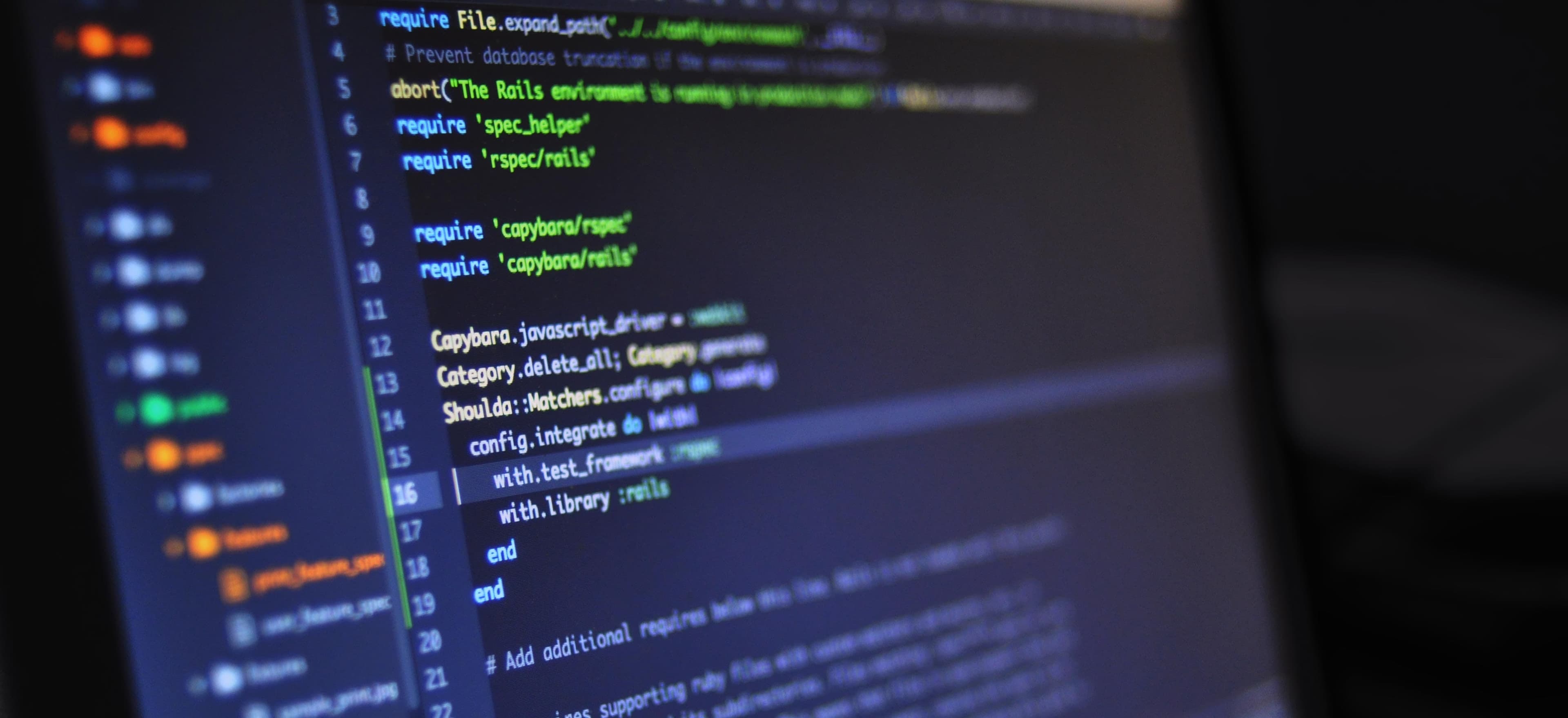
- Published on
Common Pitfalls in Spring Integration Message Processing
Spring Integration is a powerful module of the Spring framework that facilitates messaging and integration patterns. However, like any technology, it can be tricky to navigate. This blog post will cover some common pitfalls in Spring Integration message processing and how to avoid them. Whether you're a beginner or an experienced user, understanding these pitfalls can help streamline your workflow and improve overall application performance.
What is Spring Integration?
Before diving into the pitfalls, let’s briefly summarize what Spring Integration is. Spring Integration provides a framework for building messaging applications using the principles of Enterprise Integration Patterns. It allows developers to connect different applications and services via message channels, handling message flow, routing, and transformation effectively.
For additional context, you can check the Spring Integration Documentation for a deeper understanding.
Pitfall 1: Ignoring Message Metadata
One of the most significant oversights is neglecting message metadata. When processing messages, it’s essential to include relevant metadata to ensure that downstream components can make informed decisions.
Example: Adding Metadata to Message
public Message<String> enhanceMessage(String payload) {
MessageHeaders headers = new MessageHeaders();
headers.put("messageId", UUID.randomUUID().toString());
headers.put("timestamp", System.currentTimeMillis());
return MessageBuilder.withPayload(payload)
.setHeaders(headers)
.build();
}
Why This Matters
Including metadata helps in tracking messages and understanding their context. This information can be crucial for debugging and monitoring. When messages are sent across different systems, the metadata can elucidate the state and purpose, thus enhancing traceability.
Pitfall 2: Failing to Handle Error Messages
Error handling is essential in message-driven systems, yet many developers underestimate its importance. If you fail to define a clear error-handling strategy, you may encounter unprocessed messages or lost data.
Example: Defining an Error Channel
<int:channel id="errorChannel"/>
<int:service-activator input-channel="errorChannel" ref="errorHandler" method="handleError"/>
Why This Matters
Having a dedicated error channel allows you to manage errors effectively. It prevents error messages from getting lost and provides an opportunity to analyze, log, or retry failed processes. Always prepare for failures—it's not a question of if they will occur, but when.
Pitfall 3: Hardcoding Configuration
While it may be tempting to hardcode your configuration values directly into code, this practice can lead to maintenance headaches. Hardcoding can limit the flexibility of your application and make it more challenging to adapt to changing requirements.
Example: Externalizing Configuration Using Application Properties
message.queue.name=myQueue
message.retry.count=5
@Value("${message.queue.name}")
private String queueName;
@Value("${message.retry.count}")
private int retryCount;
Why This Matters
Externalizing configuration helps keep your application adaptable and maintainable. It enables dynamic changes without recompiling code. Furthermore, using Spring's dependency injection facilitates testing with different configurations effortlessly.
Pitfall 4: Inefficient Message Processing
Performance can degrade if message processing is handled inefficiently. A common mistake is not considering the nature of your workload, leading to bottlenecks in message throughput.
Example: Using Asynchronous Messaging
<int:gateway id="messageGateway" service-interface="MessageGateway" default-request-channel="inputChannel" default-reply-channel="outputChannel"/>
<int:service-activator input-channel="inputChannel" ref="messageProcessor" method="processMessage">
<int:dispatchers async="true"/>
</int:service-activator>
Why This Matters
Asynchronous message processing can significantly increase throughput, particularly in high-load scenarios. By allowing messages to be processed in parallel, you reduce waiting time and keep the system responsive.
Pitfall 5: Lack of Documentation and Monitoring
Documentation and monitoring are often sidelined, but they can save you a lot of trouble in the long run. Failing to document the message flow and processing logic can create confusion, while insufficient monitoring can lead to undetected issues.
Example: Logging Message Flow
public void processMessage(Message<String> message) {
log.info("Processing message: " + message.getPayload());
// Process message
}
Why This Matters
Clear documentation aids both current developers and future maintainers in understanding the message flow and makes onboarding new team members easier. Moreover, implementing robust monitoring allows you to catch issues early, enhancing the reliability of your system.
Pitfall 6: Overcomplicating Message Transformation
Spring Integration provides various ways to transform messages, but overcomplicating these transformations can lead to unclear code and slow processing. It’s vital to keep transformations as simple as possible.
Example: Using a Simple Transformer
<int:transformer input-channel="inputChannel" output-channel="outputChannel">
<int:json-to-object-transformer target-type="com.example.MyObject"/>
</int:transformer>
Why This Matters
By leveraging Spring’s built-in transformation capabilities, you can maintain clarity and efficiency. Overly complex transformations can introduce errors and performance issues, making your application harder to maintain.
Lessons Learned
Spring Integration offers a comprehensive suite for building robust messaging applications, but it is not without its pitfalls. Recognizing and avoiding these common mistakes can enhance your message processing effectiveness and overall application performance. Remember to include message metadata, handle errors diligently, externalize configuration, process messages efficiently, document your flows, and keep transformations simple.
By following these best practices, you will find that your applications are more maintainable and scalable. Always stay updated with the Spring Integration Guide for new features and optimization strategies.
Implementing these strategies may lead to a better development experience and, most importantly, improved results for your applications. Happy coding!
Checkout our other articles