Common Pitfalls in Architectural Patterns Beginners Face
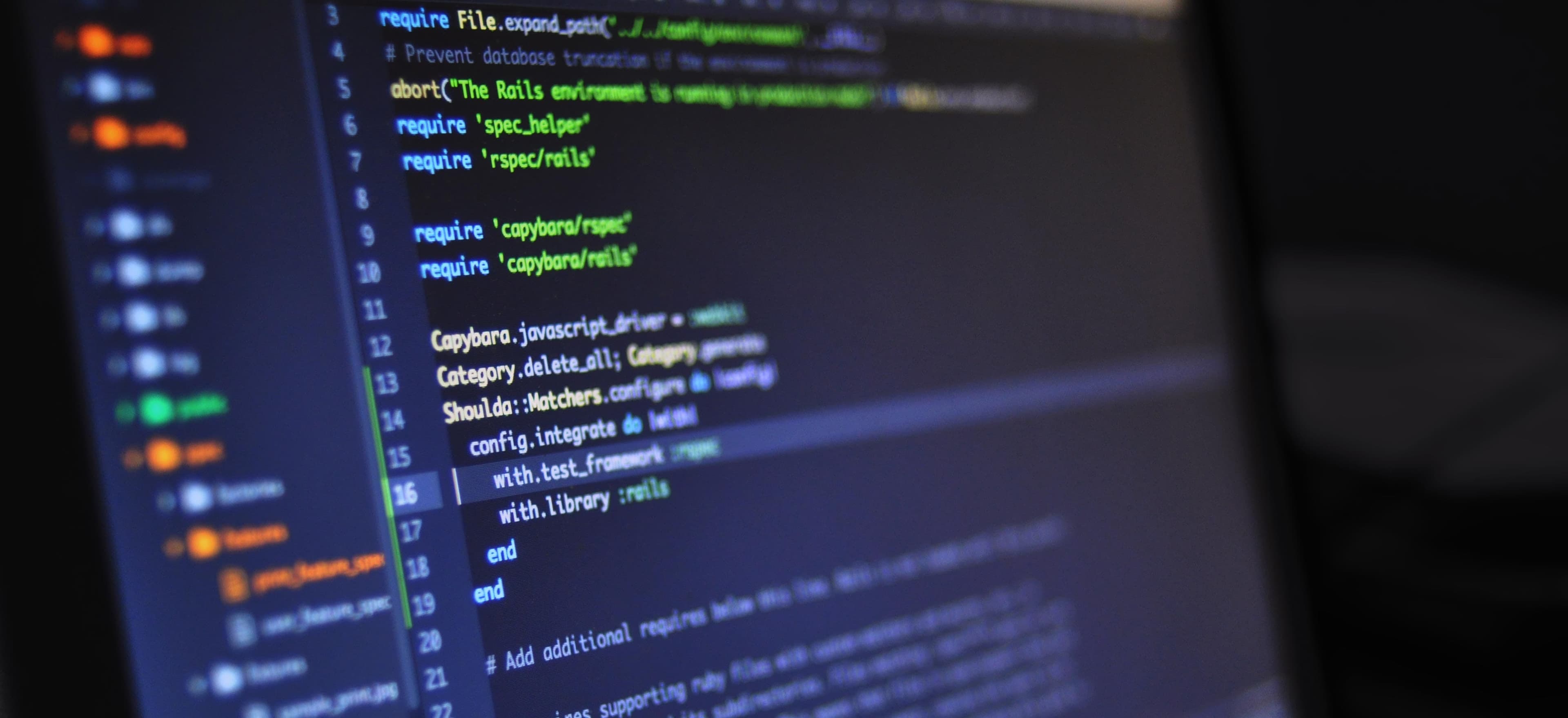
- Published on
Common Pitfalls in Architectural Patterns Beginners Face
Architectural patterns serve as guides that engineers can leverage when designing and deploying software systems. These patterns provide a sensible structure, promoting maintainability, scalability, and robustness in applications. However, newcomers to software architecture often encounter challenges that hinder their understanding and adoption of these patterns. In this blog post, we will explore some common pitfalls beginners face, explain why they occur, and delve into best practices to avoid them.
1. Over-Engineering Solutions
Understanding the Problem
One of the most prevalent mistakes that beginners make is over-engineering their solutions. This involves adding unnecessary complexity to a system by incorporating numerous architectural patterns when simpler solutions could suffice.
Example
For example, a beginner might decide to implement a microservices architecture for a small application that could have been effectively managed using a monolithic approach.
public class User {
private String name;
private String email;
// Constructor
public User(String name, String email) {
this.name = name;
this.email = email;
}
// Getters
public String getName() {
return name;
}
public String getEmail() {
return email;
}
}
In this case, using a simple class to represent a user is straightforward and effective. However, complicating the architecture by adding layers for service discovery, message brokers, and docker orchestration makes it harder to manage.
Best Practices
- Evaluate Simplicity: Always start simple and only add complexity as it is needed.
- Focus on Requirements: Design your architecture based on the current needs of your application, not on potential future requirements.
2. Ignoring Technical Debt
The Consequences of Neglect
Technical debt refers to the implied cost of additional rework caused by choosing an easy solution now instead of a better approach that would take longer. Beginners often overlook this issue, leading to cumbersome codebases that become harder to manage over time.
Reflection in Code
When developers ignore the importance of clean code and design principles, they may end up with poor-quality code like this:
public void process() {
// Multiple responsibilities mixed
for (Object obj : data) {
if (obj instanceof String) {
System.out.println((String) obj);
} else if (obj instanceof Integer) {
System.out.println((Integer) obj);
}
// Code duplication and mixed responsibilities
}
}
Best Practices
- Refactor Regularly: Regular code reviews and refactoring can help manage technical debt before it becomes unmanageable.
- Define Coding Standards: Establish clear standards for coding practices within your team to ensure uniformity and quality.
3. Underestimating Scalability
The Importance of Scalability
Scalability is critical in architectural design, and beginners often overlook this aspect, leading to performance bottlenecks as user demand grows.
Illustrative Example
Starting with a single database instance for storing all data may work initially, but it can cause significant issues later when the number of users increases.
Best Practices
- Plan for Growth: Consider deploying a distributed database architecture early on, even if you're initially using a single database.
- Load Testing: Implement load testing early in the development cycle to identify potential scalability issues.
4. Lack of Clear Boundaries
Importance of Defining Boundaries
In designs such as microservices architectures, defining boundaries is essential. Beginners often create services that overlap in responsibilities, leading to tightly coupled systems that are hard to maintain.
Example Code
For instance, if both a user service and an order service handle user information, this overlap can lead to inconsistency.
public class UserService {
public void createUser(String name) {
// User creation logic
}
}
public class OrderService {
public void createOrder(String userName) {
UserService userService = new UserService();
userService.createUser(userName); // Bad practice of direct coupling
}
}
Best Practices
- Define Responsibilities: Clearly outline what each service should do. This prevents overlaps and maintains a clear focus.
- Use API Contracts: Set up API contracts for inter-service communication to avoid direct dependencies.
5. Neglecting Documentation
The Cost of Poor Documentation
Good documentation can make a significant difference in the maintainability and understanding of an architectural pattern. Beginners often neglect this, which can create confusion later on for themselves or other developers.
The Risks
Without documentation, even straightforward architecting choices can lead newer team members to misinterpret the structure, leading to erroneous implementations.
Best Practices
- Document Everything: Maintain thorough documentation for your architecture, including diagrams and explanations of design choices.
- Update Regularly: Ensure documentation evolves alongside your code to stay relevant.
6. Disregarding Error Handling
The Importance of Robust Error Management
In any architectural pattern, error handling is crucial. However, beginners often overlook it, leading to systems that crumble at the first sign of trouble.
Example Code
A lack of error handling could look like this:
public void processOrder(Order order) {
PaymentService paymentService = new PaymentService();
paymentService.process(order.getPayment()); // No exception handling
}
Best Practices
- Anticipate Failures: Implement comprehensive error handling to manage potential failures gracefully.
- Use Logging: Proper logging mechanisms help identify and rectify errors swiftly.
Final Thoughts
Architectural patterns are indispensable tools for software engineers, but they can pose significant challenges, particularly for beginners. By understanding common pitfalls and adhering to best practices, newcomers can build more maintainable, scalable, and robust systems.
Further Reading
If you are interested in diving deeper into software architecture, consider checking out Martin Fowler's website for a wealth of resources or explore Microservices.io for specialized content on microservices.
This post arms you with the insights you need to steer clear of common pitfalls in architectural patterns. As you embark on your journey through software architecture, remember: start simple, refactor often, and prioritize understanding the 'why' behind the patterns you choose to implement.
Checkout our other articles